Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / Ink / ExtendedPropertyCollection.cs / 1 / ExtendedPropertyCollection.cs
using MS.Utility; using System; using System.ComponentModel; using System.Collections; using System.Collections.Generic; using System.IO; using System.Runtime.CompilerServices; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters.Binary; using MS.Internal.Ink.InkSerializedFormat; using System.Windows.Media; using System.Reflection; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Ink { ////// A collection of name/value pairs, called ExtendedProperties, can be stored /// in a collection to enable aggregate operations and assignment to Ink object /// model objects, such StrokeCollection and Stroke. /// internal sealed class ExtendedPropertyCollection //does not implement ICollection, we don't need it { ////// Create a new empty ExtendedPropertyCollection /// internal ExtendedPropertyCollection() { } ///Overload of the Equals method which determines if two ExtendedPropertyCollection /// objects contain equivalent key/value pairs public override bool Equals(object o) { if (o == null || o.GetType() != GetType()) { return false; } // // compare counts // ExtendedPropertyCollection that = (ExtendedPropertyCollection)o; if (that.Count != this.Count) { return false; } // // counts are equal, compare individual items // // for (int x = 0; x < that.Count; x++) { bool cont = false; for (int i = 0; i < _extendedProperties.Count; i++) { if (_extendedProperties[i].Equals(that[x])) { cont = true; break; } } if (!cont) { return false; } } return true; } ///Overload of the equality operator which determines /// if two ExtendedPropertyCollections are equal public static bool operator ==(ExtendedPropertyCollection first, ExtendedPropertyCollection second) { // compare the GC ptrs for the obvious reference equality if (((object)first == null && (object)second == null) || ((object)first == (object)second)) { return true; } // otherwise, if one of the ptrs are null, but not the other then return false else if ((object)first == null || (object)second == null) { return false; } // finally use the full `blown value-style comparison against the collection contents else { return first.Equals(second); } } ///Overload of the not equals operator to determine if two /// ExtendedPropertyCollections have different key/value pairs public static bool operator!=(ExtendedPropertyCollection first, ExtendedPropertyCollection second) { return !(first == second); } ////// GetHashCode /// public override int GetHashCode() { return base.GetHashCode(); } ////// Check to see if the attribute is defined in the collection. /// /// Attribute identifier ///True if attribute is set in the mask, false otherwise internal bool Contains(Guid attributeId) { for (int x = 0; x < _extendedProperties.Count; x++) { if (_extendedProperties[x].Id == attributeId) { // // a typical pattern is to first check if // ep.Contains(guid) // before accessing: // object o = ep[guid]; // // I'm caching the index that contains returns so that we // can look there first for the guid in the indexer // _optimisticIndex = x; return true; } } return false; } ////// Copies the ExtendedPropertyCollection /// ///Copy of the ExtendedPropertyCollection ///Any reference types held in the collection will only be deep copied (e.g. Arrays). /// internal ExtendedPropertyCollection Clone() { ExtendedPropertyCollection copied = new ExtendedPropertyCollection(); for (int x = 0; x < _extendedProperties.Count; x++) { copied.Add(_extendedProperties[x].Clone()); } return copied; } ////// Add /// /// Id /// value internal void Add(Guid id, object value) { if (this.Contains(id)) { throw new ArgumentException(SR.Get(SRID.EPExists), "id"); } ExtendedProperty extendedProperty = new ExtendedProperty(id, value); //this will raise change events this.Add(extendedProperty); } ////// Remove /// /// id internal void Remove(Guid id) { if (!Contains(id)) { throw new ArgumentException(SR.Get(SRID.EPGuidNotFound), "id"); } ExtendedProperty propertyToRemove = GetExtendedPropertyById(id); System.Diagnostics.Debug.Assert(propertyToRemove != null); _extendedProperties.Remove(propertyToRemove); // // this value is bogus now // _optimisticIndex = -1; // fire notification event if (this.Changed != null) { ExtendedPropertiesChangedEventArgs eventArgs = new ExtendedPropertiesChangedEventArgs(propertyToRemove, null); this.Changed(this, eventArgs); } } ////// Retrieve the Guid array of ExtendedProperty Ids in the collection. /// internal Guid[] GetGuidArray() { if (_extendedProperties.Count > 0) { Guid[] guids = new Guid[_extendedProperties.Count]; for (int i = 0; i < _extendedProperties.Count; i++) { guids[i] = this[i].Id; } return guids; } else { return new Guid[0]; } } ///Guid[] is of type. /// /// /// Generic accessor for the ExtendedPropertyCollection. /// /// Attribue Id to find ///Value for attribute specified by Id ///Specified identifier was not found ////// Note that you can access extended properties via this indexer. /// internal object this[Guid attributeId] { get { ExtendedProperty ep = GetExtendedPropertyById(attributeId); if (ep == null) { throw new ArgumentException(SR.Get(SRID.EPNotFound), "attributeId"); } return ep.Value; } set { if (value == null) { throw new ArgumentNullException("value"); } for (int i = 0; i < _extendedProperties.Count; i++) { ExtendedProperty currentProperty = _extendedProperties[i]; if (currentProperty.Id == attributeId) { object oldValue = currentProperty.Value; //this will raise events currentProperty.Value = value; //raise change if anyone is listening if (this.Changed != null) { ExtendedPropertiesChangedEventArgs eventArgs = new ExtendedPropertiesChangedEventArgs( new ExtendedProperty(currentProperty.Id, oldValue), //old prop currentProperty); //new prop this.Changed(this, eventArgs); } return; } } // // we didn't find the Id in the collection, we need to add it. // this will raise change notifications // ExtendedProperty attributeToAdd = new ExtendedProperty(attributeId, value); this.Add(attributeToAdd); } } ////// Generic accessor for the ExtendedPropertyCollection. /// /// index into masking collection to retrieve ///ExtendedProperty specified at index ///Index was not found ////// Note that you can access extended properties via this indexer. /// internal ExtendedProperty this[int index] { get { return _extendedProperties[index]; } } ////// Retrieve the number of ExtendedProperty objects in the collection. /// internal int Count { get { return _extendedProperties.Count; } } ///Count is of type. /// /// /// Event fired whenever a ExtendedProperty is modified in the collection /// internal event ExtendedPropertiesChangedEventHandler Changed; ////// private Add, we need to consider making this public in order to implement the generic ICollection /// private void Add(ExtendedProperty extendedProperty) { System.Diagnostics.Debug.Assert(!this.Contains(extendedProperty.Id), "ExtendedProperty already belongs to the collection"); _extendedProperties.Add(extendedProperty); // fire notification event if (this.Changed != null) { ExtendedPropertiesChangedEventArgs eventArgs = new ExtendedPropertiesChangedEventArgs(null, extendedProperty); this.Changed(this, eventArgs); } } ////// Private helper for getting an EP out of our internal collection /// /// id private ExtendedProperty GetExtendedPropertyById(Guid id) { // // a typical pattern is to first check if // ep.Contains(guid) // before accessing: // object o = ep[guid]; // // The last call to .Contains sets this index // if (_optimisticIndex != -1 && _optimisticIndex < _extendedProperties.Count && _extendedProperties[_optimisticIndex].Id == id) { return _extendedProperties[_optimisticIndex]; } //we didn't find the ep optimistically, perform linear lookup for (int i = 0; i < _extendedProperties.Count; i++) { if (_extendedProperties[i].Id == id) { return _extendedProperties[i]; } } return null; } // the set of ExtendedProperties stored in this collection private List_extendedProperties = new List (); //used to optimize across Contains / Index calls private int _optimisticIndex = -1; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using MS.Utility; using System; using System.ComponentModel; using System.Collections; using System.Collections.Generic; using System.IO; using System.Runtime.CompilerServices; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters.Binary; using MS.Internal.Ink.InkSerializedFormat; using System.Windows.Media; using System.Reflection; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Ink { /// /// A collection of name/value pairs, called ExtendedProperties, can be stored /// in a collection to enable aggregate operations and assignment to Ink object /// model objects, such StrokeCollection and Stroke. /// internal sealed class ExtendedPropertyCollection //does not implement ICollection, we don't need it { ////// Create a new empty ExtendedPropertyCollection /// internal ExtendedPropertyCollection() { } ///Overload of the Equals method which determines if two ExtendedPropertyCollection /// objects contain equivalent key/value pairs public override bool Equals(object o) { if (o == null || o.GetType() != GetType()) { return false; } // // compare counts // ExtendedPropertyCollection that = (ExtendedPropertyCollection)o; if (that.Count != this.Count) { return false; } // // counts are equal, compare individual items // // for (int x = 0; x < that.Count; x++) { bool cont = false; for (int i = 0; i < _extendedProperties.Count; i++) { if (_extendedProperties[i].Equals(that[x])) { cont = true; break; } } if (!cont) { return false; } } return true; } ///Overload of the equality operator which determines /// if two ExtendedPropertyCollections are equal public static bool operator ==(ExtendedPropertyCollection first, ExtendedPropertyCollection second) { // compare the GC ptrs for the obvious reference equality if (((object)first == null && (object)second == null) || ((object)first == (object)second)) { return true; } // otherwise, if one of the ptrs are null, but not the other then return false else if ((object)first == null || (object)second == null) { return false; } // finally use the full `blown value-style comparison against the collection contents else { return first.Equals(second); } } ///Overload of the not equals operator to determine if two /// ExtendedPropertyCollections have different key/value pairs public static bool operator!=(ExtendedPropertyCollection first, ExtendedPropertyCollection second) { return !(first == second); } ////// GetHashCode /// public override int GetHashCode() { return base.GetHashCode(); } ////// Check to see if the attribute is defined in the collection. /// /// Attribute identifier ///True if attribute is set in the mask, false otherwise internal bool Contains(Guid attributeId) { for (int x = 0; x < _extendedProperties.Count; x++) { if (_extendedProperties[x].Id == attributeId) { // // a typical pattern is to first check if // ep.Contains(guid) // before accessing: // object o = ep[guid]; // // I'm caching the index that contains returns so that we // can look there first for the guid in the indexer // _optimisticIndex = x; return true; } } return false; } ////// Copies the ExtendedPropertyCollection /// ///Copy of the ExtendedPropertyCollection ///Any reference types held in the collection will only be deep copied (e.g. Arrays). /// internal ExtendedPropertyCollection Clone() { ExtendedPropertyCollection copied = new ExtendedPropertyCollection(); for (int x = 0; x < _extendedProperties.Count; x++) { copied.Add(_extendedProperties[x].Clone()); } return copied; } ////// Add /// /// Id /// value internal void Add(Guid id, object value) { if (this.Contains(id)) { throw new ArgumentException(SR.Get(SRID.EPExists), "id"); } ExtendedProperty extendedProperty = new ExtendedProperty(id, value); //this will raise change events this.Add(extendedProperty); } ////// Remove /// /// id internal void Remove(Guid id) { if (!Contains(id)) { throw new ArgumentException(SR.Get(SRID.EPGuidNotFound), "id"); } ExtendedProperty propertyToRemove = GetExtendedPropertyById(id); System.Diagnostics.Debug.Assert(propertyToRemove != null); _extendedProperties.Remove(propertyToRemove); // // this value is bogus now // _optimisticIndex = -1; // fire notification event if (this.Changed != null) { ExtendedPropertiesChangedEventArgs eventArgs = new ExtendedPropertiesChangedEventArgs(propertyToRemove, null); this.Changed(this, eventArgs); } } ////// Retrieve the Guid array of ExtendedProperty Ids in the collection. /// internal Guid[] GetGuidArray() { if (_extendedProperties.Count > 0) { Guid[] guids = new Guid[_extendedProperties.Count]; for (int i = 0; i < _extendedProperties.Count; i++) { guids[i] = this[i].Id; } return guids; } else { return new Guid[0]; } } ///Guid[] is of type. /// /// /// Generic accessor for the ExtendedPropertyCollection. /// /// Attribue Id to find ///Value for attribute specified by Id ///Specified identifier was not found ////// Note that you can access extended properties via this indexer. /// internal object this[Guid attributeId] { get { ExtendedProperty ep = GetExtendedPropertyById(attributeId); if (ep == null) { throw new ArgumentException(SR.Get(SRID.EPNotFound), "attributeId"); } return ep.Value; } set { if (value == null) { throw new ArgumentNullException("value"); } for (int i = 0; i < _extendedProperties.Count; i++) { ExtendedProperty currentProperty = _extendedProperties[i]; if (currentProperty.Id == attributeId) { object oldValue = currentProperty.Value; //this will raise events currentProperty.Value = value; //raise change if anyone is listening if (this.Changed != null) { ExtendedPropertiesChangedEventArgs eventArgs = new ExtendedPropertiesChangedEventArgs( new ExtendedProperty(currentProperty.Id, oldValue), //old prop currentProperty); //new prop this.Changed(this, eventArgs); } return; } } // // we didn't find the Id in the collection, we need to add it. // this will raise change notifications // ExtendedProperty attributeToAdd = new ExtendedProperty(attributeId, value); this.Add(attributeToAdd); } } ////// Generic accessor for the ExtendedPropertyCollection. /// /// index into masking collection to retrieve ///ExtendedProperty specified at index ///Index was not found ////// Note that you can access extended properties via this indexer. /// internal ExtendedProperty this[int index] { get { return _extendedProperties[index]; } } ////// Retrieve the number of ExtendedProperty objects in the collection. /// internal int Count { get { return _extendedProperties.Count; } } ///Count is of type. /// /// /// Event fired whenever a ExtendedProperty is modified in the collection /// internal event ExtendedPropertiesChangedEventHandler Changed; ////// private Add, we need to consider making this public in order to implement the generic ICollection /// private void Add(ExtendedProperty extendedProperty) { System.Diagnostics.Debug.Assert(!this.Contains(extendedProperty.Id), "ExtendedProperty already belongs to the collection"); _extendedProperties.Add(extendedProperty); // fire notification event if (this.Changed != null) { ExtendedPropertiesChangedEventArgs eventArgs = new ExtendedPropertiesChangedEventArgs(null, extendedProperty); this.Changed(this, eventArgs); } } ////// Private helper for getting an EP out of our internal collection /// /// id private ExtendedProperty GetExtendedPropertyById(Guid id) { // // a typical pattern is to first check if // ep.Contains(guid) // before accessing: // object o = ep[guid]; // // The last call to .Contains sets this index // if (_optimisticIndex != -1 && _optimisticIndex < _extendedProperties.Count && _extendedProperties[_optimisticIndex].Id == id) { return _extendedProperties[_optimisticIndex]; } //we didn't find the ep optimistically, perform linear lookup for (int i = 0; i < _extendedProperties.Count; i++) { if (_extendedProperties[i].Id == id) { return _extendedProperties[i]; } } return null; } // the set of ExtendedProperties stored in this collection private List_extendedProperties = new List (); //used to optimize across Contains / Index calls private int _optimisticIndex = -1; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
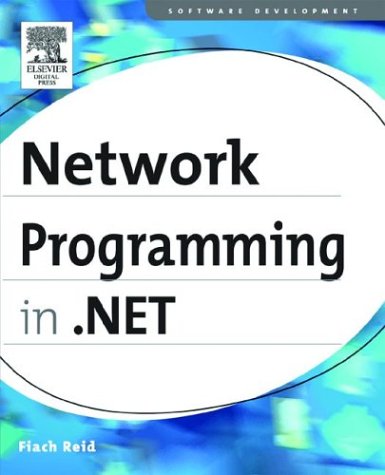
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartEditorCancelVerb.cs
- WebPartTransformer.cs
- FrameworkTextComposition.cs
- ConnectionInterfaceCollection.cs
- Transform.cs
- HeaderedContentControl.cs
- InstanceNormalEvent.cs
- MsmqElementBase.cs
- NotSupportedException.cs
- UnmanagedBitmapWrapper.cs
- ConfigurationStrings.cs
- Signature.cs
- AudienceUriMode.cs
- XsdCachingReader.cs
- DataGridTextBox.cs
- ClientFormsAuthenticationCredentials.cs
- mediapermission.cs
- OdbcConnectionOpen.cs
- Rotation3D.cs
- TableItemStyle.cs
- DetailsViewAutoFormat.cs
- DateTimeFormatInfoScanner.cs
- RoutedEventConverter.cs
- TdsRecordBufferSetter.cs
- CodePageUtils.cs
- OrderPreservingSpoolingTask.cs
- DataGridAutoFormatDialog.cs
- CatalogPartDesigner.cs
- SqlConnectionPoolGroupProviderInfo.cs
- IndicFontClient.cs
- DataGridClipboardHelper.cs
- MultiByteCodec.cs
- XPathEmptyIterator.cs
- DependencyPropertyAttribute.cs
- UIElement.cs
- HitTestDrawingContextWalker.cs
- ActiveXHost.cs
- XmlFormatWriterGenerator.cs
- ZipFileInfoCollection.cs
- ErrorRuntimeConfig.cs
- ObjectSet.cs
- CmsInterop.cs
- CreateParams.cs
- FlowNode.cs
- TypeAccessException.cs
- ValidationPropertyAttribute.cs
- BuildResult.cs
- CrossContextChannel.cs
- UnsafeNativeMethods.cs
- ReferentialConstraint.cs
- ParameterCollection.cs
- Stacktrace.cs
- DelegateArgumentReference.cs
- DBSqlParserTable.cs
- Mouse.cs
- Help.cs
- IgnoreSection.cs
- BuildResultCache.cs
- XmlNavigatorStack.cs
- ObjectResult.cs
- CodeMethodInvokeExpression.cs
- CodeTypeDelegate.cs
- CommonRemoteMemoryBlock.cs
- XamlTypeWithExplicitNamespace.cs
- Positioning.cs
- HttpCookie.cs
- ResourceIDHelper.cs
- TagMapCollection.cs
- MenuRendererStandards.cs
- GZipUtils.cs
- DashStyle.cs
- GCHandleCookieTable.cs
- ToolStripPanel.cs
- ContainerAction.cs
- DataGridBoolColumn.cs
- GraphicsContext.cs
- TransformerTypeCollection.cs
- ListSourceHelper.cs
- OracleCommand.cs
- XmlUtil.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- SerializationException.cs
- RedirectionProxy.cs
- ConfigurationValue.cs
- SerializationException.cs
- XmlSchemaType.cs
- SqlXml.cs
- SchemaNotation.cs
- DisplayMemberTemplateSelector.cs
- TableItemStyle.cs
- Win32Interop.cs
- ParameterCollection.cs
- MutexSecurity.cs
- SmtpReplyReaderFactory.cs
- HtmlGenericControl.cs
- LoginName.cs
- Rotation3D.cs
- ProxyHwnd.cs
- Perspective.cs
- LineProperties.cs