Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / TextFormatting / ThousandthOfEmRealPoints.cs / 1 / ThousandthOfEmRealPoints.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: ThousandthOfEmRealPoints class // // History: // 1/13/2005: Garyyang Created the file // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections.Generic; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.TextFormatting { ////// This is a fixed-size implementation of IList<Point>. It is aimed to reduce the double values storage /// while providing enough precision for glyph run operations. Current usage pattern suggests that there is no /// need to support resizing functionality (i.e. Add(), Insert(), Remove(), RemoveAt()). /// /// For each point being stored, it will store the X and Y coordinates of the point into two seperate ThousandthOfEmRealDoubles, /// which scales double to 16-bit integer if possible. /// /// internal sealed class ThousandthOfEmRealPoints : IList{ //---------------------------------- // Constructor //---------------------------------- internal ThousandthOfEmRealPoints( double emSize, int capacity ) { Debug.Assert(capacity >= 0); InitArrays(emSize, capacity); } internal ThousandthOfEmRealPoints( double emSize, IList pointValues ) { Debug.Assert(pointValues != null); InitArrays(emSize, pointValues.Count); // do the setting for (int i = 0; i < Count; i++) { _xArray[i] = pointValues[i].X; _yArray[i] = pointValues[i].Y; } } //------------------------------------- // Internal properties //------------------------------------- public int Count { get { return _xArray.Count; } } public bool IsReadOnly { get { return false; } } public Point this[int index] { get { // underlying array does boundary check return new Point(_xArray[index], _yArray[index]); } set { // underlying array does boundary check _xArray[index] = value.X; _yArray[index] = value.Y; } } //------------------------------------ // internal methods //------------------------------------ public int IndexOf(Point item) { // linear search for (int i = 0; i < Count; i++) { if (_xArray[i] == item.X && _yArray[i] == item.Y) { return i; } } return -1; } public void Clear() { _xArray.Clear(); _yArray.Clear(); } public bool Contains(Point item) { return IndexOf(item) >= 0; } public void CopyTo(Point[] array, int arrayIndex) { // parameter validations if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException( SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional), "array"); } if (arrayIndex < 0) { throw new ArgumentOutOfRangeException("arrayIndex"); } if (arrayIndex >= array.Length) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "arrayIndex", "array"), "arrayIndex"); } if ((array.Length - Count - arrayIndex) < 0) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); } // do the copying here for (int i = 0; i < Count; i++) { array[arrayIndex + i] = this[i]; } } public IEnumerator GetEnumerator() { for (int i = 0; i < Count; i++) { yield return this[i]; } } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } public void Add(Point value) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Insert(int index, Point item) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public bool Remove(Point item) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void RemoveAt(int index) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } //--------------------------------------------- // Private methods //--------------------------------------------- private void InitArrays(double emSize, int capacity) { _xArray = new ThousandthOfEmRealDoubles(emSize, capacity); _yArray = new ThousandthOfEmRealDoubles(emSize, capacity); } //---------------------------------------- // Private members //---------------------------------------- private ThousandthOfEmRealDoubles _xArray; // scaled double array for X coordinates private ThousandthOfEmRealDoubles _yArray; // scaled double array for Y coordinates } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: ThousandthOfEmRealPoints class // // History: // 1/13/2005: Garyyang Created the file // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Collections.Generic; using System.Windows; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.TextFormatting { ////// This is a fixed-size implementation of IList<Point>. It is aimed to reduce the double values storage /// while providing enough precision for glyph run operations. Current usage pattern suggests that there is no /// need to support resizing functionality (i.e. Add(), Insert(), Remove(), RemoveAt()). /// /// For each point being stored, it will store the X and Y coordinates of the point into two seperate ThousandthOfEmRealDoubles, /// which scales double to 16-bit integer if possible. /// /// internal sealed class ThousandthOfEmRealPoints : IList{ //---------------------------------- // Constructor //---------------------------------- internal ThousandthOfEmRealPoints( double emSize, int capacity ) { Debug.Assert(capacity >= 0); InitArrays(emSize, capacity); } internal ThousandthOfEmRealPoints( double emSize, IList pointValues ) { Debug.Assert(pointValues != null); InitArrays(emSize, pointValues.Count); // do the setting for (int i = 0; i < Count; i++) { _xArray[i] = pointValues[i].X; _yArray[i] = pointValues[i].Y; } } //------------------------------------- // Internal properties //------------------------------------- public int Count { get { return _xArray.Count; } } public bool IsReadOnly { get { return false; } } public Point this[int index] { get { // underlying array does boundary check return new Point(_xArray[index], _yArray[index]); } set { // underlying array does boundary check _xArray[index] = value.X; _yArray[index] = value.Y; } } //------------------------------------ // internal methods //------------------------------------ public int IndexOf(Point item) { // linear search for (int i = 0; i < Count; i++) { if (_xArray[i] == item.X && _yArray[i] == item.Y) { return i; } } return -1; } public void Clear() { _xArray.Clear(); _yArray.Clear(); } public bool Contains(Point item) { return IndexOf(item) >= 0; } public void CopyTo(Point[] array, int arrayIndex) { // parameter validations if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException( SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional), "array"); } if (arrayIndex < 0) { throw new ArgumentOutOfRangeException("arrayIndex"); } if (arrayIndex >= array.Length) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "arrayIndex", "array"), "arrayIndex"); } if ((array.Length - Count - arrayIndex) < 0) { throw new ArgumentException( SR.Get( SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); } // do the copying here for (int i = 0; i < Count; i++) { array[arrayIndex + i] = this[i]; } } public IEnumerator GetEnumerator() { for (int i = 0; i < Count; i++) { yield return this[i]; } } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } public void Add(Point value) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void Insert(int index, Point item) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public bool Remove(Point item) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } public void RemoveAt(int index) { // not supported, same as Point[] throw new NotSupportedException(SR.Get(SRID.CollectionIsFixedSize)); } //--------------------------------------------- // Private methods //--------------------------------------------- private void InitArrays(double emSize, int capacity) { _xArray = new ThousandthOfEmRealDoubles(emSize, capacity); _yArray = new ThousandthOfEmRealDoubles(emSize, capacity); } //---------------------------------------- // Private members //---------------------------------------- private ThousandthOfEmRealDoubles _xArray; // scaled double array for X coordinates private ThousandthOfEmRealDoubles _yArray; // scaled double array for Y coordinates } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
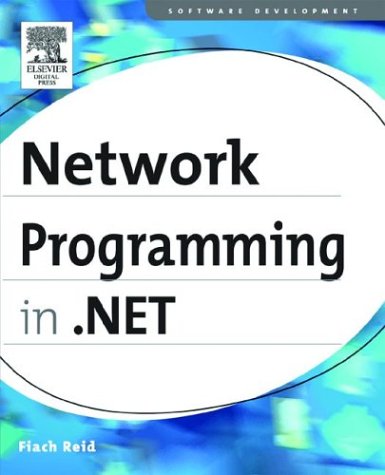
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WSFederationHttpBinding.cs
- TableItemPattern.cs
- Point.cs
- FeatureManager.cs
- glyphs.cs
- BaseConfigurationRecord.cs
- InputLanguageEventArgs.cs
- DataGridToolTip.cs
- CollectionView.cs
- Attachment.cs
- OrderedDictionaryStateHelper.cs
- StyleCollection.cs
- SpecialNameAttribute.cs
- TableStyle.cs
- FactoryRecord.cs
- ComponentCollection.cs
- TableHeaderCell.cs
- _AutoWebProxyScriptHelper.cs
- WindowsListViewItemCheckBox.cs
- UnhandledExceptionEventArgs.cs
- TreeNodeCollection.cs
- ModuleConfigurationInfo.cs
- KerberosSecurityTokenAuthenticator.cs
- TemplateColumn.cs
- _AutoWebProxyScriptHelper.cs
- WindowsRegion.cs
- TabItemWrapperAutomationPeer.cs
- SspiSafeHandles.cs
- StopStoryboard.cs
- GridViewHeaderRowPresenter.cs
- SelectionEditingBehavior.cs
- Documentation.cs
- XmlAnyElementAttributes.cs
- GeometryModel3D.cs
- infer.cs
- UpWmlMobileTextWriter.cs
- ValueQuery.cs
- SystemIPGlobalProperties.cs
- FirstQueryOperator.cs
- RuleEngine.cs
- Point3DAnimationUsingKeyFrames.cs
- _NegoStream.cs
- CheckedListBox.cs
- ProtocolException.cs
- ArrayElementGridEntry.cs
- TextEditorCopyPaste.cs
- PropertyConverter.cs
- WorkflowControlClient.cs
- PageClientProxyGenerator.cs
- LogEntryHeaderSerializer.cs
- ServiceSecurityAuditBehavior.cs
- WinEventTracker.cs
- OracleCommandBuilder.cs
- Matrix.cs
- EnumMemberAttribute.cs
- OpenTypeLayoutCache.cs
- OdbcDataReader.cs
- RequestSecurityTokenSerializer.cs
- CodeDOMProvider.cs
- HttpListenerRequest.cs
- AddingNewEventArgs.cs
- Renderer.cs
- LogFlushAsyncResult.cs
- NamespaceExpr.cs
- QilCloneVisitor.cs
- MediaPlayerState.cs
- ColumnProvider.cs
- ListenerAdapterBase.cs
- WindowsStreamSecurityUpgradeProvider.cs
- PointLightBase.cs
- Size3D.cs
- HostedHttpTransportManager.cs
- CharKeyFrameCollection.cs
- Symbol.cs
- _NegoState.cs
- AQNBuilder.cs
- ProcessInfo.cs
- CommandConverter.cs
- NoClickablePointException.cs
- DecimalConverter.cs
- OpacityConverter.cs
- OpCellTreeNode.cs
- ToolboxDataAttribute.cs
- APCustomTypeDescriptor.cs
- Compress.cs
- QilInvokeLateBound.cs
- ApplicationActivator.cs
- GridViewColumn.cs
- StylusPointPropertyUnit.cs
- Clause.cs
- Trace.cs
- NTAccount.cs
- XmlHelper.cs
- TextChange.cs
- EntityProxyFactory.cs
- SafeEventHandle.cs
- CodeMethodReturnStatement.cs
- FrameworkElementFactory.cs
- SelectionGlyphBase.cs
- WindowsSolidBrush.cs