Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / SchemaLookupTable.cs / 2 / SchemaLookupTable.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Data; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { ////// Reponsible for keep map from alias to namespace for a given schema. /// internal sealed class AliasResolver { #region Fields private Dictionary_aliasToNamespaceMap = new Dictionary (StringComparer.Ordinal); private List _usingElementCollection = new List (); private Schema _definingSchema; #endregion #region Public Methods /// /// Construct the LookUp table /// public AliasResolver(Schema schema) { _definingSchema = schema; // If there is an alias defined for the defining schema, // add it to the look up table if (!string.IsNullOrEmpty(schema.Alias)) { _aliasToNamespaceMap.Add(schema.Alias, schema.Namespace); } } ////// Add a ReferenceSchema to the table /// /// the ReferenceSchema to add public void Add(UsingElement usingElement) { Debug.Assert(usingElement != null, "refSchema parameter is null"); string newNamespace = usingElement.NamespaceName; string newAlias = usingElement.Alias; // Check whether the alias is a reserved keyword if (CheckForSystemNamespace(usingElement, newAlias, NameKind.Alias)) { newAlias = null; } //Check whether the namespace is a reserved keyword if (CheckForSystemNamespace(usingElement, newNamespace, NameKind.Namespace)) { newNamespace = null; } // see if the alias has already been used if (newAlias != null && _aliasToNamespaceMap.ContainsKey(newAlias)) { // it has, issue an error and make sure we don't try to add it usingElement.AddError(ErrorCode.AlreadyDefined, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.AliasNameIsAlreadyDefined(newAlias)); newAlias = null; } // Its okay if they add the same namespace twice, until they have different alias // if there's an alias, add it. if (newAlias != null) { _aliasToNamespaceMap.Add(newAlias, newNamespace); _usingElementCollection.Add(usingElement); } } ////// Get the Schema(s) a namespace or alias might refer to /// returned schemas may be null is called before or during Schema Resolution /// /// the name to look up ///array of schemas (0 length if no schemas match name public bool TryResolveAlias(string alias, out string namespaceName) { Debug.Assert(!String.IsNullOrEmpty(alias), "alias must never be null"); // Check if there is an alias defined with this name return _aliasToNamespaceMap.TryGetValue(alias, out namespaceName); } ////// Resolves all the namespace specified in the using elements in this schema /// public void ResolveNamespaces() { foreach (UsingElement usingElement in _usingElementCollection) { if (!_definingSchema.SchemaManager.IsValidNamespaceName(usingElement.NamespaceName)) { usingElement.AddError(ErrorCode.InvalidNamespaceInUsing, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidNamespaceInUsing(usingElement.NamespaceName)); } } } #endregion #region Private Methods ////// Check if the given name is a reserved keyword. if yes, add appropriate error to the refschema /// /// /// /// ///private bool CheckForSystemNamespace(UsingElement refSchema, string name, NameKind nameKind) { Debug.Assert(_definingSchema.ProviderManifest != null, "Since we don't allow using elements in provider manifest, provider manifest can never be null"); // We need to check for system namespace if (EdmItemCollection.IsSystemNamespace(_definingSchema.ProviderManifest, name)) { if (nameKind == NameKind.Alias) { refSchema.AddError(ErrorCode.CannotUseSystemNamespaceAsAlias, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.CannotUseSystemNamespaceAsAlias(name)); } else { refSchema.AddError(ErrorCode.NeedNotUseSystemNamespaceInUsing, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.NeedNotUseSystemNamespaceInUsing(name)); } return true; } return false; } #endregion #region Private Types /// /// Kind of Name /// private enum NameKind { ///It's an Alias Alias, ///It's a namespace Namespace, } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Data; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { ////// Reponsible for keep map from alias to namespace for a given schema. /// internal sealed class AliasResolver { #region Fields private Dictionary_aliasToNamespaceMap = new Dictionary (StringComparer.Ordinal); private List _usingElementCollection = new List (); private Schema _definingSchema; #endregion #region Public Methods /// /// Construct the LookUp table /// public AliasResolver(Schema schema) { _definingSchema = schema; // If there is an alias defined for the defining schema, // add it to the look up table if (!string.IsNullOrEmpty(schema.Alias)) { _aliasToNamespaceMap.Add(schema.Alias, schema.Namespace); } } ////// Add a ReferenceSchema to the table /// /// the ReferenceSchema to add public void Add(UsingElement usingElement) { Debug.Assert(usingElement != null, "refSchema parameter is null"); string newNamespace = usingElement.NamespaceName; string newAlias = usingElement.Alias; // Check whether the alias is a reserved keyword if (CheckForSystemNamespace(usingElement, newAlias, NameKind.Alias)) { newAlias = null; } //Check whether the namespace is a reserved keyword if (CheckForSystemNamespace(usingElement, newNamespace, NameKind.Namespace)) { newNamespace = null; } // see if the alias has already been used if (newAlias != null && _aliasToNamespaceMap.ContainsKey(newAlias)) { // it has, issue an error and make sure we don't try to add it usingElement.AddError(ErrorCode.AlreadyDefined, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.AliasNameIsAlreadyDefined(newAlias)); newAlias = null; } // Its okay if they add the same namespace twice, until they have different alias // if there's an alias, add it. if (newAlias != null) { _aliasToNamespaceMap.Add(newAlias, newNamespace); _usingElementCollection.Add(usingElement); } } ////// Get the Schema(s) a namespace or alias might refer to /// returned schemas may be null is called before or during Schema Resolution /// /// the name to look up ///array of schemas (0 length if no schemas match name public bool TryResolveAlias(string alias, out string namespaceName) { Debug.Assert(!String.IsNullOrEmpty(alias), "alias must never be null"); // Check if there is an alias defined with this name return _aliasToNamespaceMap.TryGetValue(alias, out namespaceName); } ////// Resolves all the namespace specified in the using elements in this schema /// public void ResolveNamespaces() { foreach (UsingElement usingElement in _usingElementCollection) { if (!_definingSchema.SchemaManager.IsValidNamespaceName(usingElement.NamespaceName)) { usingElement.AddError(ErrorCode.InvalidNamespaceInUsing, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidNamespaceInUsing(usingElement.NamespaceName)); } } } #endregion #region Private Methods ////// Check if the given name is a reserved keyword. if yes, add appropriate error to the refschema /// /// /// /// ///private bool CheckForSystemNamespace(UsingElement refSchema, string name, NameKind nameKind) { Debug.Assert(_definingSchema.ProviderManifest != null, "Since we don't allow using elements in provider manifest, provider manifest can never be null"); // We need to check for system namespace if (EdmItemCollection.IsSystemNamespace(_definingSchema.ProviderManifest, name)) { if (nameKind == NameKind.Alias) { refSchema.AddError(ErrorCode.CannotUseSystemNamespaceAsAlias, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.CannotUseSystemNamespaceAsAlias(name)); } else { refSchema.AddError(ErrorCode.NeedNotUseSystemNamespaceInUsing, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.NeedNotUseSystemNamespaceInUsing(name)); } return true; } return false; } #endregion #region Private Types /// /// Kind of Name /// private enum NameKind { ///It's an Alias Alias, ///It's a namespace Namespace, } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
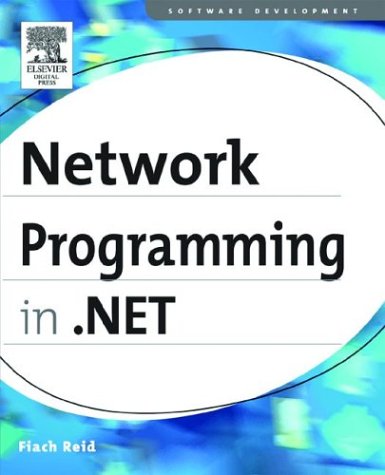
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnsafeNativeMethods.cs
- ParentControlDesigner.cs
- EventListenerClientSide.cs
- ValidationRule.cs
- ColorConvertedBitmapExtension.cs
- Or.cs
- IgnoreFlushAndCloseStream.cs
- SqlDataSourceFilteringEventArgs.cs
- DataRelationCollection.cs
- DocumentViewerBase.cs
- PingOptions.cs
- StateDesigner.Helpers.cs
- XmlSchemaAnnotation.cs
- DataTableCollection.cs
- UnregisterInfo.cs
- ToolStripDropDown.cs
- BehaviorEditorPart.cs
- ScriptReferenceEventArgs.cs
- NativeMethods.cs
- ReflectionServiceProvider.cs
- ContextQuery.cs
- BaseInfoTable.cs
- FileRecordSequenceHelper.cs
- DataGridViewRowPostPaintEventArgs.cs
- GridPatternIdentifiers.cs
- SmtpNetworkElement.cs
- ListItemCollection.cs
- TemplateInstanceAttribute.cs
- IsolatedStorageFile.cs
- IItemContainerGenerator.cs
- _LazyAsyncResult.cs
- SrgsElementFactoryCompiler.cs
- GridViewDeletedEventArgs.cs
- CodeFieldReferenceExpression.cs
- XmlTypeMapping.cs
- SafeBitVector32.cs
- Int32Storage.cs
- SoapIncludeAttribute.cs
- DesignerActionGlyph.cs
- GeneratedCodeAttribute.cs
- safelink.cs
- Win32Native.cs
- RoleProviderPrincipal.cs
- ColumnPropertiesGroup.cs
- TextMetrics.cs
- DefaultValueMapping.cs
- hwndwrapper.cs
- TreeNodeCollection.cs
- UntrustedRecipientException.cs
- ellipse.cs
- ApplicationCommands.cs
- MetadataArtifactLoaderResource.cs
- PropertyFilter.cs
- GestureRecognizer.cs
- StringArrayEditor.cs
- FormViewPageEventArgs.cs
- EmptyStringExpandableObjectConverter.cs
- SQLSingle.cs
- WindowsAuthenticationEventArgs.cs
- ApplicationId.cs
- SendParametersContent.cs
- PenCursorManager.cs
- DocumentEventArgs.cs
- RequestCachePolicyConverter.cs
- SqlXmlStorage.cs
- FormViewPageEventArgs.cs
- RoutedEvent.cs
- RegexWorker.cs
- SmuggledIUnknown.cs
- Region.cs
- InvokeHandlers.cs
- HttpCookieCollection.cs
- CombinedGeometry.cs
- SoapSchemaMember.cs
- CustomAssemblyResolver.cs
- FunctionCommandText.cs
- ScaleTransform3D.cs
- PageContentCollection.cs
- TableLayoutPanel.cs
- OutputScope.cs
- DeviceOverridableAttribute.cs
- dsa.cs
- DataConnectionHelper.cs
- XmlArrayItemAttributes.cs
- BStrWrapper.cs
- PersonalizableAttribute.cs
- IndexedGlyphRun.cs
- Span.cs
- BaseDataBoundControl.cs
- SymbolPair.cs
- EntityStoreSchemaGenerator.cs
- HttpException.cs
- ObjectConverter.cs
- TextTabProperties.cs
- InkCanvasInnerCanvas.cs
- PrimitiveSchema.cs
- EpmAttributeNameBuilder.cs
- TextContainerHelper.cs
- SQLInt16.cs
- TemplatedMailWebEventProvider.cs