Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / SMSvcHost / System / ServiceModel / Activation / WebHostUnsafeNativeMethods.cs / 1 / WebHostUnsafeNativeMethods.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.Runtime.InteropServices; using System.Security; using System.Runtime.ConstrainedExecution; using Microsoft.Win32.SafeHandles; [SuppressUnmanagedCodeSecurityAttribute()] // functions in %windir%\system32\inetsrv\wbhstipm.dll // headers in //depot/devdiv/private/webnetorcas/ndp/iis/iisrearc/core/inc/wbhst_entry.h // implementation in //depot/devdiv/private/webnetorcas/ndp/iis/iisrearc/core/ap/wbhstipm/dll/clientprotocol.cxx static class WebHostUnsafeNativeMethods { const string KERNEL32 = "kernel32.dll"; internal const int LOAD_WITH_ALTERED_SEARCH_PATH = 0x00000008; internal delegate int WebhostGetVersion(out int major, out int minor); internal delegate void WebhostListenerApplicationAppPoolChanged(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appKey, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId); internal delegate void WebhostListenerApplicationBindingsChanged(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appKey, IntPtr bindingsMultiSz, int numberOfBindings); internal delegate void WebhostListenerApplicationCreated(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appKey, [MarshalAs(UnmanagedType.LPWStr)] string path, int siteId, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId, IntPtr bindingsMultiSz, int numberOfBindings, bool requestsBlocked); internal delegate void WebhostListenerApplicationDeleted(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appKey); internal delegate void WebhostListenerApplicationPoolAllListenerChannelInstancesStopped(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId, int listenerChannelId); internal delegate void WebhostListenerApplicationPoolCanOpenNewListenerChannelInstance(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId, int listenerChannelId); internal delegate void WebhostListenerApplicationPoolCreated(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId, IntPtr sid); internal delegate void WebhostListenerApplicationPoolDeleted(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId); internal delegate void WebhostListenerApplicationPoolIdentityChanged(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId, IntPtr sid); internal delegate void WebhostListenerApplicationPoolStateChanged(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId, bool isEnabled); internal delegate void WebhostListenerApplicationRequestsBlockedChanged(IntPtr context, [MarshalAs(UnmanagedType.LPWStr)] string appKey, bool requestsBlocked); internal delegate void WebhostListenerConfigManagerConnected(IntPtr context); internal delegate void WebhostListenerConfigManagerConnectRejected(IntPtr context, int hresult); internal delegate void WebhostListenerConfigManagerDisconnected(IntPtr context, int hresult); internal delegate void WebhostListenerConfigManagerInitializationCompleted(IntPtr context); internal delegate int WebhostRegisterProtocol([MarshalAs(UnmanagedType.LPWStr)] string protocolId, ref WebhostListenerCallbacks listenerCallbacks, IntPtr context, out int protocolHandle); internal delegate int WebhostOpenListenerChannelInstance(int protocolHandle, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId, int listenerChannelId, byte[] queueBlob, int queueBlobByteCount); internal delegate int WebhostCloseAllListenerChannelInstances(int protocolHandle, [MarshalAs(UnmanagedType.LPWStr)] string appPoolId, int listenerChannelId); internal delegate int WebhostUnregisterProtocol(int protocolHandle); [DllImport(KERNEL32, CharSet = CharSet.Ansi, SetLastError = true, BestFitMapping = false, ThrowOnUnmappableChar = true)] internal static extern IntPtr GetProcAddress( [In] SafeFreeLibrary hModule, [In] string lpProcName ); [DllImport(KERNEL32, CharSet = CharSet.Unicode, SetLastError = true)] internal static extern SafeFreeLibrary LoadLibraryEx( [In] string lpFileName, [In] IntPtr hFile, [In] int dwFlags ); [StructLayout(LayoutKind.Sequential)] internal struct WebhostListenerCallbacks { internal int dwBytesInCallbackStructure; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerConfigManagerConnected webhostListenerConfigManagerConnected; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerConfigManagerDisconnected webhostListenerConfigManagerDisconnected; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerConfigManagerInitializationCompleted webhostListenerConfigManagerInitializationCompleted; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationPoolCreated webhostListenerApplicationPoolCreated; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationPoolDeleted webhostListenerApplicationPoolDeleted; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationPoolIdentityChanged webhostListenerApplicationPoolIdentityChanged; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationPoolStateChanged webhostListenerApplicationPoolStateChanged; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationPoolCanOpenNewListenerChannelInstance webhostListenerApplicationPoolCanOpenNewListenerChannelInstance; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationPoolAllListenerChannelInstancesStopped webhostListenerApplicationPoolAllListenerChannelInstancesStopped; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationCreated webhostListenerApplicationCreated; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationDeleted webhostListenerApplicationDeleted; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationBindingsChanged webhostListenerApplicationBindingsChanged; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationAppPoolChanged webhostListenerApplicationAppPoolChanged; [MarshalAs(UnmanagedType.FunctionPtr)] internal WebhostListenerApplicationRequestsBlockedChanged webhostListenerApplicationRequestsBlockedChanged; } internal sealed class SafeFreeLibrary : SafeHandleZeroOrMinusOneIsInvalid { const string KERNEL32 = "kernel32.dll"; internal SafeFreeLibrary() : base(true) { } [DllImport(KERNEL32, SetLastError = true), SuppressUnmanagedCodeSecurity] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] private static extern bool FreeLibrary(IntPtr hModule); protected override bool ReleaseHandle() { return FreeLibrary(handle); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
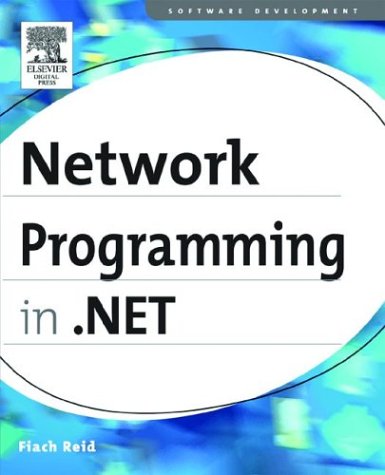
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationHelpers.cs
- FileUpload.cs
- WebSysDisplayNameAttribute.cs
- EventLogPermissionAttribute.cs
- ImageBrush.cs
- RequestResizeEvent.cs
- EdmPropertyAttribute.cs
- MDIControlStrip.cs
- InvalidProgramException.cs
- DoubleStorage.cs
- AbandonedMutexException.cs
- PageEventArgs.cs
- VirtualPathProvider.cs
- LazyTextWriterCreator.cs
- ToolStripManager.cs
- SystemNetHelpers.cs
- DBSchemaTable.cs
- ComponentChangingEvent.cs
- MessageBox.cs
- DocumentViewerConstants.cs
- TimerElapsedEvenArgs.cs
- WebPartManagerInternals.cs
- EntityDataSourceConfigureObjectContext.cs
- ExceptionUtility.cs
- EncryptedPackage.cs
- StatusBar.cs
- Trace.cs
- VirtualPath.cs
- DataGridCell.cs
- FormsAuthenticationCredentials.cs
- FontDialog.cs
- HttpsTransportElement.cs
- SeekStoryboard.cs
- VerticalAlignConverter.cs
- oledbconnectionstring.cs
- XmlIgnoreAttribute.cs
- PrimitiveCodeDomSerializer.cs
- PowerStatus.cs
- MultiAsyncResult.cs
- MessageBodyDescription.cs
- KeyGestureConverter.cs
- NamespaceEmitter.cs
- ObjectHandle.cs
- DynamicPhysicalDiscoSearcher.cs
- AppDomainInstanceProvider.cs
- RoutedUICommand.cs
- CachedBitmap.cs
- Models.cs
- ColumnWidthChangedEvent.cs
- dtdvalidator.cs
- IndexedString.cs
- BufferedStream.cs
- CommonGetThemePartSize.cs
- GlyphTypeface.cs
- DataGridViewRowCollection.cs
- Typography.cs
- LogSwitch.cs
- UInt32Storage.cs
- errorpatternmatcher.cs
- XmlDataImplementation.cs
- XmlBinaryReader.cs
- MimeFormImporter.cs
- SpellCheck.cs
- nulltextcontainer.cs
- ServicePoint.cs
- GridViewCancelEditEventArgs.cs
- KeyConverter.cs
- FontFamily.cs
- ProgressBarBrushConverter.cs
- TypeDescriptor.cs
- PreviewPrintController.cs
- PixelShader.cs
- EditBehavior.cs
- TypeDescriptionProvider.cs
- DLinqTableProvider.cs
- GridViewCellAutomationPeer.cs
- AttributeEmitter.cs
- CodeMethodInvokeExpression.cs
- Proxy.cs
- TypeUsage.cs
- Debug.cs
- FilterEventArgs.cs
- InstanceLockLostException.cs
- CompilerError.cs
- DropDownList.cs
- NullToBooleanConverter.cs
- SpanIndex.cs
- CqlLexerHelpers.cs
- XmlSerializerFactory.cs
- CombinedGeometry.cs
- Control.cs
- KnownAssemblyEntry.cs
- SharedPerformanceCounter.cs
- XslTransform.cs
- XmlElement.cs
- DesignerCommandSet.cs
- CompositeScriptReference.cs
- securestring.cs
- CorruptStoreException.cs
- ManifestSignatureInformation.cs