Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / Design / MenuCommand.cs / 1 / MenuCommand.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel.Design { using Microsoft.Win32; using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] [System.Runtime.InteropServices.ComVisible(true)] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class MenuCommand { // Events that we suface or call on // private EventHandler execHandler; private EventHandler statusHandler; private CommandID commandID; private int status; private IDictionary properties; ////// Represents a Windows /// menu or toolbar item. /// ////// Indicates that the given command is enabled. An enabled command may /// be selected by the user (it's not greyed out). /// private const int ENABLED = 0x02; //tagOLECMDF.OLECMDF_ENABLED; ////// Indicates that the given command is not visible on the command bar. /// private const int INVISIBLE = 0x10; ////// Indicates that the given command is checked in the "on" state. /// private const int CHECKED = 0x04; // tagOLECMDF.OLECMDF_LATCHED; ////// Indicates that the given command is supported. Marking a command /// as supported indicates that the shell will not look any further up /// the command target chain. /// private const int SUPPORTED = 0x01; // tagOLECMDF.OLECMDF_SUPPORTED ////// public MenuCommand(EventHandler handler, CommandID command) { this.execHandler = handler; this.commandID = command; this.status = SUPPORTED | ENABLED; } ////// Initializes a new instance of ///. /// /// public virtual bool Checked { get { return (status & CHECKED) != 0; } set { SetStatus(CHECKED, value); } } ///Gets or sets a value indicating whether this menu item is checked. ////// public virtual bool Enabled { get{ return (status & ENABLED) != 0; } set { SetStatus(ENABLED, value); } } private void SetStatus(int mask, bool value){ int newStatus = status; if (value) { newStatus |= mask; } else { newStatus &= ~mask; } if (newStatus != status) { status = newStatus; OnCommandChanged(EventArgs.Empty); } } ///Gets or /// sets a value indicating whether this menu item is available. ////// public virtual IDictionary Properties { get { if (properties == null) { properties = new HybridDictionary(); } return properties; } } ////// public virtual bool Supported { get{ return (status & SUPPORTED) != 0; } set{ SetStatus(SUPPORTED, value); } } ///Gets or sets a value /// indicating whether this menu item is supported. ////// public virtual bool Visible { get { return (status & INVISIBLE) == 0; } set { SetStatus(INVISIBLE, !value); } } ///Gets or sets a value /// indicating if this menu item is visible. ////// public event EventHandler CommandChanged { add { statusHandler += value; } remove { statusHandler -= value; } } ////// Occurs when the menu command changes. /// ////// public virtual CommandID CommandID { get { return commandID; } } ///Gets the ///associated with this menu command. /// public virtual void Invoke() { if (execHandler != null){ try { execHandler(this, EventArgs.Empty); } catch (CheckoutException cxe) { if (cxe == CheckoutException.Canceled) return; throw; } } } ////// Invokes a menu item. /// ////// public virtual void Invoke(object arg) { Invoke(); } ////// Invokes a menu item. The default implementation of this method ignores /// the argument, but deriving classes may override this method. /// ////// public virtual int OleStatus { get { return status; } } ////// Gets the OLE command status code for this menu item. /// ////// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] // Safe: FullTrust LinkDemand to instantiate an object of this class. protected virtual void OnCommandChanged(EventArgs e) { if (statusHandler != null) { statusHandler(this, e); } } ///Provides notification and is called in response to /// a ///event. /// Overrides object's ToString(). /// public override string ToString() { string str = commandID.ToString() + " : "; if ((status & SUPPORTED) != 0) { str += "Supported"; } if ((status & ENABLED) != 0) { str += "|Enabled"; } if ((status & INVISIBLE) == 0) { str += "|Visible"; } if ((status & CHECKED) != 0) { str += "|Checked"; } return str; } } }
Link Menu
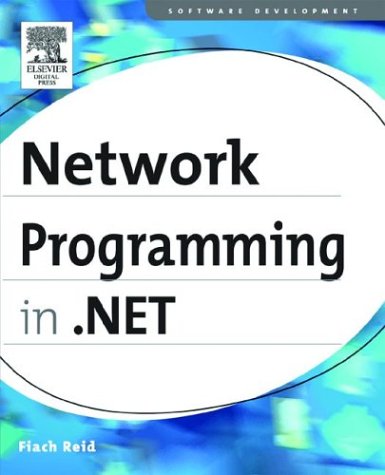
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeAttributeArgumentCollection.cs
- Int64AnimationUsingKeyFrames.cs
- ImageAutomationPeer.cs
- DeferredTextReference.cs
- OleDbParameter.cs
- MasterPageParser.cs
- HideDisabledControlAdapter.cs
- SettingsPropertyNotFoundException.cs
- AssemblyResourceLoader.cs
- SchemaCollectionCompiler.cs
- GridViewRowCollection.cs
- HitTestResult.cs
- HttpValueCollection.cs
- ListViewItemSelectionChangedEvent.cs
- DataProtectionSecurityStateEncoder.cs
- PropertySegmentSerializationProvider.cs
- NotificationContext.cs
- NumberFunctions.cs
- DataSet.cs
- XmlSchemaCompilationSettings.cs
- ReliableRequestSessionChannel.cs
- InputProcessorProfilesLoader.cs
- CodeSubDirectory.cs
- EntitySetRetriever.cs
- filewebrequest.cs
- DataGridViewToolTip.cs
- IdentityManager.cs
- TextViewSelectionProcessor.cs
- ColumnMapCopier.cs
- CopyNamespacesAction.cs
- BindingSource.cs
- SoapExtension.cs
- XamlNamespaceHelper.cs
- ErrorReporting.cs
- ParserHooks.cs
- InstallerTypeAttribute.cs
- XamlToRtfParser.cs
- InvalidPropValue.cs
- AndCondition.cs
- TriState.cs
- securitymgrsite.cs
- DllHostInitializer.cs
- XmlSecureResolver.cs
- TextFormatterHost.cs
- PeerCustomResolverBindingElement.cs
- TextSerializer.cs
- NamespaceImport.cs
- ProviderIncompatibleException.cs
- ContextStack.cs
- WebConfigurationManager.cs
- ColorContextHelper.cs
- ThreadPool.cs
- VScrollProperties.cs
- PropertyMappingExceptionEventArgs.cs
- ApplicationInterop.cs
- InputReport.cs
- WorkflowRequestContext.cs
- PreDigestedSignedInfo.cs
- ToolStripItem.cs
- DataSourceControl.cs
- MediaPlayerState.cs
- EntityCommandCompilationException.cs
- ModulesEntry.cs
- ReceiveActivity.cs
- MediaEntryAttribute.cs
- QuadTree.cs
- SchemaNames.cs
- TraceHandler.cs
- ItemsChangedEventArgs.cs
- InvalidPropValue.cs
- WindowsEditBox.cs
- TextSelectionProcessor.cs
- NumericUpDownAcceleration.cs
- ConfigurationLocationCollection.cs
- XmlPreloadedResolver.cs
- TransformerConfigurationWizardBase.cs
- OutOfMemoryException.cs
- RegisteredArrayDeclaration.cs
- TableCell.cs
- BuildProvidersCompiler.cs
- BitStack.cs
- XPathDocumentNavigator.cs
- StyleSheetDesigner.cs
- StylusEditingBehavior.cs
- DesignTimeVisibleAttribute.cs
- EnumUnknown.cs
- StylusSystemGestureEventArgs.cs
- SqlSupersetValidator.cs
- CheckBoxPopupAdapter.cs
- SqlMethodAttribute.cs
- CompositeControl.cs
- ImageButton.cs
- SqlServer2KCompatibilityCheck.cs
- BlockUIContainer.cs
- CurrentChangingEventManager.cs
- WpfPayload.cs
- ArrayList.cs
- CodeCompiler.cs
- LocalFileSettingsProvider.cs
- ListBase.cs