Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Hosting / SimpleWorkerRequest.cs / 1 / SimpleWorkerRequest.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Hosting { using System.Collections; using System.IO; using System.Runtime.InteropServices; using System.Security.Principal; using System.Security; using System.Security.Permissions; using System.Threading; using System.Web.Configuration; using System.Web.Util; // // Simple Worker Request provides a concrete implementation // of HttpWorkerRequest that writes the respone to the user // supplied writer. // ////// [ComVisible(false)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class SimpleWorkerRequest : HttpWorkerRequest { private bool _hasRuntimeInfo; private String _appVirtPath; // "/foo" private String _appPhysPath; // "c:\foo\" private String _page; private String _pathInfo; private String _queryString; private TextWriter _output; private String _installDir; private void ExtractPagePathInfo() { int i = _page.IndexOf('/'); if (i >= 0) { _pathInfo = _page.Substring(i); _page = _page.Substring(0, i); } } private String GetPathInternal(bool includePathInfo) { String s = _appVirtPath.Equals("/") ? ("/" + _page) : (_appVirtPath + "/" + _page); if (includePathInfo && _pathInfo != null) return s + _pathInfo; else return s; } // // HttpWorkerRequest implementation // // "/foo/page.aspx/tail" ///[To be supplied.] ////// public override String GetUriPath() { return GetPathInternal(true); } // "param=bar" ///[To be supplied.] ////// public override String GetQueryString() { return _queryString; } // "/foo/page.aspx/tail?param=bar" ///[To be supplied.] ////// public override String GetRawUrl() { String qs = GetQueryString(); if (!String.IsNullOrEmpty(qs)) return GetPathInternal(true) + "?" + qs; else return GetPathInternal(true); } ///[To be supplied.] ////// public override String GetHttpVerbName() { return "GET"; } ///[To be supplied.] ////// public override String GetHttpVersion() { return "HTTP/1.0"; } ///[To be supplied.] ////// public override String GetRemoteAddress() { return "127.0.0.1"; } ///[To be supplied.] ////// public override int GetRemotePort() { return 0; } ///[To be supplied.] ////// public override String GetLocalAddress() { return "127.0.0.1"; } ///[To be supplied.] ////// public override int GetLocalPort() { return 80; } ///[To be supplied.] ////// public override IntPtr GetUserToken() { return IntPtr.Zero; } ///[To be supplied.] ////// public override String GetFilePath() { return GetPathInternal(false); } ///[To be supplied.] ////// public override String GetFilePathTranslated() { String path = _appPhysPath + _page.Replace('/', '\\'); InternalSecurityPermissions.PathDiscovery(path).Demand(); return path; } ///[To be supplied.] ////// public override String GetPathInfo() { return (_pathInfo != null) ? _pathInfo : String.Empty; } ///[To be supplied.] ////// public override String GetAppPath() { return _appVirtPath; } ///[To be supplied.] ////// public override String GetAppPathTranslated() { InternalSecurityPermissions.PathDiscovery(_appPhysPath).Demand(); return _appPhysPath; } ///[To be supplied.] ////// public override String GetServerVariable(String name) { return String.Empty; } ///[To be supplied.] ////// public override String MapPath(String path) { if (!_hasRuntimeInfo) return null; String mappedPath = null; String appPath = _appPhysPath.Substring(0, _appPhysPath.Length-1); // without trailing "\" if (String.IsNullOrEmpty(path) || path.Equals("/")) { mappedPath = appPath; } if (StringUtil.StringStartsWith(path, _appVirtPath)) { mappedPath = appPath + path.Substring(_appVirtPath.Length).Replace('/', '\\'); } InternalSecurityPermissions.PathDiscovery(mappedPath).Demand(); return mappedPath; } ///[To be supplied.] ////// public override string MachineConfigPath { get { if (_hasRuntimeInfo) { string path = HttpConfigurationSystem.MachineConfigurationFilePath; InternalSecurityPermissions.PathDiscovery(path).Demand(); return path; } else return null; } } ///[To be supplied.] ////// public override string RootWebConfigPath { get { if (_hasRuntimeInfo) { string path = HttpConfigurationSystem.RootWebConfigurationFilePath; InternalSecurityPermissions.PathDiscovery(path).Demand(); return path; } else return null; } } ///[To be supplied.] ////// public override String MachineInstallDirectory { get { if (_hasRuntimeInfo) { InternalSecurityPermissions.PathDiscovery(_installDir).Demand(); return _installDir; } return null; } } ///[To be supplied.] ////// public override void SendStatus(int statusCode, String statusDescription) { } ///[To be supplied.] ////// public override void SendKnownResponseHeader(int index, String value) { } ///[To be supplied.] ////// public override void SendUnknownResponseHeader(String name, String value) { } ///[To be supplied.] ////// public override void SendResponseFromMemory(byte[] data, int length) { _output.Write(System.Text.Encoding.Default.GetChars(data, 0, length)); } ///[To be supplied.] ////// public override void SendResponseFromFile(String filename, long offset, long length) { } ///[To be supplied.] ////// public override void SendResponseFromFile(IntPtr handle, long offset, long length) { } ///[To be supplied.] ////// public override void FlushResponse(bool finalFlush) { } ///[To be supplied.] ////// public override void EndOfRequest() { } // // Ctors // private SimpleWorkerRequest() { } /* * Ctor that gets application data from HttpRuntime, assuming * HttpRuntime has been set up (app domain specially created, etc.) */ ///[To be supplied.] ////// [SecurityPermission(SecurityAction.Demand, Unrestricted = true)] public SimpleWorkerRequest(String page, String query, TextWriter output) { _queryString = query; _output = output; _page = page; ExtractPagePathInfo(); _appPhysPath = Thread.GetDomain().GetData(".appPath").ToString(); _appVirtPath = Thread.GetDomain().GetData(".appVPath").ToString(); _installDir = HttpRuntime.AspInstallDirectoryInternal; _hasRuntimeInfo = true; } /* * Ctor that gets application data as arguments,assuming HttpRuntime * has not been set up. * * This allows for limited functionality to execute handlers. */ ///[To be supplied.] ////// [SecurityPermission(SecurityAction.Demand, Unrestricted = true)] public SimpleWorkerRequest(String appVirtualDir, String appPhysicalDir, String page, String query, TextWriter output) { if (Thread.GetDomain().GetData(".appPath") != null) { throw new HttpException(SR.GetString(SR.Wrong_SimpleWorkerRequest)); } _appVirtPath = appVirtualDir; _appPhysPath = appPhysicalDir; _queryString = query; _output = output; _page = page; ExtractPagePathInfo(); if (!StringUtil.StringEndsWith(_appPhysPath, '\\')) _appPhysPath += "\\"; _hasRuntimeInfo = false; } } }[To be supplied.] ///
Link Menu
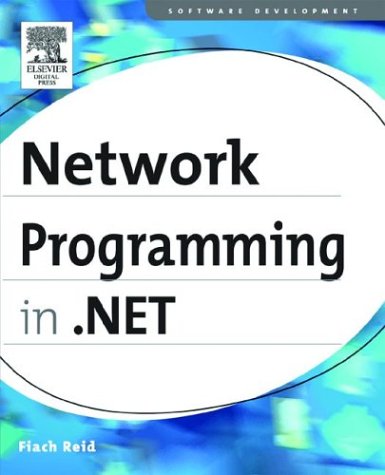
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectivityStatus.cs
- SchemaElementLookUpTableEnumerator.cs
- BmpBitmapDecoder.cs
- ConsumerConnectionPoint.cs
- BinaryConverter.cs
- ParameterModifier.cs
- DllNotFoundException.cs
- StorageConditionPropertyMapping.cs
- URLIdentityPermission.cs
- VariantWrapper.cs
- SpecularMaterial.cs
- MatrixAnimationUsingKeyFrames.cs
- DbDataReader.cs
- ValidationPropertyAttribute.cs
- ContainerCodeDomSerializer.cs
- RTLAwareMessageBox.cs
- SelectedGridItemChangedEvent.cs
- LockRecoveryTask.cs
- XmlAggregates.cs
- HashCoreRequest.cs
- WindowsEditBox.cs
- RSAProtectedConfigurationProvider.cs
- EmbeddedMailObjectsCollection.cs
- WebControlParameterProxy.cs
- BaseParser.cs
- _Semaphore.cs
- XPathExpr.cs
- CaseCqlBlock.cs
- Assert.cs
- EntitySetBaseCollection.cs
- StoreItemCollection.cs
- XmlSchemaSimpleContentExtension.cs
- SupportsPreviewControlAttribute.cs
- NativeMethods.cs
- Mapping.cs
- SslSecurityTokenParameters.cs
- ITextView.cs
- TrimSurroundingWhitespaceAttribute.cs
- ArrayItemValue.cs
- DefaultValueAttribute.cs
- WebServiceAttribute.cs
- PrePrepareMethodAttribute.cs
- TypeTypeConverter.cs
- HttpApplication.cs
- CqlParserHelpers.cs
- BufferedWebEventProvider.cs
- _NestedMultipleAsyncResult.cs
- ResizeBehavior.cs
- EtwTrace.cs
- CleanUpVirtualizedItemEventArgs.cs
- DataGridCaption.cs
- AnnotationHighlightLayer.cs
- WinFormsComponentEditor.cs
- Speller.cs
- ConnectionManagementSection.cs
- ProfileService.cs
- WMIGenerator.cs
- ValueSerializer.cs
- StandardToolWindows.cs
- ElementProxy.cs
- PropertyPathConverter.cs
- XmlQualifiedName.cs
- RoleGroupCollection.cs
- MDIClient.cs
- AuthorizationSection.cs
- Char.cs
- SerializationObjectManager.cs
- RecommendedAsConfigurableAttribute.cs
- ReversePositionQuery.cs
- WebPartExportVerb.cs
- TextFindEngine.cs
- ScaleTransform3D.cs
- X509WindowsSecurityToken.cs
- controlskin.cs
- EntityDataSourceState.cs
- AddInToken.cs
- ClrPerspective.cs
- Pts.cs
- WindowsScrollBarBits.cs
- PointLightBase.cs
- SettingsSection.cs
- PrimitiveOperationFormatter.cs
- RuleSet.cs
- SQLGuidStorage.cs
- StylusOverProperty.cs
- KernelTypeValidation.cs
- Page.cs
- Section.cs
- AttachInfo.cs
- PresentationUIStyleResources.cs
- GlyphShapingProperties.cs
- MessageSecurityProtocol.cs
- SqlClientPermission.cs
- WinInet.cs
- TileBrush.cs
- ToolStripDropTargetManager.cs
- ContextStaticAttribute.cs
- XmlSchemaGroup.cs
- HostUtils.cs
- HtmlButton.cs