Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Activities / Rules / RuleDefinitions.cs / 1305376 / RuleDefinitions.cs
// ---------------------------------------------------------------------------- // Copyright (C) 2006 Microsoft Corporation All Rights Reserved // --------------------------------------------------------------------------- #define CODE_ANALYSIS using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Workflow.ComponentModel; namespace System.Workflow.Activities.Rules { #region class RuleDefinitions public sealed class RuleDefinitions : IWorkflowChangeDiff { [SuppressMessage("Microsoft.Security", "CA2104:DoNotDeclareReadOnlyMutableReferenceTypes")] public static readonly DependencyProperty RuleDefinitionsProperty = DependencyProperty.RegisterAttached("RuleDefinitions", typeof(RuleDefinitions), typeof(RuleDefinitions), new PropertyMetadata(null, DependencyPropertyOptions.Metadata, new GetValueOverride(OnGetRuleConditions), null, new Attribute[] { new DesignerSerializationVisibilityAttribute(DesignerSerializationVisibility.Hidden) })); private RuleConditionCollection conditions; private RuleSetCollection ruleSets; private bool runtimeInitialized; [NonSerialized] private object syncLock = new object(); [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public RuleConditionCollection Conditions { get { if (this.conditions == null) this.conditions = new RuleConditionCollection(); return conditions; } } [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public RuleSetCollection RuleSets { get { if (this.ruleSets == null) this.ruleSets = new RuleSetCollection(); return this.ruleSets; } } internal static object OnGetRuleConditions(DependencyObject dependencyObject) { if (dependencyObject == null) throw new ArgumentNullException("dependencyObject"); RuleDefinitions rules = dependencyObject.GetValueBase(RuleDefinitions.RuleDefinitionsProperty) as RuleDefinitions; if (rules != null) return rules; Activity rootActivity = dependencyObject as Activity; if (rootActivity.Parent == null) { rules = ConditionHelper.GetRuleDefinitionsFromManifest(rootActivity.GetType()); if (rules != null) dependencyObject.SetValue(RuleDefinitions.RuleDefinitionsProperty, rules); } return rules; } internal void OnRuntimeInitialized() { lock (syncLock) { if (runtimeInitialized) return; Conditions.OnRuntimeInitialized(); RuleSets.OnRuntimeInitialized(); runtimeInitialized = true; } } #region IWorkflowChangeDiff Members public IListDiff(object originalDefinition, object changedDefinition) { RuleDefinitions originalRules = originalDefinition as RuleDefinitions; RuleDefinitions changedRules = changedDefinition as RuleDefinitions; if ((originalRules == null) || (changedRules == null)) return new List (); IList cdiff = Conditions.Diff(originalRules.Conditions, changedRules.Conditions); IList rdiff = RuleSets.Diff(originalRules.RuleSets, changedRules.RuleSets); // quick optimization -- if no condition changes, simply return the ruleset changes if (cdiff.Count == 0) return rdiff; // merge ruleset changes into condition changes for (int i = 0; i < rdiff.Count; ++i) { cdiff.Add(rdiff[i]); } return cdiff; } #endregion internal RuleDefinitions Clone() { RuleDefinitions newRuleDefinitions = new RuleDefinitions(); if (this.ruleSets != null) { newRuleDefinitions.ruleSets = new RuleSetCollection(); foreach (RuleSet r in this.ruleSets) newRuleDefinitions.ruleSets.Add(r.Clone()); } if (this.conditions != null) { newRuleDefinitions.conditions = new RuleConditionCollection(); foreach (RuleCondition r in this.conditions) newRuleDefinitions.conditions.Add(r.Clone()); } return newRuleDefinitions; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
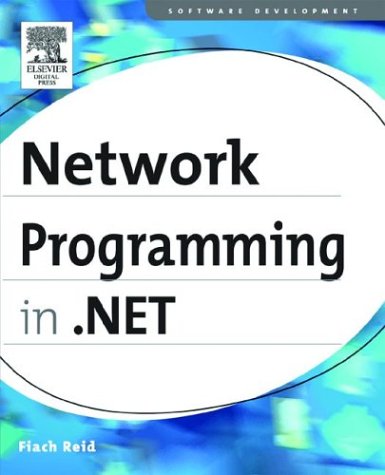
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedTextSelectionProcessor.cs
- SmtpReplyReaderFactory.cs
- WsdlExporter.cs
- NumberSubstitution.cs
- TemplateBindingExtension.cs
- WSDualHttpBindingElement.cs
- DiscardableAttribute.cs
- DocumentsTrace.cs
- SystemGatewayIPAddressInformation.cs
- PageEventArgs.cs
- ClientSponsor.cs
- TimeSpanConverter.cs
- ScriptingProfileServiceSection.cs
- FastPropertyAccessor.cs
- DesignerLoader.cs
- X509Certificate2Collection.cs
- NumberFormatter.cs
- WsdlImporterElementCollection.cs
- StructuralType.cs
- ContentPresenter.cs
- OleDbConnectionFactory.cs
- DesignTimeParseData.cs
- PlatformCulture.cs
- MexBindingBindingCollectionElement.cs
- Int32.cs
- EncryptedHeaderXml.cs
- TagPrefixCollection.cs
- UrlMappingsModule.cs
- GenericWebPart.cs
- HierarchicalDataBoundControl.cs
- ColumnResizeAdorner.cs
- ActiveXContainer.cs
- FormView.cs
- RegisteredHiddenField.cs
- Perspective.cs
- CompModSwitches.cs
- HttpHandlersInstallComponent.cs
- PackageDigitalSignature.cs
- DataGridViewButtonColumn.cs
- URI.cs
- CqlParser.cs
- Graphics.cs
- MenuBindingsEditorForm.cs
- SurrogateChar.cs
- DirectionalLight.cs
- Ref.cs
- MouseEventArgs.cs
- BeginStoryboard.cs
- XmlSchemaChoice.cs
- NativeMethodsOther.cs
- FixedMaxHeap.cs
- KeyPullup.cs
- SecurityElement.cs
- CodeSnippetCompileUnit.cs
- StringFreezingAttribute.cs
- NativeActivity.cs
- EdgeModeValidation.cs
- SamlAuthenticationClaimResource.cs
- DbExpressionVisitor.cs
- Animatable.cs
- CodeTypeDeclarationCollection.cs
- CalendarButtonAutomationPeer.cs
- SimpleWorkerRequest.cs
- TriggerAction.cs
- ServiceSecurityContext.cs
- CodeGen.cs
- GroupByExpressionRewriter.cs
- OverrideMode.cs
- SoapFaultCodes.cs
- DataTableNewRowEvent.cs
- CharEnumerator.cs
- StatusBarPanelClickEvent.cs
- SslStream.cs
- PermissionSetEnumerator.cs
- ListViewAutomationPeer.cs
- WhiteSpaceTrimStringConverter.cs
- ListMarkerSourceInfo.cs
- QueryAsyncResult.cs
- BlobPersonalizationState.cs
- TypeDescriptor.cs
- ImmComposition.cs
- TemplatePagerField.cs
- TagMapCollection.cs
- SecurityResources.cs
- EventDescriptorCollection.cs
- InvalidOleVariantTypeException.cs
- TextDecoration.cs
- InstanceNormalEvent.cs
- FontNamesConverter.cs
- ToolBarPanel.cs
- SiteMapNodeCollection.cs
- ServiceModelSecurityTokenRequirement.cs
- ListBindableAttribute.cs
- SocketAddress.cs
- Publisher.cs
- AssemblyName.cs
- MethodCallTranslator.cs
- WorkflowViewStateService.cs
- PeerDuplexChannel.cs
- GestureRecognitionResult.cs