Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / Dom / XmlProcessingInstruction.cs / 1 / XmlProcessingInstruction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.IO; using System.Diagnostics; using System.Text; using System.Xml.XPath; // Represents a processing instruction, which XML defines to keep // processor-specific information in the text of the document. public class XmlProcessingInstruction : XmlLinkedNode { string target; string data; protected internal XmlProcessingInstruction( string target, string data, XmlDocument doc ) : base( doc ) { this.target = target; this.data = data; } // Gets the name of the node. public override String Name { get { if (target != null) return target; return String.Empty; } } // Gets the name of the current node without the namespace prefix. public override string LocalName { get { return Name;} } // Gets or sets the value of the node. public override String Value { get { return data;} set { Data = value; } //use Data instead of data so that event will be fired } // Gets the target of the processing instruction. public String Target { get { return target;} } // Gets or sets the content of processing instruction, // excluding the target. public String Data { get { return data;} set { XmlNode parent = ParentNode; XmlNodeChangedEventArgs args = GetEventArgs( this, parent, parent, data, value, XmlNodeChangedAction.Change ); if (args != null) BeforeEvent( args ); data = value; if (args != null) AfterEvent( args ); } } // Gets or sets the concatenated values of the node and // all its children. public override string InnerText { get { return data;} set { Data = value; } //use Data instead of data so that change event will be fired } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.ProcessingInstruction;} } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateProcessingInstruction( target, data ); } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteProcessingInstruction(target, data); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing } internal override string XPLocalName { get { return Name; } } internal override XPathNodeType XPNodeType { get { return XPathNodeType.ProcessingInstruction; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.IO; using System.Diagnostics; using System.Text; using System.Xml.XPath; // Represents a processing instruction, which XML defines to keep // processor-specific information in the text of the document. public class XmlProcessingInstruction : XmlLinkedNode { string target; string data; protected internal XmlProcessingInstruction( string target, string data, XmlDocument doc ) : base( doc ) { this.target = target; this.data = data; } // Gets the name of the node. public override String Name { get { if (target != null) return target; return String.Empty; } } // Gets the name of the current node without the namespace prefix. public override string LocalName { get { return Name;} } // Gets or sets the value of the node. public override String Value { get { return data;} set { Data = value; } //use Data instead of data so that event will be fired } // Gets the target of the processing instruction. public String Target { get { return target;} } // Gets or sets the content of processing instruction, // excluding the target. public String Data { get { return data;} set { XmlNode parent = ParentNode; XmlNodeChangedEventArgs args = GetEventArgs( this, parent, parent, data, value, XmlNodeChangedAction.Change ); if (args != null) BeforeEvent( args ); data = value; if (args != null) AfterEvent( args ); } } // Gets or sets the concatenated values of the node and // all its children. public override string InnerText { get { return data;} set { Data = value; } //use Data instead of data so that change event will be fired } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.ProcessingInstruction;} } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateProcessingInstruction( target, data ); } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteProcessingInstruction(target, data); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing } internal override string XPLocalName { get { return Name; } } internal override XPathNodeType XPNodeType { get { return XPathNodeType.ProcessingInstruction; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
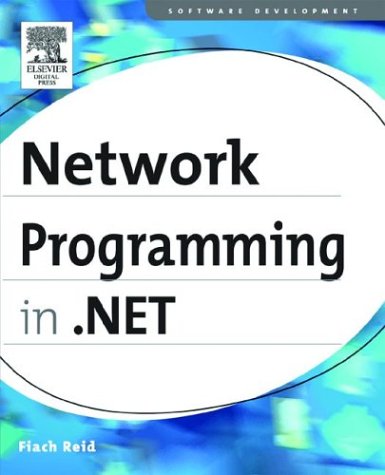
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GenericTextProperties.cs
- Point3DCollectionValueSerializer.cs
- NullableLongSumAggregationOperator.cs
- X509PeerCertificateAuthentication.cs
- ZipIOLocalFileBlock.cs
- MultiPageTextView.cs
- AppSettingsSection.cs
- RMEnrollmentPage1.cs
- SafeBuffer.cs
- WebServiceHandler.cs
- RC2CryptoServiceProvider.cs
- XmlAnyElementAttribute.cs
- Sequence.cs
- ReservationCollection.cs
- InputReport.cs
- Literal.cs
- HyperLinkColumn.cs
- ContainerControl.cs
- InvalidOperationException.cs
- SelectionProcessor.cs
- PartialTrustValidationBehavior.cs
- SecurityState.cs
- XmlEncoding.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- FixedPageStructure.cs
- RuleConditionDialog.Designer.cs
- XPathChildIterator.cs
- Stopwatch.cs
- DefaultObjectSerializer.cs
- RegistrationServices.cs
- ControlPaint.cs
- UnsafeNativeMethods.cs
- EdmToObjectNamespaceMap.cs
- TypeNameConverter.cs
- XPathNavigator.cs
- RoleManagerEventArgs.cs
- HTMLTagNameToTypeMapper.cs
- CopyNamespacesAction.cs
- BitmapDecoder.cs
- IconConverter.cs
- PieceNameHelper.cs
- TimelineClockCollection.cs
- ComEventsMethod.cs
- EntityCommand.cs
- Simplifier.cs
- IgnoreDataMemberAttribute.cs
- util.cs
- ScrollChangedEventArgs.cs
- WhitespaceRuleReader.cs
- CompilerGeneratedAttribute.cs
- UInt16Converter.cs
- PolicyValidationException.cs
- SchemaMerger.cs
- XmlSchemas.cs
- DataGridCellAutomationPeer.cs
- OdbcTransaction.cs
- PiiTraceSource.cs
- ProviderSettingsCollection.cs
- LoginUtil.cs
- HttpListenerContext.cs
- panel.cs
- RightsManagementPermission.cs
- Internal.cs
- CorrelationActionMessageFilter.cs
- BaseConfigurationRecord.cs
- TraceFilter.cs
- ErrorLog.cs
- AssociationEndMember.cs
- TableLayoutPanel.cs
- Rotation3D.cs
- ContainerCodeDomSerializer.cs
- AVElementHelper.cs
- Decoder.cs
- ReadOnlyDataSource.cs
- Odbc32.cs
- Section.cs
- SymbolTable.cs
- XmlFormatWriterGenerator.cs
- SerializerWriterEventHandlers.cs
- XmlNodeComparer.cs
- SoapElementAttribute.cs
- DodSequenceMerge.cs
- MetadataCollection.cs
- InkCanvasInnerCanvas.cs
- XsdBuildProvider.cs
- ellipse.cs
- CachedRequestParams.cs
- PropagationProtocolsTracing.cs
- XDRSchema.cs
- CachedTypeface.cs
- Matrix.cs
- PathParser.cs
- ReferenceConverter.cs
- TypeLoadException.cs
- CollectionType.cs
- StaticExtension.cs
- Visual3DCollection.cs
- TypeDependencyAttribute.cs
- BaseAsyncResult.cs
- TriggerActionCollection.cs