Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / Ink / TextClipboardData.cs / 1 / TextClipboardData.cs
//---------------------------------------------------------------------------- // // File: TextClipboardData.cs // // Description: // A base class which can copy a unicode text to the IDataObject. // It also can get the unicode text from the IDataObject and create a corresponding textbox. // // Features: // // History: // 11/17/2004 [....]: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Windows; using System.Windows.Controls; using System.Windows.Markup; using System.Text; namespace MS.Internal.Ink { internal class TextClipboardData : ElementsClipboardData { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors // The default constructor internal TextClipboardData() : this(null) {} // The constructor with a string as argument internal TextClipboardData(string text) { _text = text; } #endregion Constructors // Checks if the data can be pasted. internal override bool CanPaste(IDataObject dataObject) { return ( dataObject.GetDataPresent(DataFormats.UnicodeText, false) || dataObject.GetDataPresent(DataFormats.Text, false) || dataObject.GetDataPresent(DataFormats.OemText, false) ); } //-------------------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------------------- #region Protected Methods // Checks if the data can be copied. protected override bool CanCopy() { return !string.IsNullOrEmpty(_text); } // Copy the text to the IDataObject protected override void DoCopy(IDataObject dataObject) { // Put the text to the clipboard dataObject.SetData(DataFormats.UnicodeText, _text, true); } // Retrieves the text from the IDataObject instance. // Then create a textbox with the text data. protected override void DoPaste(IDataObject dataObject) { ElementList = new List(); // Get the string from the data object. string text = dataObject.GetData(DataFormats.UnicodeText, true) as string; if ( String.IsNullOrEmpty(text) ) { // OemText can be retrieved as CF_TEXT. text = dataObject.GetData(DataFormats.Text, true) as string; } if ( !String.IsNullOrEmpty(text) ) { // Now, create a text box and set the text to it. TextBox textBox = new TextBox(); textBox.Text = text; textBox.TextWrapping = TextWrapping.Wrap; // Add the textbox to the internal array list. ElementList.Add(textBox); } } #endregion Protected Methods //-------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private string _text; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
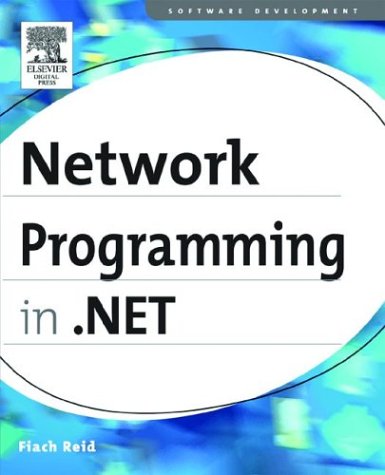
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NativeMethods.cs
- BooleanProjectedSlot.cs
- ComponentRenameEvent.cs
- SBCSCodePageEncoding.cs
- Storyboard.cs
- PersonalizationDictionary.cs
- _IPv4Address.cs
- ExtenderControl.cs
- TraceLevelStore.cs
- DataSetMappper.cs
- _CookieModule.cs
- PrimitiveCodeDomSerializer.cs
- DataGridParentRows.cs
- ImageSource.cs
- EventRecordWrittenEventArgs.cs
- Message.cs
- Style.cs
- IPAddress.cs
- HttpListener.cs
- NullReferenceException.cs
- WriterOutput.cs
- DBDataPermissionAttribute.cs
- EndpointBehaviorElementCollection.cs
- SchemaLookupTable.cs
- TableCell.cs
- PreviewPrintController.cs
- Italic.cs
- RegexCharClass.cs
- CodeLinePragma.cs
- GridViewItemAutomationPeer.cs
- JsonMessageEncoderFactory.cs
- TraversalRequest.cs
- ResourceDescriptionAttribute.cs
- TdsParserSafeHandles.cs
- PropertyToken.cs
- PreviewPrintController.cs
- FixedSOMImage.cs
- HttpWebResponse.cs
- DataListItemEventArgs.cs
- RadioButtonBaseAdapter.cs
- GenerateTemporaryTargetAssembly.cs
- TreeViewImageIndexConverter.cs
- ObjectItemConventionAssemblyLoader.cs
- DataDocumentXPathNavigator.cs
- DataSet.cs
- designeractionbehavior.cs
- StreamResourceInfo.cs
- ObjectHelper.cs
- EntityContainerEmitter.cs
- Panel.cs
- Rotation3D.cs
- Math.cs
- NegationPusher.cs
- Command.cs
- Path.cs
- SemanticTag.cs
- AnonymousIdentificationSection.cs
- WebPartRestoreVerb.cs
- CodeNamespaceCollection.cs
- HelpEvent.cs
- FormViewAutoFormat.cs
- UInt16.cs
- SolidBrush.cs
- AvTrace.cs
- TdsRecordBufferSetter.cs
- SBCSCodePageEncoding.cs
- DispatcherExceptionEventArgs.cs
- designeractionlistschangedeventargs.cs
- IdleTimeoutMonitor.cs
- SystemFonts.cs
- SpellerError.cs
- ExpressionBuilderCollection.cs
- TakeOrSkipWhileQueryOperator.cs
- XmlAttributeProperties.cs
- NativeStructs.cs
- Oid.cs
- ToolStripItemTextRenderEventArgs.cs
- DataGridViewTextBoxColumn.cs
- StylusLogic.cs
- DataListCommandEventArgs.cs
- RuntimeHelpers.cs
- CodeBlockBuilder.cs
- SendActivityDesigner.cs
- PerfCounterSection.cs
- InfoCardClaim.cs
- Variant.cs
- UnmanagedMarshal.cs
- ToolStripMenuItemDesigner.cs
- StylusPointDescription.cs
- CompletedAsyncResult.cs
- ScaleTransform3D.cs
- EnvironmentPermission.cs
- ReliableRequestSessionChannel.cs
- TimeSpanOrInfiniteConverter.cs
- cache.cs
- Size.cs
- ColorContext.cs
- SessionIDManager.cs
- Stacktrace.cs
- SqlBuffer.cs