Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / ReachSerializerAsync.cs / 1 / ReachSerializerAsync.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachSerializerAsync.cs Abstract: This file contains the definition of a Base class that defines the common functionality required to serialie on type in a graph of types rooted by some object instance Author: [....] ([....]) 25-May-2005 Revision History: --*/ using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; namespace System.Windows.Xps.Serialization { ////// Base class defining common functionalities required to /// serialize one type. /// internal abstract class ReachSerializerAsync : ReachSerializer { #region Constructor ////// Constructor for class ReachSerializer /// /// /// The serializtion manager, the services of which are /// used later for the serialization process of the type. /// public ReachSerializerAsync( PackageSerializationManager manager ) { if(manager == null) { throw new ArgumentNullException("manager"); } _serializationManager = manager as XpsSerializationManagerAsync; if(_serializationManager == null) { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_NotXpsSerializationManagerAsync)); } _xmlWriter = null; } #endregion Constructor #region Public Methods public virtual void AsyncOperation( ReachSerializerContext context ) { if(context == null) { } switch (context.Action) { case SerializerAction.endSerializeObject: { EndSerializeObject(context.ObjectContext); break; } case SerializerAction.serializeNextProperty: { SerializeNextProperty(context.ObjectContext); break; } } } ////// The main method that is called to serialize the object of /// that given type. /// /// /// Instance of object to be serialized. /// public override void SerializeObject( Object serializedObject ) { BeginSerializeObject(serializedObject); } #endregion Public Methods #region Internal Methods ////// The main method that is called to serialize the object of /// that given type and that is usually called from within the /// serialization manager when a node in the graph of objects is /// at a turn where it should be serialized. /// /// /// The context of the property being serialized at this time and /// it points internally to the object encapsulated by that node. /// internal override void SerializeObject( SerializablePropertyContext serializedProperty ) { BeginSerializeObject(serializedProperty); } ////// Critical - Access the SerializationManager GraphContextStack which is a /// ContextStack which is link critical /// /// TreatAsSafe - The context stack is used local to the function. /// All access to the GraphContextStack is marked security critical /// [SecurityCritical, SecurityTreatAsSafe] internal virtual void BeginSerializeObject( SerializablePropertyContext serializedProperty ) { if(serializedProperty == null) { throw new ArgumentNullException("serializedProperty"); } if(SerializationManager == null) { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_MustHaveSerializationManager)); } // // At this stage discover the graph of properties of the object that // need to be serialized // SerializableObjectContext serializableObjectContext = DiscoverObjectData(serializedProperty.Value, serializedProperty); if(serializableObjectContext!=null) { // // Push the object at hand on the context stack // SerializationManager.GraphContextStack.Push(serializableObjectContext); ReachSerializerContext context = new ReachSerializerContext(this, serializableObjectContext, SerializerAction.endSerializeObject); _serializationManager.OperationStack.Push(context); // // At this stage we should start streaming the markup representing the // object graph to the corresponding destination // PersistObjectData(serializableObjectContext); } } ////// /// ////// Critical - Access the SerializationManager GraphContextStack which is a /// ContextStack which is link critical /// /// TreatAsSafe - The context stack is used local to the function. /// All access to the GraphContextStack is marked security critical /// [SecurityCritical, SecurityTreatAsSafe] internal virtual void BeginSerializeObject( Object serializedObject ) { if(serializedObject == null) { throw new ArgumentNullException("serializedObject"); } if(SerializationManager == null) { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_MustHaveSerializationManager)); } // // At this stage discover the graph of properties of the object that // need to be serialized // SerializableObjectContext serializableObjectContext = DiscoverObjectData(serializedObject, null); if(serializableObjectContext!=null) { // // Push the object at hand on the context stack // SerializationManager.GraphContextStack.Push(serializableObjectContext); ReachSerializerContext context = new ReachSerializerContext(this, serializableObjectContext, SerializerAction.endSerializeObject); _serializationManager.OperationStack.Push(context); // // At this stage we should start streaming the markup representing the // object graph to the corresponding destination // PersistObjectData(serializableObjectContext); } } ////// Critical - Access the SerializationManager GraphContextStack which is a /// ContextStack which is link critical /// /// TreatAsSafe - The context stack is used local to the function. /// All access to the GraphContextStack is marked security critical /// [SecurityCritical, SecurityTreatAsSafe] internal virtual void EndSerializeObject( SerializableObjectContext serializableObjectContext ) { // // Pop the object from the context stack // SerializationManager.GraphContextStack.Pop(); // // Recycle the used SerializableObjectContext // SerializableObjectContext.RecycleContext(serializableObjectContext); } /*////// The method is called once the object data is discovered at that /// point of the serialization process. /// /// /// The context of the object to be serialized at this time. /// internal abstract void PersistObjectData( SerializableObjectContext serializableObjectContext );*/ ////// /// internal virtual void EndPersistObjectData( ) { // // Do nothing in the base class // } ////// Serialize the properties within the object /// context into METRO /// ////// Method follows these steps /// 1. Serializes the instance as string content /// if is not meant to be a complex value. Else ... /// 2. Serialize Properties as attributes /// 3. Serialize Complex Properties as separate parts /// through calling separate serializers /// Also this is the virtual to override custom attributes or /// contents need to be serialized /// /// /// The context of the object to be serialized at this time. /// internal override void SerializeObjectCore( SerializableObjectContext serializableObjectContext ) { if (serializableObjectContext == null) { throw new ArgumentNullException("serializableObjectContext"); } if (!serializableObjectContext.IsReadOnlyValue && serializableObjectContext.IsComplexValue) { SerializeProperties(serializableObjectContext); } } ////// This method is the one that writes out the attribute within /// the xml stream when serializing simple properites. /// /// /// The property that is to be serialized as an attribute at this time. /// internal override void WriteSerializedAttribute( SerializablePropertyContext serializablePropertyContext ) { if(serializablePropertyContext == null) { throw new ArgumentNullException("serializablePropertyContext"); } } #endregion Internal Methods #region Private Methods ////// This method is the one that parses down the object at hand /// to discover all the properties that are expected to be serialized /// at that object level. /// the xml stream when serializing simple properties. /// /// /// The instance of the object being serialized. /// /// /// The instance of property on the parent object from which this /// object stemmed. This could be null if this is the node object /// or the object has no parent. /// ////// Critical - Access the SerializationManager GraphContextStack which is a /// ContextStack which is link critical /// /// TreatAsSafe - The context stack is used local to the function. /// All access to the GraphContextStack is marked security critical /// [SecurityCritical, SecurityTreatAsSafe] private SerializableObjectContext DiscoverObjectData( Object serializedObject, SerializablePropertyContext serializedProperty ) { // // Trying to figure out the parent of this node, which is at this stage // the same node previously pushed on the stack or in other words it is // the node that is currently on the top of the stack // SerializableObjectContext serializableObjectParentContext = (SerializableObjectContext)SerializationManager. GraphContextStack[typeof(SerializableObjectContext)]; // // Create the context for the current object // SerializableObjectContext serializableObjectContext = SerializableObjectContext.CreateContext(SerializationManager, serializedObject, serializableObjectParentContext, serializedProperty); // // Set the root object to be serialized at the level of the SerializationManager // if(SerializationManager.RootSerializableObjectContext == null) { SerializationManager.RootSerializableObjectContext = serializableObjectContext; } return serializableObjectContext; } ////// Trigger all properties serialization /// private void SerializeProperties( SerializableObjectContext serializableObjectContext ) { if (serializableObjectContext == null) { throw new ArgumentNullException("serializableObjectContext"); } SerializablePropertyCollection propertyCollection = serializableObjectContext.PropertiesCollection; if(propertyCollection!=null) { //for(propertyCollection.Reset(); // propertyCollection.MoveNext();) //{ // SerializablePropertyContext serializablePropertyContext = // (SerializablePropertyContext)propertyCollection.Current; // // if(serializablePropertyContext!=null) // { // SerializeProperty(serializablePropertyContext); // } //} propertyCollection.Reset(); ReachSerializerContext context = new ReachSerializerContext(this, serializableObjectContext, SerializerAction.serializeNextProperty); _serializationManager.OperationStack.Push(context); } } private void SerializeNextProperty( SerializableObjectContext serializableObjectContext ) { SerializablePropertyCollection propertyCollection = serializableObjectContext.PropertiesCollection; if(propertyCollection.MoveNext()) { SerializablePropertyContext serializablePropertyContext = (SerializablePropertyContext)propertyCollection.Current; if(serializablePropertyContext!=null) { ReachSerializerContext context = new ReachSerializerContext(this, serializableObjectContext, SerializerAction.serializeNextProperty); _serializationManager.OperationStack.Push(context); SerializeProperty(serializablePropertyContext); } } } ////// Trigger serializing one property at a time. /// private void SerializeProperty( SerializablePropertyContext serializablePropertyContext ) { if(serializablePropertyContext == null) { throw new ArgumentNullException("serializablePropertyContext"); } if(!serializablePropertyContext.IsComplex) { // // Non-Complex Properties are serialized as attributes // WriteSerializedAttribute(serializablePropertyContext); } else { // // Complex properties could be treated in different ways // based on their type. Examples of that are: // // // ReachSerializer serializer = SerializationManager.GetSerializer(serializablePropertyContext.Value); // If there is no serializer for this type, we won't serialize this property if(serializer!=null) { serializer.SerializeObject(serializablePropertyContext); } } } #endregion Private Methods #region Public Properties ////// Query / Set Xml Writer for the equivelan part /// public override XmlWriter XmlWriter { get { return _xmlWriter; } set { _xmlWriter = value; } } ////// Query the SerializationManager used by this serializer. /// public override PackageSerializationManager SerializationManager { get { return _serializationManager; } } #endregion Public Properties #region Private Data members private XpsSerializationManagerAsync _serializationManager; private XmlWriter _xmlWriter; #endregion Private Data members }; internal enum SerializerAction { endSerializeObject = 1, endPersistObjectData = 2, serializeNextProperty = 3, serializeNextDocumentReference = 4, serializeDocument = 5, serializeNextPageContent = 6, serializePage = 7, endSerializeReachFixedPage = 8, serializeNextUIElement = 9, serializeNextTreeNode = 10, serializeNextDocumentPage = 11, endSerializeDocumentPage = 12 }; internal class ReachSerializerContext { public ReachSerializerContext( ReachSerializerAsync serializer, SerializerAction action ) { this._action = action; this._serializer = serializer; this._objectContext = null; } public ReachSerializerContext( ReachSerializerAsync serializer, SerializableObjectContext objectContext, SerializerAction action ) { this._action = action; this._serializer = serializer; this._objectContext = objectContext; } public virtual SerializerAction Action { get { return _action; } } public virtual ReachSerializerAsync Serializer { get { return _serializer; } } public virtual SerializableObjectContext ObjectContext { get { return _objectContext; } } private SerializerAction _action; private ReachSerializerAsync _serializer; private SerializableObjectContext _objectContext; }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
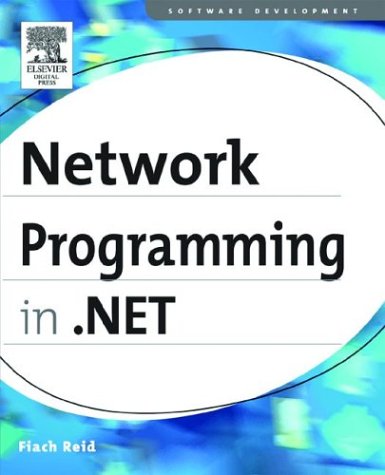
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlDataProvider.cs
- NameScopePropertyAttribute.cs
- Bezier.cs
- MarkupExtensionReturnTypeAttribute.cs
- sqlnorm.cs
- RSAPKCS1SignatureDeformatter.cs
- DataGridViewRowStateChangedEventArgs.cs
- SqlParameterCollection.cs
- CTreeGenerator.cs
- CommandHelpers.cs
- ReachSerializerAsync.cs
- AsyncResult.cs
- ProxyWebPart.cs
- SafePointer.cs
- HitTestWithGeometryDrawingContextWalker.cs
- TreeNodeCollection.cs
- ListenerConstants.cs
- IntSecurity.cs
- XmlNodeChangedEventArgs.cs
- WebServiceErrorEvent.cs
- TemplateNameScope.cs
- XhtmlBasicCalendarAdapter.cs
- RelOps.cs
- ContextMenuAutomationPeer.cs
- StateMachine.cs
- DescendantBaseQuery.cs
- CodeDomLocalizationProvider.cs
- ScriptResourceInfo.cs
- InvalidProgramException.cs
- PersonalizablePropertyEntry.cs
- TableCell.cs
- GridViewColumnCollectionChangedEventArgs.cs
- HwndTarget.cs
- ProcessInputEventArgs.cs
- DataGridViewIntLinkedList.cs
- WinCategoryAttribute.cs
- CommandConverter.cs
- HtmlInputCheckBox.cs
- Assert.cs
- BitmapVisualManager.cs
- FileSystemEventArgs.cs
- BindingNavigator.cs
- __Filters.cs
- SQLDateTimeStorage.cs
- IArgumentProvider.cs
- XmlSchemaAnnotation.cs
- SafeNativeMethods.cs
- TableNameAttribute.cs
- DiscreteKeyFrames.cs
- Hex.cs
- ScriptingSectionGroup.cs
- EventHandlerList.cs
- ServiceMetadataPublishingElement.cs
- IsolatedStorageFileStream.cs
- ToolStripPanel.cs
- WindowsListView.cs
- CollectionBuilder.cs
- CharAnimationBase.cs
- ObservableDictionary.cs
- TextDecorationCollectionConverter.cs
- RoutedCommand.cs
- FormViewPageEventArgs.cs
- EnvironmentPermission.cs
- TraceRecord.cs
- RuntimeCompatibilityAttribute.cs
- SplitterCancelEvent.cs
- ActivitiesCollection.cs
- PointAnimationUsingPath.cs
- BypassElement.cs
- CodeObjectCreateExpression.cs
- WorkflowInlining.cs
- ReliableSessionElement.cs
- JsonReader.cs
- MessageBox.cs
- EntityProviderServices.cs
- OleDbParameter.cs
- ItemChangedEventArgs.cs
- Msmq4PoisonHandler.cs
- DefaultShape.cs
- EasingFunctionBase.cs
- Debug.cs
- EntityContainerEntitySetDefiningQuery.cs
- TrailingSpaceComparer.cs
- PersistenceTypeAttribute.cs
- DBConnection.cs
- SQLInt64.cs
- ManipulationDelta.cs
- SortFieldComparer.cs
- FastPropertyAccessor.cs
- HtmlDocument.cs
- SimpleHandlerBuildProvider.cs
- SystemColorTracker.cs
- SymbolDocumentInfo.cs
- AggregationMinMaxHelpers.cs
- CodeDOMProvider.cs
- CompiledQueryCacheEntry.cs
- HtmlTableRow.cs
- WindowsPrincipal.cs
- CompilerErrorCollection.cs
- CodeNamespaceImportCollection.cs