Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / LicFileLicenseProvider.cs / 1 / LicFileLicenseProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel { using System; using System.ComponentModel.Design; using System.Diagnostics; using System.Globalization; using System.IO; using System.Reflection; using System.Runtime.Remoting; using System.Security.Permissions; ////// /// [HostProtection(SharedState = true)] public class LicFileLicenseProvider : LicenseProvider { ///Provides an implementation of a ///. The provider works in /// a similar fashion to Microsoft .NET Framework standard licensing module. /// /// protected virtual bool IsKeyValid(string key, Type type) { if (key != null) { return key.StartsWith(GetKey(type)); } return false; } ///Determines if the key retrieved by the ///method is valid /// for the specified type. /// /// Creates a key for the specified type. /// protected virtual string GetKey(Type type) { // This string should not be localized. // return string.Format(CultureInfo.InvariantCulture, "{0} is a licensed component.", type.FullName); } ////// /// public override License GetLicense(LicenseContext context, Type type, object instance, bool allowExceptions) { LicFileLicense lic = null; Debug.Assert(context != null, "No context provided!"); if (context != null) { if (context.UsageMode == LicenseUsageMode.Runtime) { string key = context.GetSavedLicenseKey(type, null); if (key != null && IsKeyValid(key, type)) { lic = new LicFileLicense(this, key); } } if (lic == null) { string modulePath = null; if (context != null) { ITypeResolutionService resolver = (ITypeResolutionService)context.GetService(typeof(ITypeResolutionService)); if (resolver != null) { modulePath = resolver.GetPathOfAssembly(type.Assembly.GetName()); } } if (modulePath == null) { modulePath = type.Module.FullyQualifiedName; } string moduleDir = Path.GetDirectoryName(modulePath); string licenseFile = moduleDir + "\\" + type.FullName + ".lic"; Debug.WriteLine("Looking for license in: " + licenseFile); if (File.Exists(licenseFile)) { Stream licStream = new FileStream(licenseFile, FileMode.Open, FileAccess.Read, FileShare.Read); StreamReader sr = new StreamReader(licStream); string s = sr.ReadLine(); sr.Close(); if (IsKeyValid(s, type)) { lic = new LicFileLicense(this, GetKey(type)); } } if (lic != null) { context.SetSavedLicenseKey(type, lic.LicenseKey); } } } return lic; } private class LicFileLicense : License { private LicFileLicenseProvider owner; private string key; public LicFileLicense(LicFileLicenseProvider owner, string key) { this.owner = owner; this.key = key; } public override string LicenseKey { get { return key; } } public override void Dispose() { } } } }Gets a license for the instance of the component and determines if it is valid. ///
Link Menu
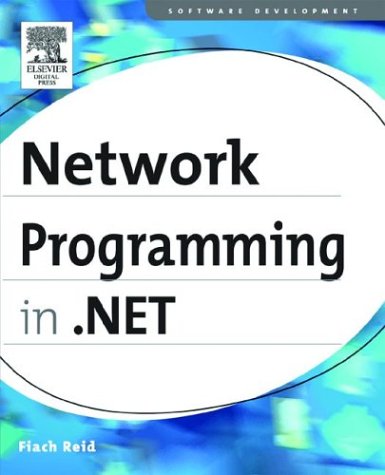
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeSnippetStatement.cs
- DataSpaceManager.cs
- ScriptIgnoreAttribute.cs
- EntityContainerAssociationSet.cs
- StylusPointCollection.cs
- MetadataUtilsSmi.cs
- AttributeXamlType.cs
- Double.cs
- CreateParams.cs
- ResXDataNode.cs
- ComponentManagerBroker.cs
- HtmlInputRadioButton.cs
- PopupRoot.cs
- ServicePointManagerElement.cs
- ProfileEventArgs.cs
- EntitySqlQueryBuilder.cs
- FocusManager.cs
- SingleKeyFrameCollection.cs
- RewritingPass.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- DispatchChannelSink.cs
- TableLayoutPanelCellPosition.cs
- ImageListUtils.cs
- ToolStripPanelRow.cs
- AccessibleObject.cs
- DateTimeValueSerializerContext.cs
- FieldMetadata.cs
- ProtocolsConfiguration.cs
- XPathNavigatorReader.cs
- XmlUnspecifiedAttribute.cs
- ThemeConfigurationDialog.cs
- StoreContentChangedEventArgs.cs
- Block.cs
- OleDbPermission.cs
- WebPart.cs
- GetPageCompletedEventArgs.cs
- BinarySecretKeyIdentifierClause.cs
- DerivedKeyCachingSecurityTokenSerializer.cs
- TypeConverter.cs
- LinqToSqlWrapper.cs
- AutoCompleteStringCollection.cs
- coordinator.cs
- RowType.cs
- BamlResourceContent.cs
- SqlError.cs
- Empty.cs
- HttpHandlersInstallComponent.cs
- Latin1Encoding.cs
- View.cs
- TextAdaptor.cs
- sqlinternaltransaction.cs
- ManagedIStream.cs
- IncrementalHitTester.cs
- Enum.cs
- WindowsHyperlink.cs
- SimpleFieldTemplateUserControl.cs
- SpeakCompletedEventArgs.cs
- LoginCancelEventArgs.cs
- EntityContainerEmitter.cs
- ListDependantCardsRequest.cs
- QueryableDataSourceView.cs
- WebServiceTypeData.cs
- ConfigurationManagerHelperFactory.cs
- DataBindingHandlerAttribute.cs
- OleCmdHelper.cs
- ClientRuntimeConfig.cs
- ActiveXHost.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- SettingsBindableAttribute.cs
- StackBuilderSink.cs
- SchemaNotation.cs
- DataPagerFieldItem.cs
- EdmProperty.cs
- SqlRewriteScalarSubqueries.cs
- MutableAssemblyCacheEntry.cs
- TransactionScope.cs
- KeyFrames.cs
- Code.cs
- ServicePointManager.cs
- TransactionManager.cs
- XmlNamedNodeMap.cs
- KnownTypesProvider.cs
- elementinformation.cs
- InvalidComObjectException.cs
- UniqueID.cs
- XmlReflectionImporter.cs
- ChannelManager.cs
- StorageBasedPackageProperties.cs
- FontStretchConverter.cs
- CodePrimitiveExpression.cs
- ExtensibleClassFactory.cs
- LocalBuilder.cs
- HTMLTagNameToTypeMapper.cs
- AdjustableArrowCap.cs
- Crypto.cs
- EllipseGeometry.cs
- FrameworkElementFactoryMarkupObject.cs
- HttpResponseInternalWrapper.cs
- ExitEventArgs.cs
- HitTestFilterBehavior.cs