Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / ListDependantCardsRequest.cs / 1 / ListDependantCardsRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Collections.Generic; using System.IO; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // Processes a request to find the list of managed cards that use a specific // self issued card for authentication to the IP // // // Specify valid parent requests. // class ListDependantCardsRequest :UIAgentRequest { Uri m_selfIssuedCardId; // Specifies the card identifier. Listm_allCards = new List (); public ListDependantCardsRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : base( rpcHandle, inArgs, outArgs, parent ) { } // // Summary // Marshals input arguments for the request. The arguments are read from a stream in binary. // protected override void OnMarshalInArgs() { BinaryReader reader = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); m_selfIssuedCardId = new Uri( Utility.DeserializeString( reader ) ); } // // Summary // Processes the request. // protected override void OnProcess() { StoreConnection connection = StoreConnection.GetConnection(); try { // // Retrieve all the managed cards that use self issued cards for authentication // // // Get the full list of all infocards. // IList rows = (IList )connection.Query( QueryDetails.FullRow, connection.LocalDataSource, new QueryParameter( SecondaryIndexDefinition.SupportedAuthIndex, (Int32)TokenFactoryCredentialType.SelfIssuedCredential ) ); if( null != rows && 0 != rows.Count ) { for( int i = 0; i < rows.Count; i++ ) { byte[ ] rawForm = rows[ i ].GetDataField(); try { using( MemoryStream stream = new MemoryStream( rawForm ) ) { InfoCard card = new InfoCard( stream ); foreach( TokenCreationParameter param in card.CreationParameters ) { if( param.CredentialType == TokenFactoryCredentialType.SelfIssuedCredential ) { string ppid = param.CredentialSelectors[ CredentialSelectorType.SelfIssuedCardIdSelector ].GetStringWithoutNullTerminator(); if( Utility.CompareByteArrays( Convert.FromBase64String( ppid ), Utility.CreateHash( card.IssuerIdentifierAsBytes, m_selfIssuedCardId ) ) ) { m_allCards.Add( card ); } } } } } finally { Array.Clear( rawForm, 0, rawForm.Length ); } } } } finally { connection.Close(); } } // // Summary // Marshals output arguments for the request. The arguments are written to a stream in binary. // protected override void OnMarshalOutArgs() { Stream stream = OutArgs; BinaryWriter writer = new BinaryWriter( stream, System.Text.Encoding.Unicode ); if( null != m_allCards ) { writer.Write( (Int32)m_allCards.Count); foreach( InfoCard card in m_allCards ) { Utility.SerializeUri( writer, card.Id ); } } else { writer.Write( (Int32)0 ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
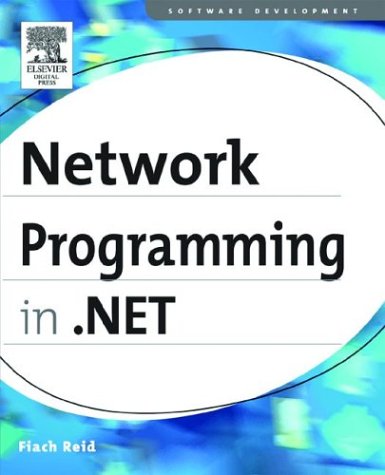
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RepeatInfo.cs
- RangeValidator.cs
- SpellerInterop.cs
- CodeDelegateInvokeExpression.cs
- ISessionStateStore.cs
- loginstatus.cs
- HandlerWithFactory.cs
- hwndwrapper.cs
- PauseStoryboard.cs
- QuaternionConverter.cs
- InvokeAction.cs
- StrongNameIdentityPermission.cs
- ObjectComplexPropertyMapping.cs
- SettingsProviderCollection.cs
- IncrementalHitTester.cs
- FirstMatchCodeGroup.cs
- QilVisitor.cs
- XmlUrlResolver.cs
- BinaryParser.cs
- StringResourceManager.cs
- ProviderConnectionPointCollection.cs
- SafeCoTaskMem.cs
- InstanceData.cs
- AggregatePushdown.cs
- ObjectViewListener.cs
- ChannelSinkStacks.cs
- VisualStyleInformation.cs
- TaskFileService.cs
- GroupItem.cs
- WebAdminConfigurationHelper.cs
- HttpException.cs
- ClaimSet.cs
- XmlText.cs
- MergeLocalizationDirectives.cs
- DataViewManager.cs
- InputLanguageEventArgs.cs
- ProtectedConfigurationSection.cs
- SmiMetaData.cs
- PublisherIdentityPermission.cs
- CheckBox.cs
- SqlDataSource.cs
- CultureInfoConverter.cs
- InputLanguageSource.cs
- StylusCollection.cs
- HtmlElementEventArgs.cs
- MessageSecurityOverHttp.cs
- DataBoundControl.cs
- PerspectiveCamera.cs
- XmlDictionaryReader.cs
- Debug.cs
- FunctionCommandText.cs
- SiteMapDataSourceView.cs
- EnumBuilder.cs
- ImageSource.cs
- Knowncolors.cs
- DataSourceSelectArguments.cs
- SelectionChangedEventArgs.cs
- AssemblyCacheEntry.cs
- WmpBitmapEncoder.cs
- Region.cs
- SimplePropertyEntry.cs
- PartialArray.cs
- Input.cs
- SiteMapHierarchicalDataSourceView.cs
- Compiler.cs
- ProcessInputEventArgs.cs
- WindowClosedEventArgs.cs
- EmptyTextWriter.cs
- RectangleGeometry.cs
- CancellationToken.cs
- Rect3DConverter.cs
- DependencyPropertyDescriptor.cs
- StatusBarItem.cs
- FontFamily.cs
- DataGridItem.cs
- DocumentXmlWriter.cs
- ObjectItemAttributeAssemblyLoader.cs
- HttpRawResponse.cs
- backend.cs
- BindableAttribute.cs
- Point.cs
- WeakEventManager.cs
- UnsafeMethods.cs
- COM2PropertyBuilderUITypeEditor.cs
- SingleAnimationBase.cs
- ConfigurationSectionHelper.cs
- IndentedTextWriter.cs
- sqlcontext.cs
- DropDownList.cs
- ThreadStartException.cs
- DataServiceHost.cs
- ListParagraph.cs
- DataGridViewColumnCollectionDialog.cs
- ClientConvert.cs
- DetailsViewCommandEventArgs.cs
- ResourceManager.cs
- StandardBindingElementCollection.cs
- LogicalExpr.cs
- ProfilePropertyNameValidator.cs
- SettingsPropertyValue.cs