Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / CngKeyCreationParameters.cs / 1305376 / CngKeyCreationParameters.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Security; using System.Security.Permissions; using System.Diagnostics.Contracts; namespace System.Security.Cryptography { ////// Settings to be applied to a CNG key before it is finalized. /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CngKeyCreationParameters { private CngExportPolicies? m_exportPolicy; private CngKeyCreationOptions m_keyCreationOptions; private CngKeyUsages? m_keyUsage; private CngPropertyCollection m_parameters = new CngPropertyCollection(); private IntPtr m_parentWindowHandle; private CngProvider m_provider = CngProvider.MicrosoftSoftwareKeyStorageProvider; private CngUIPolicy m_uiPolicy; ////// How many times can this key be exported from the KSP /// public CngExportPolicies? ExportPolicy { get { return m_exportPolicy; } set { m_exportPolicy = value; } } ////// Flags controlling how to create the key /// public CngKeyCreationOptions KeyCreationOptions { get { return m_keyCreationOptions; } set { m_keyCreationOptions = value; } } ////// Which cryptographic operations are valid for use with this key /// public CngKeyUsages? KeyUsage { get { return m_keyUsage; } set { m_keyUsage = value; } } ////// Window handle to use as the parent for the dialog shown when the key is created /// public IntPtr ParentWindowHandle { get { return m_parentWindowHandle; } [SecurityPermission(SecurityAction.Demand, UnmanagedCode = true)] set { m_parentWindowHandle = value; } } ////// Extra parameter values to set before the key is finalized /// public CngPropertyCollection Parameters { [SecurityPermission(SecurityAction.Demand, UnmanagedCode = true)] get { Contract.Ensures(Contract.Result() != null); return m_parameters; } } /// /// Internal access to the parameters method without a demand /// internal CngPropertyCollection ParametersNoDemand { get { Contract.Ensures(Contract.Result() != null); return m_parameters; } } /// /// KSP to create the key in /// public CngProvider Provider { get { Contract.Ensures(Contract.Result() != null); return m_provider; } set { if (value == null) { throw new ArgumentNullException("value"); } m_provider = value; } } /// /// Settings for UI shown on access to the key /// public CngUIPolicy UIPolicy { get { return m_uiPolicy; } [HostProtection(Action = SecurityAction.Demand, UI = true)] [UIPermission(SecurityAction.Demand, Window = UIPermissionWindow.SafeSubWindows)] set { m_uiPolicy = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
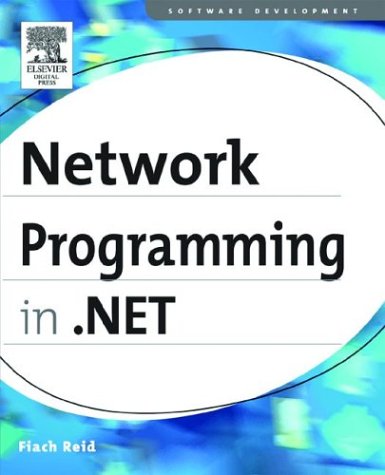
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WSDualHttpSecurity.cs
- FrameworkContentElement.cs
- RotationValidation.cs
- SecurityTokenSerializer.cs
- BufferBuilder.cs
- KeyEventArgs.cs
- GZipStream.cs
- UserMapPath.cs
- Lease.cs
- IdentityVerifier.cs
- RpcAsyncResult.cs
- DataControlCommands.cs
- DateTimeStorage.cs
- CodeExporter.cs
- ScriptRegistrationManager.cs
- ObjectStorage.cs
- XmlQuerySequence.cs
- BindingCompleteEventArgs.cs
- DecoderBestFitFallback.cs
- ColumnReorderedEventArgs.cs
- BindingGroup.cs
- SqlDataSourceSelectingEventArgs.cs
- Point4DConverter.cs
- SqlDependencyUtils.cs
- JumpItem.cs
- MetadataCache.cs
- ReadContentAsBinaryHelper.cs
- ValidationErrorCollection.cs
- SR.cs
- Int32RectValueSerializer.cs
- WebException.cs
- JpegBitmapEncoder.cs
- SelectedCellsChangedEventArgs.cs
- ConfigXmlWhitespace.cs
- SoapTypeAttribute.cs
- SizeAnimation.cs
- MenuItemAutomationPeer.cs
- OciEnlistContext.cs
- WebBrowsableAttribute.cs
- DynamicPropertyHolder.cs
- ImageMap.cs
- RangeValueProviderWrapper.cs
- X509SecurityTokenAuthenticator.cs
- ParserOptions.cs
- RoleBoolean.cs
- UIntPtr.cs
- TypedDataSourceCodeGenerator.cs
- ZoomingMessageFilter.cs
- NativeActivity.cs
- SqlInternalConnection.cs
- SqlFunctionAttribute.cs
- ErrorHandler.cs
- DebuggerAttributes.cs
- IPipelineRuntime.cs
- ConstraintStruct.cs
- WebResourceAttribute.cs
- BindingOperations.cs
- DisplayInformation.cs
- Attribute.cs
- ConstantProjectedSlot.cs
- InstanceView.cs
- ExpressionWriter.cs
- DbConnectionOptions.cs
- PerformanceCounter.cs
- RootBrowserWindowProxy.cs
- Size.cs
- MatrixConverter.cs
- SqlDataSourceQueryEditor.cs
- Permission.cs
- MimeWriter.cs
- WindowExtensionMethods.cs
- SystemUnicastIPAddressInformation.cs
- ToolboxItemAttribute.cs
- SiteMapPath.cs
- UpdateTranslator.cs
- Transform.cs
- CheckBox.cs
- CqlErrorHelper.cs
- CapabilitiesPattern.cs
- DragEvent.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- UIElementHelper.cs
- LeftCellWrapper.cs
- SocketAddress.cs
- DefaultEventAttribute.cs
- RequestBringIntoViewEventArgs.cs
- _NTAuthentication.cs
- BamlRecordWriter.cs
- DocumentPageHost.cs
- ValueSerializer.cs
- WebPartRestoreVerb.cs
- AuthenticatingEventArgs.cs
- RadioButtonList.cs
- ThreadStartException.cs
- OperationContractGenerationContext.cs
- QueryPageSettingsEventArgs.cs
- XmlReflectionMember.cs
- DictionaryContent.cs
- ImageSourceValueSerializer.cs
- DeviceContext2.cs