Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SapiInterop / SapiGrammar.cs / 1 / SapiGrammar.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // All the calls to SAPI interfaces are wrapped into the class 'SapiRecognizer', // 'SapiContext' and 'SapiGrammar'. // // The SAPI call are executed in the context of a proxy that is either a // pass-through or forward the request to an MTA thread for SAPI 5.1 // // History: // 4/1/2006 [....] //----------------------------------------------------------------- using System; using System.Collections.Generic; using System.Speech.Recognition; using System.Speech.Internal.ObjectTokens; using System.Runtime.InteropServices; using System.Text; namespace System.Speech.Internal.SapiInterop { internal class SapiGrammar : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal SapiGrammar (ISpRecoGrammar sapiGrammar, SapiProxy thread) { _sapiGrammar = sapiGrammar; _sapiProxy = thread; } public void Dispose () { if (!_disposed) { Marshal.ReleaseComObject (_sapiGrammar); GC.SuppressFinalize (this); _disposed = true; } } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods internal void SetGrammarState (SPGRAMMARSTATE state) { _sapiProxy.Invoke2 (delegate { _sapiGrammar.SetGrammarState (state); }); } internal void SetWordSequenceData (string text, SPTEXTSELECTIONINFO info) { SPTEXTSELECTIONINFO selectionInfo = info; _sapiProxy.Invoke2 (delegate { _sapiGrammar.SetWordSequenceData (text, (uint) text.Length, ref selectionInfo); }); } internal void LoadCmdFromMemory (IntPtr grammar, SPLOADOPTIONS options) { _sapiProxy.Invoke2 (delegate { _sapiGrammar.LoadCmdFromMemory (grammar, options); }); } internal void LoadDictation (string pszTopicName, SPLOADOPTIONS options) { _sapiProxy.Invoke2 (delegate { _sapiGrammar.LoadDictation (pszTopicName, options); }); } internal SAPIErrorCodes SetDictationState (SPRULESTATE state) { return (SAPIErrorCodes) _sapiProxy.Invoke (delegate { return _sapiGrammar.SetDictationState (state); }); } internal SAPIErrorCodes SetRuleState (string name, SPRULESTATE state) { return (SAPIErrorCodes) _sapiProxy.Invoke (delegate { return _sapiGrammar.SetRuleState (name, IntPtr.Zero, state); }); } /* * The Set of methods are only available with SAPI 5.3. There is no need then to use the SAPI proxy to switch * the call to an MTA thread. * */ internal void SetGrammarLoader (ISpGrammarResourceLoader resourceLoader) { SpRecoGrammar2.SetGrammarLoader (resourceLoader); } internal void LoadCmdFromMemory2 (IntPtr grammar, SPLOADOPTIONS options, string sharingUri, string baseUri) { SpRecoGrammar2.LoadCmdFromMemory2 (grammar, options, sharingUri, baseUri); } internal void SetRulePriority (string name, UInt32 id, Int32 priority) { SpRecoGrammar2.SetRulePriority (name, id, priority); } internal void SetRuleWeight (string name, UInt32 id, float weight) { SpRecoGrammar2.SetRuleWeight (name, id, weight); } internal void SetDictationWeight (float weight) { SpRecoGrammar2.SetDictationWeight (weight); } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal ISpRecoGrammar2 SpRecoGrammar2 { get { if (_sapiGrammar2 == null) { _sapiGrammar2 = (ISpRecoGrammar2) _sapiGrammar; } return (ISpRecoGrammar2) _sapiGrammar2; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Methods private ISpRecoGrammar2 _sapiGrammar2; private ISpRecoGrammar _sapiGrammar; private SapiProxy _sapiProxy; private bool _disposed; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
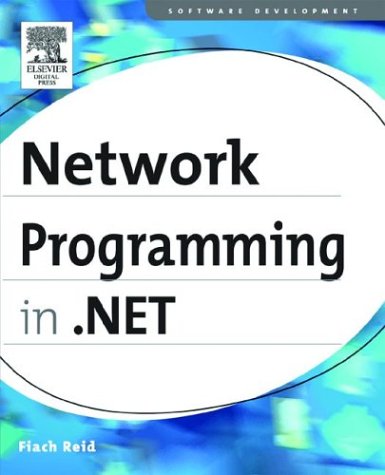
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PackageRelationshipCollection.cs
- SynchronizationContext.cs
- SerializationFieldInfo.cs
- RTLAwareMessageBox.cs
- EventsTab.cs
- KeyFrames.cs
- DebugControllerThread.cs
- ComplexTypeEmitter.cs
- EmptyStringExpandableObjectConverter.cs
- CharEntityEncoderFallback.cs
- TextBlockAutomationPeer.cs
- ParserStreamGeometryContext.cs
- SrgsItemList.cs
- NumberSubstitution.cs
- TypeGeneratedEventArgs.cs
- MatrixUtil.cs
- ModelPerspective.cs
- InternalControlCollection.cs
- RepeaterDesigner.cs
- TypedReference.cs
- SqlConnectionManager.cs
- IQueryable.cs
- EpmCustomContentWriterNodeData.cs
- HatchBrush.cs
- MarkupCompilePass1.cs
- TextTabProperties.cs
- ProxyManager.cs
- AutomationPattern.cs
- SslStreamSecurityUpgradeProvider.cs
- RadialGradientBrush.cs
- ProcessModelInfo.cs
- XmlJsonWriter.cs
- Parameter.cs
- BlurBitmapEffect.cs
- WinEventWrap.cs
- BamlTreeNode.cs
- SessionIDManager.cs
- ScopelessEnumAttribute.cs
- Quaternion.cs
- InternalsVisibleToAttribute.cs
- SelectionRangeConverter.cs
- DateTimeSerializationSection.cs
- NumericUpDown.cs
- SuppressIldasmAttribute.cs
- Registry.cs
- PasswordPropertyTextAttribute.cs
- CommonDialog.cs
- XmlSchemaValidator.cs
- DbConnectionFactory.cs
- MemoryRecordBuffer.cs
- BindingSourceDesigner.cs
- MenuDesigner.cs
- BuildManager.cs
- ContextBase.cs
- Attributes.cs
- XPathParser.cs
- RelationHandler.cs
- TemplateInstanceAttribute.cs
- IDReferencePropertyAttribute.cs
- UnsafeNativeMethods.cs
- SerializationFieldInfo.cs
- FixedSOMContainer.cs
- DbProviderSpecificTypePropertyAttribute.cs
- OracleConnection.cs
- RestHandler.cs
- CacheMemory.cs
- TextServicesManager.cs
- DeploymentSection.cs
- _Events.cs
- SecurityTokenReferenceStyle.cs
- ActivityExecutorDelegateInfo.cs
- SqlClientWrapperSmiStream.cs
- XsltInput.cs
- Claim.cs
- DefaultMergeHelper.cs
- SQLInt32Storage.cs
- RunWorkerCompletedEventArgs.cs
- MatrixConverter.cs
- WinInet.cs
- XPathPatternBuilder.cs
- AccessorTable.cs
- SerialPort.cs
- GradientStopCollection.cs
- SerializationException.cs
- SoapInteropTypes.cs
- OperationParameterInfo.cs
- NativeMethods.cs
- CriticalFinalizerObject.cs
- _SslStream.cs
- ComPlusInstanceContextInitializer.cs
- SafeProcessHandle.cs
- CodeDelegateInvokeExpression.cs
- IntegrationExceptionEventArgs.cs
- TdsRecordBufferSetter.cs
- ScriptServiceAttribute.cs
- MissingSatelliteAssemblyException.cs
- ManagedFilter.cs
- ResourceManagerWrapper.cs
- SerialPinChanges.cs
- METAHEADER.cs