Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebParts / EditorZoneDesigner.cs / 1 / EditorZoneDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.WebControls.WebParts { using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Design; using System.Diagnostics; using System.Globalization; using System.IO; using System.Web.UI.Design; using System.Web.UI.Design.WebControls; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; ////// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class EditorZoneDesigner : ToolZoneDesigner { private static DesignerAutoFormatCollection _autoFormats; private EditorZone _zone; private TemplateGroup _templateGroup; public override DesignerAutoFormatCollection AutoFormats { get { if (_autoFormats == null) { _autoFormats = CreateAutoFormats(AutoFormatSchemes.EDITORZONE_SCHEMES, delegate(DataRow schemeData) { return new EditorZoneAutoFormat(schemeData); }); } return _autoFormats; } } public override TemplateGroupCollection TemplateGroups { get { TemplateGroupCollection groups = base.TemplateGroups; if (_templateGroup == null) { _templateGroup = CreateZoneTemplateGroup(); } groups.Add(_templateGroup); return groups; } } public override string GetDesignTimeHtml() { return GetDesignTimeHtml(null); } ////// Provides the layout html for the control in the designer, including regions. /// public override string GetDesignTimeHtml(DesignerRegionCollection regions) { string designTimeHtml; try { EditorZone zone = (EditorZone)ViewControl; bool useRegions = UseRegions(regions, _zone.ZoneTemplate, zone.ZoneTemplate); // When there is an editable region, we want to use the regular control // rendering instead of the EmptyDesignTimeHtml if (zone.ZoneTemplate == null && !useRegions) { designTimeHtml = GetEmptyDesignTimeHtml(); } else { ((ICompositeControlDesignerAccessor)zone).RecreateChildControls(); if (regions != null && useRegions) { // If the tools supports editable regions, the initial rendering of the // WebParts in the Zone is thrown away by the tool anyway, so we should clear // the controls collection before rendering. If we don't clear the controls // collection and a WebPart inside the Zone throws an exception when rendering, // this would cause the whole Zone to render as an error, instead of just // the offending WebPart. This also improves perf. zone.Controls.Clear(); EditorPartEditableDesignerRegion region = new EditorPartEditableDesignerRegion(zone, TemplateDefinition); // Tells Venus that all controls inside the EditableRegion should be parented to the zone region.Properties[typeof(Control)] = zone; region.IsSingleInstanceTemplate = true; region.Description = SR.GetString(SR.ContainerControlDesigner_RegionWatermark); regions.Add(region); } designTimeHtml = base.GetDesignTimeHtml(); } // Don't substitute the placeholder if this is the AutoFormat PreviewControl if (ViewInBrowseMode && zone.ID != EditorZoneAutoFormat.PreviewControlID) { designTimeHtml = CreatePlaceHolderDesignTimeHtml(); } } catch (Exception e) { designTimeHtml = GetErrorDesignTimeHtml(e); } return designTimeHtml; } ////// /// Get the content for the specified region /// public override string GetEditableDesignerRegionContent(EditableDesignerRegion region) { Debug.Assert(region != null); // Occasionally getting NullRef here in WebMatrix. Maybe Zone is null? Debug.Assert(_zone != null); return ControlPersister.PersistTemplate(_zone.ZoneTemplate, (IDesignerHost)Component.Site.GetService(typeof(IDesignerHost))); } protected override string GetEmptyDesignTimeHtml() { return CreatePlaceHolderDesignTimeHtml(SR.GetString(SR.EditorZoneDesigner_Empty)); } public override void Initialize(IComponent component) { VerifyInitializeArgument(component, typeof(EditorZone)); base.Initialize(component); _zone = (EditorZone)component; } ////// /// Set the content for the specified region /// public override void SetEditableDesignerRegionContent(EditableDesignerRegion region, string content) { Debug.Assert(region != null); _zone.ZoneTemplate = ControlParser.ParseTemplate((IDesignerHost)Component.Site.GetService(typeof(IDesignerHost)), content); IsDirtyInternal = true; } private sealed class EditorPartEditableDesignerRegion : TemplatedEditableDesignerRegion { private EditorZone _zone; public EditorPartEditableDesignerRegion(EditorZone zone, TemplateDefinition templateDefinition) : base(templateDefinition) { _zone = zone; } public override ViewRendering GetChildViewRendering(Control control) { if (control == null) { throw new ArgumentNullException("control"); } DesignerEditorPartChrome chrome = new DesignerEditorPartChrome(_zone); return chrome.GetViewRendering(control); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
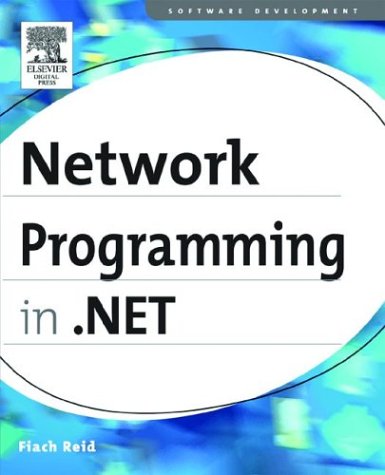
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpStreamFormatter.cs
- ManagementObject.cs
- BinaryUtilClasses.cs
- ToolStripRenderEventArgs.cs
- SegmentInfo.cs
- SafeProcessHandle.cs
- StorageEntityTypeMapping.cs
- GestureRecognizer.cs
- Invariant.cs
- AdornerDecorator.cs
- EventRouteFactory.cs
- BasicExpandProvider.cs
- OdbcEnvironmentHandle.cs
- SpinWait.cs
- HashAlgorithm.cs
- ChameleonKey.cs
- ControlEvent.cs
- Stroke2.cs
- formatter.cs
- FtpCachePolicyElement.cs
- SafeBitVector32.cs
- TextInfo.cs
- ConfigurationHelpers.cs
- TargetPerspective.cs
- RoleGroupCollectionEditor.cs
- PropertyTabAttribute.cs
- EnumConverter.cs
- ButtonBaseAdapter.cs
- ToolStripRenderEventArgs.cs
- ServiceDesigner.xaml.cs
- StackBuilderSink.cs
- odbcmetadatacollectionnames.cs
- MethodResolver.cs
- InheritablePropertyChangeInfo.cs
- BordersPage.cs
- HMACSHA256.cs
- XamlReaderHelper.cs
- DynamicMethod.cs
- DataSourceProvider.cs
- Rect.cs
- ApplicationHost.cs
- Marshal.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- ClientSettingsProvider.cs
- CryptoStream.cs
- Walker.cs
- TableParaClient.cs
- DecimalAnimationUsingKeyFrames.cs
- WebPartManagerDesigner.cs
- KeyInterop.cs
- TypeExtensionConverter.cs
- DefaultTextStore.cs
- VirtualPathUtility.cs
- ListControl.cs
- XmlCDATASection.cs
- DeploymentSection.cs
- pingexception.cs
- BindingMemberInfo.cs
- ExtendedPropertyCollection.cs
- CodeComment.cs
- BitmapCache.cs
- Vector3dCollection.cs
- ReadOnlyDataSourceView.cs
- QilGeneratorEnv.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- ThreadStateException.cs
- DataGridViewColumn.cs
- EntityDataSourceWrapper.cs
- IPipelineRuntime.cs
- ContractSearchPattern.cs
- CodeActivity.cs
- DataGridToolTip.cs
- CreateCardRequest.cs
- TableItemPattern.cs
- TextEditorMouse.cs
- RelatedCurrencyManager.cs
- LineUtil.cs
- AudioStateChangedEventArgs.cs
- Condition.cs
- CommandValueSerializer.cs
- DetailsViewUpdatedEventArgs.cs
- DataContract.cs
- Journaling.cs
- XmlWrappingWriter.cs
- safemediahandle.cs
- AlignmentXValidation.cs
- OdbcConnectionOpen.cs
- ArraySortHelper.cs
- NTAccount.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- QueryOperationResponseOfT.cs
- SqlConnectionFactory.cs
- DLinqAssociationProvider.cs
- SHA1CryptoServiceProvider.cs
- ContentElement.cs
- ClientConfigPaths.cs
- BaseCodePageEncoding.cs
- StrongNameKeyPair.cs
- PathFigureCollectionValueSerializer.cs
- DbInsertCommandTree.cs