Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Core / OrderToken.cs / 1305376 / OrderToken.cs
namespace System.Activities.Presentation { using System; using System.Collections.Generic; using System.Text; using System.Activities.Presentation; ////// OrderToken is a generic class used to identify the sort /// order of hierarchical items. OrderTokens can be used /// to define priority that is based on some predefined defaults or /// based on other OrderTokens. /// abstract class OrderToken : IComparable{ private readonly OrderToken _reference; private readonly OrderTokenPrecedence _precedence; private readonly OrderTokenConflictResolution _conflictResolution; private readonly int _depth; private readonly int _index; private int _nextChildIndex; /// /// Creates a new OrderToken instance based on the specified /// referenced OrderToken, precedence, and conflict resolution /// semantics. /// /// Precedence of this token based on the /// referenced token. /// Referenced token. May be null for the /// root token case (token that's not dependent on anything else). /// Conflict resolution semantics. /// Winning ConflictResultion semantic should only be used /// on predefined, default OrderToken instances to ensure /// their correct placement in more complex chain of order /// dependencies. protected OrderToken( OrderTokenPrecedence precedence, OrderToken reference, OrderTokenConflictResolution conflictResolution) { if (!EnumValidator.IsValid(precedence)) throw FxTrace.Exception.AsError(new ArgumentOutOfRangeException("precedence")); if (!EnumValidator.IsValid(conflictResolution)) throw FxTrace.Exception.AsError(new ArgumentOutOfRangeException("conflictResolution")); _reference = reference; _precedence = precedence; _conflictResolution = conflictResolution; _depth = reference == null ? 0 : reference._depth + 1; _index = reference == null ? -1 : reference._nextChildIndex++; } ////// Compares this order token with the specified order token. /// The comparsion for OrderTokens that don't belong to the same /// chain of OrderTokens will be resolved non-deterministically. /// /// The token to compare this token to. ///0 when the tokens have an equal order priority, /// -1 if this order comes before the specified order, /// 1 otherwise. ///When other is null public virtual int CompareTo(OrderToken other) { if (other == null) throw FxTrace.Exception.ArgumentNull("other"); if (other == this) return 0; OrderToken thisOrder = this; // Find a common parent while (thisOrder._reference != other._reference) { if (thisOrder._depth == other._depth) { thisOrder = thisOrder._reference; other = other._reference; } else{ if (thisOrder._depth > other._depth) { if (thisOrder._reference == other) return thisOrder._precedence == OrderTokenPrecedence.After ? 1 : -1; thisOrder = thisOrder._reference; } else { if (other._reference == thisOrder) return other._precedence == OrderTokenPrecedence.After ? -1 : 1; other = other._reference; } } } // One order "before", one order "after"? Easy, return the // "before" order. if (thisOrder._precedence != other._precedence) return thisOrder._precedence == OrderTokenPrecedence.Before ? -1 : 1; // Both orders "before" the parent? Roots win, otherwise call ResolveConflict(). if (thisOrder._precedence == OrderTokenPrecedence.Before) { if (thisOrder._conflictResolution == OrderTokenConflictResolution.Win) return -1; else if (other._conflictResolution == OrderTokenConflictResolution.Win) return 1; return ResolveConflict(thisOrder, other); } // Both orders "after" the parent? Roots win, otherwise call ResolveConflict(). if (thisOrder._conflictResolution == OrderTokenConflictResolution.Win) return 1; else if (other._conflictResolution == OrderTokenConflictResolution.Win) return -1; return ResolveConflict(thisOrder, other); } ////// This method is called by CompareTo()'s default implementation when two OrderTokens /// appear to be equivalent. The base functionality of this method uses the instantiation /// order of the two tokens as a tie-breaker. Override this method to /// implement custom algorithms. Note that if this method ever returns 0 /// (indicating that the two tokens are equivalent) and if these tokens /// belong to a list that gets sorted multiple times, the relative order in /// which they appear in the list will not be guaranteed. This side-effect /// may or may not be a problem depending on the application. /// /// Left OrderToken /// Right OrderToken ///0, if the two are equal, -1, if left comes before right, /// 1 otherwise. protected virtual int ResolveConflict(OrderToken left, OrderToken right) { return left._index.CompareTo(right._index); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
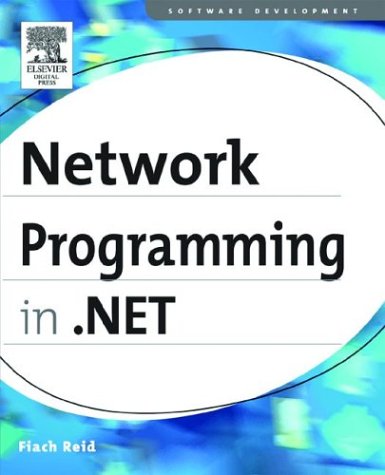
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObfuscateAssemblyAttribute.cs
- Accessible.cs
- State.cs
- FtpWebRequest.cs
- MobileResource.cs
- TranslateTransform3D.cs
- TransactionChannelListener.cs
- ComponentResourceKey.cs
- WebPartVerbCollection.cs
- ImageDrawing.cs
- Pen.cs
- TaiwanCalendar.cs
- LazyTextWriterCreator.cs
- PrivilegeNotHeldException.cs
- ItemsChangedEventArgs.cs
- FlatButtonAppearance.cs
- DataGridViewRowEventArgs.cs
- XNodeSchemaApplier.cs
- DBBindings.cs
- cookiecollection.cs
- BroadcastEventHelper.cs
- StreamGeometry.cs
- RestHandler.cs
- HotSpot.cs
- Event.cs
- SqlCaseSimplifier.cs
- Events.cs
- Menu.cs
- WorkflowApplication.cs
- CodeEntryPointMethod.cs
- HostingEnvironmentException.cs
- PeerObject.cs
- ObfuscateAssemblyAttribute.cs
- IgnoreFileBuildProvider.cs
- BamlRecordReader.cs
- ContractUtils.cs
- HybridDictionary.cs
- Brush.cs
- Polyline.cs
- EnumMemberAttribute.cs
- XmlStreamStore.cs
- SystemColors.cs
- InputMethodStateTypeInfo.cs
- Binding.cs
- WebPartChrome.cs
- UdpChannelFactory.cs
- Number.cs
- DesignTimeVisibleAttribute.cs
- BamlTreeMap.cs
- InvalidComObjectException.cs
- HexParser.cs
- VisualStyleRenderer.cs
- ThreadExceptionEvent.cs
- SimpleLine.cs
- HeaderUtility.cs
- DecimalConverter.cs
- XmlNavigatorStack.cs
- WebServiceTypeData.cs
- TextEditorCharacters.cs
- ReadOnlyDataSourceView.cs
- ModulesEntry.cs
- SQLSingleStorage.cs
- DataViewSetting.cs
- SizeConverter.cs
- AsyncInvokeOperation.cs
- BitmapSourceSafeMILHandle.cs
- SecurityContext.cs
- SortedList.cs
- PerfService.cs
- DragEventArgs.cs
- MatrixStack.cs
- SByteConverter.cs
- Rotation3DAnimationUsingKeyFrames.cs
- XPathChildIterator.cs
- counter.cs
- DiscoveryDocument.cs
- PrePostDescendentsWalker.cs
- InstanceDataCollection.cs
- LassoSelectionBehavior.cs
- DispatcherHookEventArgs.cs
- FontStretchConverter.cs
- LightweightCodeGenerator.cs
- RequestUriProcessor.cs
- DataGridTable.cs
- SimpleParser.cs
- SizeKeyFrameCollection.cs
- RuntimeWrappedException.cs
- DisposableCollectionWrapper.cs
- XmlNamespaceDeclarationsAttribute.cs
- EntityCommandDefinition.cs
- Configuration.cs
- RegisteredHiddenField.cs
- DataGridViewCellConverter.cs
- TextRangeSerialization.cs
- SendMessageChannelCache.cs
- ActivityStateQuery.cs
- Point3DConverter.cs
- DataGridViewCellFormattingEventArgs.cs
- PartManifestEntry.cs
- TextBlock.cs