Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Data / System / Data / DataViewManagerListItemTypeDescriptor.cs / 1 / DataViewManagerListItemTypeDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.ComponentModel; ////// internal sealed class DataViewManagerListItemTypeDescriptor : ICustomTypeDescriptor { private DataViewManager dataViewManager; private PropertyDescriptorCollection propsCollection; internal DataViewManagerListItemTypeDescriptor(DataViewManager dataViewManager) { this.dataViewManager = dataViewManager; } internal void Reset() { propsCollection = null; } internal DataView GetDataView(DataTable table) { DataView dataView = new DataView(table); dataView.SetDataViewManager(dataViewManager); return dataView; } ///[To be supplied.] ////// Retrieves an array of member attributes for the given object. /// AttributeCollection ICustomTypeDescriptor.GetAttributes() { return new AttributeCollection((Attribute[])null); } ////// Retrieves the class name for this object. If null is returned, /// the type name is used. /// string ICustomTypeDescriptor.GetClassName() { return null; } ////// Retrieves the name for this object. If null is returned, /// the default is used. /// string ICustomTypeDescriptor.GetComponentName() { return null; } ////// Retrieves the type converter for this object. /// TypeConverter ICustomTypeDescriptor.GetConverter() { return null; } ////// Retrieves the default event. /// EventDescriptor ICustomTypeDescriptor.GetDefaultEvent() { return null; } ////// Retrieves the default property. /// PropertyDescriptor ICustomTypeDescriptor.GetDefaultProperty() { return null; } ////// Retrieves the an editor for this object. /// object ICustomTypeDescriptor.GetEditor(Type editorBaseType) { return null; } ////// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. /// EventDescriptorCollection ICustomTypeDescriptor.GetEvents() { return new EventDescriptorCollection(null); } ////// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. The returned array of events will be /// filtered by the given set of attributes. /// EventDescriptorCollection ICustomTypeDescriptor.GetEvents(Attribute[] attributes) { return new EventDescriptorCollection(null); } ////// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. /// PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties() { return((ICustomTypeDescriptor)this).GetProperties(null); } ////// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. The returned array of properties will be /// filtered by the given set of attributes. /// PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties(Attribute[] attributes) { if (propsCollection == null) { PropertyDescriptor[] props = null; DataSet dataSet = dataViewManager.DataSet; if (dataSet != null) { int tableCount = dataSet.Tables.Count; props = new PropertyDescriptor[tableCount]; for (int i = 0; i < tableCount; i++) { props[i] = new DataTablePropertyDescriptor(dataSet.Tables[i]); } } propsCollection = new PropertyDescriptorCollection(props); } return propsCollection; } ////// Retrieves the object that directly depends on this value being edited. This is /// generally the object that is required for the PropertyDescriptor's GetValue and SetValue /// methods. If 'null' is passed for the PropertyDescriptor, the ICustomComponent /// descripotor implemementation should return the default object, that is the main /// object that exposes the properties and attributes, /// object ICustomTypeDescriptor.GetPropertyOwner(PropertyDescriptor pd) { return this; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.ComponentModel; ////// internal sealed class DataViewManagerListItemTypeDescriptor : ICustomTypeDescriptor { private DataViewManager dataViewManager; private PropertyDescriptorCollection propsCollection; internal DataViewManagerListItemTypeDescriptor(DataViewManager dataViewManager) { this.dataViewManager = dataViewManager; } internal void Reset() { propsCollection = null; } internal DataView GetDataView(DataTable table) { DataView dataView = new DataView(table); dataView.SetDataViewManager(dataViewManager); return dataView; } ///[To be supplied.] ////// Retrieves an array of member attributes for the given object. /// AttributeCollection ICustomTypeDescriptor.GetAttributes() { return new AttributeCollection((Attribute[])null); } ////// Retrieves the class name for this object. If null is returned, /// the type name is used. /// string ICustomTypeDescriptor.GetClassName() { return null; } ////// Retrieves the name for this object. If null is returned, /// the default is used. /// string ICustomTypeDescriptor.GetComponentName() { return null; } ////// Retrieves the type converter for this object. /// TypeConverter ICustomTypeDescriptor.GetConverter() { return null; } ////// Retrieves the default event. /// EventDescriptor ICustomTypeDescriptor.GetDefaultEvent() { return null; } ////// Retrieves the default property. /// PropertyDescriptor ICustomTypeDescriptor.GetDefaultProperty() { return null; } ////// Retrieves the an editor for this object. /// object ICustomTypeDescriptor.GetEditor(Type editorBaseType) { return null; } ////// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. /// EventDescriptorCollection ICustomTypeDescriptor.GetEvents() { return new EventDescriptorCollection(null); } ////// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. The returned array of events will be /// filtered by the given set of attributes. /// EventDescriptorCollection ICustomTypeDescriptor.GetEvents(Attribute[] attributes) { return new EventDescriptorCollection(null); } ////// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. /// PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties() { return((ICustomTypeDescriptor)this).GetProperties(null); } ////// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. The returned array of properties will be /// filtered by the given set of attributes. /// PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties(Attribute[] attributes) { if (propsCollection == null) { PropertyDescriptor[] props = null; DataSet dataSet = dataViewManager.DataSet; if (dataSet != null) { int tableCount = dataSet.Tables.Count; props = new PropertyDescriptor[tableCount]; for (int i = 0; i < tableCount; i++) { props[i] = new DataTablePropertyDescriptor(dataSet.Tables[i]); } } propsCollection = new PropertyDescriptorCollection(props); } return propsCollection; } ////// Retrieves the object that directly depends on this value being edited. This is /// generally the object that is required for the PropertyDescriptor's GetValue and SetValue /// methods. If 'null' is passed for the PropertyDescriptor, the ICustomComponent /// descripotor implemementation should return the default object, that is the main /// object that exposes the properties and attributes, /// object ICustomTypeDescriptor.GetPropertyOwner(PropertyDescriptor pd) { return this; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
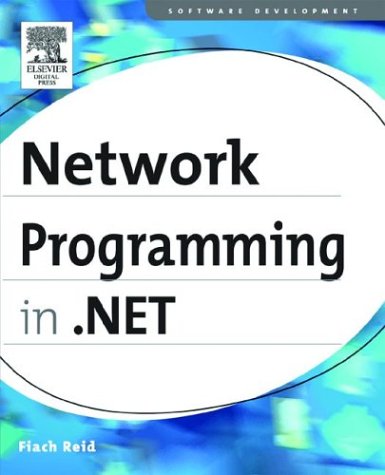
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServiceSaveChangesEventArgs.cs
- InsufficientMemoryException.cs
- CodeSubDirectory.cs
- documentsequencetextview.cs
- FixedTextView.cs
- _SafeNetHandles.cs
- EntityDataSourceColumn.cs
- UpdatePanelControlTrigger.cs
- DataGridViewSelectedRowCollection.cs
- HtmlTableRow.cs
- EditorPartCollection.cs
- CodeDirectionExpression.cs
- ManagedWndProcTracker.cs
- XmlnsDefinitionAttribute.cs
- MailBnfHelper.cs
- WebPartHelpVerb.cs
- Context.cs
- TimeZone.cs
- DesignerCommandSet.cs
- XmlSchemaSubstitutionGroup.cs
- DesignerSerializationOptionsAttribute.cs
- XMLSchema.cs
- CommandLibraryHelper.cs
- ObfuscateAssemblyAttribute.cs
- DesignBindingValueUIHandler.cs
- QilPatternVisitor.cs
- InvalidPrinterException.cs
- AutomationProperty.cs
- InlinedLocationReference.cs
- Point4DValueSerializer.cs
- XmlSchemaSimpleType.cs
- RectangleF.cs
- SharedStatics.cs
- FormsAuthenticationUser.cs
- KnownIds.cs
- CompressEmulationStream.cs
- PixelShader.cs
- Utils.cs
- PackageRelationshipCollection.cs
- QueuePropertyVariants.cs
- DataTrigger.cs
- BindToObject.cs
- AnnotationAuthorChangedEventArgs.cs
- XmlnsCache.cs
- Brush.cs
- XPathNavigatorKeyComparer.cs
- BridgeDataRecord.cs
- RoutedEvent.cs
- Image.cs
- OrderByQueryOptionExpression.cs
- MemoryStream.cs
- SmiXetterAccessMap.cs
- TrackingProfileManager.cs
- PropertyGrid.cs
- TextServicesLoader.cs
- Binding.cs
- WorkflowNamespace.cs
- MetafileHeader.cs
- CodeEventReferenceExpression.cs
- XmlFileEditor.cs
- StickyNoteContentControl.cs
- WebScriptEnablingBehavior.cs
- NumberFormatInfo.cs
- Site.cs
- Message.cs
- UnsafeNativeMethods.cs
- WrappedReader.cs
- OlePropertyStructs.cs
- URLString.cs
- TabPage.cs
- SapiGrammar.cs
- DbParameterCollection.cs
- ObjectStorage.cs
- RegexCompiler.cs
- PngBitmapEncoder.cs
- CodeSnippetCompileUnit.cs
- DesignerActionKeyboardBehavior.cs
- DataPagerField.cs
- XmlNodeChangedEventArgs.cs
- ListBase.cs
- XmlEnumAttribute.cs
- WorkflowOwnerAsyncResult.cs
- ResourceAttributes.cs
- StringExpressionSet.cs
- MethodCallConverter.cs
- PerfService.cs
- InstanceHandle.cs
- FontSourceCollection.cs
- MissingSatelliteAssemblyException.cs
- MenuItem.cs
- ConfigurationStrings.cs
- _TransmitFileOverlappedAsyncResult.cs
- TextWriterEngine.cs
- WpfKnownMember.cs
- CodeMethodReturnStatement.cs
- StylusButton.cs
- NavigationWindowAutomationPeer.cs
- FontFamilyConverter.cs
- ApplicationProxyInternal.cs
- RouteParser.cs