Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / MouseEventArgs.cs / 1305600 / MouseEventArgs.cs
using System.Collections; using System; namespace System.Windows.Input { ////// The MouseEventArgs class provides access to the logical /// Mouse device for all derived event args. /// public class MouseEventArgs : InputEventArgs { ////// Initializes a new instance of the MouseEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// public MouseEventArgs(MouseDevice mouse, int timestamp) : base(mouse, timestamp) { if( mouse == null ) { throw new System.ArgumentNullException("mouse"); } _stylusDevice = null; } ////// Initializes a new instance of the MouseEventArgs class. /// /// /// The logical Mouse device associated with this event. /// /// /// The time when the input occured. /// /// /// The stylus device that was involved with this event. /// public MouseEventArgs(MouseDevice mouse, int timestamp, StylusDevice stylusDevice) : base(mouse, timestamp) { if( mouse == null ) { throw new System.ArgumentNullException("mouse"); } _stylusDevice = stylusDevice; } ////// Read-only access to the mouse device associated with this /// event. /// public MouseDevice MouseDevice { get {return (MouseDevice) this.Device;} } ////// Read-only access to the stylus Mouse associated with this event. /// public StylusDevice StylusDevice { get {return _stylusDevice;} } ////// Calculates the position of the mouse relative to /// a particular element. /// public Point GetPosition(IInputElement relativeTo) { return this.MouseDevice.GetPosition(relativeTo); } ////// The state of the left button. /// public MouseButtonState LeftButton { get { return this.MouseDevice.LeftButton; } } ////// The state of the right button. /// public MouseButtonState RightButton { get { return this.MouseDevice.RightButton; } } ////// The state of the middle button. /// public MouseButtonState MiddleButton { get { return this.MouseDevice.MiddleButton; } } ////// The state of the first extended button. /// public MouseButtonState XButton1 { get { return this.MouseDevice.XButton1; } } ////// The state of the second extended button. /// public MouseButtonState XButton2 { get { return this.MouseDevice.XButton2; } } ////// The mechanism used to call the type-specific handler on the /// target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { MouseEventHandler handler = (MouseEventHandler) genericHandler; handler(genericTarget, this); } private StylusDevice _stylusDevice; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
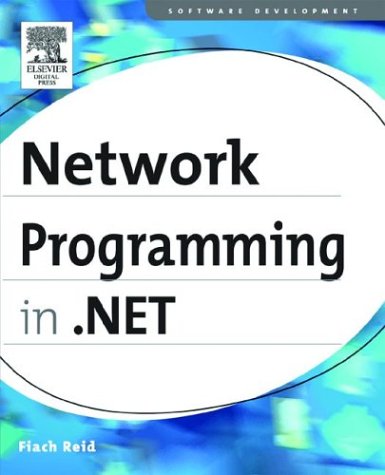
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewHitTestInfo.cs
- CommonObjectSecurity.cs
- PeerHelpers.cs
- NonBatchDirectoryCompiler.cs
- Maps.cs
- ThreadStateException.cs
- TextTreeInsertUndoUnit.cs
- ContainerFilterService.cs
- ProtocolImporter.cs
- UIHelper.cs
- ListViewTableCell.cs
- WindowsContainer.cs
- InkCanvasSelection.cs
- SqlCacheDependencySection.cs
- InputElement.cs
- DataGridViewToolTip.cs
- HostedTransportConfigurationBase.cs
- ResourceWriter.cs
- InstanceLockQueryResult.cs
- DefaultHttpHandler.cs
- FrameAutomationPeer.cs
- SoapProcessingBehavior.cs
- ButtonChrome.cs
- ProjectionCamera.cs
- ClonableStack.cs
- EpmAttributeNameBuilder.cs
- MultiView.cs
- SvcMapFileSerializer.cs
- AddInStore.cs
- InkCanvasSelectionAdorner.cs
- HttpHandlersSection.cs
- ReadOnlyObservableCollection.cs
- EraserBehavior.cs
- SignedXml.cs
- HtmlControlPersistable.cs
- DBDataPermission.cs
- CombinedHttpChannel.cs
- RequestCache.cs
- SchemaImporter.cs
- TreeViewImageIndexConverter.cs
- ObjectListFieldCollection.cs
- FreezableDefaultValueFactory.cs
- __ComObject.cs
- TypeForwardedToAttribute.cs
- DrawListViewColumnHeaderEventArgs.cs
- ColumnHeader.cs
- BuildProviderCollection.cs
- TreeNodeCollection.cs
- NamespaceQuery.cs
- ReflectionServiceProvider.cs
- ToolStripDropDownClosingEventArgs.cs
- VirtualDirectoryMappingCollection.cs
- StringUtil.cs
- DoubleCollectionValueSerializer.cs
- MimeFormReflector.cs
- MenuTracker.cs
- ShaperBuffers.cs
- GeometryDrawing.cs
- CodeSnippetCompileUnit.cs
- CustomSignedXml.cs
- ObjectHandle.cs
- PersonalizationStateInfoCollection.cs
- MetadataArtifactLoaderCompositeResource.cs
- CommandID.cs
- IIS7UserPrincipal.cs
- ItemContainerGenerator.cs
- BitmapEffectGeneralTransform.cs
- MailAddress.cs
- ListControl.cs
- IOException.cs
- TargetInvocationException.cs
- WebRequestModulesSection.cs
- DoubleIndependentAnimationStorage.cs
- AccessDataSourceDesigner.cs
- Timer.cs
- BamlStream.cs
- ControlCachePolicy.cs
- BulletedListEventArgs.cs
- DropShadowBitmapEffect.cs
- Directory.cs
- QilGenerator.cs
- ForEachAction.cs
- RuntimeIdentifierPropertyAttribute.cs
- ContentDesigner.cs
- Rfc2898DeriveBytes.cs
- SortQuery.cs
- HttpConfigurationSystem.cs
- ContentValidator.cs
- Paragraph.cs
- ReadOnlyPropertyMetadata.cs
- JoinSymbol.cs
- Subtree.cs
- ProcessInputEventArgs.cs
- GZipObjectSerializer.cs
- ObjectTag.cs
- DragAssistanceManager.cs
- RequestQueryParser.cs
- LoginView.cs
- WmlObjectListAdapter.cs
- RecognitionEventArgs.cs