Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / AnchoredBlock.cs / 1 / AnchoredBlock.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: AnchoredBlock element. // AnchoredBlock element - an abstract class used as a base for // TextElements incorporated (anchored) into text flow on inline level, // but appearing visually in separate block. // Derived concrete classes are: Floater and Figure. // //--------------------------------------------------------------------------- using System.ComponentModel; // TypeConverter using System.Windows.Media; // Brush using System.Windows.Markup; // ContentProperty using MS.Internal; namespace System.Windows.Documents { ////// AnchoredBlock element - an abstract class used as a base for /// TextElements incorporated (anchored) into text flow on inline level, /// but appearing visually in separate block. /// Derived concrete classes are: Flowter and Figure. /// [ContentProperty("Blocks")] public abstract class AnchoredBlock : Inline { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Creates a new AnchoredBlock instance. /// /// /// Optional child of the new AnchoredBlock, may be null. /// /// /// Optional position at which to insert the new AnchoredBlock. May /// be null. /// protected AnchoredBlock(Block block, TextPointer insertionPosition) { if (insertionPosition != null) { insertionPosition.TextContainer.BeginChange(); } try { if (insertionPosition != null) { // This will throw InvalidOperationException if schema validity is violated. insertionPosition.InsertInline(this); } if (block != null) { this.Blocks.Add(block); } } finally { if (insertionPosition != null) { insertionPosition.TextContainer.EndChange(); } } } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Collection of Blocks contained in this element /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public BlockCollection Blocks { get { return new BlockCollection(this, /*isOwnerParent*/true); } } ////// DependencyProperty for public static readonly DependencyProperty MarginProperty = Block.MarginProperty.AddOwner( typeof(AnchoredBlock), new FrameworkPropertyMetadata( new Thickness(Double.NaN), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Margin property specifies the margin of the element. /// public Thickness Margin { get { return (Thickness)GetValue(MarginProperty); } set { SetValue(MarginProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty PaddingProperty = Block.PaddingProperty.AddOwner( typeof(AnchoredBlock), new FrameworkPropertyMetadata( new Thickness(Double.NaN), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Padding property specifies the padding of the element. /// public Thickness Padding { get { return (Thickness)GetValue(PaddingProperty); } set { SetValue(PaddingProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderThicknessProperty = Block.BorderThicknessProperty.AddOwner( typeof(AnchoredBlock), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The BorderThickness property specifies the border of the element. /// public Thickness BorderThickness { get { return (Thickness)GetValue(BorderThicknessProperty); } set { SetValue(BorderThicknessProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderBrushProperty = Block.BorderBrushProperty.AddOwner( typeof(AnchoredBlock), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsRender)); ///property. /// /// The BorderBrush property specifies the brush of the border. /// public Brush BorderBrush { get { return (Brush)GetValue(BorderBrushProperty); } set { SetValue(BorderBrushProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextAlignmentProperty = Block.TextAlignmentProperty.AddOwner(typeof(AnchoredBlock)); ///property. /// /// /// public TextAlignment TextAlignment { get { return (TextAlignment)GetValue(TextAlignmentProperty); } set { SetValue(TextAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineHeightProperty = Block.LineHeightProperty.AddOwner(typeof(AnchoredBlock)); ///property. /// /// The LineHeight property specifies the height of each generated line box. /// [TypeConverter(typeof(LengthConverter))] public double LineHeight { get { return (double)GetValue(LineHeightProperty); } set { SetValue(LineHeightProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineStackingStrategyProperty = Block.LineStackingStrategyProperty.AddOwner(typeof(AnchoredBlock)); ///property. /// /// The LineStackingStrategy property specifies how lines are placed /// public LineStackingStrategy LineStackingStrategy { get { return (LineStackingStrategy)GetValue(LineStackingStrategyProperty); } set { SetValue(LineStackingStrategyProperty, value); } } #endregion Public Proterties #region Internal Methods ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeBlocks(XamlDesignerSerializationManager manager) { return manager != null && manager.XmlWriter == null; } #endregion //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: AnchoredBlock element. // AnchoredBlock element - an abstract class used as a base for // TextElements incorporated (anchored) into text flow on inline level, // but appearing visually in separate block. // Derived concrete classes are: Floater and Figure. // //--------------------------------------------------------------------------- using System.ComponentModel; // TypeConverter using System.Windows.Media; // Brush using System.Windows.Markup; // ContentProperty using MS.Internal; namespace System.Windows.Documents { ////// AnchoredBlock element - an abstract class used as a base for /// TextElements incorporated (anchored) into text flow on inline level, /// but appearing visually in separate block. /// Derived concrete classes are: Flowter and Figure. /// [ContentProperty("Blocks")] public abstract class AnchoredBlock : Inline { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Creates a new AnchoredBlock instance. /// /// /// Optional child of the new AnchoredBlock, may be null. /// /// /// Optional position at which to insert the new AnchoredBlock. May /// be null. /// protected AnchoredBlock(Block block, TextPointer insertionPosition) { if (insertionPosition != null) { insertionPosition.TextContainer.BeginChange(); } try { if (insertionPosition != null) { // This will throw InvalidOperationException if schema validity is violated. insertionPosition.InsertInline(this); } if (block != null) { this.Blocks.Add(block); } } finally { if (insertionPosition != null) { insertionPosition.TextContainer.EndChange(); } } } #endregion Constructors //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// Collection of Blocks contained in this element /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public BlockCollection Blocks { get { return new BlockCollection(this, /*isOwnerParent*/true); } } ////// DependencyProperty for public static readonly DependencyProperty MarginProperty = Block.MarginProperty.AddOwner( typeof(AnchoredBlock), new FrameworkPropertyMetadata( new Thickness(Double.NaN), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Margin property specifies the margin of the element. /// public Thickness Margin { get { return (Thickness)GetValue(MarginProperty); } set { SetValue(MarginProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty PaddingProperty = Block.PaddingProperty.AddOwner( typeof(AnchoredBlock), new FrameworkPropertyMetadata( new Thickness(Double.NaN), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Padding property specifies the padding of the element. /// public Thickness Padding { get { return (Thickness)GetValue(PaddingProperty); } set { SetValue(PaddingProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderThicknessProperty = Block.BorderThicknessProperty.AddOwner( typeof(AnchoredBlock), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The BorderThickness property specifies the border of the element. /// public Thickness BorderThickness { get { return (Thickness)GetValue(BorderThicknessProperty); } set { SetValue(BorderThicknessProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderBrushProperty = Block.BorderBrushProperty.AddOwner( typeof(AnchoredBlock), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsRender)); ///property. /// /// The BorderBrush property specifies the brush of the border. /// public Brush BorderBrush { get { return (Brush)GetValue(BorderBrushProperty); } set { SetValue(BorderBrushProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextAlignmentProperty = Block.TextAlignmentProperty.AddOwner(typeof(AnchoredBlock)); ///property. /// /// /// public TextAlignment TextAlignment { get { return (TextAlignment)GetValue(TextAlignmentProperty); } set { SetValue(TextAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineHeightProperty = Block.LineHeightProperty.AddOwner(typeof(AnchoredBlock)); ///property. /// /// The LineHeight property specifies the height of each generated line box. /// [TypeConverter(typeof(LengthConverter))] public double LineHeight { get { return (double)GetValue(LineHeightProperty); } set { SetValue(LineHeightProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineStackingStrategyProperty = Block.LineStackingStrategyProperty.AddOwner(typeof(AnchoredBlock)); ///property. /// /// The LineStackingStrategy property specifies how lines are placed /// public LineStackingStrategy LineStackingStrategy { get { return (LineStackingStrategy)GetValue(LineStackingStrategyProperty); } set { SetValue(LineStackingStrategyProperty, value); } } #endregion Public Proterties #region Internal Methods ////// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeBlocks(XamlDesignerSerializationManager manager) { return manager != null && manager.XmlWriter == null; } #endregion //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
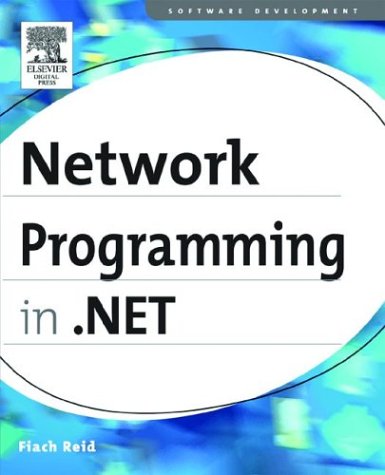
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlLinkedNode.cs
- WebPartUtil.cs
- MetadataItem.cs
- PasswordBox.cs
- MatrixTransform3D.cs
- ReadOnlyTernaryTree.cs
- XPathChildIterator.cs
- CellTreeNodeVisitors.cs
- Document.cs
- DataReceivedEventArgs.cs
- Span.cs
- PEFileEvidenceFactory.cs
- SqlDataAdapter.cs
- FileUpload.cs
- ContentTextAutomationPeer.cs
- ToolStripDropDownMenu.cs
- SHA384Managed.cs
- ConnectionPoint.cs
- BaseValidator.cs
- VisualProxy.cs
- DocumentApplicationJournalEntry.cs
- RadioButtonFlatAdapter.cs
- DrawingVisualDrawingContext.cs
- MatrixTransform3D.cs
- TypeUtils.cs
- StrokeDescriptor.cs
- SoapSchemaImporter.cs
- CapabilitiesPattern.cs
- LineGeometry.cs
- GroupBox.cs
- MetabaseServerConfig.cs
- WebAdminConfigurationHelper.cs
- RootBrowserWindowAutomationPeer.cs
- Maps.cs
- FixedFlowMap.cs
- InvalidTimeZoneException.cs
- MsmqBindingMonitor.cs
- PeerObject.cs
- ConfigXmlElement.cs
- Camera.cs
- OneToOneMappingSerializer.cs
- WmlTextViewAdapter.cs
- SafePointer.cs
- Assert.cs
- HttpWebRequestElement.cs
- PathHelper.cs
- QilTargetType.cs
- StructuralObject.cs
- CommandConverter.cs
- Positioning.cs
- HtmlTableRow.cs
- QueryPageSettingsEventArgs.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- IItemProperties.cs
- XmlExpressionDumper.cs
- RegexMatchCollection.cs
- StylusDevice.cs
- FlowDocumentFormatter.cs
- DataBoundLiteralControl.cs
- securitycriticaldataClass.cs
- TypeNameConverter.cs
- DesignerActionPanel.cs
- File.cs
- DataRelation.cs
- XmlMembersMapping.cs
- CatalogPartCollection.cs
- WsdlBuildProvider.cs
- TypeSemantics.cs
- AssemblyResolver.cs
- RequestBringIntoViewEventArgs.cs
- typedescriptorpermissionattribute.cs
- Thread.cs
- RelatedView.cs
- HttpProfileGroupBase.cs
- SerializationEventsCache.cs
- XsdDuration.cs
- WebHttpBindingCollectionElement.cs
- SubtreeProcessor.cs
- ContextDataSourceContextData.cs
- UserCancellationException.cs
- fixedPageContentExtractor.cs
- TreeView.cs
- SchemaConstraints.cs
- path.cs
- Pens.cs
- AnnotationDocumentPaginator.cs
- FunctionNode.cs
- XmlSerializerFaultFormatter.cs
- VisualProxy.cs
- BitmapEffectGeneralTransform.cs
- PlainXmlDeserializer.cs
- XmlCompatibilityReader.cs
- ContractComponent.cs
- ObjectDisposedException.cs
- DbConnectionPool.cs
- XmlIgnoreAttribute.cs
- BinaryQueryOperator.cs
- FunctionQuery.cs
- ProfileGroupSettingsCollection.cs
- XmlSchemaSimpleContentRestriction.cs