Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / ReachPrintTicketSerializerAsync.cs / 1 / ReachPrintTicketSerializerAsync.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachPrintTicketSerializerAsync.cs Abstract: This file contains the definition of a class that defines the common functionality required to serialize a PrintTicket. Author: [....] ([....]) 25-May-2005 Revision History: --*/ using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Markup; using System.Printing; namespace System.Windows.Xps.Serialization { ////// Class defining common functionality required to /// serialize a PrintTicket. /// internal class PrintTicketSerializerAsync : ReachSerializerAsync { #region Constructor ////// Constructor for class PrintTicketSerializer /// /// /// The serialization manager, the services of which are /// used later in the serialization process of the type. /// public PrintTicketSerializerAsync( PackageSerializationManager manager ): base(manager) { } #endregion Constructor #region Public Methods ////// The main method that is called to serialize a PrintTicket. /// /// /// Instance of object to be serialized. /// public override void SerializeObject( object serializedObject ) { PrintTicket printTicket = serializedObject as PrintTicket; if (printTicket == null) { // // Throw a meaningful exception // throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_TargetNotPrintTicket)); } ((XpsSerializationManagerAsync)SerializationManager). PackagingPolicy.PersistPrintTicket(printTicket); } #endregion Public Methods #region Internal Methods ////// The main method that is called to serialize the PrintTicket /// and that is usually called from within the serialization manager /// when a node in the graph of objects is at a turn where it should /// be serialized. /// /// /// The context of the property being serialized at this time and /// it points internally to the object encapsulated by that node. /// internal override void SerializeObject( SerializablePropertyContext serializedProperty ) { if(serializedProperty == null) { throw new ArgumentNullException("serializedProperty"); } SerializeObject(serializedProperty.Value); } ////// Persists the object for the print ticket but in this case it is /// not utilized /// internal override void PersistObjectData( SerializableObjectContext serializableObjectContext ) { // // Do nothing here // } #endregion Internal Methods }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
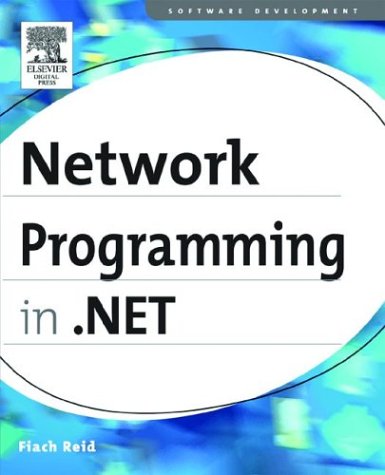
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ShaperBuffers.cs
- TypeBuilderInstantiation.cs
- CharAnimationUsingKeyFrames.cs
- CapabilitiesAssignment.cs
- RegexCaptureCollection.cs
- _ConnectStream.cs
- MouseButton.cs
- EntityConnectionStringBuilder.cs
- ListItemCollection.cs
- InvalidCommandTreeException.cs
- Matrix.cs
- ItemAutomationPeer.cs
- RealizationContext.cs
- PathFigureCollection.cs
- RestClientProxyHandler.cs
- PageContentCollection.cs
- LayoutEngine.cs
- ByteStack.cs
- StreamHelper.cs
- FragmentQueryProcessor.cs
- WebBrowserNavigatedEventHandler.cs
- TypeDescriptionProvider.cs
- PerfService.cs
- CategoryNameCollection.cs
- MenuItem.cs
- CanonicalFontFamilyReference.cs
- StatusBar.cs
- ItemsPresenter.cs
- RegisteredArrayDeclaration.cs
- ICollection.cs
- DropSourceBehavior.cs
- LogConverter.cs
- NativeObjectSecurity.cs
- ResolvedKeyFrameEntry.cs
- EmptyStringExpandableObjectConverter.cs
- XmlBinaryReader.cs
- FilterQuery.cs
- coordinator.cs
- HtmlTitle.cs
- DataColumnCollection.cs
- ProcessHost.cs
- ClientOptions.cs
- UInt32Storage.cs
- TextPointer.cs
- EntityViewGenerationAttribute.cs
- AuthorizationSection.cs
- formatter.cs
- CheckBoxRenderer.cs
- SafeWaitHandle.cs
- SerialPort.cs
- DataColumnCollection.cs
- HttpPostedFile.cs
- X509ChainPolicy.cs
- CapabilitiesSection.cs
- XmlDataContract.cs
- BitVector32.cs
- TypeConverterValueSerializer.cs
- BindingGroup.cs
- DataView.cs
- ErrorEventArgs.cs
- RequiredFieldValidator.cs
- DocumentOrderQuery.cs
- HttpWriter.cs
- ErrorView.xaml.cs
- Viewport3DAutomationPeer.cs
- AttributeCollection.cs
- XmlAttributeAttribute.cs
- HGlobalSafeHandle.cs
- shaperfactory.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- BrushConverter.cs
- AsyncStreamReader.cs
- ACE.cs
- DeclarativeCatalogPartDesigner.cs
- CodeNamespaceCollection.cs
- PropertyIDSet.cs
- RegistrySecurity.cs
- CustomTokenProvider.cs
- Selector.cs
- ClientSettingsStore.cs
- XPathArrayIterator.cs
- CachingHintValidation.cs
- MouseGestureValueSerializer.cs
- BindingEditor.xaml.cs
- UnsafeNativeMethods.cs
- COM2FontConverter.cs
- HttpPostedFile.cs
- ResourceCategoryAttribute.cs
- PrintDialog.cs
- GridPatternIdentifiers.cs
- ConstNode.cs
- IsolatedStorageFilePermission.cs
- SnapshotChangeTrackingStrategy.cs
- QilLiteral.cs
- FileFormatException.cs
- CommandPlan.cs
- BuildDependencySet.cs
- FixedSOMLineCollection.cs
- TextUtf8RawTextWriter.cs
- QilInvokeLateBound.cs