Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / XPath / Internal / ContextQuery.cs / 1305376 / ContextQuery.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; internal class ContextQuery : Query { protected XPathNavigator contextNode; public ContextQuery() { this.count = 0; } protected ContextQuery(ContextQuery other) : base(other) { this.contextNode = other.contextNode; // Don't need to clone here } public override void Reset() { count = 0; } public override XPathNavigator Current { get { return contextNode; } } public override object Evaluate(XPathNodeIterator context) { contextNode = context.Current; // We don't clone here. Because we never move it. count = 0; return this; } public override XPathNavigator Advance() { if (count == 0) { count = 1; return contextNode; } return null; } public override XPathNavigator MatchNode(XPathNavigator current) { return current; } public override XPathNodeIterator Clone() { return new ContextQuery(this); } public override XPathResultType StaticType { get { return XPathResultType.NodeSet; } } public override int CurrentPosition { get { return count; } } public override int Count { get { return 1; } } public override QueryProps Properties { get { return QueryProps.Merge | QueryProps.Cached | QueryProps.Position | QueryProps.Count; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
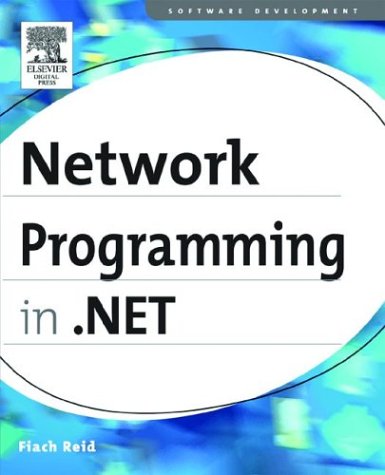
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerToPeerException.cs
- DataGridViewRowCollection.cs
- SqlServer2KCompatibilityCheck.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- HtmlAnchor.cs
- designeractionlistschangedeventargs.cs
- _IPv6Address.cs
- PanningMessageFilter.cs
- ToolBarButton.cs
- OdbcHandle.cs
- Queue.cs
- TextStore.cs
- _AutoWebProxyScriptWrapper.cs
- ConfigXmlWhitespace.cs
- RotateTransform.cs
- AssemblyInfo.cs
- smtpconnection.cs
- ToolStripItemImageRenderEventArgs.cs
- SqlDataReaderSmi.cs
- ExpanderAutomationPeer.cs
- DocumentPageView.cs
- SerialErrors.cs
- IProvider.cs
- basemetadatamappingvisitor.cs
- safex509handles.cs
- MenuItemBindingCollection.cs
- SchemaType.cs
- BlurBitmapEffect.cs
- XmlChoiceIdentifierAttribute.cs
- WebPartEditVerb.cs
- CompressedStack.cs
- SecurityUtils.cs
- MachineKeySection.cs
- BaseParagraph.cs
- PropertyConverter.cs
- WinEventHandler.cs
- HtmlInputPassword.cs
- DescendantBaseQuery.cs
- ThaiBuddhistCalendar.cs
- WindowsRebar.cs
- ResourceCategoryAttribute.cs
- XmlDigitalSignatureProcessor.cs
- WebRequestModuleElement.cs
- FileUpload.cs
- ResourcePart.cs
- FieldNameLookup.cs
- NamespaceList.cs
- TypedLocationWrapper.cs
- ServiceDefaults.cs
- EntityObject.cs
- Int64Animation.cs
- BehaviorEditorPart.cs
- SecurityPolicySection.cs
- ServicePerformanceCounters.cs
- StreamGeometry.cs
- AuthenticateEventArgs.cs
- XmlDocumentFieldSchema.cs
- SuppressMergeCheckAttribute.cs
- XmlIncludeAttribute.cs
- FSWPathEditor.cs
- Effect.cs
- XmlSchemaAnnotated.cs
- MULTI_QI.cs
- ServiceReference.cs
- LinqDataSourceContextEventArgs.cs
- arc.cs
- RegistryDataKey.cs
- ListControl.cs
- ConfigXmlAttribute.cs
- securitycriticaldataformultiplegetandset.cs
- TraceSwitch.cs
- QueryPageSettingsEventArgs.cs
- TimeoutHelper.cs
- ConfigViewGenerator.cs
- Pen.cs
- EncryptedKeyHashIdentifierClause.cs
- UIElementCollection.cs
- TableLayoutSettingsTypeConverter.cs
- XmlQuerySequence.cs
- FormatException.cs
- RIPEMD160.cs
- CounterCreationData.cs
- ExpressionPrefixAttribute.cs
- OutputCacheSettingsSection.cs
- CultureTable.cs
- ParentQuery.cs
- CollectionView.cs
- DataGridViewRowsAddedEventArgs.cs
- WebDisplayNameAttribute.cs
- ResourceDisplayNameAttribute.cs
- ParameterExpression.cs
- FragmentQueryProcessor.cs
- DbReferenceCollection.cs
- ContentPathSegment.cs
- TreeIterator.cs
- NativeWrapper.cs
- WindowsIPAddress.cs
- InkSerializer.cs
- XmlSchemaSimpleType.cs
- securitymgrsite.cs