Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridToolTip.cs / 1 / DataGridToolTip.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; using System.Diagnostics; using System.ComponentModel; // this class is basically a NativeWindow that does toolTipping // should be one for the entire grid internal class DataGridToolTip : MarshalByRefObject { // the toolTip control private NativeWindow tipWindow = null; // the dataGrid which contains this toolTip private DataGrid dataGrid = null; // CONSTRUCTOR public DataGridToolTip(DataGrid dataGrid) { Debug.Assert(dataGrid!= null, "can't attach a tool tip to a null grid"); this.dataGrid = dataGrid; } // will ensure that the toolTip window was created public void CreateToolTipHandle() { if (tipWindow == null || tipWindow.Handle == IntPtr.Zero) { NativeMethods.INITCOMMONCONTROLSEX icc = new NativeMethods.INITCOMMONCONTROLSEX(); icc.dwICC = NativeMethods.ICC_TAB_CLASSES; icc.dwSize = Marshal.SizeOf(icc); SafeNativeMethods.InitCommonControlsEx(icc); CreateParams cparams = new CreateParams(); cparams.Parent = dataGrid.Handle; cparams.ClassName = NativeMethods.TOOLTIPS_CLASS; cparams.Style = NativeMethods.TTS_ALWAYSTIP; tipWindow = new NativeWindow(); tipWindow.CreateHandle(cparams); UnsafeNativeMethods.SendMessage(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.TTM_SETMAXTIPWIDTH, 0, SystemInformation.MaxWindowTrackSize.Width); SafeNativeMethods.SetWindowPos(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.HWND_NOTOPMOST, 0, 0, 0, 0, NativeMethods.SWP_NOSIZE | NativeMethods.SWP_NOMOVE | NativeMethods.SWP_NOACTIVATE); UnsafeNativeMethods.SendMessage(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.TTM_SETDELAYTIME, NativeMethods.TTDT_INITIAL, 0); } } // this function will add a toolTip to the // windows system public void AddToolTip(String toolTipString, IntPtr toolTipId, Rectangle iconBounds) { Debug.Assert(tipWindow != null && tipWindow.Handle != IntPtr.Zero, "the tipWindow was not initialized, bailing out"); if (toolTipString == null) throw new ArgumentNullException("toolTipString"); if (iconBounds.IsEmpty) throw new ArgumentNullException("iconBounds", SR.GetString(SR.DataGridToolTipEmptyIcon)); NativeMethods.TOOLINFO_T toolInfo = new NativeMethods.TOOLINFO_T(); toolInfo.cbSize = Marshal.SizeOf(toolInfo); toolInfo.hwnd = dataGrid.Handle; toolInfo.uId = toolTipId; toolInfo.lpszText = toolTipString; toolInfo.rect = NativeMethods.RECT.FromXYWH(iconBounds.X, iconBounds.Y, iconBounds.Width, iconBounds.Height); toolInfo.uFlags = NativeMethods.TTF_SUBCLASS; UnsafeNativeMethods.SendMessage(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.TTM_ADDTOOL, 0, toolInfo); } public void RemoveToolTip(IntPtr toolTipId) { NativeMethods.TOOLINFO_T toolInfo = new NativeMethods.TOOLINFO_T(); toolInfo.cbSize = Marshal.SizeOf(toolInfo); toolInfo.hwnd = dataGrid.Handle; toolInfo.uId = toolTipId; UnsafeNativeMethods.SendMessage(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.TTM_DELTOOL, 0, toolInfo); } // will destroy the tipWindow public void Destroy() { Debug.Assert(tipWindow != null, "how can one destroy a null window"); tipWindow.DestroyHandle(); tipWindow = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.Remoting; using System.Runtime.InteropServices; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; using System.Diagnostics; using System.ComponentModel; // this class is basically a NativeWindow that does toolTipping // should be one for the entire grid internal class DataGridToolTip : MarshalByRefObject { // the toolTip control private NativeWindow tipWindow = null; // the dataGrid which contains this toolTip private DataGrid dataGrid = null; // CONSTRUCTOR public DataGridToolTip(DataGrid dataGrid) { Debug.Assert(dataGrid!= null, "can't attach a tool tip to a null grid"); this.dataGrid = dataGrid; } // will ensure that the toolTip window was created public void CreateToolTipHandle() { if (tipWindow == null || tipWindow.Handle == IntPtr.Zero) { NativeMethods.INITCOMMONCONTROLSEX icc = new NativeMethods.INITCOMMONCONTROLSEX(); icc.dwICC = NativeMethods.ICC_TAB_CLASSES; icc.dwSize = Marshal.SizeOf(icc); SafeNativeMethods.InitCommonControlsEx(icc); CreateParams cparams = new CreateParams(); cparams.Parent = dataGrid.Handle; cparams.ClassName = NativeMethods.TOOLTIPS_CLASS; cparams.Style = NativeMethods.TTS_ALWAYSTIP; tipWindow = new NativeWindow(); tipWindow.CreateHandle(cparams); UnsafeNativeMethods.SendMessage(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.TTM_SETMAXTIPWIDTH, 0, SystemInformation.MaxWindowTrackSize.Width); SafeNativeMethods.SetWindowPos(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.HWND_NOTOPMOST, 0, 0, 0, 0, NativeMethods.SWP_NOSIZE | NativeMethods.SWP_NOMOVE | NativeMethods.SWP_NOACTIVATE); UnsafeNativeMethods.SendMessage(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.TTM_SETDELAYTIME, NativeMethods.TTDT_INITIAL, 0); } } // this function will add a toolTip to the // windows system public void AddToolTip(String toolTipString, IntPtr toolTipId, Rectangle iconBounds) { Debug.Assert(tipWindow != null && tipWindow.Handle != IntPtr.Zero, "the tipWindow was not initialized, bailing out"); if (toolTipString == null) throw new ArgumentNullException("toolTipString"); if (iconBounds.IsEmpty) throw new ArgumentNullException("iconBounds", SR.GetString(SR.DataGridToolTipEmptyIcon)); NativeMethods.TOOLINFO_T toolInfo = new NativeMethods.TOOLINFO_T(); toolInfo.cbSize = Marshal.SizeOf(toolInfo); toolInfo.hwnd = dataGrid.Handle; toolInfo.uId = toolTipId; toolInfo.lpszText = toolTipString; toolInfo.rect = NativeMethods.RECT.FromXYWH(iconBounds.X, iconBounds.Y, iconBounds.Width, iconBounds.Height); toolInfo.uFlags = NativeMethods.TTF_SUBCLASS; UnsafeNativeMethods.SendMessage(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.TTM_ADDTOOL, 0, toolInfo); } public void RemoveToolTip(IntPtr toolTipId) { NativeMethods.TOOLINFO_T toolInfo = new NativeMethods.TOOLINFO_T(); toolInfo.cbSize = Marshal.SizeOf(toolInfo); toolInfo.hwnd = dataGrid.Handle; toolInfo.uId = toolTipId; UnsafeNativeMethods.SendMessage(new HandleRef(tipWindow, tipWindow.Handle), NativeMethods.TTM_DELTOOL, 0, toolInfo); } // will destroy the tipWindow public void Destroy() { Debug.Assert(tipWindow != null, "how can one destroy a null window"); tipWindow.DestroyHandle(); tipWindow = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
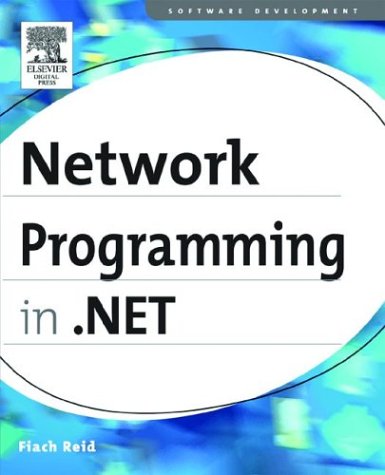
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaComplexContentExtension.cs
- ImageConverter.cs
- ReadOnlyHierarchicalDataSourceView.cs
- ListControl.cs
- ShaderEffect.cs
- FixedHighlight.cs
- VectorCollectionValueSerializer.cs
- PackageProperties.cs
- CompositeFontParser.cs
- ForwardPositionQuery.cs
- Underline.cs
- RuleSettings.cs
- WizardStepBase.cs
- FileSecurity.cs
- BindingOperations.cs
- WorkflowRuntimeServiceElement.cs
- StyleTypedPropertyAttribute.cs
- ListManagerBindingsCollection.cs
- HMACSHA512.cs
- ArraySubsetEnumerator.cs
- RotateTransform.cs
- QueueProcessor.cs
- METAHEADER.cs
- BitSet.cs
- XmlElementList.cs
- SettingsPropertyIsReadOnlyException.cs
- CommonObjectSecurity.cs
- ResXDataNode.cs
- DataGridViewAdvancedBorderStyle.cs
- SqlConnectionHelper.cs
- XmlDictionaryString.cs
- CharKeyFrameCollection.cs
- StdValidatorsAndConverters.cs
- MailAddressCollection.cs
- BindingMAnagerBase.cs
- SectionRecord.cs
- PageTrueTypeFont.cs
- GeometryGroup.cs
- XsdBuildProvider.cs
- LabelDesigner.cs
- NetStream.cs
- WindowsImpersonationContext.cs
- FragmentQueryKB.cs
- ApplicationSettingsBase.cs
- Internal.cs
- ContextMenu.cs
- TreeNodeClickEventArgs.cs
- HijriCalendar.cs
- DataGridViewTopRowAccessibleObject.cs
- storepermission.cs
- XmlSchemaNotation.cs
- BigIntegerStorage.cs
- ControlValuePropertyAttribute.cs
- ByteStream.cs
- EdmComplexPropertyAttribute.cs
- BaseParser.cs
- QuaternionValueSerializer.cs
- TargetPerspective.cs
- TextTabProperties.cs
- InvalidWMPVersionException.cs
- StatusBar.cs
- MenuItemCollection.cs
- DocumentViewerConstants.cs
- EnumValidator.cs
- SafeMILHandle.cs
- Win32.cs
- Inline.cs
- ReadOnlyDictionary.cs
- SafeIUnknown.cs
- DecimalKeyFrameCollection.cs
- InputScope.cs
- OleDbPropertySetGuid.cs
- CookielessHelper.cs
- StateMachine.cs
- IOException.cs
- CompatibleIComparer.cs
- XmlNodeChangedEventArgs.cs
- StylusShape.cs
- Label.cs
- TextBoxDesigner.cs
- SessionStateContainer.cs
- ProxyWebPartManager.cs
- DataGridViewCellStyle.cs
- RightsManagementErrorHandler.cs
- XmlSignificantWhitespace.cs
- PrivilegedConfigurationManager.cs
- AssemblyAssociatedContentFileAttribute.cs
- CanonicalXml.cs
- BulletedListDesigner.cs
- ScrollBar.cs
- StrokeNodeOperations.cs
- WebConfigurationHostFileChange.cs
- SoapServerMethod.cs
- Image.cs
- ChannelBinding.cs
- IOThreadScheduler.cs
- SystemEvents.cs
- isolationinterop.cs
- SignatureToken.cs
- Baml2006KnownTypes.cs