Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / HttpChannelListener.cs / 2 / HttpChannelListener.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel; using System.ServiceModel.Activation; using System.ServiceModel.Description; using System.Collections.ObjectModel; using System.Diagnostics; using System.IO; using System.Net; using System.Runtime.Serialization; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Selectors; using System.IdentityModel.Tokens; using System.Security.Principal; using System.Security.Cryptography.X509Certificates; using System.ServiceModel.Security.Tokens; using System.ServiceModel.Diagnostics; using System.ServiceModel.Security; using System.ServiceModel.Dispatcher; using System.Text; using System.Threading; using System.Web; using System.Web.Hosting; using System.Xml; using System.Runtime.CompilerServices; class HttpChannelListener : TransportChannelListener , IHttpTransportFactorySettings , IChannelListener{ ReplyChannelAcceptor acceptor; AuthenticationSchemes authenticationScheme; bool extractGroupsForWindowsAccounts; EndpointIdentity identity; bool keepAliveEnabled; int maxBufferSize; string method; string realm; TransferMode transferMode; bool unsafeConnectionNtlmAuthentication; ISecurityCapabilities securityCapabilities; SecurityCredentialsManager credentialProvider; SecurityTokenAuthenticator userNameTokenAuthenticator; SecurityTokenAuthenticator windowsTokenAuthenticator; static UriPrefixTable transportManagerTable = new UriPrefixTable (true); public HttpChannelListener(HttpTransportBindingElement bindingElement, BindingContext context) : base(bindingElement, context, HttpTransportDefaults.GetDefaultMessageEncoderFactory(), bindingElement.HostNameComparisonMode) { if (bindingElement.TransferMode == TransferMode.Buffered) { if (bindingElement.MaxReceivedMessageSize > int.MaxValue) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new ArgumentOutOfRangeException("bindingElement.MaxReceivedMessageSize", SR.GetString(SR.MaxReceivedMessageSizeMustBeInIntegerRange))); } if (bindingElement.MaxBufferSize != bindingElement.MaxReceivedMessageSize) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("bindingElement", SR.GetString(SR.MaxBufferSizeMustMatchMaxReceivedMessageSize)); } } else { if (bindingElement.MaxBufferSize > bindingElement.MaxReceivedMessageSize) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("bindingElement", SR.GetString(SR.MaxBufferSizeMustNotExceedMaxReceivedMessageSize)); } } this.authenticationScheme = bindingElement.AuthenticationScheme; this.keepAliveEnabled = bindingElement.KeepAliveEnabled; this.InheritBaseAddressSettings = bindingElement.InheritBaseAddressSettings; this.maxBufferSize = bindingElement.MaxBufferSize; this.method = bindingElement.Method; this.realm = bindingElement.Realm; this.transferMode = bindingElement.TransferMode; this.unsafeConnectionNtlmAuthentication = bindingElement.UnsafeConnectionNtlmAuthentication; this.credentialProvider = context.BindingParameters.Find (); this.acceptor = new TransportReplyChannelAcceptor(this); this.securityCapabilities = bindingElement.GetProperty (context); } internal override void ApplyHostedContext(VirtualPathExtension virtualPathExtension, bool isMetadataListener) { base.ApplyHostedContext(virtualPathExtension, isMetadataListener); // // Verify the authentication settings // AuthenticationSchemes supportedSchemes = HostedTransportConfigurationManager.MetabaseSettings.GetAuthenticationSchemes(HostedVirtualPath); if (this.AuthenticationScheme == AuthenticationSchemes.Anonymous) { if ((supportedSchemes & AuthenticationSchemes.Anonymous) == 0) { // if (isMetadataListener) { // We apply IIS settings to the ChannelListener to fix mex endpoints. if ((supportedSchemes & AuthenticationSchemes.Negotiate) != AuthenticationSchemes.None) { this.authenticationScheme = AuthenticationSchemes.Negotiate; } else { this.authenticationScheme = supportedSchemes; } } } } if ((supportedSchemes & this.AuthenticationScheme) == 0) { if (AuthenticationSchemesHelper.IsWindowsAuth(this.AuthenticationScheme)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR.GetString(SR.Hosting_AuthSchemesRequireWindowsAuth))); } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException(SR.GetString(SR.Hosting_AuthSchemesRequireOtherAuth, this.AuthenticationScheme.ToString()))); } } // Do not set realm for Cassini. if (!ServiceHostingEnvironment.IsSimpleApplicationHost) { // Set the realm this.realm = HostedTransportConfigurationManager.MetabaseSettings.GetRealm(virtualPathExtension.VirtualPath); } } public AuthenticationSchemes AuthenticationScheme { get { return this.authenticationScheme; } } public bool KeepAliveEnabled { get { return this.keepAliveEnabled; } } public bool ExtractGroupsForWindowsAccounts { get { return this.extractGroupsForWindowsAccounts; } } public HostNameComparisonMode HostNameComparisonMode { get { return this.HostNameComparisonModeInternal; } } bool IsAuthenticationRequired { get { return (this.AuthenticationScheme != AuthenticationSchemes.Anonymous); } } public int MaxBufferSize { get { return this.maxBufferSize; } } public string Method { get { return this.method; } } public TransferMode TransferMode { get { return transferMode; } } public string Realm { get { return this.realm; } } int IHttpTransportFactorySettings.MaxBufferSize { get { return MaxBufferSize; } } TransferMode IHttpTransportFactorySettings.TransferMode { get { return TransferMode; } } public override string Scheme { get { return Uri.UriSchemeHttp; } } internal static UriPrefixTable StaticTransportManagerTable { get { return transportManagerTable; } } public bool UnsafeConnectionNtlmAuthentication { get { return this.unsafeConnectionNtlmAuthentication; } } internal override UriPrefixTable TransportManagerTable { get { return transportManagerTable; } } internal override ITransportManagerRegistration CreateTransportManagerRegistration(Uri listenUri) { return new SharedHttpTransportManager(listenUri, this); } string GetAuthType(HttpListenerContext listenerContext) { string authType = null; IPrincipal principal = listenerContext.User; if ((principal != null) && (principal.Identity != null)) { authType = principal.Identity.AuthenticationType; } return authType; } string GetAuthType(HostedHttpRequestAsyncResult hostedAsyncResult) { string authType = null; if (hostedAsyncResult.LogonUserIdentity != null) { authType = hostedAsyncResult.LogonUserIdentity.AuthenticationType; } return authType; } bool IsAuthSchemeValid(string authType) { return AuthenticationSchemesHelper.DoesAuthTypeMatch(this.authenticationScheme, authType); } internal override int GetMaxBufferSize() { return MaxBufferSize; } public override T GetProperty () { if (typeof(T) == typeof(EndpointIdentity)) { return (T)(object)(this.identity); } else if (typeof(T) == typeof(ILogonTokenCacheManager)) { object cacheManager = (object)GetIdentityModelProperty (); if (cacheManager != null) { return (T)cacheManager; } } else if (typeof(T) == typeof(ISecurityCapabilities)) { return (T)(object)this.securityCapabilities; } return base.GetProperty (); } [MethodImpl(MethodImplOptions.NoInlining)] T GetIdentityModelProperty () { if (typeof(T) == typeof(EndpointIdentity)) { if (this.identity == null) { if (AuthenticationSchemesHelper.IsWindowsAuth(this.authenticationScheme)) { this.identity = SecurityUtils.CreateWindowsIdentity(); } } return (T)(object)this.identity; } else if (typeof(T) == typeof(ILogonTokenCacheManager) && (this.userNameTokenAuthenticator != null)) { ILogonTokenCacheManager retVal = this.userNameTokenAuthenticator as ILogonTokenCacheManager; if (retVal != null) { return (T)(object)retVal; } } return default(T); } internal bool HttpContextReceived(HttpRequestContext context, ItemDequeuedCallback callback) { bool enqueued = false; bool success = false; try { if (!context.ProcessAuthentication()) { if (DiagnosticUtility.ShouldTraceInformation) { TraceUtility.TraceEvent(TraceEventType.Information, TraceCode.HttpAuthFailed, this); } success = true; return false; } try { context.CreateMessage(); } catch (ProtocolException protocolException) { HttpStatusCode statusCode = HttpStatusCode.BadRequest; string statusDescription = string.Empty; if (protocolException.Data.Contains(HttpChannelUtilities.HttpStatusCodeExceptionKey)) { statusCode = (HttpStatusCode)protocolException.Data[HttpChannelUtilities.HttpStatusCodeExceptionKey]; protocolException.Data.Remove(HttpChannelUtilities.HttpStatusCodeExceptionKey); } if (protocolException.Data.Contains(HttpChannelUtilities.HttpStatusDescriptionExceptionKey)) { statusDescription = (string)protocolException.Data[HttpChannelUtilities.HttpStatusDescriptionExceptionKey]; protocolException.Data.Remove(HttpChannelUtilities.HttpStatusDescriptionExceptionKey); } context.SendResponseAndClose(statusCode, statusDescription); throw; } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } try { context.SendResponseAndClose(HttpStatusCode.BadRequest); } catch (Exception closeException) { if (DiagnosticUtility.IsFatal(closeException)) { throw; } if (DiagnosticUtility.ShouldTraceError) { DiagnosticUtility.ExceptionUtility.TraceHandledException(closeException, TraceEventType.Error); } } throw; } enqueued = true; acceptor.Enqueue(context, callback); success = true; } catch (CommunicationException e) { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Information); } } catch (XmlException e) { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Information); } } catch (IOException e) { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Information); } } catch (TimeoutException e) { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.ExceptionUtility.TraceHandledException(e, TraceEventType.Information); } } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } if (!ExceptionHandler.HandleTransportExceptionHelper(e)) { throw; } // containment -- we abort the context in all error cases, no additional containment action needed } finally { if (!success) { context.Abort(); } } return enqueued; } [MethodImpl(MethodImplOptions.NoInlining)] void InitializeSecurityTokenAuthenticator() { ServiceCredentials serviceCredentials = this.credentialProvider as ServiceCredentials; if (serviceCredentials != null) { this.extractGroupsForWindowsAccounts = this.AuthenticationScheme == AuthenticationSchemes.Basic ? serviceCredentials.UserNameAuthentication.IncludeWindowsGroups : serviceCredentials.WindowsAuthentication.IncludeWindowsGroups; // we will only support custom and windows validation modes, if anything else is specified, we'll fall back to windows user name. if (serviceCredentials.UserNameAuthentication.UserNamePasswordValidationMode == UserNamePasswordValidationMode.Custom) { this.userNameTokenAuthenticator = new CustomUserNameSecurityTokenAuthenticator(serviceCredentials.UserNameAuthentication.GetUserNamePasswordValidator()); } else { if (serviceCredentials.UserNameAuthentication.CacheLogonTokens) { this.userNameTokenAuthenticator = new WindowsUserNameCachingSecurityTokenAuthenticator(this.extractGroupsForWindowsAccounts, serviceCredentials.UserNameAuthentication.MaxCachedLogonTokens, serviceCredentials.UserNameAuthentication.CachedLogonTokenLifetime); } else { this.userNameTokenAuthenticator = new WindowsUserNameSecurityTokenAuthenticator(this.extractGroupsForWindowsAccounts); } } } else { this.extractGroupsForWindowsAccounts = TransportDefaults.ExtractGroupsForWindowsAccounts; this.userNameTokenAuthenticator = new WindowsUserNameSecurityTokenAuthenticator(this.extractGroupsForWindowsAccounts); } this.windowsTokenAuthenticator = new WindowsSecurityTokenAuthenticator(this.extractGroupsForWindowsAccounts); } protected override void OnOpened() { base.OnOpened(); if (this.IsAuthenticationRequired) { InitializeSecurityTokenAuthenticator(); this.identity = GetIdentityModelProperty (); } } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedOpenAsyncResult(timeout, callback, state, base.OnBeginOpen, base.OnEndOpen, this.acceptor); } protected override void OnEndOpen(IAsyncResult result) { ChainedOpenAsyncResult.End(result); } protected override void OnOpen(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnOpen(timeoutHelper.RemainingTime()); this.acceptor.Open(timeoutHelper.RemainingTime()); } protected override void OnClose(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); this.acceptor.Close(timeoutHelper.RemainingTime()); if (this.IsAuthenticationRequired) { CloseUserNameTokenAuthenticator(timeoutHelper.RemainingTime()); } base.OnClose(timeoutHelper.RemainingTime()); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); ICommunicationObject[] communicationObjects; ICommunicationObject communicationObject = this.userNameTokenAuthenticator as ICommunicationObject; if (communicationObject == null) { if (this.IsAuthenticationRequired) { CloseUserNameTokenAuthenticator(timeoutHelper.RemainingTime()); } communicationObjects = new ICommunicationObject[] { this.acceptor }; } else { communicationObjects = new ICommunicationObject[] { this.acceptor, communicationObject }; } return new ChainedCloseAsyncResult(timeoutHelper.RemainingTime(), callback, state, base.OnBeginClose, base.OnEndClose, communicationObjects); } protected override void OnEndClose(IAsyncResult result) { ChainedCloseAsyncResult.End(result); } [MethodImpl(MethodImplOptions.NoInlining)] void CloseUserNameTokenAuthenticator(TimeSpan timeout) { SecurityUtils.CloseTokenAuthenticatorIfRequired(this.userNameTokenAuthenticator, timeout); } protected override void OnAbort() { if (this.IsAuthenticationRequired) { AbortUserNameTokenAuthenticator(); } this.acceptor.Abort(); base.OnAbort(); } [MethodImpl(MethodImplOptions.NoInlining)] void AbortUserNameTokenAuthenticator() { SecurityUtils.AbortTokenAuthenticatorIfRequired(this.userNameTokenAuthenticator); } public virtual SecurityMessageProperty ProcessAuthentication(HostedHttpRequestAsyncResult result) { if (this.IsAuthenticationRequired) { SecurityMessageProperty retValue; try { retValue = this.ProcessAuthentication(result.LogonUserIdentity); } #pragma warning suppress 56500 // covered by FXCop catch (Exception exception) { if (DiagnosticUtility.IsFatal(exception)) throw; // Audit Authentication failure if (AuditLevel.Failure == (this.AuditBehavior.MessageAuthenticationAuditLevel & AuditLevel.Failure)) WriteAuditEvent(AuditLevel.Failure, (result.LogonUserIdentity != null) ? result.LogonUserIdentity.Name : String.Empty, exception); throw; } // Audit Authentication success if (AuditLevel.Success == (this.AuditBehavior.MessageAuthenticationAuditLevel & AuditLevel.Success)) WriteAuditEvent(AuditLevel.Success, (result.LogonUserIdentity != null) ? result.LogonUserIdentity.Name : String.Empty, null); return retValue; } else { return null; } } public virtual SecurityMessageProperty ProcessAuthentication(HttpListenerContext listenerContext) { if (this.IsAuthenticationRequired) { return this.ProcessRequiredAuthentication(listenerContext); } else { return null; } } SecurityMessageProperty ProcessRequiredAuthentication(HttpListenerContext listenerContext) { SecurityMessageProperty retValue; HttpListenerBasicIdentity identity = null; WindowsIdentity wid = null; try { DiagnosticUtility.DebugAssert(listenerContext.User != null, "HttpListener delivered authenticated request without an IPrincipal."); if (this.AuthenticationScheme == AuthenticationSchemes.Basic) { identity = listenerContext.User.Identity as HttpListenerBasicIdentity; DiagnosticUtility.DebugAssert(identity != null, "HttpListener delivered Basic authenticated request with a non-Basic IIdentity."); retValue = this.ProcessAuthentication(identity); } else { wid = listenerContext.User.Identity as WindowsIdentity; DiagnosticUtility.DebugAssert(wid != null, "HttpListener delivered non-Basic authenticated request with a non-Windows IIdentity."); retValue = this.ProcessAuthentication(wid); } } #pragma warning suppress 56500 // covered by FXCop catch (Exception exception) { if (DiagnosticUtility.IsFatal(exception)) throw; // Audit Authentication failure if (AuditLevel.Failure == (this.AuditBehavior.MessageAuthenticationAuditLevel & AuditLevel.Failure)) WriteAuditEvent(AuditLevel.Failure, (identity != null) ? identity.Name : ((wid != null) ? wid.Name : String.Empty), exception); throw; } // Audit Authentication success if (AuditLevel.Success == (this.AuditBehavior.MessageAuthenticationAuditLevel & AuditLevel.Success)) WriteAuditEvent(AuditLevel.Success, (identity != null) ? identity.Name : ((wid != null) ? wid.Name : String.Empty), null); return retValue; } protected override bool TryGetTransportManagerRegistration(HostNameComparisonMode hostNameComparisonMode, out ITransportManagerRegistration registration) { if (this.TransportManagerTable.TryLookupUri(this.Uri, hostNameComparisonMode, out registration)) { if (registration is HostedHttpTransportManager) { return true; } // Due to HTTP.SYS behavior, we don't reuse registrations from a higher point in the URI hierarchy. if (registration.ListenUri.Segments.Length >= this.BaseUri.Segments.Length) { return true; } } return false; } protected void WriteAuditEvent(AuditLevel auditLevel, string primaryIdentity, Exception exception) { try { if (auditLevel == AuditLevel.Success) { SecurityAuditHelper.WriteTransportAuthenticationSuccessEvent(this.AuditBehavior.AuditLogLocation, this.AuditBehavior.SuppressAuditFailure, null, this.Uri, primaryIdentity); } else { SecurityAuditHelper.WriteTransportAuthenticationFailureEvent(this.AuditBehavior.AuditLogLocation, this.AuditBehavior.SuppressAuditFailure, null, this.Uri, primaryIdentity, exception); } } #pragma warning suppress 56500 catch (Exception auditException) { if (DiagnosticUtility.IsFatal(auditException) || auditLevel == AuditLevel.Success) throw; DiagnosticUtility.ExceptionUtility.TraceHandledException(auditException, TraceEventType.Error); } } SecurityMessageProperty ProcessAuthentication(HttpListenerBasicIdentity identity) { SecurityToken securityToken = new UserNameSecurityToken(identity.Name, identity.Password); ReadOnlyCollection authorizationPolicies = this.userNameTokenAuthenticator.ValidateToken(securityToken); SecurityMessageProperty security = new SecurityMessageProperty(); security.TransportToken = new SecurityTokenSpecification(securityToken, authorizationPolicies); security.ServiceSecurityContext = new ServiceSecurityContext(authorizationPolicies); return security; } SecurityMessageProperty ProcessAuthentication(WindowsIdentity identity) { SecurityUtils.ValidateAnonymityConstraint(identity, false); SecurityToken securityToken = new WindowsSecurityToken(identity); ReadOnlyCollection authorizationPolicies = this.windowsTokenAuthenticator.ValidateToken(securityToken); SecurityMessageProperty security = new SecurityMessageProperty(); security.TransportToken = new SecurityTokenSpecification(securityToken, authorizationPolicies); security.ServiceSecurityContext = new ServiceSecurityContext(authorizationPolicies); return security; } HttpStatusCode ValidateAuthentication(string authType) { if (this.IsAuthSchemeValid(authType)) { return HttpStatusCode.OK; } else { // Audit Authentication failure if (AuditLevel.Failure == (this.AuditBehavior.MessageAuthenticationAuditLevel & AuditLevel.Failure)) { string message = SR.GetString(SR.HttpAuthenticationFailed, this.AuthenticationScheme, HttpStatusCode.Unauthorized); Exception exception = DiagnosticUtility.ExceptionUtility.ThrowHelperError(new MessageSecurityException(message)); WriteAuditEvent(AuditLevel.Failure, String.Empty, exception); } return HttpStatusCode.Unauthorized; } } public virtual HttpStatusCode ValidateAuthentication(HostedHttpRequestAsyncResult hostedAsyncResult) { HttpStatusCode result = HttpStatusCode.OK; if (this.IsAuthenticationRequired) { string authType = GetAuthType(hostedAsyncResult); result = ValidateAuthentication(authType); } return result; } public virtual HttpStatusCode ValidateAuthentication(HttpListenerContext listenerContext) { HttpStatusCode result = HttpStatusCode.OK; if (this.IsAuthenticationRequired) { string authType = GetAuthType(listenerContext); result = ValidateAuthentication(authType); } return result; } public IReplyChannel AcceptChannel() { return this.AcceptChannel(this.DefaultReceiveTimeout); } public IAsyncResult BeginAcceptChannel(AsyncCallback callback, object state) { return this.BeginAcceptChannel(this.DefaultReceiveTimeout, callback, state); } public IReplyChannel AcceptChannel(TimeSpan timeout) { base.ThrowIfNotOpened(); return acceptor.AcceptChannel(timeout); } public IAsyncResult BeginAcceptChannel(TimeSpan timeout, AsyncCallback callback, object state) { base.ThrowIfNotOpened(); return acceptor.BeginAcceptChannel(timeout, callback, state); } public IReplyChannel EndAcceptChannel(IAsyncResult result) { base.ThrowPending(); return acceptor.EndAcceptChannel(result); } protected override bool OnWaitForChannel(TimeSpan timeout) { return acceptor.WaitForChannel(timeout); } protected override IAsyncResult OnBeginWaitForChannel(TimeSpan timeout, AsyncCallback callback, object state) { return acceptor.BeginWaitForChannel(timeout, callback, state); } protected override bool OnEndWaitForChannel(IAsyncResult result) { return acceptor.EndWaitForChannel(result); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
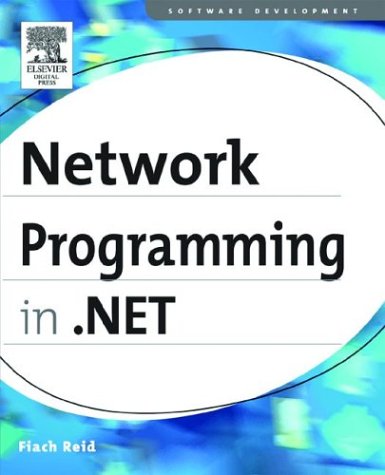
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializationAttributes.cs
- RoutedEventConverter.cs
- TypefaceCollection.cs
- JsonFormatReaderGenerator.cs
- Classification.cs
- MachineKey.cs
- ClientScriptManager.cs
- XmlSchemaCollection.cs
- SigningCredentials.cs
- TcpAppDomainProtocolHandler.cs
- XmlDataImplementation.cs
- HttpHandlerActionCollection.cs
- DesignerVerb.cs
- VBIdentifierDesigner.xaml.cs
- OperationContextScope.cs
- WebRequestModuleElement.cs
- ModulesEntry.cs
- SqlCacheDependency.cs
- OleDbEnumerator.cs
- BezierSegment.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- BitmapData.cs
- WebPartConnectVerb.cs
- ErrorRuntimeConfig.cs
- EqualityComparer.cs
- ManagedWndProcTracker.cs
- CollectionEditorDialog.cs
- srgsitem.cs
- DetailsViewPageEventArgs.cs
- XmlQueryRuntime.cs
- ReflectEventDescriptor.cs
- ModuleBuilder.cs
- SmtpTransport.cs
- SqlClientWrapperSmiStream.cs
- BufferedStream.cs
- SkinBuilder.cs
- ScrollContentPresenter.cs
- CodeTypeReference.cs
- HandledEventArgs.cs
- TemplateColumn.cs
- CollectionConverter.cs
- StringArrayConverter.cs
- SignatureHelper.cs
- EdmProviderManifest.cs
- TagPrefixInfo.cs
- UnsafeNativeMethods.cs
- Pen.cs
- WinEventHandler.cs
- CorrelationExtension.cs
- XmlNode.cs
- ModuleConfigurationInfo.cs
- LayoutSettings.cs
- DummyDataSource.cs
- OpacityConverter.cs
- XmlSchemaFacet.cs
- WebPartConnectionsCancelVerb.cs
- ReturnEventArgs.cs
- DataGridViewDataErrorEventArgs.cs
- Matrix3D.cs
- FileResponseElement.cs
- Stack.cs
- MessageQueueConverter.cs
- WebPartUtil.cs
- HScrollProperties.cs
- DataDocumentXPathNavigator.cs
- InvokeMethodDesigner.xaml.cs
- AudioBase.cs
- XpsS0ValidatingLoader.cs
- ProtocolsSection.cs
- Or.cs
- RightsManagementManager.cs
- DesignerTextBoxAdapter.cs
- DataServiceQuery.cs
- XmlExpressionDumper.cs
- ExceptionCollection.cs
- BasePattern.cs
- SplitContainer.cs
- DataGridViewSortCompareEventArgs.cs
- UInt64.cs
- ReadOnlyCollection.cs
- HelpEvent.cs
- oledbconnectionstring.cs
- LoadItemsEventArgs.cs
- XmlReturnReader.cs
- BoundColumn.cs
- StringDictionary.cs
- ToolStripPanelRenderEventArgs.cs
- FieldTemplateUserControl.cs
- brushes.cs
- Encoder.cs
- WithParamAction.cs
- SimpleMailWebEventProvider.cs
- NamespaceDisplayAutomationPeer.cs
- XamlVector3DCollectionSerializer.cs
- EventEntry.cs
- UnsafeNativeMethods.cs
- DeferredReference.cs
- ClientTargetCollection.cs
- ReachDocumentPageSerializer.cs
- GraphicsPath.cs