Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / Epm / EpmSyndicationContentSerializer.cs / 1305376 / EpmSyndicationContentSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Classes used for serializing EntityPropertyMappingAttribute content for // syndication specific mappings // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Data.Services.Common; using System.Diagnostics; using System.Xml; ////// Base visitor class for performing serialization of syndication specific content in the feed entry whose mapping /// is provided through EntityPropertyMappingAttributes /// internal sealed class EpmSyndicationContentSerializer : EpmContentSerializerBase, IDisposable { ////// Is author mapping provided in the target tree /// private bool authorInfoPresent; ////// Is updated mapping provided in the target tree /// private bool updatedPresent; ////// Is author name provided in the target tree /// private bool authorNamePresent; ////// Constructor initializes the base class be identifying itself as a syndication serializer /// /// Target tree containing mapping information /// Object to be serialized /// SyndicationItem to which content will be added internal EpmSyndicationContentSerializer(EpmTargetTree tree, object element, XmlWriter target) : base(tree, true, element, target) { } ////// Used for housecleaning once every node has been visited, creates an author and updated syndication elements if none /// have been created by the visitor /// public void Dispose() { this.CreateAuthor(true); this.CreateUpdated(); } ////// Override of the base Visitor method, which actually performs mapping search and serialization /// /// Current segment being checked for mapping /// Which sub segments to serialize protected override void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind) { if (targetSegment.HasContent) { EntityPropertyMappingInfo epmInfo = targetSegment.EpmInfo; Object propertyValue; try { propertyValue = epmInfo.PropValReader.DynamicInvoke(this.Element); } catch (System.Reflection.TargetInvocationException) { throw; } String contentType; ActioncontentWriter; switch (epmInfo.Attribute.TargetTextContentKind) { case SyndicationTextContentKind.Html: contentType = "html"; contentWriter = this.Target.WriteString; break; case SyndicationTextContentKind.Xhtml: contentType = "xhtml"; contentWriter = this.Target.WriteRaw; break; default: contentType = "text"; contentWriter = this.Target.WriteString; break; } Action textSyndicationWriter = (c, nonTextPossible, atomDateConstruct) => { this.Target.WriteStartElement(c, XmlConstants.AtomNamespace); if (nonTextPossible) { this.Target.WriteAttributeString(XmlConstants.AtomTypeAttributeName, String.Empty, contentType); } // As per atomPub spec dateConstructs must contain valid datetime. Therefore we need to fill atom:updated/atom:published // field with something (e.g. DateTimeOffset.MinValue) if the user wants to map null to either of these fields. This will // satisfy protocol requirements and Syndication API. Note that the content will still contain information saying that the // mapped property is supposed to be null - therefore the server will know that the actual value sent by the user is null. // For all other elements we can use empty string since the content is not validated. String textPropertyValue = propertyValue != null ? ClientConvert.ToString(propertyValue, atomDateConstruct) : atomDateConstruct ? ClientConvert.ToString(DateTime.MinValue, atomDateConstruct) : String.Empty; contentWriter(textPropertyValue); this.Target.WriteEndElement(); }; switch (epmInfo.Attribute.TargetSyndicationItem) { case SyndicationItemProperty.AuthorEmail: case SyndicationItemProperty.ContributorEmail: textSyndicationWriter(XmlConstants.AtomEmailElementName, false, false); break; case SyndicationItemProperty.AuthorName: case SyndicationItemProperty.ContributorName: textSyndicationWriter(XmlConstants.AtomNameElementName, false, false); this.authorNamePresent = true; break; case SyndicationItemProperty.AuthorUri: case SyndicationItemProperty.ContributorUri: textSyndicationWriter(XmlConstants.AtomUriElementName, false, false); break; case SyndicationItemProperty.Updated: textSyndicationWriter(XmlConstants.AtomUpdatedElementName, false, true); this.updatedPresent = true; break; case SyndicationItemProperty.Published: textSyndicationWriter(XmlConstants.AtomPublishedElementName, false, true); break; case SyndicationItemProperty.Rights: textSyndicationWriter(XmlConstants.AtomRightsElementName, true, false); break; case SyndicationItemProperty.Summary: textSyndicationWriter(XmlConstants.AtomSummaryElementName, true, false); break; case SyndicationItemProperty.Title: textSyndicationWriter(XmlConstants.AtomTitleElementName, true, false); break; default: Debug.Assert(false, "Unhandled SyndicationItemProperty enum value - should never get here."); break; } } else { if (targetSegment.SegmentName == XmlConstants.AtomAuthorElementName) { this.CreateAuthor(false); base.Serialize(targetSegment, kind); this.FinishAuthor(); } else if (targetSegment.SegmentName == XmlConstants.AtomContributorElementName) { this.Target.WriteStartElement(XmlConstants.AtomContributorElementName, XmlConstants.AtomNamespace); base.Serialize(targetSegment, kind); this.Target.WriteEndElement(); } else { Debug.Assert(false, "Only authors and contributors have nested elements"); } } } /// /// Creates a new author syndication specific object /// /// Whether to create a null author or a non-null author private void CreateAuthor(bool createNull) { if (!this.authorInfoPresent) { if (createNull) { this.Target.WriteStartElement(XmlConstants.AtomAuthorElementName, XmlConstants.AtomNamespace); this.Target.WriteElementString(XmlConstants.AtomNameElementName, XmlConstants.AtomNamespace, String.Empty); this.Target.WriteEndElement(); } else { this.Target.WriteStartElement(XmlConstants.AtomAuthorElementName, XmlConstants.AtomNamespace); } this.authorInfoPresent = true; } } ////// Finish writing the author atom element /// private void FinishAuthor() { Debug.Assert(this.authorInfoPresent == true, "Must have already written the start element for author"); if (this.authorNamePresent == false) { this.Target.WriteElementString(XmlConstants.AtomNameElementName, XmlConstants.AtomNamespace, String.Empty); this.authorNamePresent = true; } this.Target.WriteEndElement(); } ////// Ensures that mandatory atom:update element is creatd. The value of the created element is set to with value set to DateTime.UtcNow. /// This method is used only if there are some EPM mappings for the given resource type but there is no mapping to atom:updated /// element. Otherwise the element will be created during mapping handling or if there is no EPM mapping at all during the serialization. /// private void CreateUpdated() { if (!this.updatedPresent) { this.Target.WriteElementString(XmlConstants.AtomUpdatedElementName, XmlConstants.AtomNamespace, XmlConvert.ToString(DateTime.UtcNow, XmlDateTimeSerializationMode.RoundtripKind)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Classes used for serializing EntityPropertyMappingAttribute content for // syndication specific mappings // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Data.Services.Common; using System.Diagnostics; using System.Xml; ////// Base visitor class for performing serialization of syndication specific content in the feed entry whose mapping /// is provided through EntityPropertyMappingAttributes /// internal sealed class EpmSyndicationContentSerializer : EpmContentSerializerBase, IDisposable { ////// Is author mapping provided in the target tree /// private bool authorInfoPresent; ////// Is updated mapping provided in the target tree /// private bool updatedPresent; ////// Is author name provided in the target tree /// private bool authorNamePresent; ////// Constructor initializes the base class be identifying itself as a syndication serializer /// /// Target tree containing mapping information /// Object to be serialized /// SyndicationItem to which content will be added internal EpmSyndicationContentSerializer(EpmTargetTree tree, object element, XmlWriter target) : base(tree, true, element, target) { } ////// Used for housecleaning once every node has been visited, creates an author and updated syndication elements if none /// have been created by the visitor /// public void Dispose() { this.CreateAuthor(true); this.CreateUpdated(); } ////// Override of the base Visitor method, which actually performs mapping search and serialization /// /// Current segment being checked for mapping /// Which sub segments to serialize protected override void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind) { if (targetSegment.HasContent) { EntityPropertyMappingInfo epmInfo = targetSegment.EpmInfo; Object propertyValue; try { propertyValue = epmInfo.PropValReader.DynamicInvoke(this.Element); } catch (System.Reflection.TargetInvocationException) { throw; } String contentType; ActioncontentWriter; switch (epmInfo.Attribute.TargetTextContentKind) { case SyndicationTextContentKind.Html: contentType = "html"; contentWriter = this.Target.WriteString; break; case SyndicationTextContentKind.Xhtml: contentType = "xhtml"; contentWriter = this.Target.WriteRaw; break; default: contentType = "text"; contentWriter = this.Target.WriteString; break; } Action textSyndicationWriter = (c, nonTextPossible, atomDateConstruct) => { this.Target.WriteStartElement(c, XmlConstants.AtomNamespace); if (nonTextPossible) { this.Target.WriteAttributeString(XmlConstants.AtomTypeAttributeName, String.Empty, contentType); } // As per atomPub spec dateConstructs must contain valid datetime. Therefore we need to fill atom:updated/atom:published // field with something (e.g. DateTimeOffset.MinValue) if the user wants to map null to either of these fields. This will // satisfy protocol requirements and Syndication API. Note that the content will still contain information saying that the // mapped property is supposed to be null - therefore the server will know that the actual value sent by the user is null. // For all other elements we can use empty string since the content is not validated. String textPropertyValue = propertyValue != null ? ClientConvert.ToString(propertyValue, atomDateConstruct) : atomDateConstruct ? ClientConvert.ToString(DateTime.MinValue, atomDateConstruct) : String.Empty; contentWriter(textPropertyValue); this.Target.WriteEndElement(); }; switch (epmInfo.Attribute.TargetSyndicationItem) { case SyndicationItemProperty.AuthorEmail: case SyndicationItemProperty.ContributorEmail: textSyndicationWriter(XmlConstants.AtomEmailElementName, false, false); break; case SyndicationItemProperty.AuthorName: case SyndicationItemProperty.ContributorName: textSyndicationWriter(XmlConstants.AtomNameElementName, false, false); this.authorNamePresent = true; break; case SyndicationItemProperty.AuthorUri: case SyndicationItemProperty.ContributorUri: textSyndicationWriter(XmlConstants.AtomUriElementName, false, false); break; case SyndicationItemProperty.Updated: textSyndicationWriter(XmlConstants.AtomUpdatedElementName, false, true); this.updatedPresent = true; break; case SyndicationItemProperty.Published: textSyndicationWriter(XmlConstants.AtomPublishedElementName, false, true); break; case SyndicationItemProperty.Rights: textSyndicationWriter(XmlConstants.AtomRightsElementName, true, false); break; case SyndicationItemProperty.Summary: textSyndicationWriter(XmlConstants.AtomSummaryElementName, true, false); break; case SyndicationItemProperty.Title: textSyndicationWriter(XmlConstants.AtomTitleElementName, true, false); break; default: Debug.Assert(false, "Unhandled SyndicationItemProperty enum value - should never get here."); break; } } else { if (targetSegment.SegmentName == XmlConstants.AtomAuthorElementName) { this.CreateAuthor(false); base.Serialize(targetSegment, kind); this.FinishAuthor(); } else if (targetSegment.SegmentName == XmlConstants.AtomContributorElementName) { this.Target.WriteStartElement(XmlConstants.AtomContributorElementName, XmlConstants.AtomNamespace); base.Serialize(targetSegment, kind); this.Target.WriteEndElement(); } else { Debug.Assert(false, "Only authors and contributors have nested elements"); } } } /// /// Creates a new author syndication specific object /// /// Whether to create a null author or a non-null author private void CreateAuthor(bool createNull) { if (!this.authorInfoPresent) { if (createNull) { this.Target.WriteStartElement(XmlConstants.AtomAuthorElementName, XmlConstants.AtomNamespace); this.Target.WriteElementString(XmlConstants.AtomNameElementName, XmlConstants.AtomNamespace, String.Empty); this.Target.WriteEndElement(); } else { this.Target.WriteStartElement(XmlConstants.AtomAuthorElementName, XmlConstants.AtomNamespace); } this.authorInfoPresent = true; } } ////// Finish writing the author atom element /// private void FinishAuthor() { Debug.Assert(this.authorInfoPresent == true, "Must have already written the start element for author"); if (this.authorNamePresent == false) { this.Target.WriteElementString(XmlConstants.AtomNameElementName, XmlConstants.AtomNamespace, String.Empty); this.authorNamePresent = true; } this.Target.WriteEndElement(); } ////// Ensures that mandatory atom:update element is creatd. The value of the created element is set to with value set to DateTime.UtcNow. /// This method is used only if there are some EPM mappings for the given resource type but there is no mapping to atom:updated /// element. Otherwise the element will be created during mapping handling or if there is no EPM mapping at all during the serialization. /// private void CreateUpdated() { if (!this.updatedPresent) { this.Target.WriteElementString(XmlConstants.AtomUpdatedElementName, XmlConstants.AtomNamespace, XmlConvert.ToString(DateTime.UtcNow, XmlDateTimeSerializationMode.RoundtripKind)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
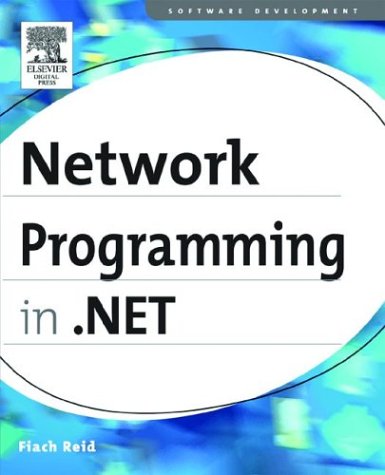
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TwoPhaseCommit.cs
- CustomErrorCollection.cs
- HMACRIPEMD160.cs
- PatternMatcher.cs
- URIFormatException.cs
- ReaderContextStackData.cs
- CompoundFileStreamReference.cs
- DbException.cs
- ArrayWithOffset.cs
- UnsafeNativeMethods.cs
- ServiceInfo.cs
- GPPOINT.cs
- EntityChangedParams.cs
- WCFBuildProvider.cs
- DataGridParentRows.cs
- WebZone.cs
- IChannel.cs
- DataServiceHostFactory.cs
- OleServicesContext.cs
- QueryContext.cs
- BamlTreeMap.cs
- EntryWrittenEventArgs.cs
- ProviderConnectionPointCollection.cs
- DbSourceParameterCollection.cs
- EntityDataSourceContextCreatedEventArgs.cs
- BufferedResponseStream.cs
- GradientBrush.cs
- BamlCollectionHolder.cs
- ToolBarButtonDesigner.cs
- PaintValueEventArgs.cs
- DataGridRow.cs
- DataBoundControlHelper.cs
- FontEmbeddingManager.cs
- FormViewPageEventArgs.cs
- WebServiceMethodData.cs
- StringBuilder.cs
- OrderedHashRepartitionStream.cs
- EntitySqlQueryCacheEntry.cs
- SoapProtocolReflector.cs
- FormsAuthenticationTicket.cs
- TagMapCollection.cs
- ConfigurationErrorsException.cs
- DataTableTypeConverter.cs
- StringValidatorAttribute.cs
- Point3DConverter.cs
- RegexReplacement.cs
- MembershipUser.cs
- ConfigurationValues.cs
- ObjectSecurity.cs
- RichTextBox.cs
- NativeMethods.cs
- InputScope.cs
- RowCache.cs
- RunClient.cs
- ExpressionNode.cs
- XmlMapping.cs
- RootProfilePropertySettingsCollection.cs
- AttributeEmitter.cs
- RequestTimeoutManager.cs
- InternalsVisibleToAttribute.cs
- UserControlParser.cs
- X500Name.cs
- StreamGeometryContext.cs
- PanelStyle.cs
- RouteData.cs
- InvalidBodyAccessException.cs
- AdRotator.cs
- mil_commands.cs
- ObjectSecurity.cs
- SchemaType.cs
- ListBoxAutomationPeer.cs
- ToolStripSystemRenderer.cs
- Win32.cs
- FlowPosition.cs
- ListItemCollection.cs
- LinearQuaternionKeyFrame.cs
- Symbol.cs
- TextElementCollectionHelper.cs
- SpeakCompletedEventArgs.cs
- TextElementCollectionHelper.cs
- WpfPayload.cs
- HybridDictionary.cs
- NoneExcludedImageIndexConverter.cs
- NetworkInterface.cs
- StylusButtonCollection.cs
- TemplatePropertyEntry.cs
- MultiTargetingUtil.cs
- FontFamily.cs
- PinnedBufferMemoryStream.cs
- ExplicitDiscriminatorMap.cs
- PartialCachingAttribute.cs
- FileDialog_Vista.cs
- GeneralTransform.cs
- ExpressionDumper.cs
- Model3DGroup.cs
- MessageHeaderInfoTraceRecord.cs
- ContainerParaClient.cs
- TemplateBuilder.cs
- WbmpConverter.cs
- SqlMethodCallConverter.cs