Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media3D / GeneralTransform2DTo3D.cs / 1 / GeneralTransform2DTo3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Declaration of the GeneralTransform2DTo3D class. // //--------------------------------------------------------------------------- using System.Diagnostics; using System.Windows.Media; using System.Windows.Media.Animation; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// TransformTo3D class provides services to transform points from 2D in to 3D /// public class GeneralTransform2DTo3D : Freezable { internal GeneralTransform2DTo3D() { } internal GeneralTransform2DTo3D(GeneralTransform transform2D, Viewport2DVisual3D containingVisual3D, GeneralTransform3D transform3D) { Visual child = containingVisual3D.Visual; Debug.Assert(child != null, "Going from 2D to 3D containingVisual3D.Visual should not be null"); _transform3D = (GeneralTransform3D)transform3D.GetAsFrozen(); // we also need to go one more level up to handle a transform being placed // on the Viewport2DVisual3D's child GeneralTransformGroup transformGroup = new GeneralTransformGroup(); transformGroup.Children.Add((GeneralTransform)transform2D.GetAsFrozen()); transformGroup.Children.Add((GeneralTransform)child.TransformToOuterSpace().GetAsFrozen()); transformGroup.Freeze(); _transform2D = transformGroup; _positions = containingVisual3D.InternalPositionsCache; _textureCoords = containingVisual3D.InternalTextureCoordinatesCache; _triIndices = containingVisual3D.InternalTriangleIndicesCache; _childBounds = child.CalculateSubgraphRenderBoundsOuterSpace(); } ////// Transform a point /// /// Input point /// Output point ///True if the point was transformed successfuly, false otherwise public bool TryTransform(Point inPoint, out Point3D result) { Point final2DPoint; // assign this now so that we can return false if needed result = new Point3D(); if (!_transform2D.TryTransform(inPoint, out final2DPoint)) { return false; } Point texCoord = Viewport2DVisual3D.VisualCoordsToTextureCoords(final2DPoint, _childBounds); // need to walk the texture coordinates on the Viewport2DVisual3D // and look for where this point intersects one of them Point3D coordPoint; if (!Viewport2DVisual3D.Get3DPointFor2DCoordinate(texCoord, out coordPoint, _positions, _textureCoords, _triIndices)) { return false; } if (!_transform3D.TryTransform(coordPoint, out result)) { return false; } return true; } ////// Transform a point from 2D in to 3D /// /// If the transformation does not succeed, this will throw an InvalidOperationException. /// If you don't want to try/catch, call TryTransform instead and check the boolean it /// returns. /// /// /// Input point ///The transformed point public Point3D Transform(Point point) { Point3D transformedPoint; if (!TryTransform(point, out transformedPoint)) { throw new InvalidOperationException(SR.Get(SRID.GeneralTransform_TransformFailed, null)); } return transformedPoint; } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new GeneralTransform2DTo3D(); } ////// Implementation of /// protected override void CloneCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCore . ////// Implementation of /// protected override void CloneCurrentValueCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCurrentValueCore . ////// Implementation of /// protected override void GetAsFrozenCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetAsFrozenCore . ////// Implementation of /// protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetCurrentValueAsFrozenCore . ////// Clones values that do not have corresponding DPs /// /// private void CopyCommon(GeneralTransform2DTo3D transform) { _transform2D = transform._transform2D; _transform3D = transform._transform3D; _positions = transform._positions; _textureCoords = transform._textureCoords; _triIndices = transform._triIndices; _childBounds = transform._childBounds; } private GeneralTransform _transform2D; private GeneralTransform3D _transform3D; private Point3DCollection _positions; private PointCollection _textureCoords; private Int32Collection _triIndices; private Rect _childBounds; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Declaration of the GeneralTransform2DTo3D class. // //--------------------------------------------------------------------------- using System.Diagnostics; using System.Windows.Media; using System.Windows.Media.Animation; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// TransformTo3D class provides services to transform points from 2D in to 3D /// public class GeneralTransform2DTo3D : Freezable { internal GeneralTransform2DTo3D() { } internal GeneralTransform2DTo3D(GeneralTransform transform2D, Viewport2DVisual3D containingVisual3D, GeneralTransform3D transform3D) { Visual child = containingVisual3D.Visual; Debug.Assert(child != null, "Going from 2D to 3D containingVisual3D.Visual should not be null"); _transform3D = (GeneralTransform3D)transform3D.GetAsFrozen(); // we also need to go one more level up to handle a transform being placed // on the Viewport2DVisual3D's child GeneralTransformGroup transformGroup = new GeneralTransformGroup(); transformGroup.Children.Add((GeneralTransform)transform2D.GetAsFrozen()); transformGroup.Children.Add((GeneralTransform)child.TransformToOuterSpace().GetAsFrozen()); transformGroup.Freeze(); _transform2D = transformGroup; _positions = containingVisual3D.InternalPositionsCache; _textureCoords = containingVisual3D.InternalTextureCoordinatesCache; _triIndices = containingVisual3D.InternalTriangleIndicesCache; _childBounds = child.CalculateSubgraphRenderBoundsOuterSpace(); } ////// Transform a point /// /// Input point /// Output point ///True if the point was transformed successfuly, false otherwise public bool TryTransform(Point inPoint, out Point3D result) { Point final2DPoint; // assign this now so that we can return false if needed result = new Point3D(); if (!_transform2D.TryTransform(inPoint, out final2DPoint)) { return false; } Point texCoord = Viewport2DVisual3D.VisualCoordsToTextureCoords(final2DPoint, _childBounds); // need to walk the texture coordinates on the Viewport2DVisual3D // and look for where this point intersects one of them Point3D coordPoint; if (!Viewport2DVisual3D.Get3DPointFor2DCoordinate(texCoord, out coordPoint, _positions, _textureCoords, _triIndices)) { return false; } if (!_transform3D.TryTransform(coordPoint, out result)) { return false; } return true; } ////// Transform a point from 2D in to 3D /// /// If the transformation does not succeed, this will throw an InvalidOperationException. /// If you don't want to try/catch, call TryTransform instead and check the boolean it /// returns. /// /// /// Input point ///The transformed point public Point3D Transform(Point point) { Point3D transformedPoint; if (!TryTransform(point, out transformedPoint)) { throw new InvalidOperationException(SR.Get(SRID.GeneralTransform_TransformFailed, null)); } return transformedPoint; } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new GeneralTransform2DTo3D(); } ////// Implementation of /// protected override void CloneCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCore . ////// Implementation of /// protected override void CloneCurrentValueCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(transform); } ///Freezable.CloneCurrentValueCore . ////// Implementation of /// protected override void GetAsFrozenCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.GetAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetAsFrozenCore . ////// Implementation of /// protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { GeneralTransform2DTo3D transform = (GeneralTransform2DTo3D)sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); CopyCommon(transform); } ///Freezable.GetCurrentValueAsFrozenCore . ////// Clones values that do not have corresponding DPs /// /// private void CopyCommon(GeneralTransform2DTo3D transform) { _transform2D = transform._transform2D; _transform3D = transform._transform3D; _positions = transform._positions; _textureCoords = transform._textureCoords; _triIndices = transform._triIndices; _childBounds = transform._childBounds; } private GeneralTransform _transform2D; private GeneralTransform3D _transform3D; private Point3DCollection _positions; private PointCollection _textureCoords; private Int32Collection _triIndices; private Rect _childBounds; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
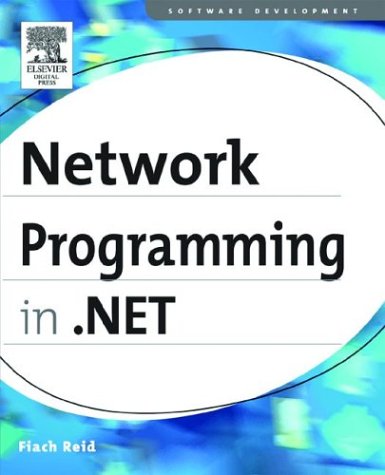
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UniqueIdentifierService.cs
- ViewLoader.cs
- TableHeaderCell.cs
- MemberCollection.cs
- WebPartHeaderCloseVerb.cs
- OrderToken.cs
- SimpleRecyclingCache.cs
- LowerCaseStringConverter.cs
- XPathNode.cs
- CellQuery.cs
- InternalTransaction.cs
- FrameSecurityDescriptor.cs
- UIElement3D.cs
- CompositeCollectionView.cs
- EdmComplexTypeAttribute.cs
- SoapInteropTypes.cs
- WinEventTracker.cs
- ModelPerspective.cs
- PolyBezierSegment.cs
- DelegateSerializationHolder.cs
- PseudoWebRequest.cs
- AudioFileOut.cs
- __Filters.cs
- SafeRightsManagementEnvironmentHandle.cs
- WpfPayload.cs
- RawStylusActions.cs
- MimeMapping.cs
- AssemblyUtil.cs
- XhtmlBasicValidationSummaryAdapter.cs
- OutputScope.cs
- WebPartDisplayModeEventArgs.cs
- ProtocolsConfigurationHandler.cs
- TypeInfo.cs
- Crc32.cs
- HandlerMappingMemo.cs
- DomainUpDown.cs
- StrokeFIndices.cs
- SoapAttributeOverrides.cs
- InvokeSchedule.cs
- ToolStripLocationCancelEventArgs.cs
- EditorBrowsableAttribute.cs
- DataMemberFieldEditor.cs
- ELinqQueryState.cs
- PersonalizationEntry.cs
- Int32.cs
- formatter.cs
- PeerNameRecord.cs
- SessionStateModule.cs
- TextProperties.cs
- EdmComplexTypeAttribute.cs
- KoreanCalendar.cs
- XmlSerializationWriter.cs
- ItemChangedEventArgs.cs
- Int32RectConverter.cs
- BufferedReadStream.cs
- QilSortKey.cs
- ClientCultureInfo.cs
- WorkerRequest.cs
- LinkArea.cs
- MenuItem.cs
- SignerInfo.cs
- BlobPersonalizationState.cs
- RijndaelCryptoServiceProvider.cs
- AssemblySettingAttributes.cs
- InvalidFilterCriteriaException.cs
- XmlMapping.cs
- DocumentGridContextMenu.cs
- TypeSystem.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- OutgoingWebRequestContext.cs
- _SSPISessionCache.cs
- TextServicesManager.cs
- ExceptionHandlerDesigner.cs
- GregorianCalendar.cs
- SolidColorBrush.cs
- FormViewDeletedEventArgs.cs
- AssertSection.cs
- HostVisual.cs
- FieldNameLookup.cs
- AlphabeticalEnumConverter.cs
- COSERVERINFO.cs
- DebugView.cs
- AppDomain.cs
- RectAnimationBase.cs
- AvTraceDetails.cs
- HandlerFactoryWrapper.cs
- ScriptBehaviorDescriptor.cs
- TraceHandler.cs
- HitTestParameters.cs
- NumericUpDown.cs
- DescendentsWalker.cs
- PreservationFileReader.cs
- RpcAsyncResult.cs
- SpellerHighlightLayer.cs
- BitmapFrame.cs
- BindingSource.cs
- DoubleUtil.cs
- SchemaImporter.cs
- SectionXmlInfo.cs
- SimpleMailWebEventProvider.cs