Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / Mail / EncodedStreamFactory.cs / 1305376 / EncodedStreamFactory.cs
namespace System.Net.Mime { using System; using System.IO; using System.Text; internal class EncodedStreamFactory { //RFC 2822: no encoded-word line should be longer than 76 characters not including the soft CRLF //since the header length is unknown (if there even is one) we're going to be slightly more conservative //and cut off at 70. This will also prevent any other folding behavior from being triggered anywhere //in the code private const int defaultMaxLineLength = 70; //default buffer size for encoder private const int initialBufferSize = 1024; internal static int DefaultMaxLineLength { get { return defaultMaxLineLength; } } //get a raw encoder, not for use with header encoding internal IEncodableStream GetEncoder(TransferEncoding encoding, Stream stream) { //raw encoder if (encoding == TransferEncoding.Base64) return new Base64Stream(stream, new Base64WriteStateInfo(initialBufferSize, new byte[0], new byte[0], DefaultMaxLineLength)); //return a QuotedPrintable stream because this is not being used for header encoding if (encoding == TransferEncoding.QuotedPrintable) return new QuotedPrintableStream(stream, true); if (encoding == TransferEncoding.SevenBit) return new SevenBitStream(stream); throw new NotSupportedException("Encoding Stream"); } //use for encoding headers internal IEncodableStream GetEncoderForHeader(Encoding encoding, bool useBase64Encoding, int headerTextLength) { WriteStateInfoBase writeState; byte[] header = CreateHeader(encoding, useBase64Encoding); byte[] footer = CreateFooter(); if (useBase64Encoding) { writeState = new Base64WriteStateInfo(initialBufferSize, header, footer, DefaultMaxLineLength); writeState.MimeHeaderLength = headerTextLength; return new Base64Stream((Base64WriteStateInfo)writeState); } writeState = new QuotedStringWriteStateInfo(initialBufferSize, header, footer, DefaultMaxLineLength); writeState.MimeHeaderLength = headerTextLength; return new QEncodedStream((QuotedStringWriteStateInfo)writeState); } //Create the header for what type of byte encoding is going to be used //based on the encoding type and if base64 encoding should be forced //sample header: =?utf-8?B? protected byte[] CreateHeader(Encoding encoding, bool useBase64Encoding) { //create encoded work header string header = String.Format("=?{0}?{1}?", encoding.HeaderName, useBase64Encoding ? "B" : "Q"); return Encoding.ASCII.GetBytes(header); } //creates the footer that marks the end of a quoted string of some sort protected byte[] CreateFooter() { byte[] footer = {(byte)'?', (byte)'='}; return footer; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Net.Mime { using System; using System.IO; using System.Text; internal class EncodedStreamFactory { //RFC 2822: no encoded-word line should be longer than 76 characters not including the soft CRLF //since the header length is unknown (if there even is one) we're going to be slightly more conservative //and cut off at 70. This will also prevent any other folding behavior from being triggered anywhere //in the code private const int defaultMaxLineLength = 70; //default buffer size for encoder private const int initialBufferSize = 1024; internal static int DefaultMaxLineLength { get { return defaultMaxLineLength; } } //get a raw encoder, not for use with header encoding internal IEncodableStream GetEncoder(TransferEncoding encoding, Stream stream) { //raw encoder if (encoding == TransferEncoding.Base64) return new Base64Stream(stream, new Base64WriteStateInfo(initialBufferSize, new byte[0], new byte[0], DefaultMaxLineLength)); //return a QuotedPrintable stream because this is not being used for header encoding if (encoding == TransferEncoding.QuotedPrintable) return new QuotedPrintableStream(stream, true); if (encoding == TransferEncoding.SevenBit) return new SevenBitStream(stream); throw new NotSupportedException("Encoding Stream"); } //use for encoding headers internal IEncodableStream GetEncoderForHeader(Encoding encoding, bool useBase64Encoding, int headerTextLength) { WriteStateInfoBase writeState; byte[] header = CreateHeader(encoding, useBase64Encoding); byte[] footer = CreateFooter(); if (useBase64Encoding) { writeState = new Base64WriteStateInfo(initialBufferSize, header, footer, DefaultMaxLineLength); writeState.MimeHeaderLength = headerTextLength; return new Base64Stream((Base64WriteStateInfo)writeState); } writeState = new QuotedStringWriteStateInfo(initialBufferSize, header, footer, DefaultMaxLineLength); writeState.MimeHeaderLength = headerTextLength; return new QEncodedStream((QuotedStringWriteStateInfo)writeState); } //Create the header for what type of byte encoding is going to be used //based on the encoding type and if base64 encoding should be forced //sample header: =?utf-8?B? protected byte[] CreateHeader(Encoding encoding, bool useBase64Encoding) { //create encoded work header string header = String.Format("=?{0}?{1}?", encoding.HeaderName, useBase64Encoding ? "B" : "Q"); return Encoding.ASCII.GetBytes(header); } //creates the footer that marks the end of a quoted string of some sort protected byte[] CreateFooter() { byte[] footer = {(byte)'?', (byte)'='}; return footer; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
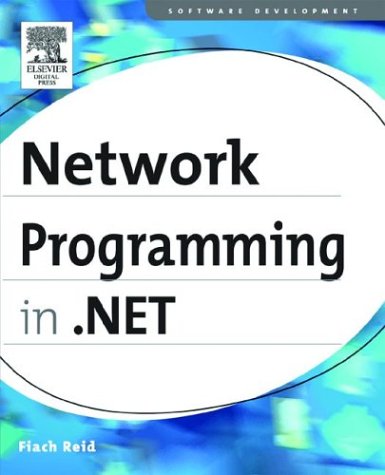
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClipboardProcessor.cs
- DynamicControlParameter.cs
- ThemeDirectoryCompiler.cs
- WorkItem.cs
- UriParserTemplates.cs
- HtmlInputSubmit.cs
- CompareValidator.cs
- BitSet.cs
- FieldMetadata.cs
- Point.cs
- EditorZoneBase.cs
- TrackingStringDictionary.cs
- AnnouncementInnerClientCD1.cs
- GCHandleCookieTable.cs
- SpecialFolderEnumConverter.cs
- PrivilegeNotHeldException.cs
- XamlWriterExtensions.cs
- RewritingProcessor.cs
- StandardBindingElementCollection.cs
- ProfileElement.cs
- IPEndPoint.cs
- BitmapCacheBrush.cs
- PocoEntityKeyStrategy.cs
- ErrorHandlingReceiver.cs
- XsdDataContractImporter.cs
- InternalsVisibleToAttribute.cs
- DataSourceExpression.cs
- Label.cs
- CqlQuery.cs
- MetafileEditor.cs
- RepeatInfo.cs
- XmlSchemaElement.cs
- BlockCollection.cs
- EntryIndex.cs
- BookmarkManager.cs
- DateRangeEvent.cs
- NetworkInformationPermission.cs
- AttributeTableBuilder.cs
- WindowsNonControl.cs
- ThreadExceptionEvent.cs
- SiteMapDataSource.cs
- ByteAnimation.cs
- BamlTreeUpdater.cs
- WebConfigurationFileMap.cs
- SyncOperationState.cs
- VersionedStreamOwner.cs
- RotationValidation.cs
- HwndProxyElementProvider.cs
- WindowsListViewGroupHelper.cs
- InkCanvasSelection.cs
- HtmlEncodedRawTextWriter.cs
- EntityDescriptor.cs
- ListViewDeleteEventArgs.cs
- TimeBoundedCache.cs
- CatalogPartCollection.cs
- OutputCacheModule.cs
- LogWriteRestartAreaAsyncResult.cs
- iisPickupDirectory.cs
- RolePrincipal.cs
- DateTimeFormatInfo.cs
- DockAndAnchorLayout.cs
- LineSegment.cs
- HandlerFactoryCache.cs
- BamlCollectionHolder.cs
- ContextStack.cs
- RemoveStoryboard.cs
- ToolBarButtonClickEvent.cs
- ActivitySurrogate.cs
- QueryInterceptorAttribute.cs
- EntityObject.cs
- XmlUnspecifiedAttribute.cs
- DataListItemCollection.cs
- RemotingHelper.cs
- httpstaticobjectscollection.cs
- AttachedPropertyBrowsableAttribute.cs
- UIElement3D.cs
- ResourceSet.cs
- ListDataBindEventArgs.cs
- AbsoluteQuery.cs
- PathParser.cs
- TemplateApplicationHelper.cs
- WindowsContainer.cs
- ObjectQuery_EntitySqlExtensions.cs
- XmlComment.cs
- RangeValuePattern.cs
- TextClipboardData.cs
- EntityStoreSchemaFilterEntry.cs
- Expression.cs
- LocatorManager.cs
- LZCodec.cs
- DocumentCollection.cs
- WebScriptMetadataMessageEncodingBindingElement.cs
- TabControl.cs
- HttpConfigurationSystem.cs
- JoinGraph.cs
- TitleStyle.cs
- TextElement.cs
- SqlUtils.cs
- TextBoxLine.cs
- ImageBrush.cs