Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Advanced / ImageCodecInfo.cs / 1 / ImageCodecInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing; using System.Drawing.Internal; // sdkinc\imaging.h ////// /// public sealed class ImageCodecInfo { Guid clsid; Guid formatID; string codecName; string dllName; string formatDescription; string filenameExtension; string mimeType; ImageCodecFlags flags; int version; byte[][] signaturePatterns; byte[][] signatureMasks; internal ImageCodecInfo() { } ///[To be supplied.] ////// /// public Guid Clsid { get { return clsid; } set { clsid = value; } } ///[To be supplied.] ////// /// public Guid FormatID { get { return formatID; } set { formatID = value; } } ///[To be supplied.] ////// /// public string CodecName { get { return codecName; } set { codecName = value; } } ///[To be supplied.] ////// /// public string DllName { [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] get { if (dllName != null) { //a valid path is a security concern, demand //path discovery permission.... new System.Security.Permissions.FileIOPermission(System.Security.Permissions.FileIOPermissionAccess.PathDiscovery, dllName).Demand(); } return dllName; } [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] set { if (value != null) { //a valid path is a security concern, demand //path discovery permission.... new System.Security.Permissions.FileIOPermission(System.Security.Permissions.FileIOPermissionAccess.PathDiscovery, value).Demand(); } dllName = value; } } ///[To be supplied.] ////// /// public string FormatDescription { get { return formatDescription; } set { formatDescription = value; } } ///[To be supplied.] ////// /// public string FilenameExtension { get { return filenameExtension; } set { filenameExtension = value; } } ///[To be supplied.] ////// /// public string MimeType { get { return mimeType; } set { mimeType = value; } } ///[To be supplied.] ////// /// public ImageCodecFlags Flags { get { return flags; } set { flags = value; } } ///[To be supplied.] ////// /// public int Version { get { return version; } set { version = value; } } ///[To be supplied.] ////// /// [CLSCompliant(false)] public byte[][] SignaturePatterns { get { return signaturePatterns; } set { signaturePatterns = value; } } ///[To be supplied.] ////// /// [CLSCompliant(false)] public byte[][] SignatureMasks { get { return signatureMasks; } set { signatureMasks = value; } } // Encoder/Decoder selection APIs ///[To be supplied.] ////// /// public static ImageCodecInfo[] GetImageDecoders() { ImageCodecInfo[] imageCodecs; int numDecoders; int size; int status = SafeNativeMethods.Gdip.GdipGetImageDecodersSize(out numDecoders, out size); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } IntPtr memory = Marshal.AllocHGlobal(size); try { status = SafeNativeMethods.Gdip.GdipGetImageDecoders(numDecoders, size, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } imageCodecs = ImageCodecInfo.ConvertFromMemory(memory, numDecoders); } finally { Marshal.FreeHGlobal(memory); } return imageCodecs; } ///[To be supplied.] ////// /// public static ImageCodecInfo[] GetImageEncoders() { ImageCodecInfo[] imageCodecs; int numEncoders; int size; int status = SafeNativeMethods.Gdip.GdipGetImageEncodersSize(out numEncoders, out size); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } IntPtr memory = Marshal.AllocHGlobal(size); try { status = SafeNativeMethods.Gdip.GdipGetImageEncoders(numEncoders, size, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } imageCodecs = ImageCodecInfo.ConvertFromMemory(memory, numEncoders); } finally { Marshal.FreeHGlobal(memory); } return imageCodecs; } /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. internal static ImageCodecInfoPrivate ConvertToMemory(ImageCodecInfo imagecs) { ImageCodecInfoPrivate imagecsp = new ImageCodecInfoPrivate(); imagecsp.Clsid = imagecs.Clsid; imagecsp.FormatID = imagecs.FormatID; imagecsp.CodecName = Marshal.StringToHGlobalUni(imagecs.CodecName); imagecsp.DllName = Marshal.StringToHGlobalUni(imagecs.DllName); imagecsp.FormatDescription = Marshal.StringToHGlobalUni(imagecs.FormatDescription); imagecsp.FilenameExtension = Marshal.StringToHGlobalUni(imagecs.FilenameExtension); imagecsp.MimeType = Marshal.StringToHGlobalUni(imagecs.MimeType); imagecsp.Flags = (int)imagecs.Flags; imagecsp.Version = (int)imagecs.Version; imagecsp.SigCount = imagecs.SignaturePatterns.Length; imagecsp.SigSize = imagecs.SignaturePatterns[0].Length; imagecsp.SigPattern = Marshal.AllocHGlobal(imagecsp.SigCount*imagecsp.SigSize); imagecsp.SigMask = Marshal.AllocHGlobal(imagecsp.SigCount*imagecsp.SigSize); for (int i=0; i[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing; using System.Drawing.Internal; // sdkinc\imaging.h /// /// /// public sealed class ImageCodecInfo { Guid clsid; Guid formatID; string codecName; string dllName; string formatDescription; string filenameExtension; string mimeType; ImageCodecFlags flags; int version; byte[][] signaturePatterns; byte[][] signatureMasks; internal ImageCodecInfo() { } ///[To be supplied.] ////// /// public Guid Clsid { get { return clsid; } set { clsid = value; } } ///[To be supplied.] ////// /// public Guid FormatID { get { return formatID; } set { formatID = value; } } ///[To be supplied.] ////// /// public string CodecName { get { return codecName; } set { codecName = value; } } ///[To be supplied.] ////// /// public string DllName { [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] get { if (dllName != null) { //a valid path is a security concern, demand //path discovery permission.... new System.Security.Permissions.FileIOPermission(System.Security.Permissions.FileIOPermissionAccess.PathDiscovery, dllName).Demand(); } return dllName; } [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] set { if (value != null) { //a valid path is a security concern, demand //path discovery permission.... new System.Security.Permissions.FileIOPermission(System.Security.Permissions.FileIOPermissionAccess.PathDiscovery, value).Demand(); } dllName = value; } } ///[To be supplied.] ////// /// public string FormatDescription { get { return formatDescription; } set { formatDescription = value; } } ///[To be supplied.] ////// /// public string FilenameExtension { get { return filenameExtension; } set { filenameExtension = value; } } ///[To be supplied.] ////// /// public string MimeType { get { return mimeType; } set { mimeType = value; } } ///[To be supplied.] ////// /// public ImageCodecFlags Flags { get { return flags; } set { flags = value; } } ///[To be supplied.] ////// /// public int Version { get { return version; } set { version = value; } } ///[To be supplied.] ////// /// [CLSCompliant(false)] public byte[][] SignaturePatterns { get { return signaturePatterns; } set { signaturePatterns = value; } } ///[To be supplied.] ////// /// [CLSCompliant(false)] public byte[][] SignatureMasks { get { return signatureMasks; } set { signatureMasks = value; } } // Encoder/Decoder selection APIs ///[To be supplied.] ////// /// public static ImageCodecInfo[] GetImageDecoders() { ImageCodecInfo[] imageCodecs; int numDecoders; int size; int status = SafeNativeMethods.Gdip.GdipGetImageDecodersSize(out numDecoders, out size); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } IntPtr memory = Marshal.AllocHGlobal(size); try { status = SafeNativeMethods.Gdip.GdipGetImageDecoders(numDecoders, size, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } imageCodecs = ImageCodecInfo.ConvertFromMemory(memory, numDecoders); } finally { Marshal.FreeHGlobal(memory); } return imageCodecs; } ///[To be supplied.] ////// /// public static ImageCodecInfo[] GetImageEncoders() { ImageCodecInfo[] imageCodecs; int numEncoders; int size; int status = SafeNativeMethods.Gdip.GdipGetImageEncodersSize(out numEncoders, out size); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } IntPtr memory = Marshal.AllocHGlobal(size); try { status = SafeNativeMethods.Gdip.GdipGetImageEncoders(numEncoders, size, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } imageCodecs = ImageCodecInfo.ConvertFromMemory(memory, numEncoders); } finally { Marshal.FreeHGlobal(memory); } return imageCodecs; } /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. internal static ImageCodecInfoPrivate ConvertToMemory(ImageCodecInfo imagecs) { ImageCodecInfoPrivate imagecsp = new ImageCodecInfoPrivate(); imagecsp.Clsid = imagecs.Clsid; imagecsp.FormatID = imagecs.FormatID; imagecsp.CodecName = Marshal.StringToHGlobalUni(imagecs.CodecName); imagecsp.DllName = Marshal.StringToHGlobalUni(imagecs.DllName); imagecsp.FormatDescription = Marshal.StringToHGlobalUni(imagecs.FormatDescription); imagecsp.FilenameExtension = Marshal.StringToHGlobalUni(imagecs.FilenameExtension); imagecsp.MimeType = Marshal.StringToHGlobalUni(imagecs.MimeType); imagecsp.Flags = (int)imagecs.Flags; imagecsp.Version = (int)imagecs.Version; imagecsp.SigCount = imagecs.SignaturePatterns.Length; imagecsp.SigSize = imagecs.SignaturePatterns[0].Length; imagecsp.SigPattern = Marshal.AllocHGlobal(imagecsp.SigCount*imagecsp.SigSize); imagecsp.SigMask = Marshal.AllocHGlobal(imagecsp.SigCount*imagecsp.SigSize); for (int i=0; i[To be supplied.] ///
Link Menu
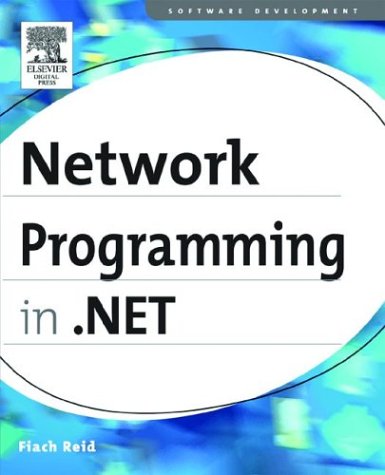
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewRowPresenter.cs
- HttpCookiesSection.cs
- TextBoxBaseDesigner.cs
- SplayTreeNode.cs
- Sentence.cs
- OrderedHashRepartitionStream.cs
- EntityCollectionChangedParams.cs
- ChangePasswordAutoFormat.cs
- SafeNativeMethods.cs
- Positioning.cs
- VectorKeyFrameCollection.cs
- EncryptedType.cs
- UIElementIsland.cs
- DefaultEventAttribute.cs
- PropertyGeneratedEventArgs.cs
- versioninfo.cs
- OperationFormatUse.cs
- WebPartConnectionsCancelVerb.cs
- WebBrowser.cs
- Drawing.cs
- SizeAnimationBase.cs
- DataRowView.cs
- StateMachineTimers.cs
- DelegateTypeInfo.cs
- WebPartsPersonalizationAuthorization.cs
- ReachIDocumentPaginatorSerializerAsync.cs
- HttpChannelListener.cs
- ProfileParameter.cs
- Visitor.cs
- UrlMappingsModule.cs
- BStrWrapper.cs
- CapiSymmetricAlgorithm.cs
- FileAuthorizationModule.cs
- SqlParameterizer.cs
- BindingMemberInfo.cs
- Oid.cs
- DirtyTextRange.cs
- HostProtectionPermission.cs
- ToolStripContentPanelDesigner.cs
- Token.cs
- SelectionWordBreaker.cs
- TypeUsageBuilder.cs
- ReferenceAssemblyAttribute.cs
- FullTextBreakpoint.cs
- HierarchicalDataTemplate.cs
- IgnoreSection.cs
- SQLBoolean.cs
- Point.cs
- HostExecutionContextManager.cs
- unsafenativemethodsother.cs
- TextParaLineResult.cs
- ScrollItemPatternIdentifiers.cs
- MethodCallExpression.cs
- Panel.cs
- Pair.cs
- TypefaceMetricsCache.cs
- TraceSwitch.cs
- DataGridClipboardCellContent.cs
- TextTreeRootTextBlock.cs
- SchemaCollectionPreprocessor.cs
- TypeInfo.cs
- NullableFloatMinMaxAggregationOperator.cs
- CircleHotSpot.cs
- StateWorkerRequest.cs
- IfAction.cs
- DomainConstraint.cs
- URIFormatException.cs
- SerializationInfoEnumerator.cs
- XslCompiledTransform.cs
- ReadWriteObjectLock.cs
- DbConnectionPoolOptions.cs
- GradientStop.cs
- IriParsingElement.cs
- InvalidateEvent.cs
- PerfCounters.cs
- securitycriticaldataClass.cs
- GlyphRun.cs
- Operand.cs
- FixedSOMLineCollection.cs
- BrowserCapabilitiesFactoryBase.cs
- SqlConnectionString.cs
- BinaryParser.cs
- XmlWriterTraceListener.cs
- PEFileReader.cs
- ListBoxItemAutomationPeer.cs
- Expression.cs
- SubMenuStyle.cs
- XmlWellformedWriterHelpers.cs
- DispatcherHooks.cs
- CodeDefaultValueExpression.cs
- LeaseManager.cs
- ServiceNameElement.cs
- SineEase.cs
- Rotation3DAnimation.cs
- EntityViewGenerationAttribute.cs
- Executor.cs
- NameSpaceExtractor.cs
- ImageCodecInfo.cs
- IgnoreSection.cs
- SqlVisitor.cs