Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / DataOracleClient / System / Data / Common / DBSqlParserTable.cs / 1 / DBSqlParserTable.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Collections; using System.Diagnostics; using System.Text; //--------------------------------------------------------------------- // DbSqlParserTable // // A parsed table reference from DbSqlParser. // sealed internal class DbSqlParserTable { private string _databaseName; private string _schemaName; private string _tableName; private string _correlationName; private DbSqlParserColumnCollection _columns; internal DbSqlParserTable(string databaseName, string schemaName, string tableName, string correlationName) { _databaseName = databaseName; _schemaName = schemaName; _tableName = tableName; _correlationName = correlationName; } internal DbSqlParserColumnCollection Columns { get { if (null == _columns) { _columns = new DbSqlParserColumnCollection(); } return _columns; } set { if (null == value) { throw new ArgumentNullException("value"); } if (!typeof(DbSqlParserColumnCollection).IsInstanceOfType(value)) { throw new InvalidCastException("value"); } _columns = value; } } internal string CorrelationName { get { return (null == _correlationName)?string.Empty : _correlationName; } } internal string DatabaseName { get { return (null == _databaseName) ? string.Empty : _databaseName; } } internal string SchemaName { get { return (null == _schemaName) ? string.Empty : _schemaName; } } internal string TableName { get { return (null == _tableName) ? string.Empty : _tableName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Collections; using System.Diagnostics; using System.Text; //--------------------------------------------------------------------- // DbSqlParserTable // // A parsed table reference from DbSqlParser. // sealed internal class DbSqlParserTable { private string _databaseName; private string _schemaName; private string _tableName; private string _correlationName; private DbSqlParserColumnCollection _columns; internal DbSqlParserTable(string databaseName, string schemaName, string tableName, string correlationName) { _databaseName = databaseName; _schemaName = schemaName; _tableName = tableName; _correlationName = correlationName; } internal DbSqlParserColumnCollection Columns { get { if (null == _columns) { _columns = new DbSqlParserColumnCollection(); } return _columns; } set { if (null == value) { throw new ArgumentNullException("value"); } if (!typeof(DbSqlParserColumnCollection).IsInstanceOfType(value)) { throw new InvalidCastException("value"); } _columns = value; } } internal string CorrelationName { get { return (null == _correlationName)?string.Empty : _correlationName; } } internal string DatabaseName { get { return (null == _databaseName) ? string.Empty : _databaseName; } } internal string SchemaName { get { return (null == _schemaName) ? string.Empty : _schemaName; } } internal string TableName { get { return (null == _tableName) ? string.Empty : _tableName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
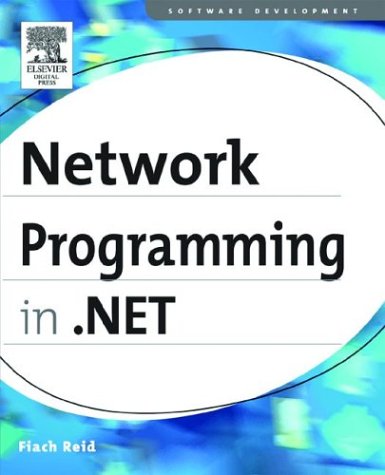
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MorphHelper.cs
- ChangeProcessor.cs
- WizardStepBase.cs
- DoubleAnimationClockResource.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- SerializationInfo.cs
- ObjectPersistData.cs
- HtmlInputButton.cs
- WpfGeneratedKnownProperties.cs
- DataGridItemCollection.cs
- ScaleTransform3D.cs
- WorkflowInvoker.cs
- CloseSequence.cs
- StateItem.cs
- DateTimeParse.cs
- PolygonHotSpot.cs
- HyperlinkAutomationPeer.cs
- Timeline.cs
- Gdiplus.cs
- FusionWrap.cs
- MenuItemStyleCollectionEditor.cs
- SettingsSection.cs
- FontFamilyIdentifier.cs
- WCFBuildProvider.cs
- InputManager.cs
- NameValueSectionHandler.cs
- HttpDictionary.cs
- _HeaderInfoTable.cs
- DataSourceExpression.cs
- ResXResourceSet.cs
- RectIndependentAnimationStorage.cs
- Page.cs
- HtmlForm.cs
- XmlExpressionDumper.cs
- ListViewAutomationPeer.cs
- ValidatingCollection.cs
- ConfigXmlSignificantWhitespace.cs
- SectionXmlInfo.cs
- QilScopedVisitor.cs
- MetadataCacheItem.cs
- ContainerControl.cs
- EncoderExceptionFallback.cs
- RSAPKCS1SignatureDeformatter.cs
- SectionVisual.cs
- ConnectionPoolManager.cs
- ObjectStateManagerMetadata.cs
- ZipIOCentralDirectoryFileHeader.cs
- ModelTreeEnumerator.cs
- GridViewCommandEventArgs.cs
- BinaryFormatterWriter.cs
- Marshal.cs
- DataMember.cs
- SqlHelper.cs
- ImmComposition.cs
- TdsValueSetter.cs
- SByteConverter.cs
- UnsafeNativeMethods.cs
- RuntimeConfigLKG.cs
- SelectionEditingBehavior.cs
- SignedXml.cs
- EventsTab.cs
- WsatServiceAddress.cs
- ErrorRuntimeConfig.cs
- ImageBrush.cs
- Graphics.cs
- Int64AnimationBase.cs
- FacetDescriptionElement.cs
- ImpersonationContext.cs
- CodeSnippetExpression.cs
- ManipulationDelta.cs
- TypeDescriptionProvider.cs
- ListenDesigner.cs
- RegionIterator.cs
- Point3D.cs
- ErrorHandler.cs
- GeneralTransform3DTo2DTo3D.cs
- RegexMatchCollection.cs
- WebPartCancelEventArgs.cs
- InstanceDataCollection.cs
- BlurEffect.cs
- HttpsHostedTransportConfiguration.cs
- InputProviderSite.cs
- ReplacementText.cs
- PointCollection.cs
- X509ClientCertificateAuthentication.cs
- MachineKeySection.cs
- XmlParserContext.cs
- ViewRendering.cs
- CollectionViewGroupRoot.cs
- BaseInfoTable.cs
- FixedSOMPageConstructor.cs
- DynamicValueConverter.cs
- UInt32Converter.cs
- PointConverter.cs
- XmlCharacterData.cs
- RadioButtonAutomationPeer.cs
- MethodBody.cs
- CodeCatchClauseCollection.cs
- IdnMapping.cs
- BaseValidator.cs