Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Objects / DataRecordObjectView.cs / 1 / DataRecordObjectView.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner jhutson // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Data.Common; using System.Data.Metadata; using System.Data.Metadata.Edm; using System.Reflection; namespace System.Data.Objects { ////// ObjectView that provides binding to a list of data records. /// ////// This class provides an implementation of ITypedList that returns property descriptors /// for each column of results in a data record. /// internal sealed class DataRecordObjectView : ObjectView, ITypedList { /// /// Cache of the property descriptors for the element type of the root list wrapped by ObjectView. /// private PropertyDescriptorCollection _propertyDescriptorsCache; ////// EDM RowType that describes the shape of record elements. /// private RowType _rowType; internal DataRecordObjectView(IObjectViewDataviewData, object eventDataSource, RowType rowType, Type propertyComponentType) : base(viewData, eventDataSource) { if (!typeof(IDataRecord).IsAssignableFrom(propertyComponentType)) { propertyComponentType = typeof(IDataRecord); } _rowType = rowType; _propertyDescriptorsCache = MaterializedDataRecord.CreatePropertyDescriptorCollection(_rowType, propertyComponentType, true); } /// /// Return a /// ///instance that represents /// a strongly-typed indexer property on the specified type. /// that may define the appropriate indexer. /// /// /// ///instance of indexer defined on supplied type /// that returns an object of any type but ; /// or null if no such indexer is defined on the supplied type. /// /// The algorithm here is lifted from System.Windows.Forms.ListBindingHelper, /// from the GetTypedIndexer method. /// The Entity Framework could not take a dependency on WinForms, /// so we lifted the appropriate parts from the WinForms code here. /// A bit ----, but much better than guessing as to what algorithm is proper for data binding. /// private static PropertyInfo GetTypedIndexer(Type type) { PropertyInfo indexer = null; if (typeof(IList).IsAssignableFrom(type) || typeof(ITypedList).IsAssignableFrom(type) || typeof(IListSource).IsAssignableFrom(type)) { System.Reflection.PropertyInfo[] props = type.GetProperties(BindingFlags.Public | BindingFlags.Instance); for (int idx = 0; idx < props.Length; idx++) { if (props[idx].GetIndexParameters().Length > 0 && props[idx].PropertyType != typeof(object)) { indexer = props[idx]; //Prefer the standard indexer, if there is one if (indexer.Name == "Item") { break; } } } } return indexer; } ////// Return the element type for the supplied type. /// /// ////// If ///represents a list type that doesn't also implement ITypedList or IListSource, /// return the element type for items in that list. /// Otherwise, return the type supplied by . /// /// The algorithm here is lifted from System.Windows.Forms.ListBindingHelper, /// from the GetListItemType(object) method. /// The Entity Framework could not take a dependency on WinForms, /// so we lifted the appropriate parts from the WinForms code here. /// A bit ----, but much better than guessing as to what algorithm is proper for data binding. /// private static Type GetListItemType(Type type) { Type itemType; if (typeof(Array).IsAssignableFrom(type)) { itemType = type.GetElementType(); } else { PropertyInfo typedIndexer = GetTypedIndexer(type); if (typedIndexer != null) { itemType = typedIndexer.PropertyType; } else { itemType = type; } } return itemType; } #region ITypedList Members PropertyDescriptorCollection System.ComponentModel.ITypedList.GetItemProperties(PropertyDescriptor[] listAccessors) { PropertyDescriptorCollection propertyDescriptors; if (listAccessors == null || listAccessors.Length == 0) { // Caller is requesting property descriptors for the root element type. propertyDescriptors = _propertyDescriptorsCache; } else { // Use the last PropertyDescriptor in the array to build the collection of returned property descriptors. PropertyDescriptor propertyDescriptor = listAccessors[listAccessors.Length - 1]; FieldDescriptor fieldDescriptor = propertyDescriptor as FieldDescriptor; // If the property descriptor describes a data record with the EDM type of RowType, // construct the collection of property descriptors from the property's EDM metadata. // Otherwise use the CLR type of the property. if (fieldDescriptor != null && fieldDescriptor.EdmProperty != null && fieldDescriptor.EdmProperty.TypeUsage.EdmType.BuiltInTypeKind == BuiltInTypeKind.RowType) { // Retrieve property descriptors from EDM metadata. propertyDescriptors = MaterializedDataRecord.CreatePropertyDescriptorCollection((RowType)fieldDescriptor.EdmProperty.TypeUsage.EdmType, typeof(IDataRecord), true); } else { // Use the CLR type. propertyDescriptors = TypeDescriptor.GetProperties(GetListItemType(propertyDescriptor.PropertyType)); } } return propertyDescriptors; } string System.ComponentModel.ITypedList.GetListName(PropertyDescriptor[] listAccessors) { return _rowType.Name; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner jhutson // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Data.Common; using System.Data.Metadata; using System.Data.Metadata.Edm; using System.Reflection; namespace System.Data.Objects { ////// ObjectView that provides binding to a list of data records. /// ////// This class provides an implementation of ITypedList that returns property descriptors /// for each column of results in a data record. /// internal sealed class DataRecordObjectView : ObjectView, ITypedList { /// /// Cache of the property descriptors for the element type of the root list wrapped by ObjectView. /// private PropertyDescriptorCollection _propertyDescriptorsCache; ////// EDM RowType that describes the shape of record elements. /// private RowType _rowType; internal DataRecordObjectView(IObjectViewDataviewData, object eventDataSource, RowType rowType, Type propertyComponentType) : base(viewData, eventDataSource) { if (!typeof(IDataRecord).IsAssignableFrom(propertyComponentType)) { propertyComponentType = typeof(IDataRecord); } _rowType = rowType; _propertyDescriptorsCache = MaterializedDataRecord.CreatePropertyDescriptorCollection(_rowType, propertyComponentType, true); } /// /// Return a /// ///instance that represents /// a strongly-typed indexer property on the specified type. /// that may define the appropriate indexer. /// /// /// ///instance of indexer defined on supplied type /// that returns an object of any type but ; /// or null if no such indexer is defined on the supplied type. /// /// The algorithm here is lifted from System.Windows.Forms.ListBindingHelper, /// from the GetTypedIndexer method. /// The Entity Framework could not take a dependency on WinForms, /// so we lifted the appropriate parts from the WinForms code here. /// A bit ----, but much better than guessing as to what algorithm is proper for data binding. /// private static PropertyInfo GetTypedIndexer(Type type) { PropertyInfo indexer = null; if (typeof(IList).IsAssignableFrom(type) || typeof(ITypedList).IsAssignableFrom(type) || typeof(IListSource).IsAssignableFrom(type)) { System.Reflection.PropertyInfo[] props = type.GetProperties(BindingFlags.Public | BindingFlags.Instance); for (int idx = 0; idx < props.Length; idx++) { if (props[idx].GetIndexParameters().Length > 0 && props[idx].PropertyType != typeof(object)) { indexer = props[idx]; //Prefer the standard indexer, if there is one if (indexer.Name == "Item") { break; } } } } return indexer; } ////// Return the element type for the supplied type. /// /// ////// If ///represents a list type that doesn't also implement ITypedList or IListSource, /// return the element type for items in that list. /// Otherwise, return the type supplied by . /// /// The algorithm here is lifted from System.Windows.Forms.ListBindingHelper, /// from the GetListItemType(object) method. /// The Entity Framework could not take a dependency on WinForms, /// so we lifted the appropriate parts from the WinForms code here. /// A bit ----, but much better than guessing as to what algorithm is proper for data binding. /// private static Type GetListItemType(Type type) { Type itemType; if (typeof(Array).IsAssignableFrom(type)) { itemType = type.GetElementType(); } else { PropertyInfo typedIndexer = GetTypedIndexer(type); if (typedIndexer != null) { itemType = typedIndexer.PropertyType; } else { itemType = type; } } return itemType; } #region ITypedList Members PropertyDescriptorCollection System.ComponentModel.ITypedList.GetItemProperties(PropertyDescriptor[] listAccessors) { PropertyDescriptorCollection propertyDescriptors; if (listAccessors == null || listAccessors.Length == 0) { // Caller is requesting property descriptors for the root element type. propertyDescriptors = _propertyDescriptorsCache; } else { // Use the last PropertyDescriptor in the array to build the collection of returned property descriptors. PropertyDescriptor propertyDescriptor = listAccessors[listAccessors.Length - 1]; FieldDescriptor fieldDescriptor = propertyDescriptor as FieldDescriptor; // If the property descriptor describes a data record with the EDM type of RowType, // construct the collection of property descriptors from the property's EDM metadata. // Otherwise use the CLR type of the property. if (fieldDescriptor != null && fieldDescriptor.EdmProperty != null && fieldDescriptor.EdmProperty.TypeUsage.EdmType.BuiltInTypeKind == BuiltInTypeKind.RowType) { // Retrieve property descriptors from EDM metadata. propertyDescriptors = MaterializedDataRecord.CreatePropertyDescriptorCollection((RowType)fieldDescriptor.EdmProperty.TypeUsage.EdmType, typeof(IDataRecord), true); } else { // Use the CLR type. propertyDescriptors = TypeDescriptor.GetProperties(GetListItemType(propertyDescriptor.PropertyType)); } } return propertyDescriptors; } string System.ComponentModel.ITypedList.GetListName(PropertyDescriptor[] listAccessors) { return _rowType.Name; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
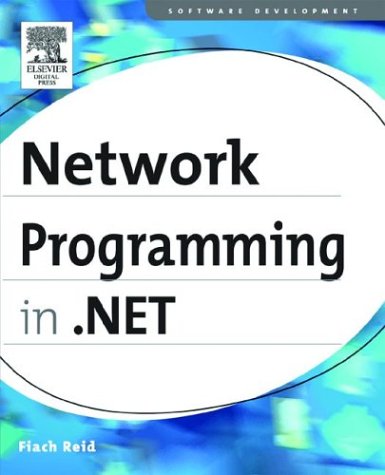
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Unit.cs
- SqlDataSourceCommandEventArgs.cs
- Assembly.cs
- Nullable.cs
- KeyEvent.cs
- ResumeStoryboard.cs
- ProjectionPathBuilder.cs
- RequestCachingSection.cs
- DataRowView.cs
- MessageEncodingBindingElementImporter.cs
- OciLobLocator.cs
- DeclaredTypeValidator.cs
- ElementHostAutomationPeer.cs
- DataServiceSaveChangesEventArgs.cs
- DiagnosticTrace.cs
- indexingfiltermarshaler.cs
- AutoGeneratedField.cs
- GridSplitterAutomationPeer.cs
- Serializer.cs
- mansign.cs
- Invariant.cs
- XmlSiteMapProvider.cs
- ResXResourceReader.cs
- OleDbTransaction.cs
- VerifyHashRequest.cs
- DataGridBoolColumn.cs
- BCLDebug.cs
- AsymmetricSignatureFormatter.cs
- KeyBinding.cs
- TextDecorationCollection.cs
- VerificationException.cs
- Error.cs
- LinqDataSourceDisposeEventArgs.cs
- DataGridViewColumnConverter.cs
- AdapterUtil.cs
- selecteditemcollection.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- HttpCookiesSection.cs
- IntSecurity.cs
- HierarchicalDataSourceControl.cs
- TargetParameterCountException.cs
- RelationshipFixer.cs
- MethodBody.cs
- DataServiceRequestOfT.cs
- AuthenticationSection.cs
- NavigationEventArgs.cs
- Stackframe.cs
- TrustLevel.cs
- TextSelectionProcessor.cs
- SmtpLoginAuthenticationModule.cs
- ExtendedTransformFactory.cs
- PageRequestManager.cs
- GridProviderWrapper.cs
- DbExpressionRules.cs
- DictionaryMarkupSerializer.cs
- ConfigurationSettings.cs
- HtmlInputFile.cs
- ObjectPersistData.cs
- SynchronizationValidator.cs
- FixedDocumentSequencePaginator.cs
- CompositeDataBoundControl.cs
- ReferenceEqualityComparer.cs
- StringUtil.cs
- EntityViewContainer.cs
- DataBinding.cs
- XmlSchemaException.cs
- Int64KeyFrameCollection.cs
- SystemIPGlobalProperties.cs
- ServiceInfo.cs
- ElementNotAvailableException.cs
- Header.cs
- Vector.cs
- VirtualDirectoryMappingCollection.cs
- SerializerWriterEventHandlers.cs
- SymmetricAlgorithm.cs
- StateDesigner.TransitionInfo.cs
- AdPostCacheSubstitution.cs
- AnchorEditor.cs
- XmlWrappingReader.cs
- NullableIntSumAggregationOperator.cs
- StickyNote.cs
- CodeNamespace.cs
- PowerModeChangedEventArgs.cs
- SrgsDocumentParser.cs
- InkSerializer.cs
- AuditLevel.cs
- SqlDataSourceSelectingEventArgs.cs
- QueuePathEditor.cs
- CompositeCollectionView.cs
- CheckPair.cs
- InstancePersistenceEvent.cs
- Label.cs
- DelegateSerializationHolder.cs
- FatalException.cs
- PersonalizableTypeEntry.cs
- TypefaceMap.cs
- BitStream.cs
- MetadataItem.cs
- OLEDB_Util.cs
- WMICapabilities.cs