Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / Command / KeyBinding.cs / 1 / KeyBinding.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: The KeyBinding class is used by the developer to create Keyboard Input Bindings // // See spec at : [....]/coreui/Specs/Commanding(new).mht // //* KeyBinding class serves the purpose of Input Bindings for Keyboard Device. // // History: // 06/01/2003 : [....] - Created // 05/01/2004 : chandra - changed to accommodate new design // ( [....]/coreui/Specs/Commanding(new).mht ) //--------------------------------------------------------------------------- using System; using System.Windows.Input; using System.Windows; using System.ComponentModel; using System.Windows.Markup; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ////// KeyBinding - Implements InputBinding (generic InputGesture-Command map) /// KeyBinding acts like a map for KeyGesture and Commands. /// Most of the logic is in InputBinding and KeyGesture, this only /// facilitates user to add Key/Modifiers directly without going in /// KeyGesture path. Also it provides the KeyGestureTypeConverter /// on the Gesture property to have KeyGesture, like Ctrl+X, Alt+V /// defined in Markup as Gesture="Ctrl+X" working /// public class KeyBinding : InputBinding { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructor ////// Constructor /// public KeyBinding() : base() { } ////// Constructor /// /// Command associated /// KeyGesture associated public KeyBinding(ICommand command, KeyGesture gesture) : base(command, gesture) { } ////// Constructor /// /// /// modifiers /// key public KeyBinding(ICommand command, Key key, ModifierKeys modifiers) : base(command, new KeyGesture(key, modifiers)) { } #endregion Constructor //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// KeyGesture Override, to ensure type-safety and provide a /// TypeConverter for KeyGesture /// [TypeConverter(typeof(KeyGestureConverter))] [ValueSerializer(typeof(KeyGestureValueSerializer))] public override InputGesture Gesture { get { return base.Gesture as KeyGesture; } set { if (value is KeyGesture) { base.Gesture = value; } else { throw new ArgumentException(SR.Get(SRID.InputBinding_ExpectedInputGesture, typeof(KeyGesture))); } } } ////// Modifier /// public ModifierKeys Modifiers { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Modifiers; } return ModifierKeys.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture(Key.None, (ModifierKeys)value, /*validateGesture = */ false); } else { Gesture = new KeyGesture(((KeyGesture)Gesture).Key, value, /*validateGesture = */ false); } } } } ////// Key /// public Key Key { get { lock (_dataLock) { if (null != Gesture) { return ((KeyGesture)Gesture).Key; } return Key.None; } } set { lock (_dataLock) { if (null == Gesture) { Gesture = new KeyGesture((Key)value, ModifierKeys.None, /*validateGesture = */ false); } else { Gesture = new KeyGesture(value, ((KeyGesture)Gesture).Modifiers, /*validateGesture = */ false); } } } } #endregion Public Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
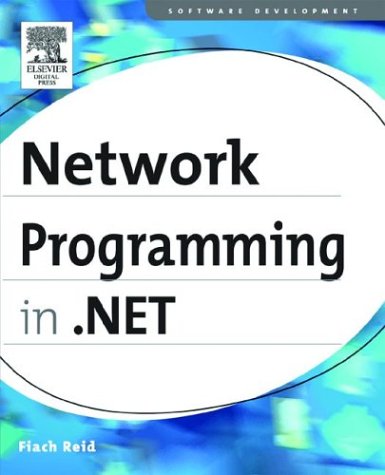
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XPathNavigatorKeyComparer.cs
- OutputCacheProfile.cs
- RoutedEventHandlerInfo.cs
- SoapEnvelopeProcessingElement.cs
- CatalogPart.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- FixUpCollection.cs
- UnsafeNativeMethods.cs
- DbCommandDefinition.cs
- EdgeModeValidation.cs
- CounterCreationDataCollection.cs
- DataGridParentRows.cs
- DockPanel.cs
- ConfigsHelper.cs
- SqlCommand.cs
- DecimalAnimation.cs
- BuildManagerHost.cs
- RepeatInfo.cs
- NotifyIcon.cs
- IDReferencePropertyAttribute.cs
- ComponentDispatcherThread.cs
- HttpCookie.cs
- Int64Converter.cs
- FacetDescriptionElement.cs
- TextTrailingWordEllipsis.cs
- SystemMulticastIPAddressInformation.cs
- ConfigurationLocation.cs
- CodeDomConfigurationHandler.cs
- CompositeScriptReference.cs
- HiddenField.cs
- SByteConverter.cs
- ImageCodecInfo.cs
- BitmapEffectGroup.cs
- EntityDescriptor.cs
- TransformationRules.cs
- Propagator.Evaluator.cs
- NativeRecognizer.cs
- DataGridItemEventArgs.cs
- UnsafeNativeMethods.cs
- CommonRemoteMemoryBlock.cs
- CurrentChangedEventManager.cs
- UrlPropertyAttribute.cs
- QueryLifecycle.cs
- ListenerElementsCollection.cs
- cache.cs
- CustomError.cs
- StringInfo.cs
- ImageConverter.cs
- NumericUpDownAcceleration.cs
- ToolStripPanelRow.cs
- TypeListConverter.cs
- PropertyTab.cs
- SystemIcons.cs
- HWStack.cs
- InheritanceAttribute.cs
- ProvidersHelper.cs
- TabPageDesigner.cs
- ProjectionPathBuilder.cs
- DateTimeFormatInfo.cs
- StylusSystemGestureEventArgs.cs
- DesignerHelpers.cs
- UniqueIdentifierService.cs
- AuthenticationModuleElementCollection.cs
- SafeNativeMethods.cs
- SchemaDeclBase.cs
- AxImporter.cs
- Track.cs
- AppDomainManager.cs
- DataTransferEventArgs.cs
- HyperLinkStyle.cs
- GetBrowserTokenRequest.cs
- CompiledQuery.cs
- CategoryGridEntry.cs
- System.Data_BID.cs
- IODescriptionAttribute.cs
- ConsoleTraceListener.cs
- PathSegment.cs
- ToggleButtonAutomationPeer.cs
- DataGridViewRowStateChangedEventArgs.cs
- Hyperlink.cs
- HtmlLink.cs
- XmlDataProvider.cs
- SemaphoreFullException.cs
- ResourceManagerWrapper.cs
- PriorityItem.cs
- Variable.cs
- CodePageUtils.cs
- newinstructionaction.cs
- controlskin.cs
- SchemaNotation.cs
- ListParagraph.cs
- RC2CryptoServiceProvider.cs
- SecurityManager.cs
- ParameterEditorUserControl.cs
- ExceptionCollection.cs
- UnsafeNativeMethods.cs
- FilterException.cs
- StrongTypingException.cs
- RenamedEventArgs.cs
- PathFigureCollectionConverter.cs