Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / EditCommandColumn.cs / 1 / EditCommandColumn.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Web.UI; using System.Security.Permissions; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class EditCommandColumn : DataGridColumn { ///Creates a special column with buttons for ///, /// , and commands to edit items /// within the selected row. /// public EditCommandColumn() { } ///Initializes a new instance of an ///class. /// [ DefaultValue(ButtonColumnType.LinkButton) ] public virtual ButtonColumnType ButtonType { get { object o = ViewState["ButtonType"]; if (o != null) return(ButtonColumnType)o; return ButtonColumnType.LinkButton; } set { if (value < ButtonColumnType.LinkButton || value > ButtonColumnType.PushButton) { throw new ArgumentOutOfRangeException("value"); } ViewState["ButtonType"] = value; OnColumnChanged(); } } ///Indicates the button type for the column. ////// [ Localizable(true), DefaultValue("") ] public virtual string CancelText { get { object o = ViewState["CancelText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["CancelText"] = value; OnColumnChanged(); } } [ DefaultValue(true), ] public virtual bool CausesValidation { get { object o = ViewState["CausesValidation"]; if (o != null) { return (bool)o; } return true; } set { ViewState["CausesValidation"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button /// in the column. /// [ Localizable(true), DefaultValue("") ] public virtual string EditText { get { object o = ViewState["EditText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["EditText"] = value; OnColumnChanged(); } } ///Indicates the text to display for the ///command button in /// the column. /// [ Localizable(true), DefaultValue("") ] public virtual string UpdateText { get { object o = ViewState["UpdateText"]; if (o != null) return(string)o; return String.Empty; } set { ViewState["UpdateText"] = value; OnColumnChanged(); } } [ DefaultValue(""), ] public virtual string ValidationGroup { get { object o = ViewState["ValidationGroup"]; if (o != null) { return (string)o; } return String.Empty; } set { ViewState["ValidationGroup"] = value; OnColumnChanged(); } } private void AddButtonToCell(TableCell cell, string commandName, string buttonText, bool causesValidation, string validationGroup) { WebControl buttonControl = null; ControlCollection controls = cell.Controls; ButtonColumnType buttonType = ButtonType; if (buttonType == ButtonColumnType.LinkButton) { LinkButton button = new DataGridLinkButton(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } else { Button button = new Button(); buttonControl = button; button.CommandName = commandName; button.Text = buttonText; button.CausesValidation = causesValidation; button.ValidationGroup = validationGroup; } controls.Add(buttonControl); } ///Indicates the text to display for the ///command button /// in the column. /// public override void InitializeCell(TableCell cell, int columnIndex, ListItemType itemType) { base.InitializeCell(cell, columnIndex, itemType); bool causesValidation = CausesValidation; if ((itemType != ListItemType.Header) && (itemType != ListItemType.Footer)) { if (itemType == ListItemType.EditItem) { ControlCollection controls = cell.Controls; AddButtonToCell(cell, DataGrid.UpdateCommandName, UpdateText, causesValidation, ValidationGroup); LiteralControl spaceControl = new LiteralControl(" "); controls.Add(spaceControl); AddButtonToCell(cell, DataGrid.CancelCommandName, CancelText, false, String.Empty); } else { AddButtonToCell(cell, DataGrid.EditCommandName, EditText, false, String.Empty); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Initializes a cell within the column. ///
Link Menu
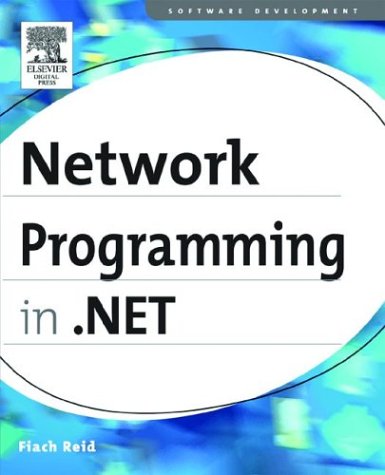
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StatementContext.cs
- EditorZoneDesigner.cs
- BamlTreeUpdater.cs
- _UriSyntax.cs
- TryExpression.cs
- TreeNode.cs
- DataServiceKeyAttribute.cs
- BindingSource.cs
- SoapFault.cs
- XmlElementAttribute.cs
- xmlsaver.cs
- HostedHttpRequestAsyncResult.cs
- BinaryOperationBinder.cs
- TextTreeUndoUnit.cs
- ThousandthOfEmRealPoints.cs
- DataGridCell.cs
- Listbox.cs
- UserControlCodeDomTreeGenerator.cs
- TransactionContext.cs
- CheckBoxAutomationPeer.cs
- AutomationEvent.cs
- X509CertificateInitiatorClientCredential.cs
- Errors.cs
- ViewKeyConstraint.cs
- SQLInt16Storage.cs
- ZipIOFileItemStream.cs
- ProjectionRewriter.cs
- PointAnimationUsingKeyFrames.cs
- LinearQuaternionKeyFrame.cs
- SplineQuaternionKeyFrame.cs
- future.cs
- GestureRecognitionResult.cs
- ChtmlSelectionListAdapter.cs
- GlyphRun.cs
- WindowsClientElement.cs
- GenericWebPart.cs
- ConfigurationManagerInternal.cs
- DataGridViewCellPaintingEventArgs.cs
- ManagementClass.cs
- SymLanguageVendor.cs
- BinHexEncoder.cs
- DataViewManagerListItemTypeDescriptor.cs
- SQLInt64Storage.cs
- TdsParserSessionPool.cs
- ParentUndoUnit.cs
- AliasedSlot.cs
- TransactionsSectionGroup.cs
- CodeDOMUtility.cs
- EdmToObjectNamespaceMap.cs
- AsyncPostBackErrorEventArgs.cs
- ImageButton.cs
- SafeEventLogReadHandle.cs
- BCryptNative.cs
- EditorReuseAttribute.cs
- XmlStringTable.cs
- WebPartCancelEventArgs.cs
- TaskFileService.cs
- AssociationTypeEmitter.cs
- ToolZone.cs
- TextFormatterHost.cs
- recordstate.cs
- StrokeNode.cs
- PathData.cs
- DefaultProfileManager.cs
- MimeWriter.cs
- safex509handles.cs
- RepeatBehavior.cs
- KeyEventArgs.cs
- BitmapEffectCollection.cs
- PauseStoryboard.cs
- ControlPropertyNameConverter.cs
- HttpCacheParams.cs
- X509ChainPolicy.cs
- EntityChangedParams.cs
- Guid.cs
- DbModificationClause.cs
- DoubleConverter.cs
- CompiledXpathExpr.cs
- RouteData.cs
- TTSEvent.cs
- DefaultTraceListener.cs
- PathNode.cs
- TempEnvironment.cs
- PagePropertiesChangingEventArgs.cs
- DataSvcMapFileSerializer.cs
- CellParaClient.cs
- ValidationHelper.cs
- CodeTypeReferenceExpression.cs
- cookiecollection.cs
- SQLBytes.cs
- ParallelQuery.cs
- TreeViewEvent.cs
- TraceFilter.cs
- Gdiplus.cs
- AccessedThroughPropertyAttribute.cs
- DriveInfo.cs
- ValuePattern.cs
- NonSerializedAttribute.cs
- RangeBase.cs
- TypeBuilderInstantiation.cs