Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / CodeGen / StrongTypingException.cs / 1 / StrongTypingException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Collections; using System.Data; using System.Runtime.Serialization; ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class StrongTypingException : DataException { protected StrongTypingException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///DEV: The exception that is throwing from strong typed DataSet when user access to DBNull value. ////// public StrongTypingException() : base() { HResult = HResults.StrongTyping; } public StrongTypingException(string message) : base(message) { HResult = HResults.StrongTyping; } ///[To be supplied.] ////// public StrongTypingException(string s, Exception innerException) : base(s, innerException) { HResult = HResults.StrongTyping; } } ///[To be supplied.] ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class TypedDataSetGeneratorException : DataException { private ArrayList errorList; private string KEY_ARRAYCOUNT = "KEY_ARRAYCOUNT"; private string KEY_ARRAYVALUES = "KEY_ARRAYVALUES"; protected TypedDataSetGeneratorException(SerializationInfo info, StreamingContext context) : base(info, context) { int count = (int) info.GetValue(KEY_ARRAYCOUNT, typeof(System.Int32)); if (count > 0) { errorList = new ArrayList(); for (int i = 0; i < count; i++) { errorList.Add(info.GetValue(KEY_ARRAYVALUES + i, typeof(System.String))); } } else errorList = null; } ///DEV: The exception that is throwing in generating strong typed DataSet when name conflict happens. ////// public TypedDataSetGeneratorException() : base() { errorList = null; HResult = HResults.StrongTyping; } public TypedDataSetGeneratorException(string message) : base(message) { HResult = HResults.StrongTyping; } public TypedDataSetGeneratorException(string message, Exception innerException) : base(message, innerException) { HResult = HResults.StrongTyping; } ///[To be supplied.] ////// public TypedDataSetGeneratorException(ArrayList list) : this() { errorList = list; HResult = HResults.StrongTyping; } ///[To be supplied.] ////// public ArrayList ErrorList { get { return errorList; } } [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData(info, context); if (errorList != null) { info.AddValue(KEY_ARRAYCOUNT, errorList.Count); for (int i = 0; i < errorList.Count; i++) { info.AddValue(KEY_ARRAYVALUES + i, errorList[i].ToString()); } } else { info.AddValue(KEY_ARRAYCOUNT, 0); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Collections; using System.Data; using System.Runtime.Serialization; ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class StrongTypingException : DataException { protected StrongTypingException(SerializationInfo info, StreamingContext context) : base(info, context) { } ///DEV: The exception that is throwing from strong typed DataSet when user access to DBNull value. ////// public StrongTypingException() : base() { HResult = HResults.StrongTyping; } public StrongTypingException(string message) : base(message) { HResult = HResults.StrongTyping; } ///[To be supplied.] ////// public StrongTypingException(string s, Exception innerException) : base(s, innerException) { HResult = HResults.StrongTyping; } } ///[To be supplied.] ////// [Serializable] #if WINFSInternalOnly internal #else public #endif class TypedDataSetGeneratorException : DataException { private ArrayList errorList; private string KEY_ARRAYCOUNT = "KEY_ARRAYCOUNT"; private string KEY_ARRAYVALUES = "KEY_ARRAYVALUES"; protected TypedDataSetGeneratorException(SerializationInfo info, StreamingContext context) : base(info, context) { int count = (int) info.GetValue(KEY_ARRAYCOUNT, typeof(System.Int32)); if (count > 0) { errorList = new ArrayList(); for (int i = 0; i < count; i++) { errorList.Add(info.GetValue(KEY_ARRAYVALUES + i, typeof(System.String))); } } else errorList = null; } ///DEV: The exception that is throwing in generating strong typed DataSet when name conflict happens. ////// public TypedDataSetGeneratorException() : base() { errorList = null; HResult = HResults.StrongTyping; } public TypedDataSetGeneratorException(string message) : base(message) { HResult = HResults.StrongTyping; } public TypedDataSetGeneratorException(string message, Exception innerException) : base(message, innerException) { HResult = HResults.StrongTyping; } ///[To be supplied.] ////// public TypedDataSetGeneratorException(ArrayList list) : this() { errorList = list; HResult = HResults.StrongTyping; } ///[To be supplied.] ////// public ArrayList ErrorList { get { return errorList; } } [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData(info, context); if (errorList != null) { info.AddValue(KEY_ARRAYCOUNT, errorList.Count); for (int i = 0; i < errorList.Count; i++) { info.AddValue(KEY_ARRAYVALUES + i, errorList[i].ToString()); } } else { info.AddValue(KEY_ARRAYCOUNT, 0); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
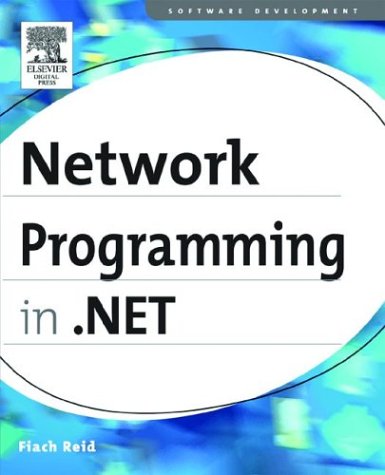
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewRowsAddedEventArgs.cs
- ResXFileRef.cs
- FixedDocumentPaginator.cs
- WeakReadOnlyCollection.cs
- AppSettingsExpressionBuilder.cs
- SqlPersonalizationProvider.cs
- KeyPressEvent.cs
- SQLSingleStorage.cs
- SynchronizationContext.cs
- TreeViewItemAutomationPeer.cs
- Base64Encoder.cs
- RSAOAEPKeyExchangeDeformatter.cs
- DisableDpiAwarenessAttribute.cs
- DataSourceCacheDurationConverter.cs
- Options.cs
- CodeConstructor.cs
- Win32Exception.cs
- CqlBlock.cs
- Focus.cs
- BuildManagerHost.cs
- AncillaryOps.cs
- SettingsContext.cs
- CollectionViewGroupRoot.cs
- DataGridViewRowStateChangedEventArgs.cs
- DataGridPageChangedEventArgs.cs
- TextDpi.cs
- UIElementCollection.cs
- OutputCacheProfileCollection.cs
- OrderByBuilder.cs
- EntityParameter.cs
- DirectoryNotFoundException.cs
- CodeCompiler.cs
- CachingHintValidation.cs
- ControlBindingsCollection.cs
- HtmlLink.cs
- AndMessageFilterTable.cs
- EntryWrittenEventArgs.cs
- SubqueryRules.cs
- Setter.cs
- SafeRegistryHandle.cs
- keycontainerpermission.cs
- SqlRecordBuffer.cs
- RootAction.cs
- Environment.cs
- DelegatingTypeDescriptionProvider.cs
- GPPOINTF.cs
- QilCloneVisitor.cs
- SimpleType.cs
- ReturnType.cs
- ScriptRef.cs
- CriticalHandle.cs
- MemberAssignmentAnalysis.cs
- Normalization.cs
- Viewport3DVisual.cs
- BuilderPropertyEntry.cs
- QueryCacheKey.cs
- SoapInteropTypes.cs
- UpdateCommand.cs
- PreservationFileWriter.cs
- StylusPointProperties.cs
- LocationUpdates.cs
- TemplateBamlTreeBuilder.cs
- XPathChildIterator.cs
- ClientFormsIdentity.cs
- SortAction.cs
- PageParser.cs
- TextComposition.cs
- SiteMembershipCondition.cs
- DateTimeUtil.cs
- DataMember.cs
- RTLAwareMessageBox.cs
- StringSorter.cs
- StringConverter.cs
- XsdCachingReader.cs
- FocusManager.cs
- bindurihelper.cs
- HttpHandlerAction.cs
- ListViewAutomationPeer.cs
- VectorCollection.cs
- UInt32.cs
- OperatingSystem.cs
- pingexception.cs
- COM2TypeInfoProcessor.cs
- XmlElementList.cs
- MonitorWrapper.cs
- TraceContext.cs
- XmlRawWriter.cs
- SQLDateTime.cs
- WeakEventTable.cs
- Visual3D.cs
- MemoryMappedViewStream.cs
- SQLGuid.cs
- HttpCacheVaryByContentEncodings.cs
- Literal.cs
- BamlWriter.cs
- SByteConverter.cs
- ProgressBar.cs
- Blend.cs
- QilGeneratorEnv.cs
- PartialCachingAttribute.cs