Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / ErrorReporting.cs / 1305376 / ErrorReporting.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Activities.Presentation { using System.Activities.Presentation.Internal.PropertyEditing; using System.Activities.Presentation.View; using System.Diagnostics; using System.Runtime; using System.Text; using System.Threading; using System.Windows; using System.Windows.Controls; using System.Windows.Input; using System.Globalization; using System.Diagnostics.CodeAnalysis; [Fx.Tag.XamlVisible(false)] static class ErrorReporting { public static DesignerView ActiveDesignerView { get; set; } public static void ShowErrorMessage(string message) { ShowErrorMessage(message, false); } public static void ShowAlertMessage(string message) { ShowAlertMessage(message, false); } public static void ShowErrorMessage(string message, bool includeStackTrace) { string stackTrace = null; if (includeStackTrace) { //generate stack trace stackTrace = new StackTrace().ToString(); //remove top frame from the trace (which is a call to ShowErrorMessage) stackTrace = stackTrace.Remove(0, stackTrace.IndexOf(Environment.NewLine, StringComparison.Ordinal)+1); } ShowMessageBox(message, MessageBoxImage.Error, stackTrace); } public static void ShowAlertMessage(string message, bool includeStackTrace) { string stackTrace = null; if (includeStackTrace) { //generate stack trace stackTrace = new StackTrace().ToString(); //remove top frame from the trace (which is a call to ShowAlertMessage) stackTrace = stackTrace.Remove(0, stackTrace.IndexOf(Environment.NewLine, StringComparison.Ordinal) + 1); } ShowMessageBox(message, MessageBoxImage.Warning, stackTrace); } public static void ShowErrorMessage(Exception err) { if (null != err) { ShowMessageBox(string.Format(CultureInfo.InvariantCulture, "{0}:\r\n{1}", err.GetType().Name, err.Message), MessageBoxImage.Error, err.StackTrace); } } static void ShowMessageBox(string message, MessageBoxImage icon, string stackTrace) { //determine an icon string iconName = icon == MessageBoxImage.Error ? "TextBoxErrorIcon" : icon == MessageBoxImage.Warning ? "WarningValidationIcon" : string.Empty; //set properties var dlg = new ErrorDialog() { ErrorDescription = message ?? "", Icon = EditorResources.GetIcons()[iconName], StackTrace = stackTrace, StackTraceVisibility = string.IsNullOrEmpty(stackTrace) ? Visibility.Collapsed : Visibility.Visible, Context = null != ActiveDesignerView ? ActiveDesignerView.Context : null, Owner = ActiveDesignerView, }; //show error window dlg.Show(); } sealed class ErrorDialog : WorkflowElementDialog { public string ErrorDescription { get; set;} public Visibility StackTraceVisibility { get; set; } public string StackTrace { get; set; } [SuppressMessage(FxCop.Category.Performance, FxCop.Rule.AvoidUncalledPrivateCode, Justification = "This property is accessed by XAML")] public object Icon { get; set; } protected override void OnInitialized(EventArgs e) { this.Title = SR.WorkflowDesignerErrorPresenterTitle; this.Content = new ContentPresenter() { ContentTemplate = (DataTemplate)EditorResources.GetResources()["ErrorPresenterDialogTemplate"], Content = this, }; this.MinWidth = 365; this.WindowResizeMode = ResizeMode.NoResize; this.WindowSizeToContent = SizeToContent.WidthAndHeight; //handle loaded event this.Loaded += this.OnDialogWindowLoaded; base.OnInitialized(e); } void OnDialogWindowLoaded(object s, RoutedEventArgs e) { //get the containing window var parentWindow = VisualTreeUtils.FindVisualAncestor (this); //and handle KeyDown event - in case of Esc, we should close the dialog parentWindow.KeyDown += OnWindowKeyDown; //add Copy command support - when user presses Ctrl+C, copy content of the error into clipboard var copyBinding = new CommandBinding(ApplicationCommands.Copy); copyBinding.PreviewCanExecute += OnCopyCanExecute; copyBinding.Executed += OnCopyExecuted; parentWindow.CommandBindings.Add(copyBinding); } void OnWindowKeyDown(object s, KeyEventArgs e) { //Esc - close the dialog box if (e.Key == Key.Escape) { ((Window)s).DialogResult = false; e.Handled = true; } } void OnCopyCanExecute(object s, CanExecuteRoutedEventArgs e) { //do not allow text boxes to handle the ApplicationCommand.Copy, i will handle it myself e.CanExecute = true; e.ContinueRouting = false; e.Handled = true; } void OnCopyExecuted(object s, ExecutedRoutedEventArgs e) { //build a string with detailed error description StringBuilder error = new StringBuilder(); error.Append('-', 25); error.Append(Environment.NewLine); error.Append(this.Title); error.Append(Environment.NewLine); error.Append('-', 25); error.Append(Environment.NewLine); error.Append(this.ErrorDescription); error.Append(Environment.NewLine); if (this.StackTraceVisibility == Visibility.Visible) { error.Append('-', 25); error.Append(Environment.NewLine); error.Append(this.StackTrace); error.Append(Environment.NewLine); } error.Append('-', 25); error.Append(Environment.NewLine); string result = error.ToString(); //attempt to set the value into clipboard - according to MSDN - if a call fails, it means some other process is accessing clipboard //so sleep and retry for (int i = 0; i < 10; ++i) { try { Clipboard.SetText(result); break; } catch (Exception err) { if (Fx.IsFatal(err)) { throw; } Thread.Sleep(50); } } e.Handled = true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
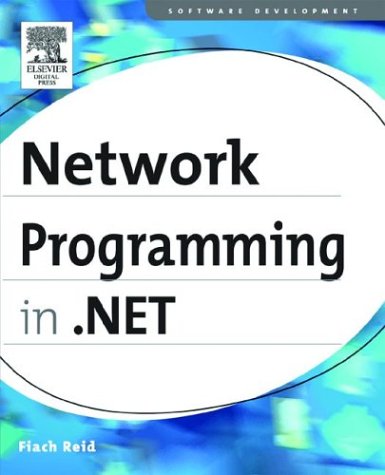
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CacheEntry.cs
- NavigationPropertyEmitter.cs
- BindingExpression.cs
- GridViewRowCollection.cs
- ConstraintCollection.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- LinearQuaternionKeyFrame.cs
- DataGridViewAdvancedBorderStyle.cs
- NavigationPropertySingletonExpression.cs
- ScriptingProfileServiceSection.cs
- TableColumn.cs
- SaveFileDialog.cs
- NegationPusher.cs
- LabelLiteral.cs
- ImageFormat.cs
- XmlSerializableReader.cs
- DbDataReader.cs
- MatrixCamera.cs
- Image.cs
- TemplateControl.cs
- AccessibilityApplicationManager.cs
- PropertyManager.cs
- Enumerable.cs
- PrintDocument.cs
- FloaterParaClient.cs
- InvalidFilterCriteriaException.cs
- COM2Properties.cs
- HtmlDocument.cs
- DbProviderSpecificTypePropertyAttribute.cs
- IsolatedStorageException.cs
- KnownBoxes.cs
- FormatterServicesNoSerializableCheck.cs
- HtmlInputText.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- RtType.cs
- _FtpDataStream.cs
- ChildTable.cs
- XmlSchemaAnnotation.cs
- SimpleWorkerRequest.cs
- MetadataItem_Static.cs
- CodePrimitiveExpression.cs
- PaintEvent.cs
- ParserExtension.cs
- ListenerConstants.cs
- RadioButton.cs
- CalendarDataBindingHandler.cs
- TableLayoutColumnStyleCollection.cs
- DrawingAttributeSerializer.cs
- RegexCaptureCollection.cs
- baseaxisquery.cs
- hwndwrapper.cs
- MsmqHostedTransportManager.cs
- MemberPath.cs
- ValidationRuleCollection.cs
- VsPropertyGrid.cs
- Baml2006KnownTypes.cs
- ListViewGroupConverter.cs
- MailAddressCollection.cs
- Set.cs
- TimeoutStream.cs
- Expr.cs
- Rotation3DAnimation.cs
- DataServiceRequestException.cs
- storepermission.cs
- ExpressionVisitorHelpers.cs
- GacUtil.cs
- TagNameToTypeMapper.cs
- Stopwatch.cs
- MD5.cs
- TableItemProviderWrapper.cs
- Model3DCollection.cs
- SqlMethodTransformer.cs
- StaticDataManager.cs
- LayoutTableCell.cs
- DSASignatureFormatter.cs
- VarRefManager.cs
- DataGridViewCellFormattingEventArgs.cs
- PaperSize.cs
- DocumentSignatureManager.cs
- TTSVoice.cs
- ExtractorMetadata.cs
- VectorAnimation.cs
- ShaperBuffers.cs
- EntityDataSourceEntityTypeFilterItem.cs
- CustomAssemblyResolver.cs
- HwndSourceKeyboardInputSite.cs
- DbReferenceCollection.cs
- ApplicationManager.cs
- ValidatorCollection.cs
- SecurityChannelFaultConverter.cs
- RuleSettingsCollection.cs
- ScriptMethodAttribute.cs
- Char.cs
- DataGridViewComboBoxCell.cs
- InstanceOwnerException.cs
- MessagePropertyFilter.cs
- SqlProcedureAttribute.cs
- DataControlField.cs
- NumberFunctions.cs
- DataGridRelationshipRow.cs