Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Core.Presentation / System / ServiceModel / Activities / Presentation / BindingEditor.xaml.cs / 1305376 / BindingEditor.xaml.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Activities.Presentation { using System.Collections.Generic; using System.Linq; using System.ServiceModel; using System.ServiceModel.Channels; using System.Windows; using System.Windows.Controls; using System.Activities.Presentation.Model; using System.Configuration; using System.ServiceModel.Configuration; using System.Activities.Presentation; partial class BindingEditor { public static readonly DependencyProperty BindingProperty = DependencyProperty.Register("Binding", typeof(object), typeof(BindingEditor), new PropertyMetadata(OnBindingChanged)); ListbindingElements = new List (); bool isInitializing; public BindingEditor() { InitializeComponent(); } public object Binding { get { return GetValue(BindingProperty); } set { SetValue(BindingProperty, value); } } void LoadBindings() { try { this.bindingElements.Add(new BindingDescriptor { BindingName = (string)(this.TryFindResource("bindingEditorEmptyBindingLabel") ?? "none"), Value = null }); Configuration machineConfig = ConfigurationManager.OpenMachineConfiguration(); ServiceModelSectionGroup section = ServiceModelSectionGroup.GetSectionGroup(machineConfig); if (null != section && null != section.Bindings) { this.bindingElements.AddRange(section.Bindings.BindingCollections. OrderBy(p => p.BindingName). Select (p => new BindingDescriptor() { BindingName = p.BindingName, Value = p })); } } catch (ConfigurationErrorsException err) { ErrorReporting.ShowErrorMessage(err.Message); } } protected override void OnInitialized(EventArgs e) { base.OnInitialized(e); this.isInitializing = true; LoadBindings(); this.ItemsSource = this.bindingElements; this.SelectedIndex = 0; this.isInitializing = false; } protected override void OnSelectionChanged(SelectionChangedEventArgs e) { base.OnSelectionChanged(e); if (!this.isInitializing) { BindingDescriptor entry = (BindingDescriptor)e.AddedItems[0]; if (null == entry.Value) { Binding = null; } // try to avoid blowing away any binding that has been custom-tweaked in XAML. else if (Binding == null || !(Binding is ModelItem) || !((ModelItem)Binding).ItemType.Equals(entry.Value.BindingType)) { Binding instance = (Binding)Activator.CreateInstance(entry.Value.BindingType); instance.Name = entry.BindingName; Binding = instance; } } } static void OnBindingChanged(DependencyObject sender, DependencyPropertyChangedEventArgs args) { BindingEditor editor = (BindingEditor)sender; object newValue = args.NewValue; Type bindingType = null; ModelItem item = newValue as ModelItem; string bindingName = null; if (item != null) { bindingType = item.ItemType; bindingName = (string)item.Properties["Name"].ComputedValue; } else if (newValue != null) { bindingType = newValue.GetType(); if (typeof(Binding).IsAssignableFrom(bindingType)) { bindingName = ((Binding)newValue).Name; } } // Make combo appear empty if the binding is not one of the ones known to us, e.g., has been custom-tweaked in XAML. BindingDescriptor toSelect = null; Func where = p => null != p.Value && p.Value.BindingType == bindingType; if (editor.bindingElements.Count(where) > 1) { toSelect = editor.bindingElements.Where(where).Where(p => string.Equals(p.BindingName, bindingName)).FirstOrDefault(); } else { toSelect = editor.bindingElements.Where(where).FirstOrDefault(); } //prevent OnSelectionChanged now - the binding is set directly to the object, no need to set again through event handler editor.isInitializing = true; if (null != toSelect) { editor.SelectedItem = toSelect; } else { editor.SelectedIndex = 0; } //allow selection changed events to be consumed again editor.isInitializing = false; } sealed class BindingDescriptor { public string BindingName { get; internal set; } public BindingCollectionElement Value { get; internal set; } public override string ToString() { return BindingName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
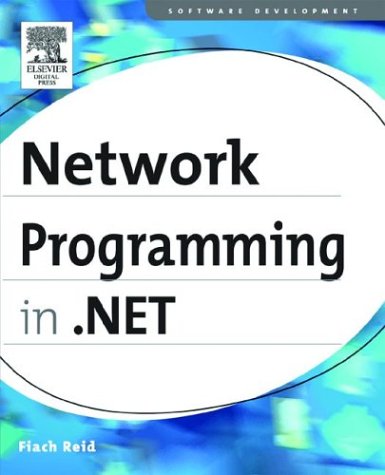
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- sqlnorm.cs
- DateTimeConverter.cs
- CompressStream.cs
- XmlC14NWriter.cs
- AggregatePushdown.cs
- XXXOnTypeBuilderInstantiation.cs
- FloaterParagraph.cs
- XomlCompilerResults.cs
- UnsafeNativeMethodsCLR.cs
- SelfIssuedAuthRSACryptoProvider.cs
- FirstMatchCodeGroup.cs
- DataSpaceManager.cs
- TreeNodeCollection.cs
- CallContext.cs
- RsaKeyIdentifierClause.cs
- StrokeNodeEnumerator.cs
- WebBrowser.cs
- FormsAuthenticationUserCollection.cs
- KeyboardDevice.cs
- NestPullup.cs
- CompoundFileStorageReference.cs
- X509Certificate2Collection.cs
- TreeNodeCollection.cs
- WebPartManagerInternals.cs
- NullRuntimeConfig.cs
- MultiAsyncResult.cs
- Currency.cs
- EventTask.cs
- SpecularMaterial.cs
- XmlSchema.cs
- DataGridAddNewRow.cs
- HttpServerUtilityWrapper.cs
- RoutedPropertyChangedEventArgs.cs
- FamilyMap.cs
- FileVersion.cs
- SelectedGridItemChangedEvent.cs
- WebPartEventArgs.cs
- InputProviderSite.cs
- XsdBuilder.cs
- AdCreatedEventArgs.cs
- AliasExpr.cs
- FaultDesigner.cs
- Validator.cs
- SqlTypeSystemProvider.cs
- Timeline.cs
- ActivityBuilder.cs
- DataMember.cs
- SrgsItemList.cs
- Paragraph.cs
- OrderPreservingSpoolingTask.cs
- CompensableActivity.cs
- XmlILConstructAnalyzer.cs
- DataGridViewColumnConverter.cs
- UnsafeNativeMethods.cs
- TimelineCollection.cs
- GeometryDrawing.cs
- TransactionTraceIdentifier.cs
- FormViewModeEventArgs.cs
- HttpRuntime.cs
- DragEvent.cs
- RtType.cs
- StylusButtonCollection.cs
- EncodingTable.cs
- NetworkAddressChange.cs
- TypeSystemProvider.cs
- DocobjHost.cs
- XmlAggregates.cs
- FlowDocumentView.cs
- WindowsAltTab.cs
- LicFileLicenseProvider.cs
- DataGridViewSelectedColumnCollection.cs
- DecimalAnimation.cs
- PlanCompilerUtil.cs
- ZoneIdentityPermission.cs
- SelfIssuedSamlTokenFactory.cs
- DesignerAdapterUtil.cs
- COM2IDispatchConverter.cs
- Identity.cs
- TrackingValidationObjectDictionary.cs
- SecurityPermission.cs
- TargetConverter.cs
- BaseHashHelper.cs
- Encoder.cs
- PluralizationServiceUtil.cs
- TextServicesPropertyRanges.cs
- SqlStream.cs
- DBAsyncResult.cs
- MethodToken.cs
- UnsafeCollabNativeMethods.cs
- DataGridDesigner.cs
- Matrix3DValueSerializer.cs
- Calendar.cs
- FtpWebRequest.cs
- nulltextcontainer.cs
- SingleConverter.cs
- RemotingException.cs
- TextProperties.cs
- DynamicContractTypeBuilder.cs
- Validator.cs
- TextUtf8RawTextWriter.cs