Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / MS / Internal / interop / OlePropertyStructs.cs / 1 / OlePropertyStructs.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // These structures and constants are managed equivalents to COM structures // used to access OLE properties. // // History: // 01/26/2004: [....]: Initial implementation. // 08/26/2004: [....]: Moved to internal namespace MS.Internal.Interop. // 06/23/2005: [....]: Split out from IndexingFilter.cs, because these // structs are now needed by both the EncryptedPackageCoreProperties // class in WindowsBase and the IFilterCode in PresentationFramework. // //----------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using MS.Internal.WindowsBase; // for FriendAccessAllowedAttribute namespace MS.Internal.Interop { [StructLayout(LayoutKind.Sequential, Pack = 0)] internal struct STATPROPSTG { [MarshalAs(UnmanagedType.LPWStr)] string lpwstrName; UInt32 propid; VARTYPE vt; } [StructLayout(LayoutKind.Sequential, Pack = 0)] internal struct STATPROPSETSTG { Guid fmtid; Guid clsid; UInt32 grfFlags; System.Runtime.InteropServices.ComTypes.FILETIME mtime; System.Runtime.InteropServices.ComTypes.FILETIME ctime; System.Runtime.InteropServices.ComTypes.FILETIME atime; UInt32 dwOSVersion; } #region PROPVARIANT ////// Managed view of unmanaged PROPVARIANT type /// ////// PROPVARIANT can represent many different things. We are only interested in strings /// for this version but the full range of values is listed her for completeness. /// /// typedef unsigned short VARTYPE; /// typedef unsigned short WORD; /// typedef struct PROPVARIANT { /// VARTYPE vt; WORD wReserved1; WORD wReserved2; WORD wReserved3; /// union { /// CHAR cVal; /// UCHAR bVal; /// SHORT iVal; /// USHORT uiVal; /// LONG lVal; /// INT intVal; /// ULONG ulVal; /// UINT uintVal; /// LARGE_INTEGER hVal; /// ULARGE_INTEGER uhVal; /// FLOAT fltVal; DOUBLE dblVal; CY cyVal; DATE date; /// BSTR bstrVal; VARIANT_BOOL boolVal; SCODE scode; /// FILETIME filetime; LPSTR pszVal; LPWSTR pwszVal; /// CLSID* puuid; CLIPDATA* pclipdata; BLOB blob; /// IStream* pStream; IStorage* pStorage; IUnknown* punkVal; /// IDispatch* pdispVal; LPSAFEARRAY parray; CAC cac; /// CAUB caub; CAI cai; CAUI caui; CAL cal; CAUL caul; /// CAH cah; CAUH cauh; CAFLT caflt; CADBL cadbl; /// CACY cacy; CADATE cadate; CABSTR cabstr; /// CABOOL cabool; CASCODE cascode; CALPSTR calpstr; /// CALPWSTR calpwstr; CAFILETIME cafiletime; CACLSID cauuid; /// CACLIPDATA caclipdata; CAPROPVARIANT capropvar; /// CHAR* pcVal; UCHAR* pbVal; SHORT* piVal; USHORT* puiVal; /// LONG* plVal; ULONG* pulVal; INT* pintVal; UINT* puintVal; /// FLOAT* pfltVal; DOUBLE* pdblVal; VARIANT_BOOL* pboolVal; /// DECIMAL* pdecVal; SCODE* pscode; CY* pcyVal; /// PROPVARIANT* pvarVal; /// }; /// } PROPVARIANT; /// [StructLayout(LayoutKind.Sequential, Pack = 0)] [FriendAccessAllowed] internal struct PROPVARIANT { ////// Variant type /// internal VARTYPE vt; ////// unused /// internal ushort wReserved1; ////// unused /// internal ushort wReserved2; ////// unused /// internal ushort wReserved3; ////// union where the actual variant value lives /// internal PropVariantUnion union; } ////// enumeration for all legal types of a PROPVARIANT /// ///add definitions as needed [FriendAccessAllowed] internal enum VARTYPE : short { ////// BSTR /// VT_BSTR = 8, // BSTR allocated using SysAllocString ////// LPSTR /// VT_LPSTR = 30, ////// FILETIME /// VT_FILETIME = 64, } ////// Union portion of PROPVARIANT /// ////// All fields (or their placeholders) are declared even if /// they are not used. This is to make sure that the size of /// the union matches the size of the union in /// the actual unmanaged PROPVARIANT structure /// for all architectures (32-bit/64-bit). /// Points to note: /// - All pointer type fields are declared as IntPtr. /// - CAxxx type fields (like CAC, CAUB, etc.) are all of same /// structural layout, hence not all declared individually /// since they are not used. A placeholder CArray /// is used to represent all of them to account for the /// size of these types. CArray is defined later. /// - Rest of the fields are declared with corresponding /// managed equivalent types. /// [StructLayout(LayoutKind.Explicit)] [FriendAccessAllowed] internal struct PropVariantUnion { ////// CHAR /// [FieldOffset(0)] internal sbyte cVal; ////// UCHAR /// [FieldOffset(0)] internal byte bVal; ////// SHORT /// [FieldOffset(0)] internal short iVal; ////// USHORT /// [FieldOffset(0)] internal ushort uiVal; ////// LONG /// [FieldOffset(0)] internal int lVal; ////// ULONG /// [FieldOffset(0)] internal uint ulVal; ////// INT /// [FieldOffset(0)] internal int intVal; ////// UINT /// [FieldOffset(0)] internal uint uintVal; ////// LARGE_INTEGER /// [FieldOffset(0)] internal Int64 hVal; ////// ULARGE_INTEGER /// [FieldOffset(0)] internal UInt64 uhVal; ////// FLOAT /// [FieldOffset(0)] internal float fltVal; ////// DOUBLE /// [FieldOffset(0)] internal double dblVal; ////// VARIANT_BOOL /// [FieldOffset(0)] internal short boolVal; ////// SCODE /// [FieldOffset(0)] internal int scode; ////// CY /// [FieldOffset(0)] internal CY cyVal; ////// DATE /// [FieldOffset(0)] internal double date; ////// FILETIME /// [FieldOffset(0)] internal System.Runtime.InteropServices.ComTypes.FILETIME filetime; ////// CLSID* /// [FieldOffset(0)] internal IntPtr puuid; ////// CLIPDATA* /// [FieldOffset(0)] internal IntPtr pclipdata; ////// BSTR /// [FieldOffset(0)] internal IntPtr bstrVal; ////// BSTRBLOB /// [FieldOffset(0)] internal BSTRBLOB bstrblobVal; ////// BLOB /// [FieldOffset(0)] internal BLOB blob; ////// LPSTR /// [FieldOffset(0)] internal IntPtr pszVal; ////// LPWSTR /// [FieldOffset(0)] internal IntPtr pwszVal; ////// IUnknown* /// [FieldOffset(0)] internal IntPtr punkVal; ////// IDispatch* /// [FieldOffset(0)] internal IntPtr pdispVal; ////// IStream* /// [FieldOffset(0)] internal IntPtr pStream; ////// IStorage* /// [FieldOffset(0)] internal IntPtr pStorage; ////// LPVERSIONEDSTREAM /// [FieldOffset(0)] internal IntPtr pVersionedStream; ////// LPSAFEARRAY /// [FieldOffset(0)] internal IntPtr parray; ////// Placeholder for /// CAC, CAUB, CAI, CAUI, CAL, CAUL, CAH, CAUH; CAFLT, /// CADBL, CABOOL, CASCODE, CACY, CADATE, CAFILETIME, /// CACLSID, CACLIPDATA, CABSTR, CABSTRBLOB, /// CALPSTR, CALPWSTR, CAPROPVARIANT /// [FieldOffset(0)] internal CArray cArray; ////// CHAR* /// [FieldOffset(0)] internal IntPtr pcVal; ////// UCHAR* /// [FieldOffset(0)] internal IntPtr pbVal; ////// SHORT* /// [FieldOffset(0)] internal IntPtr piVal; ////// USHORT* /// [FieldOffset(0)] internal IntPtr puiVal; ////// LONG* /// [FieldOffset(0)] internal IntPtr plVal; ////// ULONG* /// [FieldOffset(0)] internal IntPtr pulVal; ////// INT* /// [FieldOffset(0)] internal IntPtr pintVal; ////// UINT* /// [FieldOffset(0)] internal IntPtr puintVal; ////// FLOAT* /// [FieldOffset(0)] internal IntPtr pfltVal; ////// DOUBLE* /// [FieldOffset(0)] internal IntPtr pdblVal; ////// VARIANT_BOOL* /// [FieldOffset(0)] internal IntPtr pboolVal; ////// DECIMAL* /// [FieldOffset(0)] internal IntPtr pdecVal; ////// SCODE* /// [FieldOffset(0)] internal IntPtr pscode; ////// CY* /// [FieldOffset(0)] internal IntPtr pcyVal; ////// DATE* /// [FieldOffset(0)] internal IntPtr pdate; ////// BSTR* /// [FieldOffset(0)] internal IntPtr pbstrVal; ////// IUnknown** /// [FieldOffset(0)] internal IntPtr ppunkVal; ////// IDispatch** /// [FieldOffset(0)] internal IntPtr ppdispVal; ////// LPSAFEARRAY* /// [FieldOffset(0)] internal IntPtr pparray; ////// PROPVARIANT* /// [FieldOffset(0)] internal IntPtr pvarVal; } #region Structs used by PropVariantUnion // // NOTE: Verifiability requires that the // fields of these value-types need to be public // since PropVariantUnion has explicit layout, // and has these value-types as its fields in a way that // overlaps with other PropVariantUnion fields // (same FieldOffset for multiple fields). // ////// CY, used in PropVariantUnion. /// [StructLayout(LayoutKind.Sequential, Pack = 0)] [FriendAccessAllowed] internal struct CY { public uint Lo; public int Hi; } ////// BSTRBLOB, used in PropVariantUnion. /// [StructLayout(LayoutKind.Sequential, Pack = 0)] [FriendAccessAllowed] internal struct BSTRBLOB { public uint cbSize; public IntPtr pData; } ////// BLOB, used in PropVariantUnion. /// [StructLayout(LayoutKind.Sequential, Pack = 0)] [FriendAccessAllowed] internal struct BLOB { public uint cbSize; public IntPtr pBlobData; } ////// CArray, used in PropVariantUnion. /// [StructLayout(LayoutKind.Sequential, Pack = 0)] [FriendAccessAllowed] internal struct CArray { public uint cElems; public IntPtr pElems; } #endregion Structs used by PropVariantUnion #endregion PROPVARIANT #region PROPSPEC ////// Selector for union /// [FriendAccessAllowed] internal enum PropSpecType : int { ////// type is a string /// Name = 0, ////// type is a property id /// Id = 1, } ////// Explicitly packed union /// [StructLayout(LayoutKind.Explicit)] [FriendAccessAllowed] internal struct PROPSPECunion { ////// first value of union - String /// [FieldOffset(0)] internal IntPtr name; // kind=0 ////// second value of union - ULong /// [FieldOffset(0)] internal uint propId; // kind=1 } ////// PROPSPEC - needed for IFilter.Init to specify which properties to extract /// ////// typedef struct tagPROPSPEC /// { /// ULONG ulKind; /// union { /// PROPID propid; // ulKind = 1 /// LPOLESTR lpwstr; // ulKind = 0 /// }; /// } PROPSPEC; /// [StructLayout(LayoutKind.Sequential, Pack = 0)] [FriendAccessAllowed] internal struct PROPSPEC { ////// Selector value /// internal uint propType; // ULONG in COM is UInt32 in .NET ////// Union struct /// internal PROPSPECunion union; } #endregion PROPSPEC #region FormatId ////// Format identifiers. /// [FriendAccessAllowed] internal static class FormatId { ////// Property sets. /// ////// Can't be declared readonly since they have to passed by ref /// to EncryptedPackageCoreProperties.GetOleProperty, because /// IPropertyStorage.ReadMultiple takes a "ref Guid" as its first argument. /// internal static Guid SummaryInformation = new Guid("{F29F85E0-4FF9-1068-AB91-08002B27B3D9}"); internal static Guid DocumentSummaryInformation = new Guid("{D5CDD502-2E9C-101B-9397-08002B2CF9AE}"); } #endregion FormatId #region PropertyId ////// Property identifiers. /// [FriendAccessAllowed] internal static class PropertyId { ////// Summary Information property identifiers. /// internal const uint Title = 0x00000002; internal const uint Subject = 0x00000003; internal const uint Creator = 0x00000004; internal const uint Keywords = 0x00000005; internal const uint Description = 0x00000006; internal const uint LastModifiedBy = 0x00000008; internal const uint Revision = 0x00000009; internal const uint LastPrinted = 0x0000000B; internal const uint DateCreated = 0x0000000C; internal const uint DateModified = 0x0000000D; ////// Document Summary Information property identifiers. /// internal const uint Category = 0x00000002; internal const uint Identifier = 0x00000012; internal const uint ContentType = 0x0000001A; internal const uint Language = 0x0000001B; internal const uint Version = 0x0000001C; internal const uint ContentStatus = 0x0000001D; } #endregion PropertyId } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
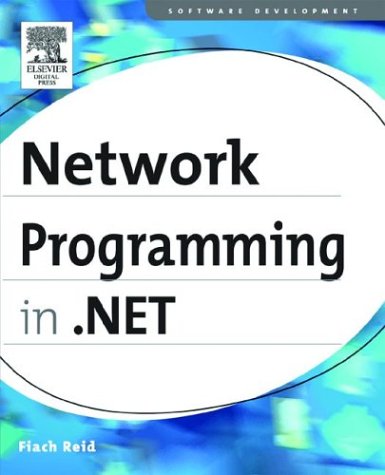
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MenuItemBinding.cs
- GeneralTransform3D.cs
- BooleanSwitch.cs
- FileDialog.cs
- LinearGradientBrush.cs
- KeyValueInternalCollection.cs
- Verify.cs
- MexHttpBindingElement.cs
- XamlHostingSection.cs
- EnlistmentTraceIdentifier.cs
- XmlSchemaCompilationSettings.cs
- XmlSchemaType.cs
- RotateTransform3D.cs
- ThicknessKeyFrameCollection.cs
- TextBoxRenderer.cs
- ControlAdapter.cs
- Invariant.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- HttpResponseWrapper.cs
- StaticExtensionConverter.cs
- ContractMapping.cs
- XDeferredAxisSource.cs
- AssemblySettingAttributes.cs
- Page.cs
- Registration.cs
- CommandManager.cs
- Content.cs
- WebMethodAttribute.cs
- streamingZipPartStream.cs
- RadioButtonAutomationPeer.cs
- StringValidator.cs
- Baml2006KeyRecord.cs
- AccessDataSource.cs
- BitArray.cs
- InputReportEventArgs.cs
- BindingsCollection.cs
- CellParaClient.cs
- ValidationEventArgs.cs
- WindowsListViewItemCheckBox.cs
- TemplatePartAttribute.cs
- EventRoute.cs
- PresentationSource.cs
- SwitchAttribute.cs
- DBNull.cs
- PackagingUtilities.cs
- DeviceContexts.cs
- NamespaceExpr.cs
- ErrorTableItemStyle.cs
- OracleBFile.cs
- ImageCodecInfoPrivate.cs
- RecordManager.cs
- SoapFault.cs
- ScriptControlDescriptor.cs
- DesignerObject.cs
- FileDocument.cs
- PerfProviderCollection.cs
- RichTextBoxContextMenu.cs
- ObjectResult.cs
- StorageComplexTypeMapping.cs
- ReachSerializerAsync.cs
- DataGridViewDataConnection.cs
- TrustSection.cs
- ReachUIElementCollectionSerializerAsync.cs
- MbpInfo.cs
- Cursors.cs
- log.cs
- DummyDataSource.cs
- KeysConverter.cs
- TextShapeableCharacters.cs
- SafeFileMapViewHandle.cs
- CodeThrowExceptionStatement.cs
- SqlWriter.cs
- ExtendedProtectionPolicyElement.cs
- ImageInfo.cs
- GPRECT.cs
- XmlDataLoader.cs
- SqlNodeAnnotations.cs
- GridViewCellAutomationPeer.cs
- LOSFormatter.cs
- ADConnectionHelper.cs
- DbTypeMap.cs
- basenumberconverter.cs
- CoreSwitches.cs
- Style.cs
- TempEnvironment.cs
- TemplatedWizardStep.cs
- EmptyQuery.cs
- FontUnit.cs
- ReflectionTypeLoadException.cs
- Image.cs
- LogReservationCollection.cs
- ParameterBuilder.cs
- SqlPersonalizationProvider.cs
- ListViewAutomationPeer.cs
- SectionInformation.cs
- MouseOverProperty.cs
- FormsAuthentication.cs
- XmlDataDocument.cs
- AnnotationComponentManager.cs
- QuaternionAnimation.cs