Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / SupportingTokenSecurityTokenResolver.cs / 1 / SupportingTokenSecurityTokenResolver.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Defines an implementation of SecurityTokenResolver that provides per-message resolution // of SCTs for incoming supporting tokens using System; using System.Collections.ObjectModel; using System.Diagnostics; using System.IdentityModel.Selectors; using System.IdentityModel.Tokens; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Security.Tokens; using System.ServiceModel.Transactions; using System.Xml; namespace Microsoft.Transactions.Wsat.Messaging { class SupportingTokenSecurityTokenResolver : SecurityTokenResolver, ISecurityContextSecurityTokenCache { // Note - this works because we understand our fixed binding set and we know we // won't switch threads between the following two steps in receiving a message: // - Our SupportingTokenXXXChannel element being called // - The SecurityChannel calling ISecurityContextSecurityTokenCache.GetContext [ThreadStatic] static SecurityContextSecurityToken currentSct; public bool FaultInSupportingToken(Message message) { DebugTrace.TraceEnter(this, "FaultInSupportingToken"); bool found = false; WsatRegistrationHeader header = ReadRegistrationHeader(message); if (header == null || header.TransactionId == Guid.Empty || string.IsNullOrEmpty(header.TokenId)) { if (DebugTrace.Warning) DebugTrace.Trace(TraceLevel.Warning, "Invalid or absent RegisterInfo in register message"); } else { currentSct = DeriveToken(header.TransactionId, header.TokenId); found = true; if (DebugTrace.Verbose) DebugTrace.Trace(TraceLevel.Verbose, "Created SCT with id {0} for transaction {1}", header.TokenId, header.TransactionId); } DebugTrace.TraceLeave(this, "FaultInSupportingToken"); return found; } WsatRegistrationHeader ReadRegistrationHeader(Message message) { WsatRegistrationHeader header = null; try { header = WsatRegistrationHeader.ReadFrom(message); } catch (InvalidEnlistmentHeaderException e) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Error); if (DebugTrace.Error) DebugTrace.Trace(TraceLevel.Error, "Invalid RegisterInfo header found in register message: {0}", e.Message); } return header; } SecurityContextSecurityToken DeriveToken(Guid transactionId, string tokenId) { byte[] derivedKey = CoordinationServiceSecurity.DeriveIssuedTokenKey(transactionId, tokenId); DateTime now = DateTime.UtcNow; return new SecurityContextSecurityToken(new UniqueId(tokenId), derivedKey, now, now + TimeSpan.FromMinutes(5)); } // // SecurityTokenResolver overrides // protected override bool TryResolveTokenCore(SecurityKeyIdentifier keyIdentifier, out SecurityToken token) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } protected override bool TryResolveTokenCore(SecurityKeyIdentifierClause keyIdentifierClause, out SecurityToken token) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } protected override bool TryResolveSecurityKeyCore(SecurityKeyIdentifierClause keyIdentifierClause, out SecurityKey key) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } // // ISecurityContextSecurityTokenCache implementation public void AddContext(SecurityContextSecurityToken token) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public void ClearContexts() { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public CollectionGetAllContexts(UniqueId contextId) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public SecurityContextSecurityToken GetContext(UniqueId contextId, UniqueId generation) { DebugTrace.TraceEnter(this, "GetContext"); SecurityContextSecurityToken sct = null; if (currentSct != null && currentSct.ContextId == contextId && currentSct.KeyGeneration == generation) { if (DebugTrace.Verbose) DebugTrace.Trace(TraceLevel.Verbose, "Found SCT with matching id {0}", contextId); sct = currentSct; currentSct = null; } DebugTrace.TraceLeave(this, "GetContext"); return sct; } public void RemoveAllContexts(UniqueId contextId) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public void RemoveContext(UniqueId contextId, UniqueId generation) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public bool TryAddContext(SecurityContextSecurityToken token) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public void UpdateContextCachingTime(SecurityContextSecurityToken context, DateTime expirationTime) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
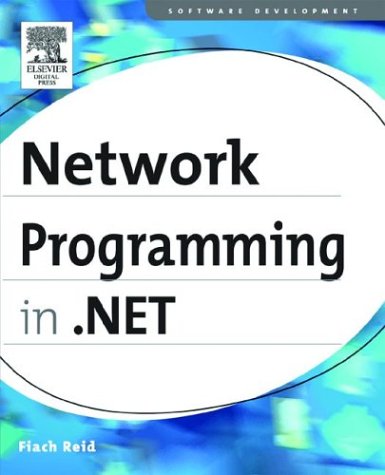
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DocumentSignatureManager.cs
- CapiSymmetricAlgorithm.cs
- AnnotationResource.cs
- FrameworkPropertyMetadata.cs
- RegistrationServices.cs
- ChildTable.cs
- FontWeightConverter.cs
- XslException.cs
- LineBreakRecord.cs
- XmlTextEncoder.cs
- Nodes.cs
- PolicyLevel.cs
- FolderBrowserDialog.cs
- SystemColorTracker.cs
- AssemblyHash.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- ListSortDescription.cs
- CodeCommentStatement.cs
- PageCache.cs
- SimpleBitVector32.cs
- FloaterBaseParagraph.cs
- PathSegment.cs
- TextBox.cs
- AuthorizationRuleCollection.cs
- XmlILCommand.cs
- DictionarySectionHandler.cs
- XmlConvert.cs
- WebProxyScriptElement.cs
- QilDataSource.cs
- ProgressiveCrcCalculatingStream.cs
- ProcessHostConfigUtils.cs
- EndpointAddress10.cs
- TextFormatterHost.cs
- StylusEditingBehavior.cs
- EnumerableRowCollectionExtensions.cs
- ServiceChannelProxy.cs
- RemotingException.cs
- SoapFormatter.cs
- ExtendedPropertyCollection.cs
- TabControl.cs
- HasCopySemanticsAttribute.cs
- DataServices.cs
- ParseNumbers.cs
- InputScopeNameConverter.cs
- StrongNamePublicKeyBlob.cs
- HttpPostProtocolReflector.cs
- StructuralType.cs
- ApplicationActivator.cs
- ItemAutomationPeer.cs
- ExpressionBuilderContext.cs
- ToolStripSettings.cs
- LineProperties.cs
- BuildProviderUtils.cs
- _SslSessionsCache.cs
- AudioFileOut.cs
- SamlAssertionDirectKeyIdentifierClause.cs
- SymmetricAlgorithm.cs
- TextChange.cs
- DropShadowBitmapEffect.cs
- HMACSHA1.cs
- SqlBulkCopyColumnMapping.cs
- DynamicActivityProperty.cs
- HttpCachePolicyElement.cs
- ExtenderControl.cs
- SimplePropertyEntry.cs
- EmptyQuery.cs
- JoinElimination.cs
- ContextMenuStripGroupCollection.cs
- PageBreakRecord.cs
- ISAPIRuntime.cs
- XPathMultyIterator.cs
- VisualStyleElement.cs
- Oid.cs
- Journal.cs
- ConditionCollection.cs
- X509SecurityTokenParameters.cs
- RotateTransform3D.cs
- DecimalConstantAttribute.cs
- RSAPKCS1SignatureDeformatter.cs
- WindowsFormsHelpers.cs
- InlineObject.cs
- WebServiceResponseDesigner.cs
- VectorCollection.cs
- KeyTimeConverter.cs
- DropDownButton.cs
- ListInitExpression.cs
- PartialCachingAttribute.cs
- ImageConverter.cs
- TableItemPattern.cs
- XmlAnyAttributeAttribute.cs
- ExpandableObjectConverter.cs
- RegistryKey.cs
- ArraySubsetEnumerator.cs
- Range.cs
- pingexception.cs
- ImagingCache.cs
- DataGridViewButtonColumn.cs
- ScopelessEnumAttribute.cs
- LinearGradientBrush.cs
- DbMetaDataColumnNames.cs