Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / DataOracleClient / System / Data / OracleClient / OciEnlistContext.cs / 1 / OciEnlistContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.Common; using System.Diagnostics; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Threading; using SysTx = System.Transactions; using System.Runtime.ConstrainedExecution; sealed internal class OciEnlistContext : SafeHandle { private OciServiceContextHandle _serviceContextHandle; internal OciEnlistContext(byte[] userName, byte[] password, byte[] serverName, OciServiceContextHandle serviceContextHandle, OciErrorHandle errorHandle) : base(IntPtr.Zero, true) { RuntimeHelpers.PrepareConstrainedRegions(); try {} finally { _serviceContextHandle = serviceContextHandle; int rc = 0; try { rc = TracedNativeMethods.OraMTSEnlCtxGet(userName, password, serverName, _serviceContextHandle, errorHandle, out base.handle); } catch (DllNotFoundException e) { throw ADP.DistribTxRequiresOracleServicesForMTS(e); } if (0 != rc) { OracleException.Check(errorHandle, rc); } // Make sure the transaction context is disposed before the service // context is. serviceContextHandle.AddRef(); } } public override bool IsInvalid { get { return (IntPtr.Zero == base.handle); } } internal void Join(OracleInternalConnection internalConnection, SysTx.Transaction indigoTransaction) { SysTx.IDtcTransaction oleTxTransaction = ADP.GetOletxTransaction(indigoTransaction); int rc = TracedNativeMethods.OraMTSJoinTxn(this, oleTxTransaction); if (0 != rc) { OracleException.Check(rc, internalConnection); } } override protected bool ReleaseHandle() { // NOTE: The SafeHandle class guarantees this will be called exactly once. IntPtr ptr = base.handle; base.handle = IntPtr.Zero; if (IntPtr.Zero != ptr) { TracedNativeMethods.OraMTSEnlCtxRel(ptr); } // OK, now we can release the service context. if (null != _serviceContextHandle) { _serviceContextHandle.Release(); _serviceContextHandle = null; } return true; } internal static void SafeDispose(ref OciEnlistContext ociEnlistContext) { // Safely disposes of the handle (even if it is already null) and // then nulls it out. if (null != ociEnlistContext) { ociEnlistContext.Dispose(); } ociEnlistContext = null; } //--------------------------------------------------------------------- static internal IntPtr HandleValueToTrace (OciEnlistContext handle) { return handle.DangerousGetHandle(); // for tracing purposes, it's safe to just print this -- no handle recycling issues. } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.Common; using System.Diagnostics; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Threading; using SysTx = System.Transactions; using System.Runtime.ConstrainedExecution; sealed internal class OciEnlistContext : SafeHandle { private OciServiceContextHandle _serviceContextHandle; internal OciEnlistContext(byte[] userName, byte[] password, byte[] serverName, OciServiceContextHandle serviceContextHandle, OciErrorHandle errorHandle) : base(IntPtr.Zero, true) { RuntimeHelpers.PrepareConstrainedRegions(); try {} finally { _serviceContextHandle = serviceContextHandle; int rc = 0; try { rc = TracedNativeMethods.OraMTSEnlCtxGet(userName, password, serverName, _serviceContextHandle, errorHandle, out base.handle); } catch (DllNotFoundException e) { throw ADP.DistribTxRequiresOracleServicesForMTS(e); } if (0 != rc) { OracleException.Check(errorHandle, rc); } // Make sure the transaction context is disposed before the service // context is. serviceContextHandle.AddRef(); } } public override bool IsInvalid { get { return (IntPtr.Zero == base.handle); } } internal void Join(OracleInternalConnection internalConnection, SysTx.Transaction indigoTransaction) { SysTx.IDtcTransaction oleTxTransaction = ADP.GetOletxTransaction(indigoTransaction); int rc = TracedNativeMethods.OraMTSJoinTxn(this, oleTxTransaction); if (0 != rc) { OracleException.Check(rc, internalConnection); } } override protected bool ReleaseHandle() { // NOTE: The SafeHandle class guarantees this will be called exactly once. IntPtr ptr = base.handle; base.handle = IntPtr.Zero; if (IntPtr.Zero != ptr) { TracedNativeMethods.OraMTSEnlCtxRel(ptr); } // OK, now we can release the service context. if (null != _serviceContextHandle) { _serviceContextHandle.Release(); _serviceContextHandle = null; } return true; } internal static void SafeDispose(ref OciEnlistContext ociEnlistContext) { // Safely disposes of the handle (even if it is already null) and // then nulls it out. if (null != ociEnlistContext) { ociEnlistContext.Dispose(); } ociEnlistContext = null; } //--------------------------------------------------------------------- static internal IntPtr HandleValueToTrace (OciEnlistContext handle) { return handle.DangerousGetHandle(); // for tracing purposes, it's safe to just print this -- no handle recycling issues. } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
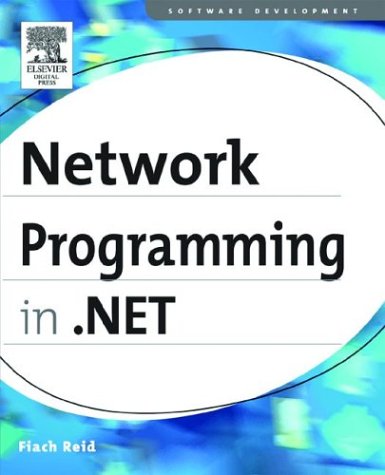
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewColumnCollection.cs
- ShutDownListener.cs
- ArrayTypeMismatchException.cs
- BackgroundFormatInfo.cs
- ProxyHelper.cs
- PersonalizableTypeEntry.cs
- BaseAsyncResult.cs
- MemoryStream.cs
- WebPartTransformerCollection.cs
- IProvider.cs
- EntityConnection.cs
- ShaderEffect.cs
- DockingAttribute.cs
- ZipIOLocalFileBlock.cs
- WFItemsToSpacerVisibility.cs
- ImageSourceValueSerializer.cs
- safex509handles.cs
- SafeNativeMethods.cs
- UnhandledExceptionEventArgs.cs
- ImageMapEventArgs.cs
- SqlNotificationEventArgs.cs
- CacheMemory.cs
- DoubleLinkList.cs
- ItemList.cs
- InputProcessorProfilesLoader.cs
- _CommandStream.cs
- FileNotFoundException.cs
- DoubleConverter.cs
- LambdaCompiler.Statements.cs
- SqlGatherProducedAliases.cs
- ApplicationInfo.cs
- DoubleAnimation.cs
- _LocalDataStoreMgr.cs
- NamespaceQuery.cs
- __FastResourceComparer.cs
- SqlFormatter.cs
- ByteBufferPool.cs
- DataSourceXmlSerializer.cs
- Registry.cs
- MessageSmuggler.cs
- TextModifierScope.cs
- XPathMultyIterator.cs
- ImageInfo.cs
- TypeReference.cs
- WindowsSpinner.cs
- Assembly.cs
- BooleanAnimationUsingKeyFrames.cs
- Positioning.cs
- ParameterReplacerVisitor.cs
- CatalogPart.cs
- SchemaManager.cs
- FileUtil.cs
- PointAnimationUsingKeyFrames.cs
- DataSourceControlBuilder.cs
- EntityContainerEmitter.cs
- ServiceHostFactory.cs
- Grant.cs
- StatusBarAutomationPeer.cs
- List.cs
- ListChangedEventArgs.cs
- IpcPort.cs
- InternalConfigHost.cs
- SchemaCollectionPreprocessor.cs
- BamlResourceSerializer.cs
- DocumentSequence.cs
- Comparer.cs
- TextProperties.cs
- GeometryModel3D.cs
- TaskFormBase.cs
- CodeLinePragma.cs
- RuleConditionDialog.cs
- SourceFileInfo.cs
- SafeCertificateStore.cs
- IDQuery.cs
- WindowsGraphics2.cs
- XmlBaseWriter.cs
- WpfGeneratedKnownProperties.cs
- SpecularMaterial.cs
- PassportPrincipal.cs
- Point4D.cs
- SecurityManager.cs
- BaseCodeDomTreeGenerator.cs
- FormViewModeEventArgs.cs
- ReverseQueryOperator.cs
- SecurityDescriptor.cs
- GifBitmapEncoder.cs
- hresults.cs
- ScriptServiceAttribute.cs
- LayoutEditorPart.cs
- cookiecontainer.cs
- IgnoreSectionHandler.cs
- CrossContextChannel.cs
- IOThreadScheduler.cs
- PocoEntityKeyStrategy.cs
- UnionCodeGroup.cs
- DataColumnMappingCollection.cs
- ListDictionaryInternal.cs
- JsonWriter.cs
- TagPrefixCollection.cs
- TemplatedWizardStep.cs