Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWebControls / System / Data / WebControls / WebControlParameterProxy.cs / 1 / WebControlParameterProxy.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner objsdev //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Web.UI.WebControls; using System.Diagnostics; using System.Data; using System.Globalization; namespace System.Web.UI.WebControls { internal class WebControlParameterProxy { ParameterCollection _collection; EntityDataSource _entityDataSource; Parameter _parameter; // Can be null, that's why this class doesn't subclass Parameter internal WebControlParameterProxy(string propertyName, ParameterCollection parameterCollection, EntityDataSource entityDataSource) { Debug.Assert(null != entityDataSource); Debug.Assert(!String.IsNullOrEmpty(propertyName)); _parameter = EntityDataSourceUtil.GetParameter(propertyName, parameterCollection); _collection = parameterCollection; _entityDataSource = entityDataSource; VerifyUniqueType(_parameter); } internal WebControlParameterProxy(Parameter parameter, ParameterCollection parameterCollection, EntityDataSource entityDataSource) { Debug.Assert(null != entityDataSource); _parameter = parameter; _collection = parameterCollection; _entityDataSource = entityDataSource; VerifyUniqueType(_parameter); } internal string Name { get { if (null != _parameter) { return _parameter.Name; } return null; } } internal bool HasValue { get { return null != _parameter && null != Value; } } internal bool ConvertEmptyStringToNull { get { if (null != _parameter) { return _parameter.ConvertEmptyStringToNull; } return false; } } internal TypeCode TypeCode { get { if (null != _parameter) { return _parameter.Type; } return TypeCode.Empty; } } internal DbType DbType { get { if (null != _parameter) { return _parameter.DbType; } return DbType.Object; } } internal Type ClrType { get { Debug.Assert(this.TypeCode != TypeCode.Empty || this.DbType != DbType.Object, "Need to have TypeCode or DbType to get a ClrType"); if (this.TypeCode != TypeCode.Empty) { return EntityDataSourceUtil.ConvertTypeCodeToType(this.TypeCode); } return EntityDataSourceUtil.ConvertTypeCodeToType( EntityDataSourceUtil.ConvertDbTypeToTypeCode(this.DbType)); } } internal object Value { get { if (_parameter != null) { object paramValue = EntityDataSourceUtil.GetParameterValue(_parameter.Name, _collection, _entityDataSource); if (paramValue != null) { if (this.DbType == DbType.DateTimeOffset) { object value = (paramValue is DateTimeOffset) ? paramValue : DateTimeOffset.Parse(this.Value.ToString(), CultureInfo.CurrentCulture); return value; } else if (this.DbType == DbType.Time) { object value = (paramValue is TimeSpan) ? paramValue : TimeSpan.Parse(paramValue.ToString()); return value; } else if (this.DbType == DbType.Guid) { object value = (paramValue is Guid) ? paramValue : new Guid(paramValue.ToString()); return value; } } return paramValue; } return null; } } private static void VerifyUniqueType(Parameter parameter) { if (parameter != null && parameter.Type == TypeCode.Empty && parameter.DbType == DbType.Object) { throw new InvalidOperationException(Strings.WebControlParameterProxy_TypeDbTypeMutuallyExclusive); } if (parameter != null && parameter.DbType != DbType.Object && parameter.Type != TypeCode.Empty) { throw new InvalidOperationException(Strings.WebControlParameterProxy_TypeDbTypeMutuallyExclusive); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner objsdev //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Web.UI.WebControls; using System.Diagnostics; using System.Data; using System.Globalization; namespace System.Web.UI.WebControls { internal class WebControlParameterProxy { ParameterCollection _collection; EntityDataSource _entityDataSource; Parameter _parameter; // Can be null, that's why this class doesn't subclass Parameter internal WebControlParameterProxy(string propertyName, ParameterCollection parameterCollection, EntityDataSource entityDataSource) { Debug.Assert(null != entityDataSource); Debug.Assert(!String.IsNullOrEmpty(propertyName)); _parameter = EntityDataSourceUtil.GetParameter(propertyName, parameterCollection); _collection = parameterCollection; _entityDataSource = entityDataSource; VerifyUniqueType(_parameter); } internal WebControlParameterProxy(Parameter parameter, ParameterCollection parameterCollection, EntityDataSource entityDataSource) { Debug.Assert(null != entityDataSource); _parameter = parameter; _collection = parameterCollection; _entityDataSource = entityDataSource; VerifyUniqueType(_parameter); } internal string Name { get { if (null != _parameter) { return _parameter.Name; } return null; } } internal bool HasValue { get { return null != _parameter && null != Value; } } internal bool ConvertEmptyStringToNull { get { if (null != _parameter) { return _parameter.ConvertEmptyStringToNull; } return false; } } internal TypeCode TypeCode { get { if (null != _parameter) { return _parameter.Type; } return TypeCode.Empty; } } internal DbType DbType { get { if (null != _parameter) { return _parameter.DbType; } return DbType.Object; } } internal Type ClrType { get { Debug.Assert(this.TypeCode != TypeCode.Empty || this.DbType != DbType.Object, "Need to have TypeCode or DbType to get a ClrType"); if (this.TypeCode != TypeCode.Empty) { return EntityDataSourceUtil.ConvertTypeCodeToType(this.TypeCode); } return EntityDataSourceUtil.ConvertTypeCodeToType( EntityDataSourceUtil.ConvertDbTypeToTypeCode(this.DbType)); } } internal object Value { get { if (_parameter != null) { object paramValue = EntityDataSourceUtil.GetParameterValue(_parameter.Name, _collection, _entityDataSource); if (paramValue != null) { if (this.DbType == DbType.DateTimeOffset) { object value = (paramValue is DateTimeOffset) ? paramValue : DateTimeOffset.Parse(this.Value.ToString(), CultureInfo.CurrentCulture); return value; } else if (this.DbType == DbType.Time) { object value = (paramValue is TimeSpan) ? paramValue : TimeSpan.Parse(paramValue.ToString()); return value; } else if (this.DbType == DbType.Guid) { object value = (paramValue is Guid) ? paramValue : new Guid(paramValue.ToString()); return value; } } return paramValue; } return null; } } private static void VerifyUniqueType(Parameter parameter) { if (parameter != null && parameter.Type == TypeCode.Empty && parameter.DbType == DbType.Object) { throw new InvalidOperationException(Strings.WebControlParameterProxy_TypeDbTypeMutuallyExclusive); } if (parameter != null && parameter.DbType != DbType.Object && parameter.Type != TypeCode.Empty) { throw new InvalidOperationException(Strings.WebControlParameterProxy_TypeDbTypeMutuallyExclusive); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
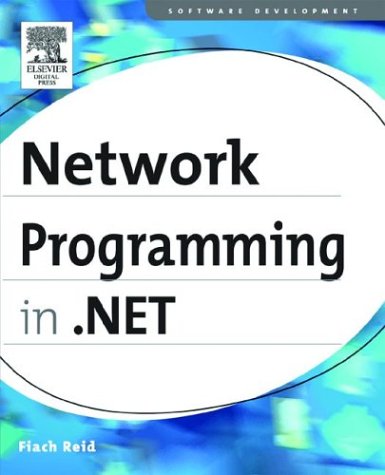
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputElement.cs
- OperationExecutionFault.cs
- ConfigurationManagerHelper.cs
- BoundField.cs
- FileDialogPermission.cs
- Stream.cs
- AnnotationElement.cs
- Activator.cs
- ElementsClipboardData.cs
- CompositeActivityTypeDescriptorProvider.cs
- DependsOnAttribute.cs
- SqlUserDefinedAggregateAttribute.cs
- HeaderUtility.cs
- MutableAssemblyCacheEntry.cs
- SignerInfo.cs
- CodeTypeParameterCollection.cs
- WorkflowShape.cs
- SynthesizerStateChangedEventArgs.cs
- DataGridLinkButton.cs
- DeviceContexts.cs
- SiteMapProvider.cs
- HyperlinkAutomationPeer.cs
- Processor.cs
- TogglePattern.cs
- NetworkInformationPermission.cs
- SQLSingle.cs
- SqlFormatter.cs
- CodeGenerator.cs
- SecurityTokenRequirement.cs
- IProducerConsumerCollection.cs
- FormViewInsertEventArgs.cs
- LinkConverter.cs
- AssemblyName.cs
- ComplexBindingPropertiesAttribute.cs
- Logging.cs
- InvalidProgramException.cs
- XmlSubtreeReader.cs
- Transform.cs
- ThrowHelper.cs
- EventManager.cs
- WindowsSecurityToken.cs
- WebPartTracker.cs
- Coordinator.cs
- MemberDescriptor.cs
- TextEditorSpelling.cs
- StrongTypingException.cs
- AuthenticationManager.cs
- PairComparer.cs
- TextEditorSelection.cs
- HostDesigntimeLicenseContext.cs
- AnonymousIdentificationSection.cs
- SRGSCompiler.cs
- Interop.cs
- Int32CollectionConverter.cs
- NodeInfo.cs
- KeyValueSerializer.cs
- GradientBrush.cs
- ObjectConverter.cs
- XmlProcessingInstruction.cs
- DeviceSpecificDesigner.cs
- LoginName.cs
- CngKeyCreationParameters.cs
- MsmqDiagnostics.cs
- X509UI.cs
- MultilineStringEditor.cs
- DataGridTableCollection.cs
- SimpleColumnProvider.cs
- CodeObject.cs
- ItemsPresenter.cs
- ListenerUnsafeNativeMethods.cs
- CodeTryCatchFinallyStatement.cs
- CircleHotSpot.cs
- Misc.cs
- SafeRightsManagementEnvironmentHandle.cs
- TemplatedWizardStep.cs
- ModuleBuilder.cs
- Pair.cs
- WebPartEventArgs.cs
- DecoratedNameAttribute.cs
- NamedPipeConnectionPoolSettingsElement.cs
- DoubleLinkList.cs
- WebPartMovingEventArgs.cs
- PrintPageEvent.cs
- XmlTypeMapping.cs
- ColumnBinding.cs
- TextServicesDisplayAttributePropertyRanges.cs
- RSAOAEPKeyExchangeFormatter.cs
- WsatStrings.cs
- IndexedString.cs
- MemberBinding.cs
- DateTimeStorage.cs
- RegistrySecurity.cs
- XamlToRtfWriter.cs
- PackagePartCollection.cs
- Path.cs
- DbMetaDataCollectionNames.cs
- XsdBuildProvider.cs
- DesignerWebPartChrome.cs
- InkSerializer.cs
- WindowsRichEdit.cs