Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Automation / Peers / RangeBaseAutomationPeer.cs / 1 / RangeBaseAutomationPeer.cs
using System; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class RangeBaseAutomationPeer : FrameworkElementAutomationPeer, IRangeValueProvider { /// public RangeBaseAutomationPeer(RangeBase owner): base(owner) { } /// override public object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.RangeValue) return this; return null; } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseMinimumPropertyChangedEvent(double oldValue, double newValue) { RaisePropertyChangedEvent(RangeValuePatternIdentifiers.MinimumProperty, oldValue, newValue); } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseMaximumPropertyChangedEvent(double oldValue, double newValue) { RaisePropertyChangedEvent(RangeValuePatternIdentifiers.MaximumProperty, oldValue, newValue); } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseValuePropertyChangedEvent(double oldValue, double newValue) { RaisePropertyChangedEvent(RangeValuePatternIdentifiers.ValueProperty, oldValue, newValue); } ////// Request to set the value that this UI element is representing /// /// Value to set the UI to, as an object ///true if the UI element was successfully set to the specified value //[CodeAnalysis("AptcaMethodsShouldOnlyCallAptcaMethods")] //Tracking Bug: 29647 void IRangeValueProvider.SetValue(double val) { if (!IsEnabled()) throw new ElementNotEnabledException(); RangeBase owner = (RangeBase)Owner; if (val < owner.Minimum || val > owner.Maximum) { throw new ArgumentOutOfRangeException("val"); } owner.Value = (double)val; } ///Value of a value control, as an object double IRangeValueProvider.Value { get { return ((RangeBase)Owner).Value; } } ///Indicates that the value can only be read, not modified. ///returns True if the control is read-only bool IRangeValueProvider.IsReadOnly { get { return !IsEnabled(); } } ///maximum value double IRangeValueProvider.Maximum { get { return ((RangeBase)Owner).Maximum; } } ///minimum value double IRangeValueProvider.Minimum { get { return ((RangeBase)Owner).Minimum; } } ///Value of a Large Change double IRangeValueProvider.LargeChange { get { return ((RangeBase)Owner).LargeChange; } } ///Value of a Small Change double IRangeValueProvider.SmallChange { get { return ((RangeBase)Owner).SmallChange; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class RangeBaseAutomationPeer : FrameworkElementAutomationPeer, IRangeValueProvider { /// public RangeBaseAutomationPeer(RangeBase owner): base(owner) { } /// override public object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.RangeValue) return this; return null; } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseMinimumPropertyChangedEvent(double oldValue, double newValue) { RaisePropertyChangedEvent(RangeValuePatternIdentifiers.MinimumProperty, oldValue, newValue); } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseMaximumPropertyChangedEvent(double oldValue, double newValue) { RaisePropertyChangedEvent(RangeValuePatternIdentifiers.MaximumProperty, oldValue, newValue); } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseValuePropertyChangedEvent(double oldValue, double newValue) { RaisePropertyChangedEvent(RangeValuePatternIdentifiers.ValueProperty, oldValue, newValue); } ////// Request to set the value that this UI element is representing /// /// Value to set the UI to, as an object ///true if the UI element was successfully set to the specified value //[CodeAnalysis("AptcaMethodsShouldOnlyCallAptcaMethods")] //Tracking Bug: 29647 void IRangeValueProvider.SetValue(double val) { if (!IsEnabled()) throw new ElementNotEnabledException(); RangeBase owner = (RangeBase)Owner; if (val < owner.Minimum || val > owner.Maximum) { throw new ArgumentOutOfRangeException("val"); } owner.Value = (double)val; } ///Value of a value control, as an object double IRangeValueProvider.Value { get { return ((RangeBase)Owner).Value; } } ///Indicates that the value can only be read, not modified. ///returns True if the control is read-only bool IRangeValueProvider.IsReadOnly { get { return !IsEnabled(); } } ///maximum value double IRangeValueProvider.Maximum { get { return ((RangeBase)Owner).Maximum; } } ///minimum value double IRangeValueProvider.Minimum { get { return ((RangeBase)Owner).Minimum; } } ///Value of a Large Change double IRangeValueProvider.LargeChange { get { return ((RangeBase)Owner).LargeChange; } } ///Value of a Small Change double IRangeValueProvider.SmallChange { get { return ((RangeBase)Owner).SmallChange; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
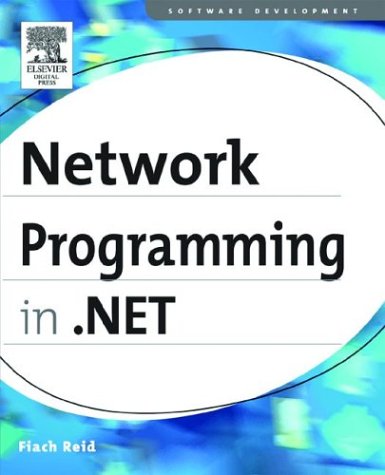
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlWriter.cs
- ContractReference.cs
- EncryptedKeyIdentifierClause.cs
- FormViewModeEventArgs.cs
- AddressingProperty.cs
- XmlSchemaAttributeGroupRef.cs
- DataSourceCacheDurationConverter.cs
- WebResourceUtil.cs
- CodeTypeDeclarationCollection.cs
- UndoManager.cs
- CatalogZoneBase.cs
- SQLStringStorage.cs
- MSG.cs
- WebServiceErrorEvent.cs
- XmlAttribute.cs
- XmlIncludeAttribute.cs
- ExternalCalls.cs
- ReachPrintTicketSerializerAsync.cs
- MatrixConverter.cs
- VirtualPathProvider.cs
- PeerNameRecordCollection.cs
- MulticastDelegate.cs
- BitmapImage.cs
- Utilities.cs
- ConditionedDesigner.cs
- SessionIDManager.cs
- ApplicationServiceHelper.cs
- MatrixAnimationUsingKeyFrames.cs
- ThicknessKeyFrameCollection.cs
- SiteMapNodeCollection.cs
- DbCommandTree.cs
- WebPartConnectionsConfigureVerb.cs
- AlgoModule.cs
- XmlSchemaImport.cs
- WebPartChrome.cs
- UpdatePanel.cs
- HwndTarget.cs
- DiscoveryExceptionDictionary.cs
- WebSysDescriptionAttribute.cs
- UserControlDocumentDesigner.cs
- ToolStripDropDown.cs
- IImplicitResourceProvider.cs
- RadioButtonRenderer.cs
- Int64AnimationUsingKeyFrames.cs
- RootBrowserWindowAutomationPeer.cs
- AuthorizationSection.cs
- SubMenuStyleCollection.cs
- CookieParameter.cs
- DesignBindingConverter.cs
- Win32Exception.cs
- IIS7UserPrincipal.cs
- WebBrowserDesigner.cs
- COAUTHINFO.cs
- shaper.cs
- Stacktrace.cs
- NotifyCollectionChangedEventArgs.cs
- FileDialogPermission.cs
- DummyDataSource.cs
- TableItemStyle.cs
- DBParameter.cs
- DateTimeFormat.cs
- DeviceContexts.cs
- DateTimeConverter2.cs
- DrawingDrawingContext.cs
- LinqDataSourceDeleteEventArgs.cs
- WebPartVerbCollection.cs
- InternalCache.cs
- DesignerProperties.cs
- AssemblyNameProxy.cs
- _TransmitFileOverlappedAsyncResult.cs
- ConfigurationErrorsException.cs
- TableRow.cs
- ReachVisualSerializer.cs
- EnumMemberAttribute.cs
- InitiatorSessionSymmetricTransportSecurityProtocol.cs
- SimpleMailWebEventProvider.cs
- ReferenceSchema.cs
- InfoCardCryptoHelper.cs
- IIS7UserPrincipal.cs
- SerializationAttributes.cs
- CustomValidator.cs
- WindowsStatic.cs
- QueueAccessMode.cs
- IncrementalReadDecoders.cs
- ErrorEventArgs.cs
- MouseCaptureWithinProperty.cs
- ServiceParser.cs
- Currency.cs
- StaticTextPointer.cs
- Token.cs
- RunInstallerAttribute.cs
- BitmapImage.cs
- _FtpDataStream.cs
- ResXDataNode.cs
- linebase.cs
- recordstatefactory.cs
- JournalNavigationScope.cs
- ComponentCommands.cs
- UIElement3DAutomationPeer.cs
- GridViewColumn.cs