Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / FlowNode.cs / 1 / FlowNode.cs
//---------------------------------------------------------------------------- //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // Description: // FlowNode represents a structural node for a flow document. They // are inserted into a content flow backing store content flow their // relative orders signal the content flow order. // // History: // 11/03/2003 - Zhenbin Xu (ZhenbinX) - Created. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Diagnostics; //===================================================================== ////// FlowNode represents a structural node in the Flow Order of a fixed document. /// They are inserted into a content flow backing store (the Flow Order) to /// represent a flow order view of the fixed document. Their relative position /// in the Flow Order indicates their relative reading order. /// /// A FlowNode is identified by its ScopeId and is compared by its relative position /// in the Flow Order (Fp). /// /// Content structure and scope can be deduced from the Flow Order. /// For instance /// S1 S2 R2 E2 E1 /// would indicate element 1 (S1-E1) is the parent of element 2 (S1-E2) /// internal sealed class FlowNode : IComparable { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors internal FlowNode(int scopeId, FlowNodeType type, object cookie) { // // If cookie is FixedElement, you have to set it later, otherwise, // please set it here. // In that case, cookie will be set as a FixedElement later. // It cann't be set here because of the circular reference. // // // We don't allow to create a glyph node with zero run length. // it will lots of problem in textOM due to some assumption there. // Debug.Assert( (type != FlowNodeType.Run) || ((int)cookie != 0)); _scopeId = scopeId; _type = type; _cookie = cookie; } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods // force object comparision public static bool IsNull(FlowNode flow) { return (object)flow == null; } ////// ///int - hash code public override int GetHashCode() { return _scopeId.GetHashCode()^_fp.GetHashCode(); } ////// Compares this FlowNode with the passed in object /// /// The object to compare to "this" ///bool - true if the FlowNodes are equal, false otherwise public override bool Equals(object o) { if (o == null || this.GetType() != o.GetType()) { return false; } FlowNode fn = (FlowNode)o; Debug.Assert(_fp != fn._fp || (_fp == fn._fp) && (_scopeId == fn._scopeId)); return (this._fp == fn._fp); } ////// Compare the flow order of this FlowNode to another FlowNode /// /// FlowNode to compare to ///-1, 0, 1 public int CompareTo(object o) { if (o == null) { throw new ArgumentNullException("o"); } FlowNode fp = o as FlowNode; if (fp == null) { throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, o.GetType(), typeof(FlowNode)), "o"); } if (Object.ReferenceEquals(this, fp)) { return 0; } int fd = this._fp - fp._fp; if (fd == 0) { return 0; } else if (fd < 0) { return -1; } else { return 1; } } #if DEBUG ////// Create a string representation of this object /// ///string - A string representation of this object public override string ToString() { int page = -1; switch (_type) { case FlowNodeType.Boundary: case FlowNodeType.Start: case FlowNodeType.End: case FlowNodeType.Object: { FixedElement element = _cookie as FixedElement; if (element != null) { page = element.PageIndex; } break; } case FlowNodeType.Virtual: case FlowNodeType.Noop: { page = (int) _cookie; break; } case FlowNodeType.Run: { if (this.FixedSOMElements != null && this.FixedSOMElements.Length > 0) { page = this.FixedSOMElements[0].FixedNode.Page; } break; } default: break; } return String.Format("Pg{0}-nCp{1}-Id{2}-Tp{3}", page, _fp, _scopeId, System.Enum.GetName(typeof(FlowNodeType), _type)); } #endif #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- //-------------------------------------------------------------------- // // Public Events // //--------------------------------------------------------------------- //------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods // // Note: Make these explicit method so that it won't be used mistakenly // Only FixedTextBuilder can modify Fp // internal void SetFp(int fp) { _fp = fp; } internal void IncreaseFp() { _fp++; } internal void DecreaseFp() { _fp--; } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //--------------------------------------------------------------------- #region Internal Properties // Get/Set position within flow order internal int Fp { get { return _fp; } } // Get the scope to which this node belongs internal int ScopeId { get { return _scopeId; } } // Get the Flag associated with this node internal FlowNodeType Type { get { return _type; } } // Get/Set the cookie associated with this position. // Higher level protocol decides what to put inside // the cookie internal object Cookie { get { return _cookie; } } internal FixedSOMElement[] FixedSOMElements { get { return _elements; } set { _elements = value; } } internal void AttachElement(FixedElement fixedElement) { _cookie = fixedElement; } #endregion Internal Properties //-------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields private readonly int _scopeId; // Node Identifier private readonly FlowNodeType _type; // type of the node private int _fp; // Position in flow private object _cookie; // Associated object private FixedSOMElement[] _elements; // Used for mapping between fixed and flow representations #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // Description: // FlowNode represents a structural node for a flow document. They // are inserted into a content flow backing store content flow their // relative orders signal the content flow order. // // History: // 11/03/2003 - Zhenbin Xu (ZhenbinX) - Created. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Diagnostics; //===================================================================== ////// FlowNode represents a structural node in the Flow Order of a fixed document. /// They are inserted into a content flow backing store (the Flow Order) to /// represent a flow order view of the fixed document. Their relative position /// in the Flow Order indicates their relative reading order. /// /// A FlowNode is identified by its ScopeId and is compared by its relative position /// in the Flow Order (Fp). /// /// Content structure and scope can be deduced from the Flow Order. /// For instance /// S1 S2 R2 E2 E1 /// would indicate element 1 (S1-E1) is the parent of element 2 (S1-E2) /// internal sealed class FlowNode : IComparable { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors internal FlowNode(int scopeId, FlowNodeType type, object cookie) { // // If cookie is FixedElement, you have to set it later, otherwise, // please set it here. // In that case, cookie will be set as a FixedElement later. // It cann't be set here because of the circular reference. // // // We don't allow to create a glyph node with zero run length. // it will lots of problem in textOM due to some assumption there. // Debug.Assert( (type != FlowNodeType.Run) || ((int)cookie != 0)); _scopeId = scopeId; _type = type; _cookie = cookie; } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods // force object comparision public static bool IsNull(FlowNode flow) { return (object)flow == null; } ////// ///int - hash code public override int GetHashCode() { return _scopeId.GetHashCode()^_fp.GetHashCode(); } ////// Compares this FlowNode with the passed in object /// /// The object to compare to "this" ///bool - true if the FlowNodes are equal, false otherwise public override bool Equals(object o) { if (o == null || this.GetType() != o.GetType()) { return false; } FlowNode fn = (FlowNode)o; Debug.Assert(_fp != fn._fp || (_fp == fn._fp) && (_scopeId == fn._scopeId)); return (this._fp == fn._fp); } ////// Compare the flow order of this FlowNode to another FlowNode /// /// FlowNode to compare to ///-1, 0, 1 public int CompareTo(object o) { if (o == null) { throw new ArgumentNullException("o"); } FlowNode fp = o as FlowNode; if (fp == null) { throw new ArgumentException(SR.Get(SRID.UnexpectedParameterType, o.GetType(), typeof(FlowNode)), "o"); } if (Object.ReferenceEquals(this, fp)) { return 0; } int fd = this._fp - fp._fp; if (fd == 0) { return 0; } else if (fd < 0) { return -1; } else { return 1; } } #if DEBUG ////// Create a string representation of this object /// ///string - A string representation of this object public override string ToString() { int page = -1; switch (_type) { case FlowNodeType.Boundary: case FlowNodeType.Start: case FlowNodeType.End: case FlowNodeType.Object: { FixedElement element = _cookie as FixedElement; if (element != null) { page = element.PageIndex; } break; } case FlowNodeType.Virtual: case FlowNodeType.Noop: { page = (int) _cookie; break; } case FlowNodeType.Run: { if (this.FixedSOMElements != null && this.FixedSOMElements.Length > 0) { page = this.FixedSOMElements[0].FixedNode.Page; } break; } default: break; } return String.Format("Pg{0}-nCp{1}-Id{2}-Tp{3}", page, _fp, _scopeId, System.Enum.GetName(typeof(FlowNodeType), _type)); } #endif #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- //-------------------------------------------------------------------- // // Public Events // //--------------------------------------------------------------------- //------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods // // Note: Make these explicit method so that it won't be used mistakenly // Only FixedTextBuilder can modify Fp // internal void SetFp(int fp) { _fp = fp; } internal void IncreaseFp() { _fp++; } internal void DecreaseFp() { _fp--; } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //--------------------------------------------------------------------- #region Internal Properties // Get/Set position within flow order internal int Fp { get { return _fp; } } // Get the scope to which this node belongs internal int ScopeId { get { return _scopeId; } } // Get the Flag associated with this node internal FlowNodeType Type { get { return _type; } } // Get/Set the cookie associated with this position. // Higher level protocol decides what to put inside // the cookie internal object Cookie { get { return _cookie; } } internal FixedSOMElement[] FixedSOMElements { get { return _elements; } set { _elements = value; } } internal void AttachElement(FixedElement fixedElement) { _cookie = fixedElement; } #endregion Internal Properties //-------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields private readonly int _scopeId; // Node Identifier private readonly FlowNodeType _type; // type of the node private int _fp; // Position in flow private object _cookie; // Associated object private FixedSOMElement[] _elements; // Used for mapping between fixed and flow representations #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
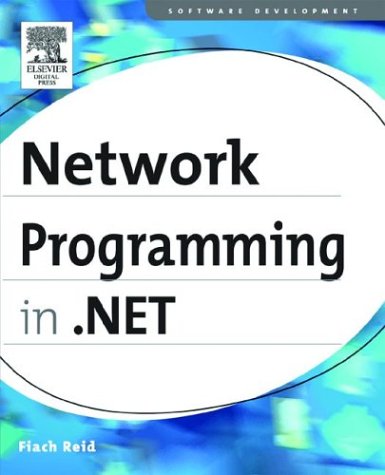
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeViewItemAutomationPeer.cs
- DoubleSumAggregationOperator.cs
- Utils.cs
- RegexNode.cs
- _SslStream.cs
- EntryIndex.cs
- LocatorPartList.cs
- AnimationLayer.cs
- ServiceSettingsResponseInfo.cs
- IndexedEnumerable.cs
- EllipseGeometry.cs
- URLBuilder.cs
- HtmlInputControl.cs
- BuildProvidersCompiler.cs
- PerformanceCountersBase.cs
- SQLGuidStorage.cs
- CorrelationService.cs
- ToolboxControl.cs
- ConstantSlot.cs
- HitTestParameters3D.cs
- PeerCollaboration.cs
- MemoryMappedView.cs
- StoragePropertyMapping.cs
- Component.cs
- ServiceDefaults.cs
- HandlerFactoryCache.cs
- ImagingCache.cs
- StrokeCollection.cs
- ItemList.cs
- UserPersonalizationStateInfo.cs
- panel.cs
- OleDbDataReader.cs
- SerialPort.cs
- ExtractedStateEntry.cs
- Expression.cs
- WindowsListViewItem.cs
- MailBnfHelper.cs
- CodeGenerator.cs
- GregorianCalendar.cs
- XsltQilFactory.cs
- BaseParaClient.cs
- CharAnimationBase.cs
- ViewEvent.cs
- BinaryUtilClasses.cs
- PersianCalendar.cs
- FontStretches.cs
- LogicalMethodInfo.cs
- RadioButtonList.cs
- RequestBringIntoViewEventArgs.cs
- DropDownList.cs
- GridItemPattern.cs
- Base64Encoder.cs
- TypeLibConverter.cs
- WebUtil.cs
- CommandHelper.cs
- SQLInt64.cs
- HttpCookiesSection.cs
- TypeLoadException.cs
- MarshalByRefObject.cs
- XmlSchemaAttributeGroup.cs
- SqlProvider.cs
- ContextInformation.cs
- SerializationEventsCache.cs
- AdPostCacheSubstitution.cs
- MarginCollapsingState.cs
- PaintValueEventArgs.cs
- _HeaderInfo.cs
- _StreamFramer.cs
- StreamGeometryContext.cs
- RequestQueryParser.cs
- Compiler.cs
- UICuesEvent.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- DisableDpiAwarenessAttribute.cs
- SqlProviderManifest.cs
- ISAPIWorkerRequest.cs
- CultureSpecificCharacterBufferRange.cs
- PhysicalOps.cs
- AuthenticationService.cs
- NativeDirectoryServicesQueryAPIs.cs
- XmlEntityReference.cs
- exports.cs
- ScrollableControl.cs
- GreenMethods.cs
- CodeDefaultValueExpression.cs
- ScopelessEnumAttribute.cs
- NetMsmqSecurityElement.cs
- MSHTMLHost.cs
- HttpClientChannel.cs
- RuntimeArgumentHandle.cs
- SharedStatics.cs
- ExtensibleClassFactory.cs
- XPathNavigatorReader.cs
- CallSiteOps.cs
- EnvelopedPkcs7.cs
- ProcessThread.cs
- RuleDefinitions.cs
- BreakRecordTable.cs
- ICspAsymmetricAlgorithm.cs
- User.cs