Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Objects / ELinq / TypeSystem.cs / 2 / TypeSystem.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Reflection; using System.Data.Entity; namespace System.Data.Objects.ELinq { ////// Static utility class. Replica of query\DLinq\TypeSystem.cs /// internal static class TypeSystem { private static readonly MethodInfo s_getDefaultMethod = typeof(TypeSystem).GetMethod( "GetDefault", BindingFlags.Static | BindingFlags.NonPublic); private static T GetDefault() { return default(T); } internal static object GetDefaultValue(Type type) { // null is always the default for non value types and Nullable<> if (!type.IsValueType || (type.IsGenericType && typeof(Nullable<>) == type.GetGenericTypeDefinition())) { return null; } MethodInfo getDefaultMethod = s_getDefaultMethod.MakeGenericMethod(type); object defaultValue = getDefaultMethod.Invoke(null, new object[] { }); return defaultValue; } internal static bool IsSequenceType(Type seqType) { return FindIEnumerable(seqType) != null; } /// /// Resolves MemberInfo to a property or field. /// /// Member to test. /// Name of member. /// Type of member. ///Given member normalized as a property or field. internal static MemberInfo PropertyOrField(MemberInfo member, out string name, out Type type) { name = null; type = null; if (member.MemberType == MemberTypes.Field) { FieldInfo field = (FieldInfo)member; name = field.Name; type = field.FieldType; return field; } else if (member.MemberType == MemberTypes.Property) { PropertyInfo property = (PropertyInfo)member; if (0 != property.GetIndexParameters().Length) { // don't support indexed properties throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_PropertyIndexNotSupported); } name = property.Name; type = property.PropertyType; return property; } else if (member.MemberType == MemberTypes.Method) { // this may be a property accessor in disguise (if it's a RuntimeMethodHandle) MethodInfo method = (MethodInfo)member; if (method.IsSpecialName) // property accessor methods must set IsSpecialName { // try to find a property with the given getter foreach (PropertyInfo property in method.DeclaringType.GetProperties( BindingFlags.Static | BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic)) { if (property.CanRead && (property.GetGetMethod(true) == method)) { return PropertyOrField(property, out name, out type); } } } } throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_NotPropertyOrField(member.Name)); } private static Type FindIEnumerable(Type seqType) { // Ignores "terminal" primitive types in the EDM although they may implement IEnumerable<> if (seqType == null || seqType == typeof(string) || seqType == typeof(byte[])) return null; if (seqType.IsArray) return typeof(IEnumerable<>).MakeGenericType(seqType.GetElementType()); if (seqType.IsGenericType) { foreach (Type arg in seqType.GetGenericArguments()) { Type ienum = typeof(IEnumerable<>).MakeGenericType(arg); if (ienum.IsAssignableFrom(seqType)) { return ienum; } } } Type[] ifaces = seqType.GetInterfaces(); if (ifaces != null && ifaces.Length > 0) { foreach (Type iface in ifaces) { Type ienum = FindIEnumerable(iface); if (ienum != null) return ienum; } } if (seqType.BaseType != null && seqType.BaseType != typeof(object)) { return FindIEnumerable(seqType.BaseType); } return null; } internal static Type GetElementType(Type seqType) { Type ienum = FindIEnumerable(seqType); if (ienum == null) return seqType; return ienum.GetGenericArguments()[0]; } internal static bool IsNullableType(Type type) { return type != null && type.IsGenericType && type.GetGenericTypeDefinition() == typeof(Nullable<>); } internal static Type GetNonNullableType(Type type) { if (IsNullableType(type)) { return type.GetGenericArguments()[0]; } return type; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Reflection; using System.Data.Entity; namespace System.Data.Objects.ELinq { ////// Static utility class. Replica of query\DLinq\TypeSystem.cs /// internal static class TypeSystem { private static readonly MethodInfo s_getDefaultMethod = typeof(TypeSystem).GetMethod( "GetDefault", BindingFlags.Static | BindingFlags.NonPublic); private static T GetDefault() { return default(T); } internal static object GetDefaultValue(Type type) { // null is always the default for non value types and Nullable<> if (!type.IsValueType || (type.IsGenericType && typeof(Nullable<>) == type.GetGenericTypeDefinition())) { return null; } MethodInfo getDefaultMethod = s_getDefaultMethod.MakeGenericMethod(type); object defaultValue = getDefaultMethod.Invoke(null, new object[] { }); return defaultValue; } internal static bool IsSequenceType(Type seqType) { return FindIEnumerable(seqType) != null; } /// /// Resolves MemberInfo to a property or field. /// /// Member to test. /// Name of member. /// Type of member. ///Given member normalized as a property or field. internal static MemberInfo PropertyOrField(MemberInfo member, out string name, out Type type) { name = null; type = null; if (member.MemberType == MemberTypes.Field) { FieldInfo field = (FieldInfo)member; name = field.Name; type = field.FieldType; return field; } else if (member.MemberType == MemberTypes.Property) { PropertyInfo property = (PropertyInfo)member; if (0 != property.GetIndexParameters().Length) { // don't support indexed properties throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_PropertyIndexNotSupported); } name = property.Name; type = property.PropertyType; return property; } else if (member.MemberType == MemberTypes.Method) { // this may be a property accessor in disguise (if it's a RuntimeMethodHandle) MethodInfo method = (MethodInfo)member; if (method.IsSpecialName) // property accessor methods must set IsSpecialName { // try to find a property with the given getter foreach (PropertyInfo property in method.DeclaringType.GetProperties( BindingFlags.Static | BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic)) { if (property.CanRead && (property.GetGetMethod(true) == method)) { return PropertyOrField(property, out name, out type); } } } } throw EntityUtil.NotSupported(System.Data.Entity.Strings.ELinq_NotPropertyOrField(member.Name)); } private static Type FindIEnumerable(Type seqType) { // Ignores "terminal" primitive types in the EDM although they may implement IEnumerable<> if (seqType == null || seqType == typeof(string) || seqType == typeof(byte[])) return null; if (seqType.IsArray) return typeof(IEnumerable<>).MakeGenericType(seqType.GetElementType()); if (seqType.IsGenericType) { foreach (Type arg in seqType.GetGenericArguments()) { Type ienum = typeof(IEnumerable<>).MakeGenericType(arg); if (ienum.IsAssignableFrom(seqType)) { return ienum; } } } Type[] ifaces = seqType.GetInterfaces(); if (ifaces != null && ifaces.Length > 0) { foreach (Type iface in ifaces) { Type ienum = FindIEnumerable(iface); if (ienum != null) return ienum; } } if (seqType.BaseType != null && seqType.BaseType != typeof(object)) { return FindIEnumerable(seqType.BaseType); } return null; } internal static Type GetElementType(Type seqType) { Type ienum = FindIEnumerable(seqType); if (ienum == null) return seqType; return ienum.GetGenericArguments()[0]; } internal static bool IsNullableType(Type type) { return type != null && type.IsGenericType && type.GetGenericTypeDefinition() == typeof(Nullable<>); } internal static Type GetNonNullableType(Type type) { if (IsNullableType(type)) { return type.GetGenericArguments()[0]; } return type; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
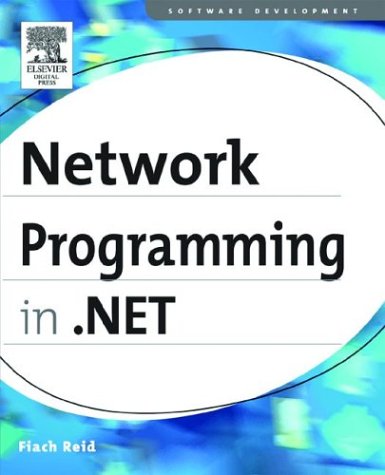
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IssuedSecurityTokenProvider.cs
- SingleObjectCollection.cs
- ConstraintStruct.cs
- XmlElementList.cs
- OutputCacheSettings.cs
- Line.cs
- WSSecurityOneDotZeroReceiveSecurityHeader.cs
- ToolStripSeparator.cs
- InheritanceContextChangedEventManager.cs
- EntityDataSourceWrapper.cs
- OdbcConnection.cs
- GroupLabel.cs
- HtmlButton.cs
- DataControlPagerLinkButton.cs
- DocumentPaginator.cs
- WsdlExporter.cs
- ErrorFormatterPage.cs
- DataControlFieldCollection.cs
- ClipboardData.cs
- RoutingChannelExtension.cs
- DataSourceControl.cs
- TraceXPathNavigator.cs
- GenericTextProperties.cs
- CaseInsensitiveHashCodeProvider.cs
- smtpconnection.cs
- SqlDataSourceEnumerator.cs
- TdsRecordBufferSetter.cs
- ErrorWebPart.cs
- AuthorizationContext.cs
- TransformerInfo.cs
- OdbcParameter.cs
- StoreItemCollection.Loader.cs
- CqlParser.cs
- DoWorkEventArgs.cs
- PathSegment.cs
- NetworkStream.cs
- MaskedTextBox.cs
- TableCell.cs
- TableCellAutomationPeer.cs
- ObjectSpanRewriter.cs
- WorkerRequest.cs
- EventLogPermissionEntryCollection.cs
- DBConcurrencyException.cs
- OleDbTransaction.cs
- XmlSchemaAll.cs
- TextBoxLine.cs
- EntityDataSource.cs
- MgmtConfigurationRecord.cs
- ClassHandlersStore.cs
- FileInfo.cs
- AnnotationComponentChooser.cs
- XslAst.cs
- AppDomainProtocolHandler.cs
- _HeaderInfoTable.cs
- HijriCalendar.cs
- TileBrush.cs
- RangeValuePattern.cs
- LayoutEvent.cs
- HtmlTableRow.cs
- BaseTemplatedMobileComponentEditor.cs
- DoubleUtil.cs
- MultiSelector.cs
- Menu.cs
- AutomationPatternInfo.cs
- NativeObjectSecurity.cs
- ComponentSerializationService.cs
- MsmqTransportElement.cs
- _ScatterGatherBuffers.cs
- ParameterDataSourceExpression.cs
- LineSegment.cs
- SettingsSection.cs
- CodeIdentifiers.cs
- SapiAttributeParser.cs
- BitmapEffectGroup.cs
- WebPartEditorCancelVerb.cs
- ModelItem.cs
- GenerateTemporaryTargetAssembly.cs
- DataTableReaderListener.cs
- ConfigXmlElement.cs
- CompilerCollection.cs
- WebPartCollection.cs
- AuthenticationSchemesHelper.cs
- Highlights.cs
- XmlSchemaSubstitutionGroup.cs
- ConstantCheck.cs
- StatusBarPanelClickEvent.cs
- TcpStreams.cs
- IncrementalReadDecoders.cs
- PageContent.cs
- MLangCodePageEncoding.cs
- EventLogPermissionEntry.cs
- BoolExpression.cs
- Psha1DerivedKeyGenerator.cs
- EventMetadata.cs
- DefaultTextStore.cs
- WindowsListViewItemCheckBox.cs
- XmlAttributes.cs
- CellRelation.cs
- TabItem.cs
- AspProxy.cs