Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / LinearGradientBrush.cs / 1 / LinearGradientBrush.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: This file contains the implementation of LinearGradientBrush. // The LinearGradientBrush is a GradientBrush which defines its // Gradient as a linear interpolation between two parallel lines. // // History: // 05/07/2003 : [....] - Created it. // 09/21/2004 : [....] - Added GradientStopCollection constructors. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Runtime.InteropServices; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// LinearGradientBrush - This GradientBrush defines its Gradient as an interpolation /// between two parallel lines. /// public sealed partial class LinearGradientBrush : GradientBrush { #region Constructors ////// Default constructor for LinearGradientBrush. The resulting brush has no content. /// public LinearGradientBrush() : base() { } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops specified at offset 0.0 and /// 1.0. The StartPoint is set to (0,0) and the EndPoint is derived from the angle /// such that 1) the line containing the StartPoint and EndPoint is 'angle' degrees /// from the horizontal in the direction of positive Y, and 2) the EndPoint lies on /// the perimeter of the unit circle. /// /// The Color at offset 0.0. /// The Color at offset 1.0. /// The angle, in degrees, that the gradient will be away from horizontal. public LinearGradientBrush(Color startColor, Color endColor, double angle) : base() { EndPoint = EndPointFromAngle(angle); GradientStops.Add(new GradientStop(startColor, 0.0)); GradientStops.Add(new GradientStop(endColor, 1.0)); } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with two colors at the specified start and end points. /// /// The Color at offset 0.0. /// The Color at offset 1.0. /// The start point /// The end point public LinearGradientBrush(Color startColor, Color endColor, Point startPoint, Point endPoint) : base() { StartPoint = startPoint; EndPoint = endPoint; GradientStops.Add(new GradientStop(startColor, 0.0)); GradientStops.Add(new GradientStop(endColor, 1.0)); } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops set to the passed-in collection. /// /// GradientStopCollection to set on this brush. public LinearGradientBrush(GradientStopCollection gradientStopCollection) : base (gradientStopCollection) { } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops set to the passed-in collection. /// Constructs a LinearGradientBrush with GradientStops specified at offset 0.0 and /// 1.0. The StartPoint is set to (0,0) and the EndPoint is derived from the angle /// such that 1) the line containing the StartPoint and EndPoint is 'angle' degrees /// from the horizontal in the direction of positive Y, and 2) the EndPoint lies on /// the perimeter of the unit circle. /// /// GradientStopCollection to set on this brush. /// The angle, in degrees, that the gradient will be away from horizontal. public LinearGradientBrush(GradientStopCollection gradientStopCollection, double angle) : base (gradientStopCollection) { EndPoint = EndPointFromAngle(angle); } ////// LinearGradientBrush Constructor /// Constructs a LinearGradientBrush with GradientStops set to the passed-in collection. /// The StartPoint and EndPoint are set to the specified startPoint and endPoint. /// /// GradientStopCollection to set on this brush. /// The start point /// The end point public LinearGradientBrush(GradientStopCollection gradientStopCollection, Point startPoint, Point endPoint) : base (gradientStopCollection) { StartPoint = startPoint; EndPoint = endPoint; } #endregion Constructors ////// Critical: This code accesses unsafe code blocks /// TreatAsSafe: This code does is safe to call but needs to be verified for correctness /// [SecurityCritical, SecurityTreatAsSafe] private void ManualUpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { Transform vTransform = Transform; Transform vRelativeTransform = RelativeTransform; GradientStopCollection vGradientStops = GradientStops; DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } DUCE.ResourceHandle hRelativeTransform; if (vRelativeTransform == null || Object.ReferenceEquals(vRelativeTransform, Transform.Identity) ) { hRelativeTransform = DUCE.ResourceHandle.Null; } else { hRelativeTransform = ((DUCE.IResource)vRelativeTransform).GetHandle(channel); } DUCE.ResourceHandle hOpacityAnimations = GetAnimationResourceHandle(OpacityProperty, channel); DUCE.ResourceHandle hStartPointAnimations = GetAnimationResourceHandle(StartPointProperty, channel); DUCE.ResourceHandle hEndPointAnimations = GetAnimationResourceHandle(EndPointProperty, channel); unsafe { DUCE.MILCMD_LINEARGRADIENTBRUSH data; data.Type = MILCMD.MilCmdLinearGradientBrush; data.Handle = _duceResource.GetHandle(channel); double tempOpacity = Opacity; DUCE.CopyBytes((byte*)&data.Opacity, (byte*)&tempOpacity, 8); data.hOpacityAnimations = hOpacityAnimations; data.hTransform = hTransform; data.hRelativeTransform = hRelativeTransform; data.ColorInterpolationMode = ColorInterpolationMode; data.MappingMode = MappingMode; data.SpreadMethod = SpreadMethod; Point tempStartPoint = StartPoint; DUCE.CopyBytes((byte*)&data.StartPoint, (byte*)&tempStartPoint, 16); data.hStartPointAnimations = hStartPointAnimations; Point tempEndPoint = EndPoint; DUCE.CopyBytes((byte*)&data.EndPoint, (byte*)&tempEndPoint, 16); data.hEndPointAnimations = hEndPointAnimations; // int count = (vGradientStops == null) ? 0 : vGradientStops.Count; data.GradientStopsSize = (UInt32)(sizeof(DUCE.MIL_GRADIENTSTOP)*count); channel.BeginCommand( (byte*)&data, sizeof(DUCE.MILCMD_LINEARGRADIENTBRUSH), sizeof(DUCE.MIL_GRADIENTSTOP)*count ); for (int i=0; i
Link Menu
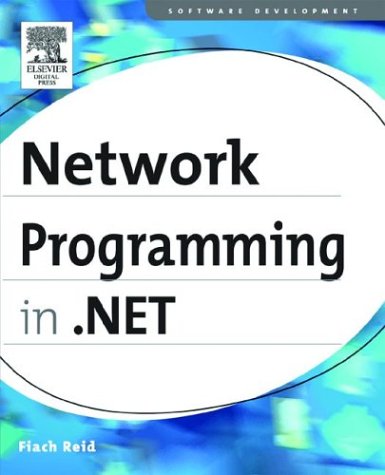
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- __TransparentProxy.cs
- StylusPlugin.cs
- ObjectSecurity.cs
- VirtualDirectoryMappingCollection.cs
- Soap.cs
- _BaseOverlappedAsyncResult.cs
- IMembershipProvider.cs
- CheckBoxRenderer.cs
- ExternalException.cs
- SocketAddress.cs
- LinkGrep.cs
- CommandHelper.cs
- PriorityItem.cs
- RankException.cs
- HttpPostedFile.cs
- TimelineClockCollection.cs
- BezierSegment.cs
- BitmapDecoder.cs
- IOThreadTimer.cs
- FilterQueryOptionExpression.cs
- MouseGestureValueSerializer.cs
- DataGridViewComboBoxColumn.cs
- MarkupExtensionSerializer.cs
- TreeViewImageKeyConverter.cs
- PowerModeChangedEventArgs.cs
- InputReport.cs
- QuaternionAnimation.cs
- InvokeGenerator.cs
- NullableDecimalMinMaxAggregationOperator.cs
- SystemIPv4InterfaceProperties.cs
- FeatureAttribute.cs
- InputManager.cs
- DeferredTextReference.cs
- RelationshipSet.cs
- FlagsAttribute.cs
- SqlConnectionHelper.cs
- DataRecordObjectView.cs
- documentsequencetextpointer.cs
- MsmqInputChannel.cs
- ClientConvert.cs
- SystemResourceKey.cs
- MetadataUtil.cs
- WinHttpWebProxyFinder.cs
- PropertyItemInternal.cs
- FacetEnabledSchemaElement.cs
- FieldNameLookup.cs
- AddressUtility.cs
- ProjectionPathBuilder.cs
- ModelFunctionTypeElement.cs
- RealProxy.cs
- TouchesOverProperty.cs
- ExceptionHelpers.cs
- WaitHandle.cs
- DataGridViewAdvancedBorderStyle.cs
- PointKeyFrameCollection.cs
- BitArray.cs
- XmlSchemaComplexType.cs
- ViewCellSlot.cs
- InstanceView.cs
- DesignerCategoryAttribute.cs
- SqlServer2KCompatibilityAnnotation.cs
- Pointer.cs
- NameValueConfigurationCollection.cs
- AspNetHostingPermission.cs
- TypeNameConverter.cs
- GPPOINT.cs
- LambdaCompiler.Lambda.cs
- ExtenderControl.cs
- x509utils.cs
- WebPartDisplayModeCancelEventArgs.cs
- SQLSingle.cs
- XmlTextAttribute.cs
- Grant.cs
- TextInfo.cs
- BindingCompleteEventArgs.cs
- LinqDataSourceStatusEventArgs.cs
- CaretElement.cs
- SqlResolver.cs
- WebPartConnectionsCloseVerb.cs
- CommandValueSerializer.cs
- FormatterServices.cs
- PrintDialog.cs
- OleDbTransaction.cs
- ConfigLoader.cs
- ReliabilityContractAttribute.cs
- DataGridViewCellStateChangedEventArgs.cs
- DbConnectionPoolOptions.cs
- SoapObjectReader.cs
- FormViewUpdatedEventArgs.cs
- SQLDouble.cs
- MachineSettingsSection.cs
- ClaimSet.cs
- BehaviorEditorPart.cs
- SecurityState.cs
- CompiledQuery.cs
- DataControlFieldCollection.cs
- XmlEventCache.cs
- GeometryDrawing.cs
- ErrorTableItemStyle.cs
- XhtmlBasicValidationSummaryAdapter.cs