Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Objects / DataClasses / StructuralObject.cs / 4 / StructuralObject.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.ComponentModel; using System.Runtime.Serialization; namespace System.Data.Objects.DataClasses { ////// This class contains the common methods need for an date object. /// [DataContract(IsReference = true)] [Serializable] public abstract class StructuralObject : INotifyPropertyChanging, INotifyPropertyChanged { // ------ // Fields // ------ // This class contains no fields that are serialized, but it's important to realize that // adding or removing a serialized field is considered a breaking change. This includes // changing the field type or field name of existing serialized fields. If you need to make // this kind of change, it may be possible, but it will require some custom // serialization/deserialization code. ////// Public constant name used for change tracking /// Providing this definition allows users to use this constant instead of /// hard-coding the string. This helps to ensure the property name is correct /// and allows faster comparisons in places where we are looking for this specific string. /// Users can still use the case-sensitive string directly instead of the constant, /// it will just be slightly slower on comparison. /// Including the dash (-) character around the name ensures that this will not conflict with /// a real data property, because -EntityKey- is not a valid identifier name /// public static readonly string EntityKeyPropertyName = "-EntityKey-"; #region INotifyPropertyChanged Members ////// Notification that a property has been changed. /// ////// The PropertyChanged event can indicate all properties on the /// object have changed by using either a null reference /// (Nothing in Visual Basic) or String.Empty as the property name /// in the PropertyChangedEventArgs. /// [field: NonSerialized] public event PropertyChangedEventHandler PropertyChanged; #endregion #region INotifyPropertyChanging Members ////// Notification that a property is about to be changed. /// ////// The PropertyChanging event can indicate all properties on the /// object are changing by using either a null reference /// (Nothing in Visual Basic) or String.Empty as the property name /// in the PropertyChangingEventArgs. /// [field: NonSerialized] public event PropertyChangingEventHandler PropertyChanging; #endregion #region Protected Overrideable ////// Invokes the PropertyChanged event. /// /// /// The string name of the of the changed property. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Property")] protected virtual void OnPropertyChanged(string property) { if (PropertyChanged != null) { PropertyChanged.Invoke(this, new PropertyChangedEventArgs(property)); } } ////// Invokes the PropertyChanging event. /// /// /// The string name of the of the changing property. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Property")] protected virtual void OnPropertyChanging(string property) { if (PropertyChanging != null) { PropertyChanging.Invoke(this, new PropertyChangingEventArgs(property)); } } #endregion #region Protected Helper ////// The minimum DateTime value allowed in the store /// ////// The minimum DateTime value allowed in the store /// protected static DateTime DefaultDateTimeValue() { return DateTime.Now; } ////// This method is called whenever a change is going to be made to an object /// property's value. /// /// /// The name of the changing property. /// /// /// The current value of the property. /// ////// When parameter member is null (Nothing in Visual Basic). /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Property")] protected virtual void ReportPropertyChanging( string property) { EntityUtil.CheckStringArgument(property, "property"); OnPropertyChanging(property); } ////// This method is called whenever a change is made to an object /// property's value. /// /// /// The name for the changed property. /// ////// When parameter member is null (Nothing in Visual Basic). /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Property")] protected virtual void ReportPropertyChanged( string property) { EntityUtil.CheckStringArgument(property, "property"); OnPropertyChanged(property); } ////// Lazily creates a complex type if the current value is null /// ////// Unlike most of the other helper methods in this class, this one is not static /// because it references the SetValidValue for complex objects, which is also not static /// because it needs a reference to this. /// ////// Type of complex type to get a valid value for /// /// /// The current value of the complex type property /// /// /// The name of the property that is calling this method /// /// /// True if the field has already been explicitly set by the user. /// ////// The new value of the complex type property /// protected internal T GetValidValue(T currentValue, string property, bool isNullable, bool isInitialized) where T : ComplexObject, new() { // If we support complex type inheritance we will also need to check if T is abstract if (!isNullable && !isInitialized) { currentValue = SetValidValue(currentValue, new T(), property); } return currentValue; } /// /// This method is called by a ComplexObject contained in this Entity /// whenever a change is about to be made to a property of the /// ComplexObject so that the change can be forwarded to the change tracker. /// /// /// The name of the top-level entity property that contains the ComplexObject that is calling this method. /// /// /// The instance of the ComplexObject on which the property is changing. /// /// /// The name of the changing property on complexObject. /// internal abstract void ReportComplexPropertyChanging( string entityMemberName, ComplexObject complexObject, string complexMemberName); ////// This method is called by a ComplexObject contained in this Entity /// whenever a change has been made to a property of the /// ComplexObject so that the change can be forwarded to the change tracker. /// /// /// The name of the top-level entity property that contains the ComplexObject that is calling this method. /// /// /// The instance of the ComplexObject on which the property is changing. /// /// /// The name of the changing property on complexObject. /// internal abstract void ReportComplexPropertyChanged( string entityMemberName, ComplexObject complexObject, string complexMemberName); ////// Determines whether the structural object is attached to a change tracker or not /// internal abstract bool IsChangeTracked { get; } ////// Duplicates the current byte value. /// /// /// The current byte array value /// ////// Must return a copy of the values because byte arrays are mutable without providing a /// reliable mechanism for us to track changes. This allows us to treat byte arrays like /// structs which is at least a somewhat understood mechanism. /// protected internal static byte[] GetValidValue(byte[] currentValue) { if (currentValue == null) { return null; } return (byte[])currentValue.Clone(); } ////// Makes sure the Byte [] value being set for a property is valid. /// /// /// The value passed into the property setter. /// /// /// Flag indicating if this property is allowed to be null. /// ////// Returns the value if valid. /// ////// If value is null for a non nullable value. /// protected internal static Byte[] SetValidValue(Byte[] value, bool isNullable) { if (value == null) { if (!isNullable) { EntityUtil.ThrowPropertyIsNotNullable(); } return value; } return (byte[])value.Clone(); } ////// Makes sure the boolean value being set for a property is valid. /// /// /// Boolean value. /// ////// The Boolean value. /// protected internal static bool SetValidValue(bool value) { // no checks yet return value; } ////// Makes sure the boolean value being set for a property is valid. /// /// /// Boolean value /// ////// The Boolean value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the byte value being set for a property is valid. /// /// /// Byte value /// ////// The Byte value. /// protected internal static byte SetValidValue(byte value) { // no checks yet return value; } ////// Makes sure the byte value being set for a property is valid. /// /// /// Byte value /// ////// The Byte value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the sbyte value being set for a property is valid. /// /// /// sbyte value /// ////// The sbyte value. /// [CLSCompliant(false)] protected internal static sbyte SetValidValue(sbyte value) { // no checks yet return value; } ////// Makes sure the sbyte value being set for a property is valid. /// /// /// sbyte value /// ////// The sbyte value. /// [CLSCompliant(false)] protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the datetime value being set for a property is valid. /// /// /// datetime value /// ////// The datetime value. /// protected internal static DateTime SetValidValue(DateTime value) { // no checks yet return value; } ////// Makes sure the datetime value being set for a property is valid. /// /// /// datetime value /// ////// The datetime value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the timespan value being set for a property is valid. /// /// /// timespan value /// ////// The timspan value. /// protected internal static TimeSpan SetValidValue(TimeSpan value) { // no checks yet return value; } ////// Makes sure the TimeSpan value being set for a property is valid. /// /// /// timespan value /// ////// The timespan value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the datetimeoffset value being set for a property is valid. /// /// /// datetimeoffset value /// ////// The datetimeoffset value. /// protected internal static DateTimeOffset SetValidValue(DateTimeOffset value) { // no checks yet return value; } ////// Makes sure the datetimeoffset value being set for a property is valid. /// /// /// datetimeoffset value /// ////// The datetimeoffset value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Ensure that the input is a valid decimal value /// /// proposed value ///new value protected internal static Decimal SetValidValue(Decimal value) { // no checks yet return value; } ////// Ensure that the value is a valid decimal /// /// proposed value ///the new value protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the double value being set for a property is valid. /// /// /// double value /// ////// the double value /// protected internal static double SetValidValue(double value) { // no checks yet return value; } ////// Makes sure the double value being set for a property is valid. /// /// /// double value /// ////// the double value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Single value being set for a property is valid. /// /// /// float value /// ////// the folad value. /// protected internal static float SetValidValue(Single value) { // no checks yet return value; } ////// Makes sure the Single value being set for a property is valid. /// /// /// nullable Single value /// ////// the nullabel Single value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Guid value being set for a property is valid. /// /// /// Guid value /// ////// The Guid value /// protected internal static Guid SetValidValue(Guid value) { // no checks yet return value; } ////// Makes sure the Guid value being set for a property is valid. /// /// /// nullable Guid value /// ////// The nullable Guid value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Int16 value being set for a property is valid. /// /// /// Int16 value /// ////// The Int16 value /// protected internal static Int16 SetValidValue(Int16 value) { // no checks yet return value; } ////// Makes sure the Int16 value being set for a property is valid. /// /// /// nullable Int16 /// ////// The Int16 value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Int32 value being set for a property is valid. /// /// /// Int32 value /// ////// The Int32 value /// protected internal static Int32 SetValidValue(Int32 value) { // no checks yet return value; } ////// Makes sure the Int32 value being set for a property is valid. /// /// /// nullable Int32 value /// ////// The nullable Int32 protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Int64 value being set for a property is valid. /// /// /// Int64 value /// ////// The Int64 value /// protected internal static Int64 SetValidValue(Int64 value) { // no checks yet return value; } ////// Makes sure the Int64 value being set for a property is valid. /// /// /// nullable Int64 value /// ////// The nullable Int64 value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the UInt16 value being set for a property is valid. /// /// /// UInt16 value /// ////// The UInt16 value /// [CLSCompliant(false)] protected internal static UInt16 SetValidValue(UInt16 value) { // no checks yet return value; } ////// Makes sure the UInt16 value being set for a property is valid. /// /// /// nullable UInt16 value /// ////// The nullable UInt16 value /// [CLSCompliant(false)] protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the UInt32 value being set for a property is valid. /// /// /// UInt32 value /// ////// The UInt32 value /// [CLSCompliant(false)] protected internal static UInt32 SetValidValue(UInt32 value) { // no checks yet return value; } ////// Makes sure the UInt32 value being set for a property is valid. /// /// /// nullable UInt32 value /// ////// The nullable UInt32 value /// [CLSCompliant(false)] protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the UInt64 value being set for a property is valid. /// /// /// UInt64 value /// ////// The UInt64 value /// [CLSCompliant(false)] protected internal static UInt64 SetValidValue(UInt64 value) { // no checks yet return value; } ////// Makes sure the UInt64 value being set for a property is valid. /// /// /// nullable UInt64 value /// ////// The nullable UInt64 value /// [CLSCompliant(false)] protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Validates that the property is not longer than allowed, and throws if it is /// /// /// string value to be checked. /// /// /// Flag indicating if this property is allowed to be null. /// ////// The string value is null for a non-nullable string /// protected internal static string SetValidValue(string value, bool isNullable) { if (value == null) { if (!isNullable) { EntityUtil.ThrowPropertyIsNotNullable(); } } return value; } ////// Set a whole ComplexObject on an Entity or another ComplexObject /// ////// Unlike most of the other SetValidValue methods, this one is not static /// because it uses a reference to this in order to set the parent reference for the complex object. /// /// /// The current value that is set. /// /// /// The new value that will be set. /// /// /// The name of the complex type property that is being set. /// ////// The new value of the complex type property /// protected internal T SetValidValue(T oldValue, T newValue, string property) where T : ComplexObject { // Nullable complex types are not supported in v1, but we allow setting null here if the parent entity is detached if (newValue == null && IsChangeTracked) { throw EntityUtil.NullableComplexTypesNotSupported(property); } if (oldValue != null) { oldValue.DetachFromParent(); } if (newValue != null) { newValue.AttachToParent(this, property); } return newValue; } /// /// Helper method used in entity/complex object factory methods to verify that a complex object is not null /// ///Type of the complex property /// Complex object being verified /// Property name associated with this complex object ///the same complex object that was passed in, if an exception didn't occur protected internal static TComplex VerifyComplexObjectIsNotNull(TComplex complexObject, string propertyName) where TComplex : ComplexObject { if (complexObject == null) { EntityUtil.ThrowPropertyIsNotNullable(propertyName); } return complexObject; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.ComponentModel; using System.Runtime.Serialization; namespace System.Data.Objects.DataClasses { ////// This class contains the common methods need for an date object. /// [DataContract(IsReference = true)] [Serializable] public abstract class StructuralObject : INotifyPropertyChanging, INotifyPropertyChanged { // ------ // Fields // ------ // This class contains no fields that are serialized, but it's important to realize that // adding or removing a serialized field is considered a breaking change. This includes // changing the field type or field name of existing serialized fields. If you need to make // this kind of change, it may be possible, but it will require some custom // serialization/deserialization code. ////// Public constant name used for change tracking /// Providing this definition allows users to use this constant instead of /// hard-coding the string. This helps to ensure the property name is correct /// and allows faster comparisons in places where we are looking for this specific string. /// Users can still use the case-sensitive string directly instead of the constant, /// it will just be slightly slower on comparison. /// Including the dash (-) character around the name ensures that this will not conflict with /// a real data property, because -EntityKey- is not a valid identifier name /// public static readonly string EntityKeyPropertyName = "-EntityKey-"; #region INotifyPropertyChanged Members ////// Notification that a property has been changed. /// ////// The PropertyChanged event can indicate all properties on the /// object have changed by using either a null reference /// (Nothing in Visual Basic) or String.Empty as the property name /// in the PropertyChangedEventArgs. /// [field: NonSerialized] public event PropertyChangedEventHandler PropertyChanged; #endregion #region INotifyPropertyChanging Members ////// Notification that a property is about to be changed. /// ////// The PropertyChanging event can indicate all properties on the /// object are changing by using either a null reference /// (Nothing in Visual Basic) or String.Empty as the property name /// in the PropertyChangingEventArgs. /// [field: NonSerialized] public event PropertyChangingEventHandler PropertyChanging; #endregion #region Protected Overrideable ////// Invokes the PropertyChanged event. /// /// /// The string name of the of the changed property. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Property")] protected virtual void OnPropertyChanged(string property) { if (PropertyChanged != null) { PropertyChanged.Invoke(this, new PropertyChangedEventArgs(property)); } } ////// Invokes the PropertyChanging event. /// /// /// The string name of the of the changing property. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Property")] protected virtual void OnPropertyChanging(string property) { if (PropertyChanging != null) { PropertyChanging.Invoke(this, new PropertyChangingEventArgs(property)); } } #endregion #region Protected Helper ////// The minimum DateTime value allowed in the store /// ////// The minimum DateTime value allowed in the store /// protected static DateTime DefaultDateTimeValue() { return DateTime.Now; } ////// This method is called whenever a change is going to be made to an object /// property's value. /// /// /// The name of the changing property. /// /// /// The current value of the property. /// ////// When parameter member is null (Nothing in Visual Basic). /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Property")] protected virtual void ReportPropertyChanging( string property) { EntityUtil.CheckStringArgument(property, "property"); OnPropertyChanging(property); } ////// This method is called whenever a change is made to an object /// property's value. /// /// /// The name for the changed property. /// ////// When parameter member is null (Nothing in Visual Basic). /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1716:IdentifiersShouldNotMatchKeywords", MessageId = "Property")] protected virtual void ReportPropertyChanged( string property) { EntityUtil.CheckStringArgument(property, "property"); OnPropertyChanged(property); } ////// Lazily creates a complex type if the current value is null /// ////// Unlike most of the other helper methods in this class, this one is not static /// because it references the SetValidValue for complex objects, which is also not static /// because it needs a reference to this. /// ////// Type of complex type to get a valid value for /// /// /// The current value of the complex type property /// /// /// The name of the property that is calling this method /// /// /// True if the field has already been explicitly set by the user. /// ////// The new value of the complex type property /// protected internal T GetValidValue(T currentValue, string property, bool isNullable, bool isInitialized) where T : ComplexObject, new() { // If we support complex type inheritance we will also need to check if T is abstract if (!isNullable && !isInitialized) { currentValue = SetValidValue(currentValue, new T(), property); } return currentValue; } /// /// This method is called by a ComplexObject contained in this Entity /// whenever a change is about to be made to a property of the /// ComplexObject so that the change can be forwarded to the change tracker. /// /// /// The name of the top-level entity property that contains the ComplexObject that is calling this method. /// /// /// The instance of the ComplexObject on which the property is changing. /// /// /// The name of the changing property on complexObject. /// internal abstract void ReportComplexPropertyChanging( string entityMemberName, ComplexObject complexObject, string complexMemberName); ////// This method is called by a ComplexObject contained in this Entity /// whenever a change has been made to a property of the /// ComplexObject so that the change can be forwarded to the change tracker. /// /// /// The name of the top-level entity property that contains the ComplexObject that is calling this method. /// /// /// The instance of the ComplexObject on which the property is changing. /// /// /// The name of the changing property on complexObject. /// internal abstract void ReportComplexPropertyChanged( string entityMemberName, ComplexObject complexObject, string complexMemberName); ////// Determines whether the structural object is attached to a change tracker or not /// internal abstract bool IsChangeTracked { get; } ////// Duplicates the current byte value. /// /// /// The current byte array value /// ////// Must return a copy of the values because byte arrays are mutable without providing a /// reliable mechanism for us to track changes. This allows us to treat byte arrays like /// structs which is at least a somewhat understood mechanism. /// protected internal static byte[] GetValidValue(byte[] currentValue) { if (currentValue == null) { return null; } return (byte[])currentValue.Clone(); } ////// Makes sure the Byte [] value being set for a property is valid. /// /// /// The value passed into the property setter. /// /// /// Flag indicating if this property is allowed to be null. /// ////// Returns the value if valid. /// ////// If value is null for a non nullable value. /// protected internal static Byte[] SetValidValue(Byte[] value, bool isNullable) { if (value == null) { if (!isNullable) { EntityUtil.ThrowPropertyIsNotNullable(); } return value; } return (byte[])value.Clone(); } ////// Makes sure the boolean value being set for a property is valid. /// /// /// Boolean value. /// ////// The Boolean value. /// protected internal static bool SetValidValue(bool value) { // no checks yet return value; } ////// Makes sure the boolean value being set for a property is valid. /// /// /// Boolean value /// ////// The Boolean value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the byte value being set for a property is valid. /// /// /// Byte value /// ////// The Byte value. /// protected internal static byte SetValidValue(byte value) { // no checks yet return value; } ////// Makes sure the byte value being set for a property is valid. /// /// /// Byte value /// ////// The Byte value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the sbyte value being set for a property is valid. /// /// /// sbyte value /// ////// The sbyte value. /// [CLSCompliant(false)] protected internal static sbyte SetValidValue(sbyte value) { // no checks yet return value; } ////// Makes sure the sbyte value being set for a property is valid. /// /// /// sbyte value /// ////// The sbyte value. /// [CLSCompliant(false)] protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the datetime value being set for a property is valid. /// /// /// datetime value /// ////// The datetime value. /// protected internal static DateTime SetValidValue(DateTime value) { // no checks yet return value; } ////// Makes sure the datetime value being set for a property is valid. /// /// /// datetime value /// ////// The datetime value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the timespan value being set for a property is valid. /// /// /// timespan value /// ////// The timspan value. /// protected internal static TimeSpan SetValidValue(TimeSpan value) { // no checks yet return value; } ////// Makes sure the TimeSpan value being set for a property is valid. /// /// /// timespan value /// ////// The timespan value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the datetimeoffset value being set for a property is valid. /// /// /// datetimeoffset value /// ////// The datetimeoffset value. /// protected internal static DateTimeOffset SetValidValue(DateTimeOffset value) { // no checks yet return value; } ////// Makes sure the datetimeoffset value being set for a property is valid. /// /// /// datetimeoffset value /// ////// The datetimeoffset value. /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Ensure that the input is a valid decimal value /// /// proposed value ///new value protected internal static Decimal SetValidValue(Decimal value) { // no checks yet return value; } ////// Ensure that the value is a valid decimal /// /// proposed value ///the new value protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the double value being set for a property is valid. /// /// /// double value /// ////// the double value /// protected internal static double SetValidValue(double value) { // no checks yet return value; } ////// Makes sure the double value being set for a property is valid. /// /// /// double value /// ////// the double value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Single value being set for a property is valid. /// /// /// float value /// ////// the folad value. /// protected internal static float SetValidValue(Single value) { // no checks yet return value; } ////// Makes sure the Single value being set for a property is valid. /// /// /// nullable Single value /// ////// the nullabel Single value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Guid value being set for a property is valid. /// /// /// Guid value /// ////// The Guid value /// protected internal static Guid SetValidValue(Guid value) { // no checks yet return value; } ////// Makes sure the Guid value being set for a property is valid. /// /// /// nullable Guid value /// ////// The nullable Guid value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Int16 value being set for a property is valid. /// /// /// Int16 value /// ////// The Int16 value /// protected internal static Int16 SetValidValue(Int16 value) { // no checks yet return value; } ////// Makes sure the Int16 value being set for a property is valid. /// /// /// nullable Int16 /// ////// The Int16 value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Int32 value being set for a property is valid. /// /// /// Int32 value /// ////// The Int32 value /// protected internal static Int32 SetValidValue(Int32 value) { // no checks yet return value; } ////// Makes sure the Int32 value being set for a property is valid. /// /// /// nullable Int32 value /// ////// The nullable Int32 protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the Int64 value being set for a property is valid. /// /// /// Int64 value /// ////// The Int64 value /// protected internal static Int64 SetValidValue(Int64 value) { // no checks yet return value; } ////// Makes sure the Int64 value being set for a property is valid. /// /// /// nullable Int64 value /// ////// The nullable Int64 value /// protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the UInt16 value being set for a property is valid. /// /// /// UInt16 value /// ////// The UInt16 value /// [CLSCompliant(false)] protected internal static UInt16 SetValidValue(UInt16 value) { // no checks yet return value; } ////// Makes sure the UInt16 value being set for a property is valid. /// /// /// nullable UInt16 value /// ////// The nullable UInt16 value /// [CLSCompliant(false)] protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the UInt32 value being set for a property is valid. /// /// /// UInt32 value /// ////// The UInt32 value /// [CLSCompliant(false)] protected internal static UInt32 SetValidValue(UInt32 value) { // no checks yet return value; } ////// Makes sure the UInt32 value being set for a property is valid. /// /// /// nullable UInt32 value /// ////// The nullable UInt32 value /// [CLSCompliant(false)] protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Makes sure the UInt64 value being set for a property is valid. /// /// /// UInt64 value /// ////// The UInt64 value /// [CLSCompliant(false)] protected internal static UInt64 SetValidValue(UInt64 value) { // no checks yet return value; } ////// Makes sure the UInt64 value being set for a property is valid. /// /// /// nullable UInt64 value /// ////// The nullable UInt64 value /// [CLSCompliant(false)] protected internal static NullableSetValidValue(Nullable value) { // no checks yet return value; } /// /// Validates that the property is not longer than allowed, and throws if it is /// /// /// string value to be checked. /// /// /// Flag indicating if this property is allowed to be null. /// ////// The string value is null for a non-nullable string /// protected internal static string SetValidValue(string value, bool isNullable) { if (value == null) { if (!isNullable) { EntityUtil.ThrowPropertyIsNotNullable(); } } return value; } ////// Set a whole ComplexObject on an Entity or another ComplexObject /// ////// Unlike most of the other SetValidValue methods, this one is not static /// because it uses a reference to this in order to set the parent reference for the complex object. /// /// /// The current value that is set. /// /// /// The new value that will be set. /// /// /// The name of the complex type property that is being set. /// ////// The new value of the complex type property /// protected internal T SetValidValue(T oldValue, T newValue, string property) where T : ComplexObject { // Nullable complex types are not supported in v1, but we allow setting null here if the parent entity is detached if (newValue == null && IsChangeTracked) { throw EntityUtil.NullableComplexTypesNotSupported(property); } if (oldValue != null) { oldValue.DetachFromParent(); } if (newValue != null) { newValue.AttachToParent(this, property); } return newValue; } /// /// Helper method used in entity/complex object factory methods to verify that a complex object is not null /// ///Type of the complex property /// Complex object being verified /// Property name associated with this complex object ///the same complex object that was passed in, if an exception didn't occur protected internal static TComplex VerifyComplexObjectIsNotNull(TComplex complexObject, string propertyName) where TComplex : ComplexObject { if (complexObject == null) { EntityUtil.ThrowPropertyIsNotNullable(propertyName); } return complexObject; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
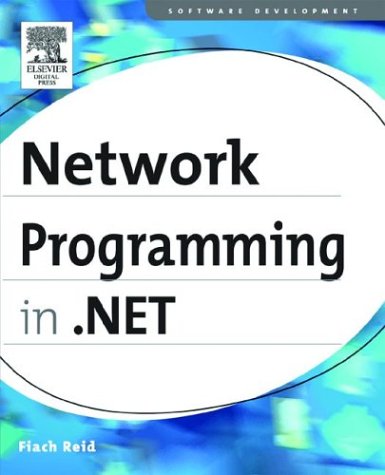
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaMapping.cs
- BitmapSourceSafeMILHandle.cs
- ObjectComplexPropertyMapping.cs
- SmtpDigestAuthenticationModule.cs
- SQLInt32Storage.cs
- ReflectionHelper.cs
- TickBar.cs
- RootBrowserWindow.cs
- CodeDefaultValueExpression.cs
- CalendarDataBindingHandler.cs
- TransformerInfo.cs
- ResourceSetExpression.cs
- WindowsListViewItem.cs
- ElementMarkupObject.cs
- CatalogPartCollection.cs
- DataSourceXmlSerializationAttribute.cs
- StrokeNodeEnumerator.cs
- UITypeEditors.cs
- CompositeFontParser.cs
- Ppl.cs
- ParserContext.cs
- Simplifier.cs
- DataTransferEventArgs.cs
- UncommonField.cs
- SubpageParaClient.cs
- ListenerAdapterBase.cs
- SourceChangedEventArgs.cs
- ResourceExpression.cs
- CallbackTimeoutsBehavior.cs
- SystemIcmpV4Statistics.cs
- GenericXmlSecurityToken.cs
- MaskedTextBoxTextEditorDropDown.cs
- SqlTypesSchemaImporter.cs
- ExceptionHelpers.cs
- UriSchemeKeyedCollection.cs
- OutputCacheSettingsSection.cs
- ThemeConfigurationDialog.cs
- CustomError.cs
- GraphicsState.cs
- SByteConverter.cs
- WindowsFormsEditorServiceHelper.cs
- CancelRequestedQuery.cs
- ListView.cs
- SymbolDocumentGenerator.cs
- BindingsCollection.cs
- RepeaterItemEventArgs.cs
- PinnedBufferMemoryStream.cs
- ApplicationGesture.cs
- HtmlInputPassword.cs
- MultitargetUtil.cs
- XmlRootAttribute.cs
- Sql8ExpressionRewriter.cs
- ProcessExitedException.cs
- GridPattern.cs
- SynchronousChannel.cs
- XmlDocumentType.cs
- DataQuery.cs
- ZipIOLocalFileHeader.cs
- MultiDataTrigger.cs
- Activator.cs
- EpmContentSerializerBase.cs
- ComplexTypeEmitter.cs
- IgnoreFlushAndCloseStream.cs
- QilVisitor.cs
- SqlNodeTypeOperators.cs
- SecurityCriticalDataForSet.cs
- ExpandedWrapper.cs
- SiteMapHierarchicalDataSourceView.cs
- ConstructorArgumentAttribute.cs
- BCLDebug.cs
- SchemaImporterExtensionsSection.cs
- XPathPatternBuilder.cs
- BooleanAnimationBase.cs
- ScriptResourceHandler.cs
- __Filters.cs
- BrowserDefinitionCollection.cs
- _NetworkingPerfCounters.cs
- ping.cs
- CheckPair.cs
- GcHandle.cs
- GlobalEventManager.cs
- SqlDataSourceCache.cs
- DataGridViewCheckBoxColumn.cs
- XmlIlTypeHelper.cs
- SoapInteropTypes.cs
- TextTreeFixupNode.cs
- ClientScriptItem.cs
- ScriptResourceAttribute.cs
- WindowsAuthenticationEventArgs.cs
- _Semaphore.cs
- ObjectHelper.cs
- SmiConnection.cs
- PropertyMappingExceptionEventArgs.cs
- DependencySource.cs
- Base64Encoder.cs
- DbXmlEnabledProviderManifest.cs
- OperandQuery.cs
- SafeEventLogReadHandle.cs
- ToolStripItem.cs
- WeakReferenceKey.cs