Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / Xml / System / Xml / Base64Encoder.cs / 2 / Base64Encoder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Text; using System.Diagnostics; namespace System.Xml { internal abstract class Base64Encoder { byte[] leftOverBytes; int leftOverBytesCount; char[] charsLine; internal const int Base64LineSize = 76; internal const int LineSizeInBytes = Base64LineSize/4*3; internal Base64Encoder() { charsLine = new char[Base64LineSize]; } internal abstract void WriteChars( char[] chars, int index, int count ); internal void Encode( byte[] buffer, int index, int count ) { if ( buffer == null ) { throw new ArgumentNullException( "buffer" ); } if ( index < 0 ) { throw new ArgumentOutOfRangeException( "index" ); } if ( count < 0 ) { throw new ArgumentOutOfRangeException( "count" ); } if ( count > buffer.Length - index ) { throw new ArgumentOutOfRangeException( "count" ); } // encode left-over buffer if( leftOverBytesCount > 0 ) { int i = leftOverBytesCount; while ( i < 3 && count > 0 ) { leftOverBytes[i++] = buffer[index++]; count--; } // the total number of buffer we have is less than 3 -> return if ( count == 0 && i < 3 ) { leftOverBytesCount = i; return; } // encode the left-over buffer and write out int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, 3, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); } // store new left-over buffer leftOverBytesCount = count % 3; if ( leftOverBytesCount > 0 ) { count -= leftOverBytesCount; if ( leftOverBytes == null ) { leftOverBytes = new byte[3]; } for( int i = 0; i < leftOverBytesCount; i++ ) { leftOverBytes[i] = buffer[ index + count + i ]; } } // encode buffer in 76 character long chunks int endIndex = index + count; int chunkSize = LineSizeInBytes; while( index < endIndex ) { if ( index + chunkSize > endIndex ) { chunkSize = endIndex - index; } int charCount = Convert.ToBase64CharArray( buffer, index, chunkSize, charsLine, 0 ); WriteChars( charsLine, 0, charCount ); index += chunkSize; } } internal void Flush() { if ( leftOverBytesCount > 0 ) { int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, leftOverBytesCount, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); leftOverBytesCount = 0; } } } internal class XmlRawWriterBase64Encoder : Base64Encoder { XmlRawWriter rawWriter; internal XmlRawWriterBase64Encoder( XmlRawWriter rawWriter ) { this.rawWriter = rawWriter; } internal override void WriteChars( char[] chars, int index, int count ) { rawWriter.WriteRaw( chars, index, count ); } } internal class XmlTextWriterBase64Encoder : Base64Encoder { XmlTextEncoder xmlTextEncoder; internal XmlTextWriterBase64Encoder( XmlTextEncoder xmlTextEncoder ) { this.xmlTextEncoder = xmlTextEncoder; } internal override void WriteChars( char[] chars, int index, int count ) { xmlTextEncoder.WriteRaw( chars, index, count ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
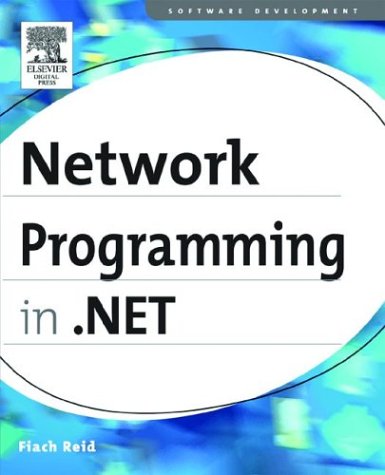
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmtpMail.cs
- HttpGetProtocolImporter.cs
- GridViewPageEventArgs.cs
- Delay.cs
- PackagingUtilities.cs
- DecoderFallbackWithFailureFlag.cs
- OutputCacheSettingsSection.cs
- DataSet.cs
- DiscoveryRequestHandler.cs
- ColorConverter.cs
- Rules.cs
- ToolStripRenderEventArgs.cs
- SvcMapFile.cs
- ToggleButton.cs
- ContextProperty.cs
- ItemDragEvent.cs
- XmlCharCheckingWriter.cs
- HttpApplicationFactory.cs
- NestedContainer.cs
- BuildProviderUtils.cs
- SpeechRecognizer.cs
- XsltArgumentList.cs
- OracleCommand.cs
- StringStorage.cs
- __FastResourceComparer.cs
- ComponentConverter.cs
- LinqDataSourceValidationException.cs
- HtmlShimManager.cs
- EntityDataSourceDesigner.cs
- PreviewPrintController.cs
- EntityDataSourceWrapper.cs
- SystemBrushes.cs
- RegexParser.cs
- COM2TypeInfoProcessor.cs
- HttpBrowserCapabilitiesWrapper.cs
- FontCollection.cs
- SafeNativeMethodsOther.cs
- ListDictionary.cs
- CommonObjectSecurity.cs
- ConstantProjectedSlot.cs
- AssemblyName.cs
- DiscardableAttribute.cs
- ProfileEventArgs.cs
- HtmlValidationSummaryAdapter.cs
- AssemblyResourceLoader.cs
- FileChangesMonitor.cs
- SchemaConstraints.cs
- KnownTypes.cs
- XmlArrayAttribute.cs
- PrimitiveXmlSerializers.cs
- Camera.cs
- StateWorkerRequest.cs
- ConfigXmlAttribute.cs
- MemberJoinTreeNode.cs
- DatePickerDateValidationErrorEventArgs.cs
- Peer.cs
- StringArrayConverter.cs
- IPPacketInformation.cs
- RotateTransform3D.cs
- AnchoredBlock.cs
- UnsafeNativeMethodsMilCoreApi.cs
- TemplateBindingExpression.cs
- XmlSiteMapProvider.cs
- Activity.cs
- ErrorEventArgs.cs
- BoundColumn.cs
- OutputCacheSection.cs
- MatrixAnimationBase.cs
- DataGridParentRows.cs
- TextProperties.cs
- XmlAttributeProperties.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- Win32SafeHandles.cs
- BindToObject.cs
- GridLengthConverter.cs
- HttpServerVarsCollection.cs
- DesignerCommandSet.cs
- XmlReader.cs
- AssemblyBuilder.cs
- ConvertersCollection.cs
- StringUtil.cs
- DbConnectionPoolCounters.cs
- CodeCompiler.cs
- ExpandButtonVisibilityConverter.cs
- StringKeyFrameCollection.cs
- DesignTimeVisibleAttribute.cs
- ChtmlSelectionListAdapter.cs
- DefaultValueConverter.cs
- NativeMethods.cs
- MatchSingleFxEngineOpcode.cs
- ServicesExceptionNotHandledEventArgs.cs
- SQLRoleProvider.cs
- ResolveRequestResponseAsyncResult.cs
- SqlTriggerAttribute.cs
- XmlNodeComparer.cs
- CookieParameter.cs
- XmlChoiceIdentifierAttribute.cs
- Point.cs
- DataSvcMapFileSerializer.cs
- GridViewRow.cs