Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Region.cs / 1305376 / Region.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Drawing2D; using System.Drawing; using System.Drawing.Internal; using System.Globalization; using System.Runtime.Versioning; /** * Represent a Region object */ ////// /// public sealed class Region : MarshalByRefObject, IDisposable { #if FINALIZATION_WATCH private string allocationSite = Graphics.GetAllocationStack(); #endif /** * Construct a new region object */ ////// Describes the interior of a graphics shape /// composed of rectangles and paths. /// ////// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public Region() { IntPtr region = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateRegion(out region); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeRegion(region); } ////// Initializes a new instance of the ///class. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public Region(RectangleF rect) { IntPtr region = IntPtr.Zero; GPRECTF gprectf = rect.ToGPRECTF(); int status = SafeNativeMethods.Gdip.GdipCreateRegionRect(ref gprectf, out region); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeRegion(region); } ////// Initializes a new instance of the ///class from the specified . /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public Region(Rectangle rect) { IntPtr region = IntPtr.Zero; GPRECT gprect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCreateRegionRectI(ref gprect, out region); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeRegion(region); } ////// Initializes a new instance of the ///class from the specified . /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public Region(GraphicsPath path) { if (path == null) throw new ArgumentNullException("path"); IntPtr region = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateRegionPath(new HandleRef(path, path.nativePath), out region); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeRegion(region); } ////// Initializes a new instance of the ///class /// with the specified . /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public Region(RegionData rgnData) { if (rgnData == null) throw new ArgumentNullException("rgnData"); IntPtr region = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateRegionRgnData(rgnData.Data, rgnData.Data.Length, out region); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeRegion(region); } internal Region(IntPtr nativeRegion) { SetNativeRegion(nativeRegion); } ///class /// from the specified data. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public static Region FromHrgn(IntPtr hrgn) { IntSecurity.ObjectFromWin32Handle.Demand(); IntPtr region = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateRegionHrgn(new HandleRef(null, hrgn), out region); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new Region(region); } [System.Runtime.TargetedPatchingOptOutAttribute("Performance critical to inline across NGen image boundaries")] private void SetNativeRegion(IntPtr nativeRegion) { if (nativeRegion == IntPtr.Zero) throw new ArgumentNullException("nativeRegion"); this.nativeRegion = nativeRegion; } /** * Make a copy of the region object */ ///class /// from the specified existing . /// /// /// Creates an exact copy if this [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public Region Clone() { IntPtr region = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneRegion(new HandleRef(this, nativeRegion), out region); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new Region(region); } /** * Dispose of resources associated with the */ ///. /// /// /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } void Dispose(bool disposing) { #if FINALIZATION_WATCH if (!disposing && nativeRegion != IntPtr.Zero) Debug.WriteLine("**********************\nDisposed through finalization:\n" + allocationSite); #endif if (nativeRegion != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeleteRegion(new HandleRef(this, nativeRegion)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeRegion = IntPtr.Zero; } } } ////// Cleans up Windows resources for this /// ///. /// /// /// Cleans up Windows resources for this /// ~Region() { Dispose(false); } /* * Region operations */ ///. /// /// /// Initializes this public void MakeInfinite() { int status = SafeNativeMethods.Gdip.GdipSetInfinite(new HandleRef(this, nativeRegion)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///to an /// infinite interior. /// /// /// Initializes this public void MakeEmpty() { int status = SafeNativeMethods.Gdip.GdipSetEmpty(new HandleRef(this, nativeRegion)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // float version ///to an /// empty interior. /// /// /// Updates this public void Intersect(RectangleF rect) { GPRECTF gprectf = rect.ToGPRECTF(); int status = SafeNativeMethods.Gdip.GdipCombineRegionRect(new HandleRef(this, nativeRegion), ref gprectf, CombineMode.Intersect); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // int version ///to the intersection of itself /// with the specified . /// /// /// public void Intersect(Rectangle rect) { GPRECT gprect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCombineRegionRectI(new HandleRef(this, nativeRegion), ref gprect, CombineMode.Intersect); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Updates this ///to the intersection of itself with the specified /// . /// /// /// public void Intersect(GraphicsPath path) { if (path == null) throw new ArgumentNullException("path"); int status = SafeNativeMethods.Gdip.GdipCombineRegionPath(new HandleRef(this, nativeRegion), new HandleRef(path, path.nativePath), CombineMode.Intersect); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Updates this ///to the intersection of itself with the specified /// . /// /// /// public void Intersect(Region region) { if (region == null) throw new ArgumentNullException("region"); int status = SafeNativeMethods.Gdip.GdipCombineRegionRegion(new HandleRef(this, nativeRegion), new HandleRef(region, region.nativeRegion), CombineMode.Intersect); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Updates this ///to the intersection of itself with the specified /// . /// /// /// Releases the handle to the region handle. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] public void ReleaseHrgn(IntPtr regionHandle) { IntSecurity.ObjectFromWin32Handle.Demand(); if (regionHandle == IntPtr.Zero) { throw new ArgumentNullException("regionHandle"); } SafeNativeMethods.IntDeleteObject(new HandleRef(this, regionHandle)); } // float version ////// /// public void Union(RectangleF rect) { GPRECTF gprectf = new GPRECTF(rect); int status = SafeNativeMethods.Gdip.GdipCombineRegionRect(new HandleRef(this, nativeRegion), ref gprectf, CombineMode.Union); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // int version ////// Updates this ///to the union of itself and the /// specified . /// /// /// Updates this public void Union(Rectangle rect) { GPRECT gprect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCombineRegionRectI(new HandleRef(this, nativeRegion), ref gprect, CombineMode.Union); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///to the union of itself and the /// specified . /// /// /// Updates this public void Union(GraphicsPath path) { if (path == null) throw new ArgumentNullException("path"); int status = SafeNativeMethods.Gdip.GdipCombineRegionPath(new HandleRef(this, nativeRegion), new HandleRef(path, path.nativePath), CombineMode.Union); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///to the union of itself and the /// specified . /// /// /// public void Union(Region region) { if (region == null) throw new ArgumentNullException("region"); int status = SafeNativeMethods.Gdip.GdipCombineRegionRegion(new HandleRef(this, nativeRegion), new HandleRef(region, region.nativeRegion), CombineMode.Union); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // float version ////// Updates this ///to the union of itself and the specified . /// /// /// Updates this public void Xor(RectangleF rect) { GPRECTF gprectf = new GPRECTF(rect); int status = SafeNativeMethods.Gdip.GdipCombineRegionRect(new HandleRef(this, nativeRegion), ref gprectf, CombineMode.Xor); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // int version ///to the union minus the /// intersection of itself with the specified . /// /// /// Updates this public void Xor(Rectangle rect) { GPRECT gprect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCombineRegionRectI(new HandleRef(this, nativeRegion), ref gprect, CombineMode.Xor); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///to the union minus the /// intersection of itself with the specified . /// /// /// Updates this public void Xor(GraphicsPath path) { if (path == null) throw new ArgumentNullException("path"); int status = SafeNativeMethods.Gdip.GdipCombineRegionPath(new HandleRef(this, nativeRegion), new HandleRef(path, path.nativePath), CombineMode.Xor); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///to the union minus the /// intersection of itself with the specified . /// /// /// Updates this public void Xor(Region region) { if (region == null) throw new ArgumentNullException("region"); int status = SafeNativeMethods.Gdip.GdipCombineRegionRegion(new HandleRef(this, nativeRegion), new HandleRef(region, region.nativeRegion), CombineMode.Xor); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // float version ///to the union minus the /// intersection of itself with the specified . /// /// /// Updates this public void Exclude(RectangleF rect) { GPRECTF gprectf = new GPRECTF(rect); int status = SafeNativeMethods.Gdip.GdipCombineRegionRect(new HandleRef(this, nativeRegion), ref gprectf, CombineMode.Exclude); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // int version ///to the portion of its interior /// that does not intersect with the specified . /// /// /// Updates this public void Exclude(Rectangle rect) { GPRECT gprect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCombineRegionRectI(new HandleRef(this, nativeRegion), ref gprect, CombineMode.Exclude); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///to the portion of its interior /// that does not intersect with the specified . /// /// /// Updates this public void Exclude(GraphicsPath path) { if (path == null) throw new ArgumentNullException("path"); int status = SafeNativeMethods.Gdip.GdipCombineRegionPath(new HandleRef(this, nativeRegion), new HandleRef(path, path.nativePath), CombineMode.Exclude); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///to the portion of its interior /// that does not intersect with the specified . /// /// /// Updates this public void Exclude(Region region) { if (region == null) throw new ArgumentNullException("region"); int status = SafeNativeMethods.Gdip.GdipCombineRegionRegion(new HandleRef(this, nativeRegion), new HandleRef(region, region.nativeRegion), CombineMode.Exclude); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // float version ///to the portion of its interior /// that does not intersect with the specified . /// /// /// Updates this public void Complement(RectangleF rect) { GPRECTF gprectf = rect.ToGPRECTF(); int status = SafeNativeMethods.Gdip.GdipCombineRegionRect(new HandleRef(this, nativeRegion), ref gprectf, CombineMode.Complement); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // int version ///to the portion of the /// specified that does not intersect with this . /// /// /// Updates this public void Complement(Rectangle rect) { GPRECT gprect = new GPRECT(rect); int status = SafeNativeMethods.Gdip.GdipCombineRegionRectI(new HandleRef(this, nativeRegion), ref gprect, CombineMode.Complement); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///to the portion of the /// specified that does not intersect with this . /// /// /// Updates this public void Complement(GraphicsPath path) { if (path == null) throw new ArgumentNullException("path"); int status = SafeNativeMethods.Gdip.GdipCombineRegionPath(new HandleRef(this, nativeRegion), new HandleRef(path, path.nativePath), CombineMode.Complement); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///to the portion of the /// specified that does not intersect with this /// . /// /// /// public void Complement(Region region) { if (region == null) throw new ArgumentNullException("region"); int status = SafeNativeMethods.Gdip.GdipCombineRegionRegion(new HandleRef(this, nativeRegion), new HandleRef(region, region.nativeRegion), CombineMode.Complement); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } /** * Transform operations */ ////// Updates this ///to the portion of the /// specified that does not intersect with this . /// /// /// Offsets the coordinates of this public void Translate(float dx, float dy) { int status = SafeNativeMethods.Gdip.GdipTranslateRegion(new HandleRef(this, nativeRegion), dx, dy); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///by the /// specified amount. /// /// /// Offsets the coordinates of this public void Translate(int dx, int dy) { int status = SafeNativeMethods.Gdip.GdipTranslateRegionI(new HandleRef(this, nativeRegion), dx, dy); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///by the /// specified amount. /// /// /// Transforms this public void Transform(Matrix matrix) { if (matrix == null) throw new ArgumentNullException("matrix"); int status = SafeNativeMethods.Gdip.GdipTransformRegion(new HandleRef(this, nativeRegion), new HandleRef(matrix, matrix.nativeMatrix)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } /** * Get region attributes */ ///by the /// specified . /// /// /// Returns a public RectangleF GetBounds(Graphics g) { if (g == null) throw new ArgumentNullException("g"); GPRECTF gprectf = new GPRECTF(); int status = SafeNativeMethods.Gdip.GdipGetRegionBounds(new HandleRef(this, nativeRegion), new HandleRef(g, g.NativeGraphics), ref gprectf); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return gprectf.ToRectangleF(); } ///that represents a rectangular /// region that bounds this on the drawing surface of a . /// /// /// Returns a Windows handle to this public IntPtr GetHrgn(Graphics g) { if (g == null) throw new ArgumentNullException("g"); IntPtr hrgn = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipGetRegionHRgn(new HandleRef(this, nativeRegion), new HandleRef(g, g.NativeGraphics), out hrgn); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return hrgn; } ///in the /// specified graphics context. /// /// Remarks from MSDN: /// It is the caller's responsibility to call the GDI function /// DeleteObject to free the GDI region when it is no longer needed. /// /// /// public bool IsEmpty(Graphics g) { if (g == null) throw new ArgumentNullException("g"); int isEmpty; int status = SafeNativeMethods.Gdip.GdipIsEmptyRegion(new HandleRef(this, nativeRegion), new HandleRef(g, g.NativeGraphics), out isEmpty); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return isEmpty != 0; } ////// Tests whether this ///has an /// empty interior on the specified drawing surface. /// /// /// public bool IsInfinite(Graphics g) { if (g == null) throw new ArgumentNullException("g"); int isInfinite; int status = SafeNativeMethods.Gdip.GdipIsInfiniteRegion(new HandleRef(this, nativeRegion), new HandleRef(g, g.NativeGraphics), out isInfinite); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return isInfinite != 0; } ////// Tests whether this ///has /// an infinite interior on the specified drawing surface. /// /// /// public bool Equals(Region region, Graphics g) { if (g == null) throw new ArgumentNullException("g"); if (region == null) throw new ArgumentNullException("region"); int isEqual; int status = SafeNativeMethods.Gdip.GdipIsEqualRegion(new HandleRef(this, nativeRegion), new HandleRef(region, region.nativeRegion), new HandleRef(g, g.NativeGraphics), out isEqual); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return isEqual != 0; } ////// Tests whether the specified ///is /// identical to this /// on the specified drawing surface. /// /// /// Returns a public RegionData GetRegionData() { int regionSize = 0; int status = SafeNativeMethods.Gdip.GdipGetRegionDataSize(new HandleRef(this, nativeRegion), out regionSize); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); if (regionSize == 0) return null; byte[] regionData = new byte[regionSize]; status = SafeNativeMethods.Gdip.GdipGetRegionData(new HandleRef(this, nativeRegion), regionData, regionSize, out regionSize); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new RegionData(regionData); } /* * Hit testing operations */ // float version ///that represents the /// information that describes this . /// /// /// public bool IsVisible(float x, float y) { return IsVisible(new PointF(x, y), null); } ////// Tests whether the specified point is /// contained within this ///in the specified graphics context. /// /// /// public bool IsVisible(PointF point) { return IsVisible(point, null); } ////// Tests whether the specified ///is contained within this . /// /// /// public bool IsVisible(float x, float y, Graphics g) { return IsVisible(new PointF(x, y), g); } ////// Tests whether the specified point is contained within this ///in the /// specified graphics context. /// /// /// public bool IsVisible(PointF point, Graphics g) { int isVisible; int status = SafeNativeMethods.Gdip.GdipIsVisibleRegionPoint(new HandleRef(this, nativeRegion), point.X, point.Y, new HandleRef(g, (g==null) ? IntPtr.Zero : g.NativeGraphics), out isVisible); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return isVisible != 0; } ////// Tests whether the specified ///is /// contained within this in the specified graphics context. /// /// /// public bool IsVisible(float x, float y, float width, float height) { return IsVisible(new RectangleF(x, y, width, height), null); } ////// Tests whether the specified rectangle is contained within this ////// . /// /// /// public bool IsVisible(RectangleF rect) { return IsVisible(rect, null); } ////// Tests whether the specified ///is contained within this /// . /// /// /// public bool IsVisible(float x, float y, float width, float height, Graphics g) { return IsVisible(new RectangleF(x, y, width, height), g); } ////// Tests whether the specified rectangle is contained within this ///in the /// specified graphics context. /// /// /// public bool IsVisible(RectangleF rect, Graphics g) { int isVisible = 0; int status = SafeNativeMethods.Gdip.GdipIsVisibleRegionRect(new HandleRef(this, nativeRegion), rect.X, rect.Y, rect.Width, rect.Height, new HandleRef(g, (g==null) ? IntPtr.Zero : g.NativeGraphics), out isVisible); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return isVisible != 0; } // int version ////// Tests whether the specified ///is contained within this in the specified graphics context. /// /// /// public bool IsVisible(int x, int y, Graphics g) { return IsVisible(new Point(x, y), g); } ////// Tests whether the specified point is contained within this ///in the /// specified graphics context. /// /// /// public bool IsVisible(Point point) { return IsVisible(point, null); } ////// Tests whether the specified ///is contained within this . /// /// /// public bool IsVisible(Point point, Graphics g) { int isVisible = 0; int status = SafeNativeMethods.Gdip.GdipIsVisibleRegionPointI(new HandleRef(this, nativeRegion), point.X, point.Y, new HandleRef(g, (g == null) ? IntPtr.Zero : g.NativeGraphics), out isVisible); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return isVisible != 0; } ////// Tests whether the specified ///is contained within this /// in the specified /// graphics context. /// /// /// public bool IsVisible(int x, int y, int width, int height) { return IsVisible(new Rectangle(x, y, width, height), null); } ////// Tests whether the specified rectangle is contained within this ////// . /// /// /// public bool IsVisible(Rectangle rect) { return IsVisible(rect, null); } ////// Tests whether the specified ///is contained within this /// . /// /// /// public bool IsVisible(int x, int y, int width, int height, Graphics g) { return IsVisible(new Rectangle(x, y, width, height), g); } ////// Tests whether the specified rectangle is contained within this ///in the /// specified graphics context. /// /// /// public bool IsVisible(Rectangle rect, Graphics g) { int isVisible = 0; int status = SafeNativeMethods.Gdip.GdipIsVisibleRegionRectI(new HandleRef(this, nativeRegion), rect.X, rect.Y, rect.Width, rect.Height, new HandleRef(g, (g == null) ? IntPtr.Zero : g.NativeGraphics), out isVisible); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return isVisible != 0; } ////// Tests whether the specified ///is contained within this /// /// in the specified graphics context. /// /// /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] public RectangleF[] GetRegionScans(Matrix matrix) { if (matrix == null) throw new ArgumentNullException("matrix"); int count = 0; // call first time to get actual count of rectangles int status = SafeNativeMethods.Gdip.GdipGetRegionScansCount(new HandleRef(this, nativeRegion), out count, new HandleRef(matrix, matrix.nativeMatrix)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); RectangleF[] rectangles; int rectsize = (int)Marshal.SizeOf(typeof(GPRECTF)); IntPtr memoryRects = Marshal.AllocHGlobal(rectsize*count); try { status = SafeNativeMethods.Gdip.GdipGetRegionScans(new HandleRef(this, nativeRegion), memoryRects, out count, new HandleRef(matrix, matrix.nativeMatrix)); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } int index; GPRECTF gprectf = new GPRECTF(); rectangles = new RectangleF[count]; for (index=0; index/// Returns an array of ////// objects that approximate this Region on the specified ///
Link Menu
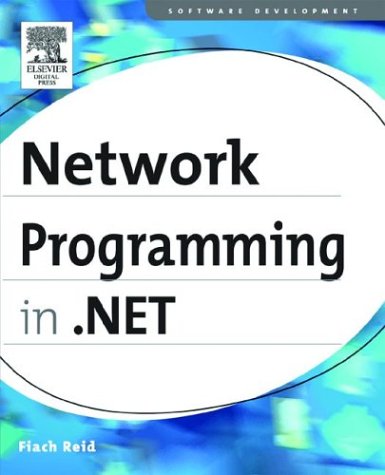
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLString.cs
- TableItemPatternIdentifiers.cs
- ErrorTableItemStyle.cs
- IItemProperties.cs
- IChannel.cs
- Condition.cs
- DataSysAttribute.cs
- Avt.cs
- DataBindingsDialog.cs
- elementinformation.cs
- SystemIPInterfaceStatistics.cs
- TableParagraph.cs
- RelationalExpressions.cs
- SqlParameterizer.cs
- DesignerDataParameter.cs
- SafeHandles.cs
- WebBrowserNavigatedEventHandler.cs
- arc.cs
- PinnedBufferMemoryStream.cs
- XsltOutput.cs
- Rule.cs
- SafeSerializationManager.cs
- RadioButtonBaseAdapter.cs
- PublisherMembershipCondition.cs
- PrintPreviewControl.cs
- ContextMarshalException.cs
- CodeDOMUtility.cs
- UserControlParser.cs
- Point3DCollection.cs
- DataSourceProvider.cs
- InterleavedZipPartStream.cs
- PartitionedStream.cs
- CallSiteOps.cs
- TheQuery.cs
- BitmapEffectCollection.cs
- DataSet.cs
- Vector.cs
- SafeFileMappingHandle.cs
- HMACSHA512.cs
- XmlNamespaceManager.cs
- CheckBoxStandardAdapter.cs
- PageAdapter.cs
- ClientProxyGenerator.cs
- Compilation.cs
- ChangePassword.cs
- SelectManyQueryOperator.cs
- WeakReferenceEnumerator.cs
- DataGridColumn.cs
- SpeechDetectedEventArgs.cs
- WebPartConnectionsCancelVerb.cs
- SecurityRuntime.cs
- ThumbAutomationPeer.cs
- HttpTransportElement.cs
- SchemaElement.cs
- HttpDictionary.cs
- ParserStreamGeometryContext.cs
- Panel.cs
- CharacterBufferReference.cs
- KeyBinding.cs
- TemplatePartAttribute.cs
- DynamicDocumentPaginator.cs
- TransformerConfigurationWizardBase.cs
- SyntaxCheck.cs
- SqlNodeAnnotation.cs
- BaseResourcesBuildProvider.cs
- URLIdentityPermission.cs
- StyleReferenceConverter.cs
- GeometryGroup.cs
- XmlIlGenerator.cs
- ResourcesBuildProvider.cs
- codemethodreferenceexpression.cs
- ReturnEventArgs.cs
- RequestTimeoutManager.cs
- ListArgumentProvider.cs
- ScriptRegistrationManager.cs
- ToolStripManager.cs
- MenuScrollingVisibilityConverter.cs
- SQLInt64Storage.cs
- WebCodeGenerator.cs
- CustomErrorsSectionWrapper.cs
- ExceptionHelpers.cs
- FilterException.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- ValidatingPropertiesEventArgs.cs
- EventlogProvider.cs
- HtmlTableCellCollection.cs
- TripleDES.cs
- WindowHideOrCloseTracker.cs
- WebPartZoneBase.cs
- objectquery_tresulttype.cs
- GridViewUpdateEventArgs.cs
- RegexCapture.cs
- Publisher.cs
- GrowingArray.cs
- HttpFileCollection.cs
- ExtensionSimplifierMarkupObject.cs
- MultipleViewProviderWrapper.cs
- ByteConverter.cs
- ConfigurationManagerHelper.cs
- ToolStripDropDownClosedEventArgs.cs