Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / View / WindowExtensionMethods.cs / 1305376 / WindowExtensionMethods.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.Runtime.InteropServices; using System.Windows; using System.Windows.Interop; static class WindowExtensionMethods { public static void ShowContextHelpButton(this Window window) { IntPtr hwnd = new WindowInteropHelper(window).Handle; IntPtr exStyle = Win32Interop.GetWindowLongPtr(hwnd, Win32Interop.GWL_EXSTYLE); if (IntPtr.Size == 4) { exStyle = new IntPtr(exStyle.ToInt32() | Win32Interop.WS_EX_CONTEXTHELP); } else { exStyle = new IntPtr(exStyle.ToInt64() | ((long)Win32Interop.WS_EX_CONTEXTHELP)); } Win32Interop.SetWindowLongPtr(new HandleRef(window, hwnd), Win32Interop.GWL_EXSTYLE, exStyle); } public static void HideMinMaxButton(this Window window) { IntPtr hwnd = new WindowInteropHelper(window).Handle; IntPtr style = Win32Interop.GetWindowLongPtr(hwnd, Win32Interop.GWL_STYLE); if (IntPtr.Size == 4) { int intValue = style.ToInt32(); intValue = SetBit(Win32Interop.WS_MAXIMIZEBOX, intValue, false); intValue = SetBit(Win32Interop.WS_MINIMIZEBOX, intValue, false); style = new IntPtr(intValue); } else { long longValue = style.ToInt64(); longValue = SetBit((long)Win32Interop.WS_MAXIMIZEBOX, longValue, false); longValue = SetBit((long)Win32Interop.WS_MINIMIZEBOX, longValue, false); style = new IntPtr(longValue); } Win32Interop.SetWindowLongPtr(new HandleRef(window, hwnd), Win32Interop.GWL_STYLE, style); } public static void AddWindowsHook(this Window window, HwndSourceHook wmHandler) { IntPtr hwnd = new WindowInteropHelper(window).Handle; HwndSource source = HwndSource.FromHwnd(hwnd); source.AddHook(wmHandler); } public static void RemoveWindowsHook(this Window window, HwndSourceHook wmHandler) { IntPtr hwnd = new WindowInteropHelper(window).Handle; HwndSource source = HwndSource.FromHwnd(hwnd); source.RemoveHook(wmHandler); } public static void HideIcon(this Window window) { IntPtr hwnd = new WindowInteropHelper(window).Handle; IntPtr exStyle = Win32Interop.GetWindowLongPtr(hwnd, Win32Interop.GWL_EXSTYLE); if (IntPtr.Size == 4) { exStyle = new IntPtr(exStyle.ToInt32() | Win32Interop.WS_EX_DLGMODALFRAME); } else { exStyle = new IntPtr(exStyle.ToInt64() | ((long)Win32Interop.WS_EX_DLGMODALFRAME)); } Win32Interop.SetWindowLongPtr(new HandleRef(window, hwnd), Win32Interop.GWL_EXSTYLE, exStyle); Win32Interop.SendMessage(hwnd, Win32Interop.WM_SETICON, new IntPtr(Win32Interop.ICON_SMALL), IntPtr.Zero); Win32Interop.SendMessage(hwnd, Win32Interop.WM_SETICON, new IntPtr(Win32Interop.ICON_BIG), IntPtr.Zero); } private static long SetBit(long mask, long value, bool flag) { if (flag) { return value | mask; } else { return value & ~mask; } } private static int SetBit(int mask, int value, bool flag) { if (flag) { return value | mask; } else { return value & ~mask; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.Runtime.InteropServices; using System.Windows; using System.Windows.Interop; static class WindowExtensionMethods { public static void ShowContextHelpButton(this Window window) { IntPtr hwnd = new WindowInteropHelper(window).Handle; IntPtr exStyle = Win32Interop.GetWindowLongPtr(hwnd, Win32Interop.GWL_EXSTYLE); if (IntPtr.Size == 4) { exStyle = new IntPtr(exStyle.ToInt32() | Win32Interop.WS_EX_CONTEXTHELP); } else { exStyle = new IntPtr(exStyle.ToInt64() | ((long)Win32Interop.WS_EX_CONTEXTHELP)); } Win32Interop.SetWindowLongPtr(new HandleRef(window, hwnd), Win32Interop.GWL_EXSTYLE, exStyle); } public static void HideMinMaxButton(this Window window) { IntPtr hwnd = new WindowInteropHelper(window).Handle; IntPtr style = Win32Interop.GetWindowLongPtr(hwnd, Win32Interop.GWL_STYLE); if (IntPtr.Size == 4) { int intValue = style.ToInt32(); intValue = SetBit(Win32Interop.WS_MAXIMIZEBOX, intValue, false); intValue = SetBit(Win32Interop.WS_MINIMIZEBOX, intValue, false); style = new IntPtr(intValue); } else { long longValue = style.ToInt64(); longValue = SetBit((long)Win32Interop.WS_MAXIMIZEBOX, longValue, false); longValue = SetBit((long)Win32Interop.WS_MINIMIZEBOX, longValue, false); style = new IntPtr(longValue); } Win32Interop.SetWindowLongPtr(new HandleRef(window, hwnd), Win32Interop.GWL_STYLE, style); } public static void AddWindowsHook(this Window window, HwndSourceHook wmHandler) { IntPtr hwnd = new WindowInteropHelper(window).Handle; HwndSource source = HwndSource.FromHwnd(hwnd); source.AddHook(wmHandler); } public static void RemoveWindowsHook(this Window window, HwndSourceHook wmHandler) { IntPtr hwnd = new WindowInteropHelper(window).Handle; HwndSource source = HwndSource.FromHwnd(hwnd); source.RemoveHook(wmHandler); } public static void HideIcon(this Window window) { IntPtr hwnd = new WindowInteropHelper(window).Handle; IntPtr exStyle = Win32Interop.GetWindowLongPtr(hwnd, Win32Interop.GWL_EXSTYLE); if (IntPtr.Size == 4) { exStyle = new IntPtr(exStyle.ToInt32() | Win32Interop.WS_EX_DLGMODALFRAME); } else { exStyle = new IntPtr(exStyle.ToInt64() | ((long)Win32Interop.WS_EX_DLGMODALFRAME)); } Win32Interop.SetWindowLongPtr(new HandleRef(window, hwnd), Win32Interop.GWL_EXSTYLE, exStyle); Win32Interop.SendMessage(hwnd, Win32Interop.WM_SETICON, new IntPtr(Win32Interop.ICON_SMALL), IntPtr.Zero); Win32Interop.SendMessage(hwnd, Win32Interop.WM_SETICON, new IntPtr(Win32Interop.ICON_BIG), IntPtr.Zero); } private static long SetBit(long mask, long value, bool flag) { if (flag) { return value | mask; } else { return value & ~mask; } } private static int SetBit(int mask, int value, bool flag) { if (flag) { return value | mask; } else { return value & ~mask; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
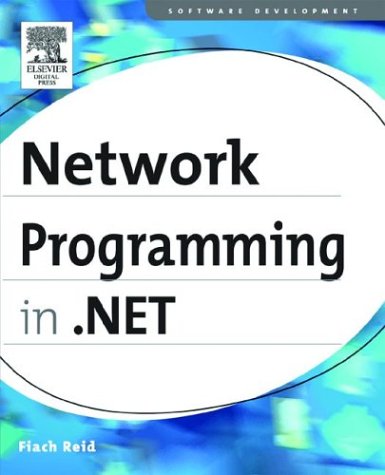
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Exception.cs
- Assert.cs
- EmptyStringExpandableObjectConverter.cs
- FormsAuthenticationEventArgs.cs
- ListViewDeletedEventArgs.cs
- HyperlinkAutomationPeer.cs
- DbDataAdapter.cs
- RightsManagementEncryptionTransform.cs
- StyleReferenceConverter.cs
- CursorInteropHelper.cs
- ProtectedProviderSettings.cs
- ConnectorSelectionGlyph.cs
- DocumentCollection.cs
- MultiByteCodec.cs
- ConstantCheck.cs
- DefaultValueTypeConverter.cs
- StoreAnnotationsMap.cs
- SqlDataSource.cs
- ExpressionPrinter.cs
- UnsafeNativeMethods.cs
- DATA_BLOB.cs
- StickyNoteAnnotations.cs
- XmlSerializableServices.cs
- TextBoxAutomationPeer.cs
- BitmapEffectInput.cs
- ActivityContext.cs
- _AcceptOverlappedAsyncResult.cs
- mediaeventshelper.cs
- DataKey.cs
- StateMachineDesignerPaint.cs
- MessagePropertyVariants.cs
- UntypedNullExpression.cs
- WSFederationHttpBindingElement.cs
- StrongNameIdentityPermission.cs
- XmlEncodedRawTextWriter.cs
- BamlCollectionHolder.cs
- SmtpMail.cs
- SqlDataSourceCommandEventArgs.cs
- WorkflowRuntimeServicesBehavior.cs
- __Filters.cs
- Table.cs
- FormViewUpdateEventArgs.cs
- WindowsImpersonationContext.cs
- ShortcutKeysEditor.cs
- ProfileEventArgs.cs
- StylusShape.cs
- MaterialGroup.cs
- XmlPreloadedResolver.cs
- HttpCapabilitiesBase.cs
- SafeNativeMethods.cs
- SQLRoleProvider.cs
- ReferenceSchema.cs
- TypeDependencyAttribute.cs
- DataGridViewMethods.cs
- DebugInfo.cs
- CodeLabeledStatement.cs
- ExternalFile.cs
- RawStylusActions.cs
- ParentUndoUnit.cs
- CompilerGeneratedAttribute.cs
- HttpHandlerActionCollection.cs
- SafeLocalAllocation.cs
- StructuralComparisons.cs
- ACL.cs
- Enum.cs
- UIElement3D.cs
- ServiceAuthorizationManager.cs
- ProcessHostServerConfig.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- MetafileHeader.cs
- OdbcCommandBuilder.cs
- SelectionList.cs
- IndexedEnumerable.cs
- ISCIIEncoding.cs
- DependencyObjectProvider.cs
- RegexWorker.cs
- XslUrlEditor.cs
- ImmutableObjectAttribute.cs
- MailDefinition.cs
- SimpleLine.cs
- DisplayMemberTemplateSelector.cs
- LicenseException.cs
- StylusPlugin.cs
- DateTimeOffsetStorage.cs
- LocalizationParserHooks.cs
- CacheDependency.cs
- DES.cs
- MailHeaderInfo.cs
- InstanceKey.cs
- SelectionGlyph.cs
- BufferBuilder.cs
- ExpressionConverter.cs
- DbProviderFactory.cs
- DataTableClearEvent.cs
- BuilderInfo.cs
- SocketPermission.cs
- ProjectionPathSegment.cs
- NestedContainer.cs
- BamlReader.cs
- PackageDigitalSignature.cs