Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Epm / EpmContentSerializerBase.cs / 1305376 / EpmContentSerializerBase.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Base Classes used for EntityPropertyMappingAttribute related content // serializers // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { using System.Collections.Generic; using System.Diagnostics; using System.Linq; #if ASTORIA_CLIENT using System.Xml; #else using System.ServiceModel.Syndication; using System.Data.Services.Providers; #endif ////// What kind of xml nodes to serialize /// internal enum EpmSerializationKind { ////// Serialize only attributes /// Attributes, ////// Serialize only elements /// Elements, ////// Serialize everything /// All } ////// Base visitor class for performing serialization of content whose location in the syndication /// feed is provided through EntityPropertyMappingAttributes /// internal abstract class EpmContentSerializerBase { ////// Constructor decided whether to use syndication or non-syndication sub-tree for target content mappings /// /// Target tree containing mapping information /// Helps in deciding whether to use syndication sub-tree or non-syndication one /// Object to be serialized /// SyndicationItem to which content will be added #if ASTORIA_CLIENT protected EpmContentSerializerBase(EpmTargetTree tree, bool isSyndication, object element, XmlWriter target) #else protected EpmContentSerializerBase(EpmTargetTree tree, bool isSyndication, object element, SyndicationItem target) #endif { this.Root = isSyndication ? tree.SyndicationRoot : tree.NonSyndicationRoot; this.Element = element; this.Target = target; this.Success = false; } ///Root of the target tree containing mapped xml elements/attribute protected EpmTargetPathSegment Root { get; private set; } ///Object whose properties we will read protected object Element { get; private set; } ///Target SyndicationItem on which we are going to add the serialized content #if ASTORIA_CLIENT protected XmlWriter Target #else protected SyndicationItem Target #endif { get; private set; } ///Indicates the success or failure of serialization protected bool Success { get; private set; } #if ASTORIA_CLIENT ///Public interface used by the EpmContentSerializer class internal void Serialize() #else ///Public interface used by the EpmContentSerializer class /// Data Service provider used for rights verification. internal void Serialize(DataServiceProviderWrapper provider) #endif { foreach (EpmTargetPathSegment targetSegment in this.Root.SubSegments) { #if ASTORIA_CLIENT this.Serialize(targetSegment, EpmSerializationKind.All); #else this.Serialize(targetSegment, EpmSerializationKind.All, provider); #endif } this.Success = true; } #if ASTORIA_CLIENT ////// Internal interface to be overridden in the subclasses. /// Goes through each subsegments and invokes itself for the children /// /// Current root segment in the target tree /// Which sub segments to serialize protected virtual void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind) #else ////// Internal interface to be overridden in the subclasses. /// Goes through each subsegments and invokes itself for the children /// /// Current root segment in the target tree /// Which sub segments to serialize /// Data Service provider used for rights verification. protected virtual void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind, DataServiceProviderWrapper provider) #endif { IEnumerablesegmentsToSerialize; switch (kind) { case EpmSerializationKind.Attributes: segmentsToSerialize = targetSegment.SubSegments.Where(s => s.IsAttribute == true); break; case EpmSerializationKind.Elements: segmentsToSerialize = targetSegment.SubSegments.Where(s => s.IsAttribute == false); break; default: Debug.Assert(kind == EpmSerializationKind.All, "Must serialize everything"); segmentsToSerialize = targetSegment.SubSegments; break; } foreach (EpmTargetPathSegment segment in segmentsToSerialize) { #if ASTORIA_CLIENT this.Serialize(segment, kind); #else this.Serialize(segment, kind, provider); #endif } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// Base Classes used for EntityPropertyMappingAttribute related content // serializers // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { using System.Collections.Generic; using System.Diagnostics; using System.Linq; #if ASTORIA_CLIENT using System.Xml; #else using System.ServiceModel.Syndication; using System.Data.Services.Providers; #endif ////// What kind of xml nodes to serialize /// internal enum EpmSerializationKind { ////// Serialize only attributes /// Attributes, ////// Serialize only elements /// Elements, ////// Serialize everything /// All } ////// Base visitor class for performing serialization of content whose location in the syndication /// feed is provided through EntityPropertyMappingAttributes /// internal abstract class EpmContentSerializerBase { ////// Constructor decided whether to use syndication or non-syndication sub-tree for target content mappings /// /// Target tree containing mapping information /// Helps in deciding whether to use syndication sub-tree or non-syndication one /// Object to be serialized /// SyndicationItem to which content will be added #if ASTORIA_CLIENT protected EpmContentSerializerBase(EpmTargetTree tree, bool isSyndication, object element, XmlWriter target) #else protected EpmContentSerializerBase(EpmTargetTree tree, bool isSyndication, object element, SyndicationItem target) #endif { this.Root = isSyndication ? tree.SyndicationRoot : tree.NonSyndicationRoot; this.Element = element; this.Target = target; this.Success = false; } ///Root of the target tree containing mapped xml elements/attribute protected EpmTargetPathSegment Root { get; private set; } ///Object whose properties we will read protected object Element { get; private set; } ///Target SyndicationItem on which we are going to add the serialized content #if ASTORIA_CLIENT protected XmlWriter Target #else protected SyndicationItem Target #endif { get; private set; } ///Indicates the success or failure of serialization protected bool Success { get; private set; } #if ASTORIA_CLIENT ///Public interface used by the EpmContentSerializer class internal void Serialize() #else ///Public interface used by the EpmContentSerializer class /// Data Service provider used for rights verification. internal void Serialize(DataServiceProviderWrapper provider) #endif { foreach (EpmTargetPathSegment targetSegment in this.Root.SubSegments) { #if ASTORIA_CLIENT this.Serialize(targetSegment, EpmSerializationKind.All); #else this.Serialize(targetSegment, EpmSerializationKind.All, provider); #endif } this.Success = true; } #if ASTORIA_CLIENT ////// Internal interface to be overridden in the subclasses. /// Goes through each subsegments and invokes itself for the children /// /// Current root segment in the target tree /// Which sub segments to serialize protected virtual void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind) #else ////// Internal interface to be overridden in the subclasses. /// Goes through each subsegments and invokes itself for the children /// /// Current root segment in the target tree /// Which sub segments to serialize /// Data Service provider used for rights verification. protected virtual void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind, DataServiceProviderWrapper provider) #endif { IEnumerablesegmentsToSerialize; switch (kind) { case EpmSerializationKind.Attributes: segmentsToSerialize = targetSegment.SubSegments.Where(s => s.IsAttribute == true); break; case EpmSerializationKind.Elements: segmentsToSerialize = targetSegment.SubSegments.Where(s => s.IsAttribute == false); break; default: Debug.Assert(kind == EpmSerializationKind.All, "Must serialize everything"); segmentsToSerialize = targetSegment.SubSegments; break; } foreach (EpmTargetPathSegment segment in segmentsToSerialize) { #if ASTORIA_CLIENT this.Serialize(segment, kind); #else this.Serialize(segment, kind, provider); #endif } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
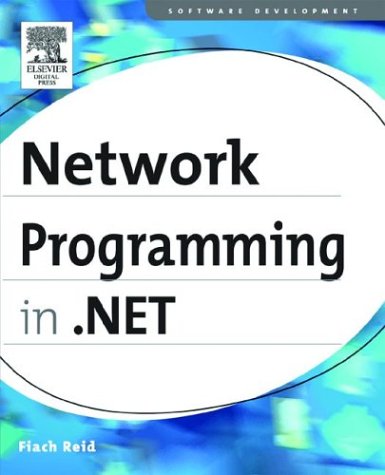
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbConvert.cs
- XmlObjectSerializerWriteContextComplex.cs
- DashStyle.cs
- ObfuscationAttribute.cs
- AddingNewEventArgs.cs
- AccessKeyManager.cs
- WmpBitmapEncoder.cs
- DateTimeConstantAttribute.cs
- FirstMatchCodeGroup.cs
- ConfigurationProviderException.cs
- PathGeometry.cs
- MemberRestriction.cs
- ImageClickEventArgs.cs
- WindowsServiceElement.cs
- DataGridViewRow.cs
- PlanCompiler.cs
- LinqTreeNodeEvaluator.cs
- DataObjectAttribute.cs
- DataGridViewHeaderCell.cs
- UrlPath.cs
- DirtyTextRange.cs
- TreeNodeSelectionProcessor.cs
- StringCollection.cs
- EntityTypeEmitter.cs
- DynamicMetaObjectBinder.cs
- URLMembershipCondition.cs
- Rijndael.cs
- TextRunCacheImp.cs
- AlphabeticalEnumConverter.cs
- DetailsViewInsertEventArgs.cs
- UpdatePanel.cs
- QueryComponents.cs
- WebZoneDesigner.cs
- ExceptionHandler.cs
- httpapplicationstate.cs
- ResourceDescriptionAttribute.cs
- HostingEnvironmentException.cs
- ElementHost.cs
- SoapAttributeOverrides.cs
- DataGridAutoFormat.cs
- SHA512CryptoServiceProvider.cs
- SlipBehavior.cs
- DataSourceSerializationException.cs
- ProcessRequestArgs.cs
- OleServicesContext.cs
- LassoSelectionBehavior.cs
- DataGridView.cs
- Cursor.cs
- DataGridColumn.cs
- WebPart.cs
- IPEndPointCollection.cs
- GroupItem.cs
- BrushConverter.cs
- ZipIOLocalFileDataDescriptor.cs
- SQLGuidStorage.cs
- ObjectStateFormatter.cs
- EdmValidator.cs
- LowerCaseStringConverter.cs
- NamespaceList.cs
- BamlLocalizer.cs
- Int32Collection.cs
- TransformPatternIdentifiers.cs
- Literal.cs
- CodeLabeledStatement.cs
- LinqDataSourceSelectEventArgs.cs
- ConditionalExpression.cs
- PrintDialogException.cs
- DataFormats.cs
- CompilationRelaxations.cs
- PageBreakRecord.cs
- SqlTrackingQuery.cs
- DefaultSection.cs
- CompositeCollectionView.cs
- ApplicationServiceHelper.cs
- ProxyWebPartConnectionCollection.cs
- ServiceInstanceProvider.cs
- Int32Converter.cs
- SplitContainer.cs
- QueryServiceConfigHandle.cs
- TCPClient.cs
- IODescriptionAttribute.cs
- BuildTopDownAttribute.cs
- DeadCharTextComposition.cs
- OleDbTransaction.cs
- cryptoapiTransform.cs
- MouseWheelEventArgs.cs
- HiddenFieldPageStatePersister.cs
- WebPartConnectionsEventArgs.cs
- GenericsNotImplementedException.cs
- MILUtilities.cs
- TextWriter.cs
- AutomationTextAttribute.cs
- InstanceDataCollection.cs
- ContextMenu.cs
- SHA1.cs
- EditorPart.cs
- PseudoWebRequest.cs
- TreeIterator.cs
- SiteMapHierarchicalDataSourceView.cs
- MenuCommand.cs