Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / Dom / XmlText.cs / 1 / XmlText.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.Text; using System.Diagnostics; using System.Xml.XPath; // Represents the text content of an element or attribute. public class XmlText : XmlCharacterData { internal XmlText( string strData ): this( strData, null ) { } protected internal XmlText( string strData, XmlDocument doc ): base( strData, doc ) { } // Gets the name of the node. public override String Name { get { return OwnerDocument.strTextName; } } // Gets the name of the current node without the namespace prefix. public override String LocalName { get { return OwnerDocument.strTextName; } } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.Text; } } public override XmlNode ParentNode { get { switch (parentNode.NodeType) { case XmlNodeType.Document: return null; case XmlNodeType.Text: case XmlNodeType.CDATA: case XmlNodeType.Whitespace: case XmlNodeType.SignificantWhitespace: XmlNode parent = parentNode.parentNode; while (parent.IsText) { parent = parent.parentNode; } return parent; default: return parentNode; } } } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateTextNode( Data ); } public override String Value { get { return Data; } set { Data = value; XmlNode parent = parentNode; if ( parent != null && parent.NodeType == XmlNodeType.Attribute ) { XmlUnspecifiedAttribute attr = parent as XmlUnspecifiedAttribute; if ( attr != null && !attr.Specified ) { attr.SetSpecified( true ); } } } } // Splits the node into two nodes at the specified offset, keeping // both in the tree as siblings. public virtual XmlText SplitText(int offset) { XmlNode parentNode = this.ParentNode; int length = this.Length; if( offset > length ) throw new ArgumentOutOfRangeException( "offset" ); //if the text node is out of the living tree, throw exception. if ( parentNode == null ) throw new InvalidOperationException(Res.GetString(Res.Xdom_TextNode_SplitText)); int count = length - offset; String splitData = Substring(offset, count); DeleteData(offset, count); XmlText newTextNode = OwnerDocument.CreateTextNode(splitData); parentNode.InsertAfter(newTextNode, this); return newTextNode; } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteString(Data); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing } internal override XPathNodeType XPNodeType { get { return XPathNodeType.Text; } } internal override bool IsText { get { return true; } } internal override XmlNode PreviousText { get { if (parentNode.IsText) { return parentNode; } return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.Text; using System.Diagnostics; using System.Xml.XPath; // Represents the text content of an element or attribute. public class XmlText : XmlCharacterData { internal XmlText( string strData ): this( strData, null ) { } protected internal XmlText( string strData, XmlDocument doc ): base( strData, doc ) { } // Gets the name of the node. public override String Name { get { return OwnerDocument.strTextName; } } // Gets the name of the current node without the namespace prefix. public override String LocalName { get { return OwnerDocument.strTextName; } } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.Text; } } public override XmlNode ParentNode { get { switch (parentNode.NodeType) { case XmlNodeType.Document: return null; case XmlNodeType.Text: case XmlNodeType.CDATA: case XmlNodeType.Whitespace: case XmlNodeType.SignificantWhitespace: XmlNode parent = parentNode.parentNode; while (parent.IsText) { parent = parent.parentNode; } return parent; default: return parentNode; } } } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateTextNode( Data ); } public override String Value { get { return Data; } set { Data = value; XmlNode parent = parentNode; if ( parent != null && parent.NodeType == XmlNodeType.Attribute ) { XmlUnspecifiedAttribute attr = parent as XmlUnspecifiedAttribute; if ( attr != null && !attr.Specified ) { attr.SetSpecified( true ); } } } } // Splits the node into two nodes at the specified offset, keeping // both in the tree as siblings. public virtual XmlText SplitText(int offset) { XmlNode parentNode = this.ParentNode; int length = this.Length; if( offset > length ) throw new ArgumentOutOfRangeException( "offset" ); //if the text node is out of the living tree, throw exception. if ( parentNode == null ) throw new InvalidOperationException(Res.GetString(Res.Xdom_TextNode_SplitText)); int count = length - offset; String splitData = Substring(offset, count); DeleteData(offset, count); XmlText newTextNode = OwnerDocument.CreateTextNode(splitData); parentNode.InsertAfter(newTextNode, this); return newTextNode; } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteString(Data); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing } internal override XPathNodeType XPNodeType { get { return XPathNodeType.Text; } } internal override bool IsText { get { return true; } } internal override XmlNode PreviousText { get { if (parentNode.IsText) { return parentNode; } return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
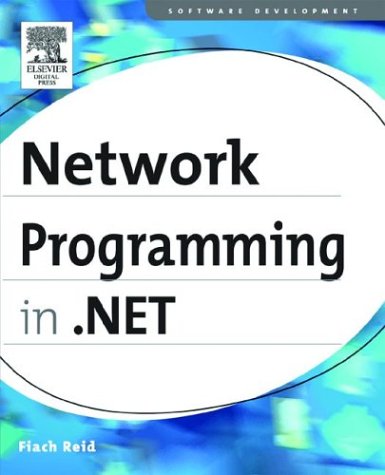
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColorKeyFrameCollection.cs
- ListBoxItemAutomationPeer.cs
- FieldAccessException.cs
- DoubleCollectionConverter.cs
- DependencyPropertyHelper.cs
- UnmanagedMarshal.cs
- SignatureHelper.cs
- DbConnectionPoolGroup.cs
- TdsParserStateObject.cs
- HMACSHA1.cs
- MenuItemBinding.cs
- HttpWebResponse.cs
- TrackingLocation.cs
- DesignerDataStoredProcedure.cs
- ApplyHostConfigurationBehavior.cs
- ConfigXmlElement.cs
- StrokeCollection2.cs
- CommentEmitter.cs
- ListSourceHelper.cs
- LocalizationComments.cs
- UpdatePanelTrigger.cs
- AlgoModule.cs
- processwaithandle.cs
- ColorKeyFrameCollection.cs
- Descriptor.cs
- GridSplitterAutomationPeer.cs
- RegexNode.cs
- HttpHandlerAction.cs
- NameValuePermission.cs
- PageMediaSize.cs
- WebPartsPersonalization.cs
- ScaleTransform.cs
- RtfControlWordInfo.cs
- AnchoredBlock.cs
- DataAdapter.cs
- PriorityItem.cs
- SqlDataRecord.cs
- StreamSecurityUpgradeInitiator.cs
- WindowsGrip.cs
- TypeConverterHelper.cs
- Regex.cs
- UnionExpr.cs
- TreeViewItemAutomationPeer.cs
- ValueChangedEventManager.cs
- CommonDialog.cs
- RegexMatchCollection.cs
- CacheDependency.cs
- DataGridViewCellStyle.cs
- AssemblyFilter.cs
- ParentQuery.cs
- ScriptModule.cs
- EntityContainerEmitter.cs
- ImageCodecInfo.cs
- PolicyUtility.cs
- ReceiveContextCollection.cs
- InheritedPropertyChangedEventArgs.cs
- ResourcePermissionBaseEntry.cs
- IconConverter.cs
- UInt64Converter.cs
- TemplatePropertyEntry.cs
- HtmlControl.cs
- X509Certificate2Collection.cs
- IResourceProvider.cs
- ExpressionBindings.cs
- MiniMapControl.xaml.cs
- HtmlInputHidden.cs
- CurrentTimeZone.cs
- XmlReaderSettings.cs
- assertwrapper.cs
- ConfigXmlAttribute.cs
- DocumentViewerBase.cs
- Error.cs
- MethodSet.cs
- MergeExecutor.cs
- Image.cs
- SafeNativeMethods.cs
- COAUTHINFO.cs
- DynamicDiscoSearcher.cs
- BaseTemplateParser.cs
- ClientTargetCollection.cs
- ADRoleFactory.cs
- ScriptDescriptor.cs
- HttpRuntimeSection.cs
- GroupBoxRenderer.cs
- ReadOnlyTernaryTree.cs
- AutomationElementIdentifiers.cs
- XmlHierarchicalEnumerable.cs
- PtsContext.cs
- webclient.cs
- PropertyMetadata.cs
- VerificationException.cs
- InfoCardXmlSerializer.cs
- Utils.cs
- SafeProcessHandle.cs
- DesignerAdRotatorAdapter.cs
- IntPtr.cs
- WebHostUnsafeNativeMethods.cs
- UnSafeCharBuffer.cs
- RoleBoolean.cs
- ExpressionBindingCollection.cs