Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / Design / PropertyTab.cs / 1 / PropertyTab.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] public abstract class PropertyTab : IExtenderProvider { private Object[] components; private Bitmap bitmap; private bool checkedBmp; ///Provides a base class for property tabs. ///~PropertyTab() { Dispose(false); } // don't override this. Just put a 16x16 bitmap in a file with the same name as your class in your resources. /// /// /// public virtual Bitmap Bitmap { get { if (!checkedBmp && bitmap == null) { string bmpName = GetType().Name + ".bmp"; try { bitmap = new Bitmap(GetType(), bmpName); } catch (Exception ex) { Debug.Fail("Failed to find bitmap '" + bmpName + "' for class " + GetType().FullName, ex.ToString()); } checkedBmp = true; } return bitmap; } } // don't override this either. ///Gets or sets a bitmap to display in the property tab. ////// /// public virtual Object[] Components { get { return components; } set { this.components = value; } } // okay. Override this to give a good TabName. ///Gets or sets the array of components the property tab is associated with. ////// /// public abstract string TabName { get; } ///Gets or sets the name for the property tab. ////// /// public virtual string HelpKeyword { get { return TabName; } } // override this to reject components you don't want to support. ///Gets or sets the help keyword that is to be associated with this tab. This defaults /// to the tab name. ////// /// public virtual bool CanExtend(Object extendee) { return true; } ///Gets a value indicating whether the specified object be can extended. ////// /// public virtual void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///[To be supplied.] ///protected virtual void Dispose(bool disposing) { if (disposing) { if (bitmap != null) { bitmap.Dispose(); bitmap = null; } } } // return the default property item /// /// /// public virtual PropertyDescriptor GetDefaultProperty(Object component) { return TypeDescriptor.GetDefaultProperty(component); } // okay, override this to return whatever you want to return... All properties must apply to component. ///Gets the default property of the specified component. ////// /// public virtual PropertyDescriptorCollection GetProperties(Object component) { return GetProperties(component, null); } ///Gets the properties of the specified component. ////// /// public abstract PropertyDescriptorCollection GetProperties(object component, Attribute[] attributes); ///Gets the properties of the specified component which match the specified /// attributes. ////// /// public virtual PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object component, Attribute[] attributes) { return GetProperties(component, attributes); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Gets the properties of the specified component... ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] public abstract class PropertyTab : IExtenderProvider { private Object[] components; private Bitmap bitmap; private bool checkedBmp; ///Provides a base class for property tabs. ///~PropertyTab() { Dispose(false); } // don't override this. Just put a 16x16 bitmap in a file with the same name as your class in your resources. /// /// /// public virtual Bitmap Bitmap { get { if (!checkedBmp && bitmap == null) { string bmpName = GetType().Name + ".bmp"; try { bitmap = new Bitmap(GetType(), bmpName); } catch (Exception ex) { Debug.Fail("Failed to find bitmap '" + bmpName + "' for class " + GetType().FullName, ex.ToString()); } checkedBmp = true; } return bitmap; } } // don't override this either. ///Gets or sets a bitmap to display in the property tab. ////// /// public virtual Object[] Components { get { return components; } set { this.components = value; } } // okay. Override this to give a good TabName. ///Gets or sets the array of components the property tab is associated with. ////// /// public abstract string TabName { get; } ///Gets or sets the name for the property tab. ////// /// public virtual string HelpKeyword { get { return TabName; } } // override this to reject components you don't want to support. ///Gets or sets the help keyword that is to be associated with this tab. This defaults /// to the tab name. ////// /// public virtual bool CanExtend(Object extendee) { return true; } ///Gets a value indicating whether the specified object be can extended. ////// /// public virtual void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///[To be supplied.] ///protected virtual void Dispose(bool disposing) { if (disposing) { if (bitmap != null) { bitmap.Dispose(); bitmap = null; } } } // return the default property item /// /// /// public virtual PropertyDescriptor GetDefaultProperty(Object component) { return TypeDescriptor.GetDefaultProperty(component); } // okay, override this to return whatever you want to return... All properties must apply to component. ///Gets the default property of the specified component. ////// /// public virtual PropertyDescriptorCollection GetProperties(Object component) { return GetProperties(component, null); } ///Gets the properties of the specified component. ////// /// public abstract PropertyDescriptorCollection GetProperties(object component, Attribute[] attributes); ///Gets the properties of the specified component which match the specified /// attributes. ////// /// public virtual PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object component, Attribute[] attributes) { return GetProperties(component, attributes); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Gets the properties of the specified component... ///
Link Menu
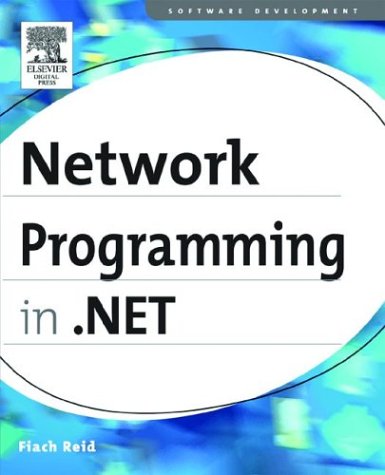
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActivityExecutionContextCollection.cs
- QilIterator.cs
- CommandField.cs
- ThousandthOfEmRealPoints.cs
- SafeSystemMetrics.cs
- UnsafeNativeMethods.cs
- ScriptingAuthenticationServiceSection.cs
- PagerSettings.cs
- DataProtectionSecurityStateEncoder.cs
- CmsInterop.cs
- AvtEvent.cs
- NavigationService.cs
- IIS7ConfigurationLoader.cs
- PersistenceIOParticipant.cs
- PassportAuthentication.cs
- CodeGeneratorOptions.cs
- ChangePassword.cs
- IndependentAnimationStorage.cs
- ComponentCommands.cs
- HttpModuleActionCollection.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- AsymmetricCryptoHandle.cs
- TraceListener.cs
- PanelStyle.cs
- BeginEvent.cs
- SHA1.cs
- XMLUtil.cs
- TextLineBreak.cs
- InputLanguageManager.cs
- HtmlButton.cs
- GraphicsContext.cs
- UInt32Storage.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- DynamicDiscoveryDocument.cs
- RenderDataDrawingContext.cs
- CodeDOMProvider.cs
- ApplicationCommands.cs
- TextParagraphProperties.cs
- TableItemStyle.cs
- XmlWrappingReader.cs
- RecognizedAudio.cs
- VectorCollection.cs
- UrlPath.cs
- SmtpDigestAuthenticationModule.cs
- ForeignKeyConstraint.cs
- AdRotator.cs
- ClientProxyGenerator.cs
- ListItemConverter.cs
- ObservableCollection.cs
- UrlUtility.cs
- ComplexObject.cs
- ListViewPagedDataSource.cs
- SystemBrushes.cs
- TagMapCollection.cs
- _FtpDataStream.cs
- XmlStreamNodeWriter.cs
- MenuItemBinding.cs
- TdsEnums.cs
- FlowDocumentPageViewerAutomationPeer.cs
- WindowsListViewGroupSubsetLink.cs
- CornerRadiusConverter.cs
- DataSourceXmlElementAttribute.cs
- XsltOutput.cs
- Throw.cs
- ShaperBuffers.cs
- TypographyProperties.cs
- UserNameSecurityToken.cs
- precedingsibling.cs
- ScopelessEnumAttribute.cs
- ControlCachePolicy.cs
- _AcceptOverlappedAsyncResult.cs
- ReadOnlyHierarchicalDataSourceView.cs
- JpegBitmapDecoder.cs
- Visual3D.cs
- PageVisual.cs
- CultureTable.cs
- WorkflowInlining.cs
- KnownBoxes.cs
- SqlWebEventProvider.cs
- WindowsPen.cs
- DynamicResourceExtensionConverter.cs
- BorderSidesEditor.cs
- XhtmlBasicCommandAdapter.cs
- DataGridViewCellPaintingEventArgs.cs
- AnnotationMap.cs
- BinaryFormatterSinks.cs
- WindowsHyperlink.cs
- IgnoreFlushAndCloseStream.cs
- CompositeKey.cs
- SchemaImporter.cs
- TrackingStringDictionary.cs
- SID.cs
- BaseDataList.cs
- FilteredAttributeCollection.cs
- ClientData.cs
- IUnknownConstantAttribute.cs
- CaretElement.cs
- PropertyMapper.cs
- PathData.cs
- ElementProxy.cs