Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DLinq / Dlinq / CompiledQuery.cs / 1 / CompiledQuery.cs
using System; using System.Collections; using System.Collections.Generic; using System.Configuration; using System.Data; using System.Data.Common; using System.Linq.Expressions; using System.Globalization; using System.IO; using System.Linq; using System.Reflection; using System.Text; using System.Xml; using System.Transactions; using System.Data.Linq.Provider; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq { public sealed class CompiledQuery { LambdaExpression query; ICompiledQuery compiled; private CompiledQuery(LambdaExpression query) { this.query = query; } public LambdaExpression Expression { get { return this.query; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static FuncCompile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } private static bool UseExpressionCompile(LambdaExpression query) { return typeof(ITable).IsAssignableFrom(query.Body.Type); } private TResult Invoke (TArg0 arg0) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0}); } private TResult Invoke (TArg0 arg0, TArg1 arg1) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3}); } private object ExecuteQuery(DataContext context, object[] args) { if (context == null) { throw Error.ArgumentNull("context"); } if (this.compiled == null) { lock (this) { if (this.compiled == null) { this.compiled = context.Provider.Compile(this.query); } } } return this.compiled.Execute(context.Provider, args).ReturnValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Configuration; using System.Data; using System.Data.Common; using System.Linq.Expressions; using System.Globalization; using System.IO; using System.Linq; using System.Reflection; using System.Text; using System.Xml; using System.Transactions; using System.Data.Linq.Provider; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq { public sealed class CompiledQuery { LambdaExpression query; ICompiledQuery compiled; private CompiledQuery(LambdaExpression query) { this.query = query; } public LambdaExpression Expression { get { return this.query; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } [SuppressMessage("Microsoft.Design", "CA1006:DoNotNestGenericTypesInMemberSignatures", Justification = "[....]: Generic types are an important part of Linq APIs and they could not exist without nested generic support.")] public static Func Compile (Expression > query) where TArg0 : DataContext { if (query == null) { Error.ArgumentNull("query"); } if (UseExpressionCompile(query)) { return query.Compile(); } else { return new CompiledQuery(query).Invoke ; } } private static bool UseExpressionCompile(LambdaExpression query) { return typeof(ITable).IsAssignableFrom(query.Body.Type); } private TResult Invoke (TArg0 arg0) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0}); } private TResult Invoke (TArg0 arg0, TArg1 arg1) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2}); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3) where TArg0 : DataContext { return (TResult) this.ExecuteQuery(arg0, new object[] {arg0, arg1, arg2, arg3}); } private object ExecuteQuery(DataContext context, object[] args) { if (context == null) { throw Error.ArgumentNull("context"); } if (this.compiled == null) { lock (this) { if (this.compiled == null) { this.compiled = context.Provider.Compile(this.query); } } } return this.compiled.Execute(context.Provider, args).ReturnValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
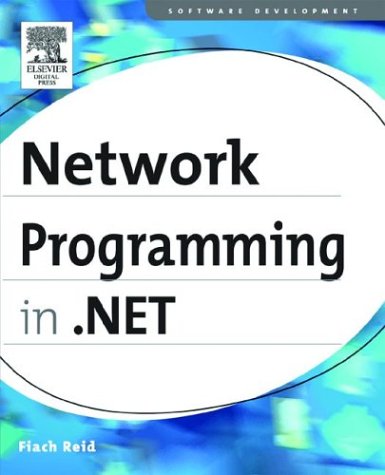
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlCDATASection.cs
- ToolBarButton.cs
- TextLineBreak.cs
- WebPartConnectionsCancelEventArgs.cs
- PolygonHotSpot.cs
- NotSupportedException.cs
- LeftCellWrapper.cs
- BaseTemplateParser.cs
- DbMetaDataCollectionNames.cs
- SingleTagSectionHandler.cs
- Icon.cs
- XmlQueryOutput.cs
- UpdateException.cs
- OptimalBreakSession.cs
- GrammarBuilderWildcard.cs
- UnsafeNativeMethods.cs
- EditorZone.cs
- SslStream.cs
- PageSettings.cs
- ObjectDataSourceMethodEditor.cs
- GeneralTransformGroup.cs
- Clock.cs
- Identity.cs
- Menu.cs
- TableCellCollection.cs
- StrokeCollectionDefaultValueFactory.cs
- HostingEnvironmentWrapper.cs
- BlockUIContainer.cs
- PointCollection.cs
- FrameworkElement.cs
- WeakReferenceList.cs
- HttpRequestTraceRecord.cs
- AccessViolationException.cs
- ListDictionary.cs
- TreeNodeCollectionEditorDialog.cs
- GridErrorDlg.cs
- DataBindingHandlerAttribute.cs
- HexParser.cs
- QuadraticEase.cs
- QueryCursorEventArgs.cs
- WebPartsSection.cs
- XmlBaseReader.cs
- SerializationObjectManager.cs
- MsdtcWrapper.cs
- CodeAssignStatement.cs
- WebPartsPersonalizationAuthorization.cs
- SystemPens.cs
- TextLineResult.cs
- TCEAdapterGenerator.cs
- FileVersion.cs
- TabItem.cs
- SearchForVirtualItemEventArgs.cs
- Int32Collection.cs
- KnownAssemblyEntry.cs
- UrlPath.cs
- CompositeScriptReferenceEventArgs.cs
- BookmarkList.cs
- DesignerObjectListAdapter.cs
- FlowDocumentScrollViewer.cs
- TypeUtil.cs
- IntSecurity.cs
- DataTableMapping.cs
- WindowsListViewGroup.cs
- BreakRecordTable.cs
- TreeIterator.cs
- BamlRecordReader.cs
- OrCondition.cs
- CompletionBookmark.cs
- ContainerFilterService.cs
- PriorityBinding.cs
- ControlCollection.cs
- HyperLinkField.cs
- ContainerParagraph.cs
- NullableFloatSumAggregationOperator.cs
- MetadataUtil.cs
- CheckedPointers.cs
- SqlClientPermission.cs
- GorillaCodec.cs
- DeferredSelectedIndexReference.cs
- WebPartMinimizeVerb.cs
- OrderToken.cs
- bindurihelper.cs
- CodeDOMUtility.cs
- PropertyEmitter.cs
- BamlWriter.cs
- PrinterResolution.cs
- AccessedThroughPropertyAttribute.cs
- FamilyMapCollection.cs
- IISUnsafeMethods.cs
- CollectionType.cs
- EpmSyndicationContentSerializer.cs
- ApplicationInfo.cs
- ComPlusAuthorization.cs
- VisualTreeUtils.cs
- WebControl.cs
- Normalization.cs
- Italic.cs
- MethodCallTranslator.cs
- DockPatternIdentifiers.cs
- ExpressionPrinter.cs